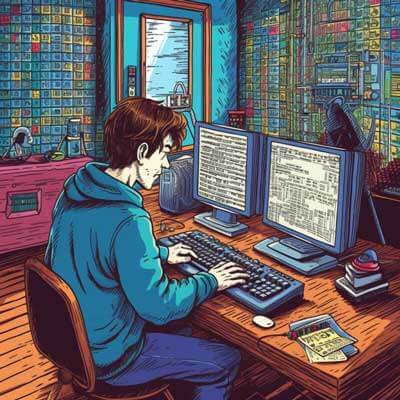
Table of Contents
Joining Two Result Values in SQL
SQL is a useful language for managing and manipulating data in relational databases. One common task in SQL is joining two result values, which allows you to combine the results of two separate queries into a single result set. This can be useful when you need to analyze data from multiple sources or when you want to combine related information from different tables.
There are several ways to join two result values in SQL, depending on the specific requirements of your query. In this article, we will explore different approaches and provide practical examples to help you understand how to effectively join two result values in SQL.
Related Article: Using Stored Procedures in MySQL
The Syntax for Joining Two Result Value Queries in SQL
To join two result value queries in SQL, you can use the JOIN keyword along with the appropriate join type. The basic syntax for joining two result values is as follows:
SELECT column1, column2, ... FROM table1 JOIN table2 ON condition;
In this syntax, table1 and table2 are the names of the tables you want to join. The condition specifies how the two tables are related, and it is typically based on one or more column values. The SELECT statement specifies the columns you want to include in the result set.
Combining the Results of Two Queries in SQL
Joining two result values in SQL allows you to combine the results of two separate queries into a single result set. This can be achieved using the appropriate join type and condition.
Let's consider an example to illustrate how to combine the results of two queries using a join. Suppose we have two tables: customers and orders. The customers table contains information about customers, such as their names and email addresses. The orders table contains information about orders, such as the order ID and the customer ID.
We can join these two tables to get a result set that includes both the customer information and the corresponding order information. The following SQL query demonstrates how to achieve this using an INNER JOIN:
SELECT customers.name, orders.order_id FROM customers INNER JOIN orders ON customers.customer_id = orders.customer_id;
In this example, we are selecting the customer's name from the customers table and the order ID from the orders table. We are joining the two tables based on the customer ID, which is a common column between the two tables.
Different Types of Joins in SQL
In SQL, there are different types of joins that you can use to combine result values from two or more tables. Each join type has its own characteristics and use cases. The most commonly used join types are:
- INNER JOIN: Returns only the rows that have matching values in both tables.
- LEFT JOIN: Returns all the rows from the left table and the matching rows from the right table. If there is no match, NULL values are returned for the right table columns.
- RIGHT JOIN: Returns all the rows from the right table and the matching rows from the left table. If there is no match, NULL values are returned for the left table columns.
- FULL JOIN: Returns all the rows from both tables, regardless of whether there is a match or not. If there is no match, NULL values are returned for the columns of the table that does not have a match.
Related Article: Step-by-Step Process to Uninstall PostgreSQL on Ubuntu
Example of Using INNER JOIN in SQL
To further illustrate the concept of joining two result values in SQL, let's consider an example using the INNER JOIN.
Suppose we have two tables: employees and departments. The employees table contains information about employees, such as their names and job titles. The departments table contains information about departments, such as the department ID and the department name.
We can join these two tables to get a result set that includes both the employee information and the corresponding department information. The following SQL query demonstrates how to achieve this using an INNER JOIN:
SELECT employees.name, departments.department_name FROM employees INNER JOIN departments ON employees.department_id = departments.department_id;
In this example, we are selecting the employee's name from the employees table and the department name from the departments table. We are joining the two tables based on the department ID, which is a common column between the two tables.
Purpose of Using LEFT JOIN in SQL
The LEFT JOIN in SQL is used to return all the rows from the left table and the matching rows from the right table. If there is no match, NULL values are returned for the right table columns.
The purpose of using a LEFT JOIN is to include all the rows from the left table, regardless of whether there is a match in the right table. This can be useful when you want to include all the records from one table and only the matching records from another table.
Let's consider an example to better understand the purpose of using a LEFT JOIN. Suppose we have two tables: customers and orders. The customers table contains information about customers, such as their names and email addresses. The orders table contains information about orders, such as the order ID and the customer ID.
We can use a LEFT JOIN to get a result set that includes all the customers and their corresponding orders, if any. The following SQL query demonstrates how to achieve this using a LEFT JOIN:
SELECT customers.name, orders.order_id FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id;
In this example, we are selecting the customer's name from the customers table and the order ID from the orders table. We are using a LEFT JOIN to include all the customers and their corresponding orders, even if they haven't placed any orders yet.
When to Use a RIGHT JOIN in SQL
The RIGHT JOIN in SQL is used to return all the rows from the right table and the matching rows from the left table. If there is no match, NULL values are returned for the left table columns.
The right join is the reverse of the left join. It returns all the rows from the right table, regardless of whether there is a match in the left table.
The right join can be useful when you want to include all the records from one table and only the matching records from another table, but in the reverse order.
Let's consider an example to better understand when to use a RIGHT JOIN. Suppose we have two tables: customers and orders. The customers table contains information about customers, such as their names and email addresses. The orders table contains information about orders, such as the order ID and the customer ID.
We can use a RIGHT JOIN to get a result set that includes all the orders and their corresponding customers, if any. The following SQL query demonstrates how to achieve this using a RIGHT JOIN:
SELECT customers.name, orders.order_id FROM customers RIGHT JOIN orders ON customers.customer_id = orders.customer_id;
In this example, we are selecting the customer's name from the customers table and the order ID from the orders table. We are using a RIGHT JOIN to include all the orders and their corresponding customers, even if they don't have any customer information associated with them.
Merging Results of Two Queries Using UNION in SQL
In SQL, the UNION operator is used to combine the results of two or more SELECT statements into a single result set. The UNION operator removes duplicate rows from the result set by default.
The UNION operator can be used to merge the results of two queries that have the same number of columns and compatible data types. This can be useful when you want to combine the results of two separate queries into a single result set.
Let's consider an example to illustrate how to merge the results of two queries using the UNION operator. Suppose we have two tables: employees and contractors. The employees table contains information about employees, such as their names and job titles. The contractors table contains information about contractors, such as their names and project names.
We can use the UNION operator to merge the results of two queries that select the names from the employees and contractors tables, respectively. The following SQL query demonstrates how to achieve this using the UNION operator:
SELECT name FROM employees UNION SELECT name FROM contractors;
In this example, we are selecting the names from the employees table and the names from the contractors table. We are using the UNION operator to merge the results of these two queries into a single result set.
Related Article: How to Compare & Manipulate Dates in PostgreSQL
Understanding Subqueries in SQL
In SQL, a subquery is a query that is nested inside another query. A subquery can be used to retrieve data from one or more tables and use that data as part of the main query.
Subqueries can be used in various parts of a SQL statement, such as the SELECT, FROM, WHERE, and HAVING clauses. They can be used to filter data, calculate aggregates, perform calculations, and more.
Let's consider an example to demonstrate how to use subqueries in SQL. Suppose we have two tables: customers and orders. The customers table contains information about customers, such as their names and email addresses. The orders table contains information about orders, such as the order ID and the customer ID.
We can use a subquery to retrieve the total number of orders placed by each customer. The following SQL query demonstrates how to achieve this using a subquery:
SELECT customers.name, ( SELECT COUNT(*) FROM orders WHERE orders.customer_id = customers.customer_id ) AS total_orders FROM customers;
In this example, we are selecting the customer's name from the customers table and using a subquery to calculate the total number of orders placed by each customer. The subquery retrieves the count of orders from the orders table, filtered by the customer ID.
Using Alias in Joining Query Results in SQL
In SQL, an alias is a temporary name assigned to a table or a column in a query. Aliases can be used to make the query more readable and to avoid naming conflicts with other tables or columns.
When joining query results in SQL, you can use aliases to assign temporary names to the tables involved in the join. This can make the query more concise and easier to understand.
Let's consider an example to demonstrate how to use aliases in joining query results in SQL. Suppose we have two tables: customers and orders. The customers table contains information about customers, such as their names and email addresses. The orders table contains information about orders, such as the order ID and the customer ID.
We can use aliases to assign temporary names to the tables and simplify the join condition. The following SQL query demonstrates how to achieve this using aliases:
SELECT c.name, o.order_id FROM customers AS c JOIN orders AS o ON c.customer_id = o.customer_id;
In this example, we are selecting the customer's name from the customers table and the order ID from the orders table. We are using aliases (c and o) to assign temporary names to the tables customers and orders, respectively. This makes the query more concise and easier to read.
Examples of Aggregate Functions in Joining Query Results
In SQL, aggregate functions are used to perform calculations on a set of values and return a single value. Aggregate functions can be used in joining query results to calculate summary statistics or perform calculations on the combined data.
Let's consider some examples of aggregate functions in joining query results in SQL. Suppose we have two tables: customers and orders. The customers table contains information about customers, such as their names and email addresses. The orders table contains information about orders, such as the order ID, the customer ID, and the order amount.
We can use aggregate functions to calculate the total order amount for each customer. The following SQL query demonstrates how to achieve this using the SUM aggregate function:
SELECT customers.name, SUM(orders.order_amount) AS total_order_amount FROM customers JOIN orders ON customers.customer_id = orders.customer_id GROUP BY customers.name;
In this example, we are selecting the customer's name from the customers table and using the SUM aggregate function to calculate the total order amount from the orders table. We are joining the two tables based on the customer ID and grouping the results by the customer's name.
This query will return the total order amount for each customer, allowing us to analyze the combined data from the customers and orders tables.
Additional Resources
- Difference between INNER JOIN and OUTER JOIN in SQL