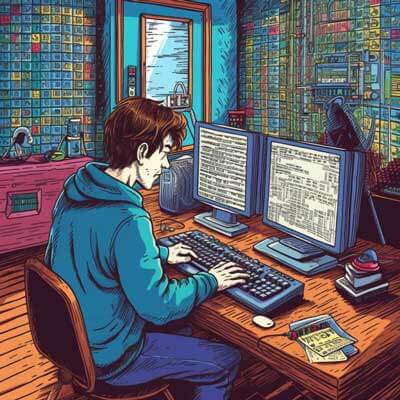
The Purpose of a SQL Join
In SQL, a join is used to combine rows from two or more tables based on a related column between them. It allows us to retrieve data from multiple tables in a single query, providing a way to establish relationships and extract meaningful information.
The main purpose of a SQL join is to retrieve data that is spread across multiple tables and combine it into a single result set. By using join operations, we can avoid the need for multiple queries or manual data merging, making our queries more efficient and concise.
There are different types of joins available in SQL, each serving a specific purpose based on the relationship between the tables involved. Understanding the different types of joins and how to use them effectively is essential for writing efficient and accurate SQL queries.
Related Article: Tutorial: ON for JOIN SQL in Databases
How the SQL Where Clause Works
The WHERE clause is an essential part of SQL queries as it allows us to filter rows based on specific conditions. It is used to specify a search condition that determines which rows should be included in the result set.
When the WHERE clause is used in combination with a join operation, it helps to further narrow down the result set by applying conditions on the joined tables. This is particularly useful when we need to retrieve only the rows that satisfy specific criteria from the joined tables.
Let’s consider an example to illustrate how the WHERE clause works with a join in SQL:
Suppose we have two tables: “Customers” and “Orders”. The “Customers” table contains information about customers, such as their names and addresses, while the “Orders” table contains information about orders, such as the order date and total amount.
We want to retrieve all the orders made by customers from a specific city, let’s say “New York”. We can achieve this by using the WHERE clause in combination with a join operation:
SELECT Orders.order_id, Customers.customer_name, Orders.order_date FROM Orders JOIN Customers ON Orders.customer_id = Customers.customer_id WHERE Customers.city = 'New York';
In this example, we are joining the “Orders” and “Customers” tables based on the “customer_id” column. The WHERE clause is then used to filter the result set and retrieve only the orders made by customers from the city of “New York”.
Different Types of SQL Joins
In SQL, there are several types of joins that can be used to combine rows from multiple tables. The choice of join type depends on the relationship between the tables and the desired result set.
The main types of SQL joins are:
1. Inner Join: An inner join returns only the rows that have matching values in both tables being joined. It combines rows from the tables based on the specified join condition.
2. Left Join: A left join returns all the rows from the left table and the matching rows from the right table. If there is no match, NULL values are returned for the columns of the right table.
3. Right Join: A right join returns all the rows from the right table and the matching rows from the left table. If there is no match, NULL values are returned for the columns of the left table.
4. Full Outer Join: A full outer join returns all the rows from both tables, including the unmatched rows. If there is no match, NULL values are returned for the columns of the other table.
Let’s explore each type of join in more detail and provide examples for better understanding.
Using the SQL Select Statement
The SELECT statement is used to retrieve data from one or more tables in SQL. It allows us to specify the columns we want to retrieve and apply conditions to filter the result set.
The basic syntax of the SELECT statement is as follows:
SELECT column1, column2, ... FROM table_name;
We can also use the * wildcard character to retrieve all columns from a table:
SELECT * FROM table_name;
The SELECT statement can be extended to include join operations and the WHERE clause to retrieve data from multiple tables based on specific conditions.
Related Article: Analyzing SQL Join and Its Effect on Records
Understanding Inner Joins in SQL
An inner join is the most common type of join in SQL. It returns only the rows that have matching values in both tables being joined. It combines rows from the tables based on the specified join condition.
The syntax for an inner join is as follows:
SELECT column1, column2, ... FROM table1 INNER JOIN table2 ON table1.column = table2.column;
Here’s an example to illustrate the usage of an inner join:
Suppose we have two tables: “Employees” and “Departments”. The “Employees” table contains information about employees, such as their names and job titles, while the “Departments” table contains information about departments, such as the department name and location.
We want to retrieve the names of employees along with their department names. We can achieve this by using an inner join:
SELECT Employees.name, Departments.department_name FROM Employees INNER JOIN Departments ON Employees.department_id = Departments.department_id;
In this example, we are joining the “Employees” and “Departments” tables based on the “department_id” column. The inner join combines the rows from both tables where the department IDs match, and we retrieve the names of employees along with their respective department names.
Exploring Outer Joins in SQL
Outer joins are used to retrieve data from multiple tables, even if there is no match between the joined columns. They allow us to include unmatched rows from one or both tables in the result set.
There are three types of outer joins in SQL: left join, right join, and full outer join.
The Left Join in SQL
A left join returns all the rows from the left table and the matching rows from the right table. If there is no match, NULL values are returned for the columns of the right table.
The syntax for a left join is as follows:
SELECT column1, column2, ... FROM table1 LEFT JOIN table2 ON table1.column = table2.column;
Here’s an example to illustrate the usage of a left join:
Suppose we have two tables: “Customers” and “Orders”. The “Customers” table contains information about customers, such as their names and addresses, while the “Orders” table contains information about orders, such as the order date and total amount.
We want to retrieve all the customers along with their order information, even if they haven’t placed any orders. We can achieve this by using a left join:
SELECT Customers.customer_name, Orders.order_date, Orders.total_amount FROM Customers LEFT JOIN Orders ON Customers.customer_id = Orders.customer_id;
In this example, we are joining the “Customers” and “Orders” tables based on the “customer_id” column. The left join returns all the customers from the left table (Customers) and the matching orders from the right table (Orders). If a customer has not placed any orders, NULL values are returned for the order date and total amount.
The Right Join in SQL
A right join returns all the rows from the right table and the matching rows from the left table. If there is no match, NULL values are returned for the columns of the left table.
The syntax for a right join is as follows:
SELECT column1, column2, ... FROM table1 RIGHT JOIN table2 ON table1.column = table2.column;
Here’s an example to illustrate the usage of a right join:
Suppose we have two tables: “Orders” and “Customers”. The “Orders” table contains information about orders, such as the order date and total amount, while the “Customers” table contains information about customers, such as their names and addresses.
We want to retrieve all the orders along with the customer information, even if there are no matching customers. We can achieve this by using a right join:
SELECT Orders.order_id, Customers.customer_name, Orders.order_date FROM Orders RIGHT JOIN Customers ON Orders.customer_id = Customers.customer_id;
In this example, we are joining the “Orders” and “Customers” tables based on the “customer_id” column. The right join returns all the orders from the right table (Orders) and the matching customers from the left table (Customers). If there are no matching customers, NULL values are returned for the customer name.
The Full Outer Join in SQL
A full outer join returns all the rows from both tables, including the unmatched rows. If there is no match, NULL values are returned for the columns of the other table.
The syntax for a full outer join varies between different database management systems. Here’s an example using the standard SQL syntax:
SELECT column1, column2, ... FROM table1 FULL OUTER JOIN table2 ON table1.column = table2.column;
Here’s an example to illustrate the usage of a full outer join:
Suppose we have two tables: “Customers” and “Orders”. The “Customers” table contains information about customers, such as their names and addresses, while the “Orders” table contains information about orders, such as the order date and total amount.
We want to retrieve all the customers and their order information, regardless of whether they have placed any orders or not. We can achieve this by using a full outer join:
SELECT Customers.customer_name, Orders.order_date, Orders.total_amount FROM Customers FULL OUTER JOIN Orders ON Customers.customer_id = Orders.customer_id;
In this example, we are joining the “Customers” and “Orders” tables based on the “customer_id” column. The full outer join returns all the customers and their matching orders, as well as the unmatched customers and their NULL order information.
Primary Key vs. Foreign Key in SQL
In SQL, a primary key is a column or a set of columns that uniquely identifies each row in a table. It is used to enforce data integrity and provide a way to reference and link data between tables.
A foreign key, on the other hand, is a column or a set of columns in a table that refers to the primary key of another table. It establishes a relationship between the two tables and ensures the referential integrity of the data.
Let’s consider an example to understand the difference between a primary key and a foreign key:
Suppose we have two tables: “Customers” and “Orders”. The “Customers” table contains information about customers, such as their names and addresses, while the “Orders” table contains information about orders, such as the order date and total amount.
In this scenario, the “customer_id” column in the “Customers” table can be defined as the primary key. It uniquely identifies each customer in the table.
The “customer_id” column in the “Orders” table can be defined as a foreign key. It refers to the primary key of the “Customers” table and establishes a relationship between the two tables. This allows us to link each order to the corresponding customer.
Related Article: Tutorial: Full Outer Join versus Join in SQL