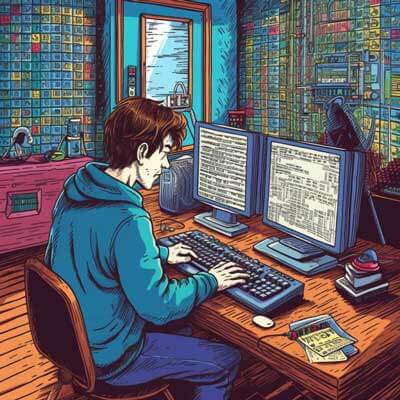
- SQL Query Optimization Techniques
- Code Snippet: Implementing a Cross Join in SQL
- Understanding the Inner Join in SQL
- Exploring the Outer Join in SQL
- Code Snippet: Implementing an Outer Join in SQL
- Code Snippet: Using SQL to Join Multiple Tables
- Optimizing Query Performance in SQL
- Additional Resources
SQL Query Optimization Techniques
When working with SQL databases, it is important to optimize your queries to ensure efficient and fast data retrieval. SQL query optimization techniques involve analyzing and modifying queries to improve their performance. Here are a few commonly used techniques:
1. Use Indexes: Indexes are data structures that improve the speed of data retrieval operations. By creating indexes on columns frequently used in queries, you can significantly reduce the time it takes to fetch data. For example, if you often search for records based on a customer’s last name, you can create an index on the “last_name” column to speed up these queries.
Example: Creating an index on the “last_name” column in a table named “customers”.
CREATE INDEX idx_last_name ON customers (last_name);
2. Avoid SELECT *: Instead of selecting all columns from a table using the wildcard (*) operator, specify only the required columns. This reduces the amount of data returned and improves query performance.
Example: Selecting specific columns from a table named “products.
SELECT product_name, price FROM products;
3. Use Joins Efficiently: Joins are useful operations in SQL, but they can also impact query performance. Use appropriate join types (inner join, outer join, etc.) based on your data requirements. Additionally, ensure that you join tables using indexed columns to improve performance.
Example: Joining two tables named “orders” and “customers” based on the “customer_id” column.
SELECT o.order_id, c.customer_name FROM orders o INNER JOIN customers c ON o.customer_id = c.customer_id;
4. Limit the Result Set: If you only need a subset of the data, use the LIMIT clause to restrict the number of rows returned. This can significantly improve query performance, especially for large tables.
Example: Selecting the top 10 rows from a table named “employees”.
SELECT * FROM employees LIMIT 10;
Related Article: Resolving Scalar Join Issues with SQL Tables in Databases
Code Snippet: Implementing a Cross Join in SQL
In SQL, a cross join (also known as a Cartesian join) returns the Cartesian product of two or more tables. It combines each row from the first table with every row from the second table, resulting in a large result set. Here’s an example of implementing a cross join in SQL:
SELECT * FROM table1 CROSS JOIN table2;
This query will return all possible combinations of rows from table1 and table2.
Understanding the Inner Join in SQL
An inner join is one of the most commonly used join types in SQL. It returns only the rows that have matching values in both tables being joined. Here’s an example of using an inner join in SQL:
SELECT * FROM table1 INNER JOIN table2 ON table1.column = table2.column;
In this example, the rows from table1 and table2 will be joined based on the matching values in the specified columns.
Exploring the Outer Join in SQL
An outer join is used to combine rows from two tables, even if there are no matching values. It returns all the rows from one table and the matching rows from the other table. If there are no matching rows, NULL values are returned. Here’s an example of using an outer join in SQL:
SELECT * FROM table1 LEFT/RIGHT/FULL OUTER JOIN table2 ON table1.column = table2.column;
In this example, the left/ right/ full outer join will return all rows from table1 and the matching rows from table2.
Related Article: How to Use Alias Field Joining in SQL
Code Snippet: Implementing an Outer Join in SQL
In SQL, an outer join can be implemented using the LEFT, RIGHT, or FULL keywords. Here’s an example of implementing a left outer join in SQL:
SELECT * FROM table1 LEFT JOIN table2 ON table1.column = table2.column;
This query will return all rows from table1 and the matching rows from table2. If there are no matching rows, NULL values will be returned for the columns of table2.
Code Snippet: Using SQL to Join Multiple Tables
In SQL, you can join multiple tables to retrieve data from related tables. Here’s an example of joining three tables using SQL:
SELECT * FROM table1 INNER JOIN table2 ON table1.column = table2.column INNER JOIN table3 ON table2.column = table3.column;
This query will join table1, table2, and table3 based on their common columns and return the result set.
Optimizing Query Performance in SQL
Query performance optimization is essential for improving the speed and efficiency of SQL queries. Here are some techniques to optimize query performance:
1. Use Indexes: Create indexes on columns frequently used in queries to speed up data retrieval.
Example: Creating an index on the “product_name” column in a table named “products”.
CREATE INDEX idx_product_name ON products (product_name);
2. Avoid Subqueries: Instead of using subqueries, try to rewrite the query using joins or other techniques to improve performance.
Example: Rewriting a subquery using a join.
SELECT * FROM orders WHERE customer_id IN (SELECT customer_id FROM customers);
Rewritten using a join:
SELECT o.* FROM orders o INNER JOIN customers c ON o.customer_id = c.customer_id;
3. Limit the Result Set: Use the LIMIT or TOP clause to restrict the number of rows returned, especially for large tables.
Example: Selecting the top 10 rows from a table named “employees”.
SELECT * FROM employees LIMIT 10;
4. Normalize Database Schema: Normalize the database schema to reduce data duplication and improve query performance.
5. Use Stored Procedures: Use stored procedures to precompile and optimize frequently executed queries.
Related Article: How to Use Nested Queries in Databases
Additional Resources
– SQL Tutorial – Learn SQL
– SQL – Wikipedia
– What is SQL? – W3Schools