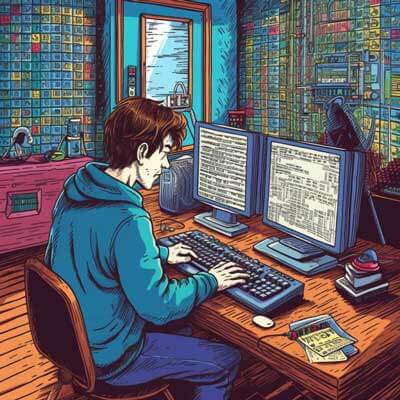
- Advantages of Positioning WHERE Clause After JOINs
- Implementing WHERE Clause After JOINs
- Best Practices for Using WHERE Clause After JOINs
- Common Mistakes to Avoid When Using WHERE Clause After JOINs
- Code Snippet: Positioning WHERE Clause After JOINs in SQL
- Troubleshooting Tips for WHERE Clause After JOINs
- Understanding Joins in SQL
- Exploring Inner Joins
- Understanding Outer Joins
- Understanding Right Joins
- Exploring Full Joins
- Additional Resources
Advantages of Positioning WHERE Clause After JOINs
In SQL, the WHERE clause is used to filter the rows returned by a query based on specified conditions. The standard practice is to position the WHERE clause after the JOINs in a query. This has several advantages:
1. Improved Performance: Placing the WHERE clause after the JOINs allows the database engine to optimize the query execution plan. By joining the tables first and then applying the filtering conditions, the database engine can make better use of indexes and reduce the number of rows that need to be processed.
2. Ease of Readability: By positioning the WHERE clause after the JOINs, the query becomes more readable and easier to understand. The JOINs define the relationships between the tables, and the WHERE clause specifies the conditions for filtering the data. This separation of concerns makes the query easier to comprehend and maintain.
3. Flexibility in Query Building: Placing the WHERE clause after the JOINs provides more flexibility in constructing complex queries. It allows you to specify conditions that involve multiple tables and take advantage of the relationships defined by the JOINs. This flexibility is particularly useful when working with larger databases or when dealing with complex business logic.
Related Article: Tutorial: ON for JOIN SQL in Databases
Implementing WHERE Clause After JOINs
To implement the WHERE clause after the JOINs in a SQL query, follow these steps:
1. Start by writing the SELECT statement and specify the columns you want to retrieve from the tables involved in the query.
2. Use the JOIN keyword to combine the tables based on the related columns.
3. Specify the join conditions using the ON keyword, which defines how the columns in the tables are related.
4. After the JOINs, add the WHERE clause to filter the rows based on the desired conditions.
Here’s an example that demonstrates the implementation of the WHERE clause after the JOINs:
SELECT Customers.customer_name, Orders.order_number FROM Customers <a href="https://www.squash.io/tutorial-the-functionality-of-inner-join-in-sql/">INNER JOIN</a> Orders ON Customers.customer_id = Orders.customer_id WHERE Customers.customer_country = 'USA';
In this example, we are retrieving the customer name and order number from the “Customers” and “Orders” tables, respectively. We are joining the tables based on the “customer_id” column. After the JOIN, we add the WHERE clause to filter the customers based on their country of origin.
Best Practices for Using WHERE Clause After JOINs
When using the WHERE clause after the JOINs in SQL, it is important to follow some best practices to ensure efficient and readable code:
1. Use meaningful table aliases: When joining multiple tables, use aliases for table names to make the query more readable. This helps to avoid confusion when referencing columns from different tables in the WHERE clause.
2. Be specific in conditions: Be specific in the conditions used in the WHERE clause to filter the rows. Avoid using generic conditions that could potentially match a large number of rows. This helps to improve query performance and reduce the risk of retrieving unnecessary data.
3. Use parentheses for clarity: If a query involves multiple JOINs and conditions, use parentheses to group related conditions together. This helps to improve the readability and clarity of the query.
4. Consider the order of JOINs: The order in which tables are joined can impact query performance. Consider the size of the tables and the selectivity of the conditions when deciding the order of JOINs. Joining smaller tables first can often improve performance.
5. Use appropriate indexes: Ensure that the columns used in the JOIN conditions and the WHERE clause are indexed. Indexes can significantly improve query performance by allowing the database engine to quickly locate the relevant rows.
Common Mistakes to Avoid When Using WHERE Clause After JOINs
When using the WHERE clause after the JOINs in SQL, there are some common mistakes that developers should avoid:
1. Incorrect join conditions: Ensure that the join conditions are accurate and correctly specify the relationship between the tables. Incorrect join conditions can lead to unexpected results or missing data.
2. Missing table aliases: When using table aliases, make sure to include them in the column references in the WHERE clause. Forgetting to include aliases can cause syntax errors or lead to ambiguous column references.
3. Confusing AND and OR operators: Be careful when using multiple conditions in the WHERE clause, especially when mixing AND and OR operators. Incorrectly grouping conditions can produce unexpected results or alter the intended logic of the query.
4. Neglecting to use parentheses: When a query involves multiple JOINs and conditions, failing to use parentheses to group related conditions can lead to unexpected results. Always use parentheses to clearly define the logical grouping of conditions.
5. Ignoring query optimization: Optimizing the query execution plan is crucial for improving performance. Neglecting to consider indexing, table order, or condition selectivity can result in slow query performance.
Related Article: Analyzing SQL Join and Its Effect on Records
Code Snippet: Positioning WHERE Clause After JOINs in SQL
Here’s an example of a code snippet that demonstrates the positioning of the WHERE clause after JOINs in a SQL query:
SELECT Customers.customer_name, Orders.order_number FROM Customers INNER JOIN Orders ON Customers.customer_id = Orders.customer_id WHERE Customers.customer_country = 'USA';
In this example, we are selecting the customer name from the “Customers” table and the order number from the “Orders” table. We are joining the tables based on the “customer_id” column and using the WHERE clause to filter the customers from the USA.
Troubleshooting Tips for WHERE Clause After JOINs
When using the WHERE clause after the JOINs in SQL, you may encounter some common issues. Here are some troubleshooting tips to help you resolve them:
1. Syntax errors: Double-check the syntax of your query, especially the placement of JOINs, ON conditions, and the WHERE clause. Syntax errors can occur if these elements are not correctly positioned or if they contain typographical errors.
2. Ambiguous column references: If you receive an error message regarding ambiguous column references, ensure that you are referencing the columns using the appropriate table aliases or fully qualified table names. Ambiguity can occur if multiple tables have columns with the same name.
3. Missing or incorrect join conditions: Check the join conditions to ensure they correctly specify the relationship between the tables. Missing or incorrect join conditions can lead to unexpected results or missing data.
4. Incorrectly grouped conditions: Review the grouping of conditions in the WHERE clause to ensure logical consistency. Incorrect grouping can alter the intended logic of the query and produce unexpected results.
5. Slow query performance: If your query is running slowly, consider optimizing the query execution plan. Ensure that the relevant columns used in the JOINs and WHERE clause are properly indexed. Also, evaluate the order of JOINs and the selectivity of conditions to improve query performance.
Understanding Joins in SQL
Joins are used to combine data from two or more tables based on a related column between them. By using joins, we can retrieve data that is spread across multiple tables and create a virtual table that contains all the required information.
There are several types of joins in SQL, including inner joins, outer joins, left joins, right joins, and full joins. Each type of join serves a specific purpose and allows us to retrieve different sets of data.
Related Article: Tutorial: Full Outer Join versus Join in SQL
Exploring Inner Joins
Inner joins return only the rows that have matching values in both tables being joined. It combines rows from two or more tables based on a related column between them.
Let’s consider an example where we have two tables: “Customers” and “Orders”. The “Customers” table contains customer information, and the “Orders” table contains order information. Both tables have a common column, which is the “customer_id” column.
SELECT Customers.customer_name, Orders.order_number FROM Customers INNER JOIN Orders ON Customers.customer_id = Orders.customer_id;
In this example, we are selecting the “customer_name” column from the “Customers” table and the “order_number” column from the “Orders” table. The INNER JOIN keyword is used to combine the two tables based on the “customer_id” column.
This query will return only the rows where there is a match between the “customer_id” column in the “Customers” table and the “customer_id” column in the “Orders” table.
Understanding Outer Joins
Outer joins return all rows from one table and the matching rows from the other table(s). If there is no match, NULL values are returned for the columns of the table that does not have a matching row.
There are three types of outer joins: left join, right join, and full join.
Exploring Left Joins
A left join returns all rows from the left table and the matching rows from the right table. If there is no match, NULL values are returned for the columns of the right table.
Let’s consider an example where we have two tables: “Customers” and “Orders”. The “Customers” table contains customer information, and the “Orders” table contains order information. Both tables have a common column, which is the “customer_id” column.
SELECT Customers.customer_name, Orders.order_number FROM Customers LEFT JOIN Orders ON Customers.customer_id = Orders.customer_id;
In this example, we are selecting the “customer_name” column from the “Customers” table and the “order_number” column from the “Orders” table. The LEFT JOIN keyword is used to combine the two tables based on the “customer_id” column.
This query will return all rows from the “Customers” table, regardless of whether there is a match in the “Orders” table. If there is no match, NULL values will be returned for the “order_number” column.
Understanding Right Joins
A right join returns all rows from the right table and the matching rows from the left table. If there is no match, NULL values are returned for the columns of the left table.
Let’s consider an example where we have two tables: “Customers” and “Orders”. The “Customers” table contains customer information, and the “Orders” table contains order information. Both tables have a common column, which is the “customer_id” column.
SELECT Customers.customer_name, Orders.order_number FROM Customers RIGHT JOIN Orders ON Customers.customer_id = Orders.customer_id;
In this example, we are selecting the “customer_name” column from the “Customers” table and the “order_number” column from the “Orders” table. The RIGHT JOIN keyword is used to combine the two tables based on the “customer_id” column.
This query will return all rows from the “Orders” table, regardless of whether there is a match in the “Customers” table. If there is no match, NULL values will be returned for the “customer_name” column.
Related Article: Exploring SQL Join Conditions: The Role of Primary Keys
Exploring Full Joins
A full join returns all rows from both tables, regardless of whether there is a match or not. If there is no match, NULL values are returned for the columns of the table(s) that do not have a matching row.
Let’s consider an example where we have two tables: “Customers” and “Orders”. The “Customers” table contains customer information, and the “Orders” table contains order information. Both tables have a common column, which is the “customer_id” column.
SELECT Customers.customer_name, Orders.order_number FROM Customers FULL JOIN Orders ON Customers.customer_id = Orders.customer_id;
In this example, we are selecting the “customer_name” column from the “Customers” table and the “order_number” column from the “Orders” table. The FULL JOIN keyword is used to combine the two tables based on the “customer_id” column.
This query will return all rows from both the “Customers” and “Orders” tables, regardless of whether there is a match or not. If there is no match, NULL values will be returned for the columns of the table(s) that do not have a matching row.