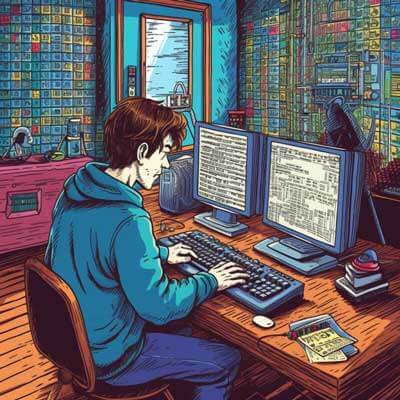
Table of Contents
Introduction to Generics in TypeScript
Generics in TypeScript provide a way to create reusable components that can work with multiple types. They allow us to define functions, classes, and interfaces that can operate on a variety of data types, while still maintaining type safety. The use of generics enhances code reusability and type checking, making our code more robust and maintainable.
To understand generics, let's start with a simple example. We'll create a function that takes an argument of type T and returns the same value. Here's what the code looks like:
function identity(arg: T): T { return arg; } let output = identity(5); console.log(output); // Output: 5 let result = identity("Hello"); console.log(result); // Output: Hello
In this example, we define a generic function identity
that takes a type parameter T
. The function parameter arg
is of type T
and the return type is also T
. We can then call this function with different types, such as number
and string
.
Related Article: How to Verify if a Value is in Enum in TypeScript
Syntax and Basic Concepts of Generics
To use generics in TypeScript, we use angle brackets (<>
) to specify the type parameter. This type parameter can have any name, but it is common to use single uppercase letters, such as T
, U
, or V
. Here's an example that demonstrates the syntax:
function printArray(arr: T[]): void { for (let element of arr) { console.log(element); } } let numbers: number[] = [1, 2, 3, 4, 5]; let strings: string[] = ["apple", "banana", "orange"]; printArray(numbers); // Output: 1 2 3 4 5 printArray(strings); // Output: apple banana orange
In this example, we define a generic function printArray
that takes an array of type T
and prints each element. We then call this function with arrays of numbers and strings, specifying the type parameter explicitly.
Example: Creating a Generic Stack
A common use case of generics is to create data structures that can hold elements of any type. Let's create a generic stack class that allows us to push and pop elements. Here's the code:
class Stack { private items: T[] = []; push(item: T): void { this.items.push(item); } pop(): T | undefined { return this.items.pop(); } } let numberStack = new Stack(); numberStack.push(1); numberStack.push(2); numberStack.push(3); console.log(numberStack.pop()); // Output: 3 let stringStack = new Stack(); stringStack.push("Hello"); stringStack.push("World"); console.log(stringStack.pop()); // Output: World
In this example, we define a generic class Stack
that can hold elements of any type T
. We use an array items
to store the elements. The push
method adds an item to the stack, and the pop
method removes and returns the topmost item. We create instances of this class with different type parameters, such as number
and string
.
Example: Implementing a Generic Identity Function
Let's implement a generic identity function that takes an argument of type T
and returns the same value. Here's the code:
function identity(arg: T): T { return arg; } let output = identity(5); console.log(output); // Output: 5 let result = identity("Hello"); console.log(result); // Output: Hello
In this example, we define a generic function identity
that takes a type parameter T
. The function parameter arg
is of type T
and the return type is also T
. We can then call this function with different types, such as number
and string
.
Related Article: Tutorial on Circuit Breaker Pattern in TypeScript
Practical Use Cases of Generics
Generics are a powerful tool that can be applied to a wide range of scenarios. Let's explore some practical use cases where generics can be beneficial.
Use Case: Creating a Generic Repository
When working with databases or APIs, it's common to have different entities with similar CRUD (Create, Read, Update, Delete) operations. We can use generics to create a generic repository that provides a consistent interface for performing these operations. Here's an example:
interface Entity { id: number; } class Repository { private items: T[] = []; add(item: T): void { this.items.push(item); } getById(id: number): T | undefined { return this.items.find(item => item.id === id); } update(item: T): void { const index = this.items.findIndex(i => i.id === item.id); if (index !== -1) { this.items[index] = item; } } delete(id: number): void { const index = this.items.findIndex(item => item.id === id); if (index !== -1) { this.items.splice(index, 1); } } } // Usage example class User implements Entity { id: number; name: string; } const userRepository = new Repository(); userRepository.add({ id: 1, name: "John" }); userRepository.add({ id: 2, name: "Jane" }); const user = userRepository.getById(1); console.log(user); // Output: { id: 1, name: "John" } user.name = "Alice"; userRepository.update(user); userRepository.delete(2); console.log(userRepository.getById(2)); // Output: undefined
In this example, we define an Entity
interface that represents an entity with an id
property. We then create a generic Repository
class that operates on entities that extend the Entity
interface. The class provides methods for adding, retrieving, updating, and deleting entities. We can create instances of this class with different entity types, such as User
in the usage example.
Use Case: Creating a Generic Map
Another practical use case of generics is when creating a generic map data structure. Let's implement a simple map class that can store key-value pairs of any type. Here's the code:
class Map<K, V> { private data: { key: K; value: V }[] = []; set(key: K, value: V): void { const existingIndex = this.data.findIndex(item => item.key === key); if (existingIndex !== -1) { this.data[existingIndex] = { key, value }; } else { this.data.push({ key, value }); } } get(key: K): V | undefined { const item = this.data.find(item => item.key === key); return item ? item.value : undefined; } delete(key: K): void { const index = this.data.findIndex(item => item.key === key); if (index !== -1) { this.data.splice(index, 1); } } } // Usage example const numberMap = new Map<string, number>(); numberMap.set("one", 1); numberMap.set("two", 2); console.log(numberMap.get("one")); // Output: 1 numberMap.delete("two"); console.log(numberMap.get("two")); // Output: undefined
In this example, we define a generic Map
class that stores key-value pairs. The class uses two type parameters K
and V
to represent the types of the keys and values. The set
method adds or updates a key-value pair, the get
method retrieves the value for a given key, and the delete
method removes a key-value pair. We can create instances of this class with different key and value types, such as string
and number
in the usage example.
Best Practices for Using Generics
When using generics in TypeScript, it's important to follow best practices to ensure clean and maintainable code. Here are some best practices to consider:
Related Article: Tutorial: Generating GUID in TypeScript
Best Practice: Use Descriptive Type Parameter Names
When defining generics, use descriptive type parameter names that convey the purpose of the parameter. This makes the code more readable and helps other developers understand the intent of the generic. For example, instead of using T
, consider using TItem
or TResult
to provide more context.
Best Practice: Provide Default Types for Type Parameters
In some cases, it may be helpful to provide default types for type parameters. This allows users of your generic code to omit the type argument if the default type is suitable for their needs. Here's an example:
function identity(arg: T): T { return arg; } let output = identity(5); // Type argument is optional console.log(output); // Output: 5
In this example, the identity
function has a default type any
for the type parameter T
. This allows us to call the function without specifying the type argument, as TypeScript will infer the type based on the argument.
Error Handling with Generics
When working with generics, it's important to handle errors effectively to ensure the integrity of your code. Here are some techniques for error handling with generics:
Technique: Using Conditional Types for Error Handling
Conditional types in TypeScript allow us to perform type checks and handle errors based on the inferred or specified types. We can use conditional types to enforce certain constraints on the generic types and provide error messages when the constraints are not met. Here's an example:
type NonEmptyArray = T[] extends [] ? "Array must not be empty" : T[]; function createNonEmptyArray(items: T[]): NonEmptyArray { if (items.length === 0) { throw new Error("Array must not be empty"); } return items as NonEmptyArray; } const emptyArray: number[] = []; const nonEmptyArray = createNonEmptyArray(emptyArray); // Error: Array must not be empty
In this example, we define a conditional type NonEmptyArray
that checks if the generic type T[]
is assignable to []
. If it is, the type is set to "Array must not be empty"
, indicating an error. We then create a function createNonEmptyArray
that takes an array and returns a non-empty array, throwing an error if the input array is empty.
Related Article: Tutorial: Extending the Window Object in TypeScript
Technique: Using Assertion Functions for Error Handling
Assertion functions in TypeScript allow us to perform runtime checks and throw errors when certain conditions are not met. We can use assertion functions to validate the generic types and provide informative error messages. Here's an example:
function assertNonNull(value: T | null | undefined): asserts value is T { if (value === null || value === undefined) { throw new Error("Value must not be null or undefined"); } } function processValue(value: T): void { assertNonNull(value); // Continue processing the value } processValue("Hello"); // No error processValue(null); // Error: Value must not be null or undefined
In this example, we define an assertion function assertNonNull
that checks if the value is not null
or undefined
. If it is, an error is thrown. We then create a function processValue
that takes a generic value and asserts that it is not null
or undefined
. If the value passes the assertion, we can safely continue processing it.
Performance Considerations of Using Generics
While generics provide flexibility and type safety, they can also introduce some performance considerations. Here are some factors to keep in mind when using generics:
Consideration: Code Bloat
Generics can lead to code bloat, especially when used extensively throughout a codebase. Each instantiation of a generic type or function creates a new copy of the code, potentially increasing the size of the compiled output. This can impact the loading time and runtime performance of your application, especially if the generics are used in performance-critical sections.
To mitigate code bloat, consider using more specific types where possible instead of relying on generics. Only use generics when the flexibility they provide is necessary for the functionality of your code.
Consideration: Type Inference and Compilation Time
Type inference in TypeScript can sometimes struggle with complex or deeply nested generics. This can result in longer compilation times, especially when working with large codebases.
To improve compilation performance, consider providing explicit type annotations for generic functions and classes. This helps TypeScript infer the types more accurately and reduces the time spent by the compiler trying to infer them.
Related Article: How Static Typing Works in TypeScript
Consideration: Boxing and Unboxing
When working with generic types, TypeScript may need to box or unbox values to ensure type safety. Boxing refers to wrapping a value with its corresponding type information, while unboxing refers to extracting the value from its boxed form. These boxing and unboxing operations can introduce a slight overhead in terms of memory and performance.
While the overhead is generally negligible, it's worth considering in performance-critical scenarios. If performance is a concern, consider using specific types or non-generic alternatives where the boxing and unboxing operations can be avoided.
Advanced Techniques Using Generics
Generics in TypeScript can be used in advanced ways to solve complex problems and achieve powerful abstractions. Let's explore some advanced techniques and patterns you can use with generics.
Technique: Using Conditional Types with Generics
Conditional types in TypeScript allow us to define types that depend on a condition. This can be useful when working with generics to create more precise and flexible types. Here's an example:
type NonNullable = T extends null | undefined ? never : T; function processValue(value: NonNullable): void { // Process the non-null and non-undefined value } processValue("Hello"); // No error processValue(null); // Error: Argument of type 'null' is not assignable to parameter of type 'never'
In this example, we define a conditional type NonNullable
that checks if the generic type T
is assignable to null
or undefined
. If it is, the type is set to never
, indicating an error. We then create a function processValue
that takes a generic value of type NonNullable
, ensuring that the value is neither null
nor undefined
.
Technique: Using Mapped Types with Generics
Mapped types in TypeScript allow us to transform and manipulate the properties of an existing type. We can combine mapped types with generics to create generic mapped types that adapt to different input types. Here's an example:
type Optional = { [K in keyof T]?: T[K]; }; interface User { id: number; name: string; email: string; } let optionalUser: Optional = { id: 1, name: "John", };
In this example, we define a generic mapped type Optional
that takes a type T
. The mapped type iterates over each property K
in T
and creates an optional version of each property. We then use this mapped type to create an optionalUser
object where some properties of the User
interface are optional.
Related Article: Comparing Go with TypeScript
Technique: Using Type Guards with Generics
Type guards in TypeScript allow us to narrow down the type of a value based on a runtime check. We can use type guards with generics to create more precise type checks and enable specific behavior based on the type. Here's an example:
function processValue(value: T): void { if (typeof value === "string") { // Process the string value } else if (Array.isArray(value)) { // Process the array value } else { // Process other types } }
In this example, we define a generic function processValue
that takes a value of type T
and performs different actions based on the runtime type of the value. We use type guards (typeof
and Array.isArray
) to narrow down the type and enable specific processing logic for different types.
Code Snippet: Implementing a Generic Function
Here's a code snippet that demonstrates how to implement a generic function in TypeScript:
function reverseArray(arr: T[]): T[] { return arr.reverse(); } let numbers: number[] = [1, 2, 3, 4, 5]; let reversed = reverseArray(numbers); console.log(reversed); // Output: [5, 4, 3, 2, 1]
In this example, we define a generic function reverseArray
that takes an array of type T
and returns the reversed array. We then call this function with an array of numbers and store the result in the reversed
variable.
Code Snippet: Using a Generic Class
Here's a code snippet that demonstrates how to use a generic class in TypeScript:
class Box { private value: T; constructor(value: T) { this.value = value; } getValue(): T { return this.value; } } let numberBox = new Box(42); let numberValue = numberBox.getValue(); console.log(numberValue); // Output: 42 let stringBox = new Box("Hello"); let stringValue = stringBox.getValue(); console.log(stringValue); // Output: Hello
In this example, we define a generic class Box
that holds a value of type T
. The constructor takes an argument of type T
, and the getValue
method returns the stored value. We create instances of this class with different type parameters, such as number
and string
, and retrieve the values using the getValue
method.
Code Snippet: Extending Generic Classes
Here's a code snippet that demonstrates how to extend a generic class in TypeScript:
class Stack { private items: T[] = []; push(item: T): void { this.items.push(item); } pop(): T | undefined { return this.items.pop(); } } class NumberStack extends Stack { sum(): number { return this.items.reduce((acc, val) => acc + val, 0); } } let numberStack = new NumberStack(); numberStack.push(1); numberStack.push(2); numberStack.push(3); console.log(numberStack.sum()); // Output: 6
In this example, we define a generic class Stack
that holds a stack of items of type T
. We then create a subclass NumberStack
that extends Stack
. The NumberStack
class adds an additional method sum
that calculates the sum of all numbers in the stack. We create an instance of NumberStack
, push some numbers onto the stack, and call the sum
method to get the sum of the numbers.
Related Article: Building a Rules Engine with TypeScript
Code Snippet: Generic Constraints
Here's a code snippet that demonstrates how to use generic constraints in TypeScript:
interface Shape { getArea(): number; } class Rectangle implements Shape { constructor(private width: number, private height: number) {} getArea(): number { return this.width * this.height; } } class Circle implements Shape { constructor(private radius: number) {} getArea(): number { return Math.PI * this.radius * this.radius; } } function calculateTotalArea(shapes: T[]): number { let totalArea = 0; for (let shape of shapes) { totalArea += shape.getArea(); } return totalArea; } let rectangle = new Rectangle(5, 10); let circle = new Circle(3); let totalArea = calculateTotalArea([rectangle, circle]); console.log(totalArea); // Output: 104.71238898038469
In this example, we define an interface Shape
with a getArea
method. We then create two classes Rectangle
and Circle
that implement the Shape
interface and provide their own implementations of the getArea
method. We also define a generic function calculateTotalArea
that takes an array of shapes and calculates the total area by calling the getArea
method on each shape. We create instances of Rectangle
and Circle
, pass them to the calculateTotalArea
function, and log the result.
Code Snippet: Using Generic Interfaces
Here's a code snippet that demonstrates how to use generic interfaces in TypeScript:
interface Repository { getById(id: number): T | undefined; add(item: T): void; update(item: T): void; delete(id: number): void; } interface User { id: number; name: string; } class UserRepository implements Repository { private users: User[] = []; getById(id: number): User | undefined { return this.users.find(user => user.id === id); } add(user: User): void { this.users.push(user); } update(user: User): void { const index = this.users.findIndex(u => u.id === user.id); if (index !== -1) { this.users[index] = user; } } delete(id: number): void { const index = this.users.findIndex(user => user.id === id); if (index !== -1) { this.users.splice(index, 1); } } } let userRepository = new UserRepository(); userRepository.add({ id: 1, name: "John" }); let user = userRepository.getById(1); console.log(user); // Output: { id: 1, name: "John" }
In this example, we define a generic interface Repository
that represents a data repository with common CRUD operations. We then create a class UserRepository
that implements the Repository
interface with User
as the type parameter. The class provides implementations for the interface methods specific to the User
type. We create an instance of UserRepository
, add a user, and retrieve it using the getById
method.
Real World Examples: Generics in Large Scale Applications
Generics are widely used in large-scale applications to improve code reusability and maintainability. Here are some real-world examples of how generics are used in large-scale applications:
Example: Promises and Async Operations
In asynchronous programming, promises are commonly used to handle the results of asynchronous operations. Promises can be generic, allowing developers to specify the type of the value that will be resolved or rejected. Here's an example:
function fetchData(url: string): Promise { return fetch(url).then(response => response.json()); } fetchData("https://api.example.com/users/1") .then(user => console.log(user)) .catch(error => console.error(error));
In this example, the fetchData
function returns a promise that resolves to a value of type T
, where T
is the type parameter specified when calling the function. We call the fetchData
function with the User
type parameter to fetch user data from an API and then log the user object.
Related Article: How to Run Typescript Ts-Node in Databases
Example: Collections and Data Structures
Generics are often used in collections and data structures to ensure type safety and provide flexibility. Libraries and frameworks often provide generic implementations of common data structures, such as lists, maps, or queues. Here's an example using the Array
class:
let numbers: Array = [1, 2, 3, 4, 5]; let strings: Array = ["apple", "banana", "orange"]; console.log(numbers[0]); // Output: 1 console.log(strings[1]); // Output: banana
In this example, we create arrays of numbers and strings using the Array
class with type parameters. This ensures that only numbers can be added to the numbers
array and only strings can be added to the strings
array.
Example: Database Abstraction Layers
In database abstraction layers, generics are commonly used to create type-safe queries and operations. Generics allow developers to define query builders or data access methods that can work with different entity types. Here's an example:
interface Entity { id: number; } class Repository { getById(id: number): Promise { // Perform database query and return the entity } create(entity: T): Promise { // Insert the entity into the database } update(entity: T): Promise { // Update the entity in the database } delete(id: number): Promise { // Delete the entity from the database } } class User implements Entity { id: number; name: string; // Other properties } let userRepository = new Repository(); userRepository.getById(1).then(user => console.log(user));
In this example, we define a generic Repository
class that provides common database operations for entities that extend the Entity
interface. We then create a User
class that implements the Entity
interface and use the Repository
class to perform CRUD operations on users. The type parameter User
is used to ensure type safety and provide a clear interface for working with user entities.
Real World Examples: Generics in Data Structures
Generics are widely used in data structures to provide type safety and flexibility. Here are some real-world examples of how generics are used in data structures:
Example: Linked List
A linked list is a common data structure that consists of nodes linked together in a sequence. Generics can be used to create a linked list implementation that can store elements of any type. Here's an example:
class ListNode { constructor(public value: T, public next: ListNode | null = null) {} } class LinkedList { private head: ListNode | null = null; add(value: T): void { const newNode = new ListNode(value); if (this.head === null) { this.head = newNode; } else { let current = this.head; while (current.next !== null) { current = current.next; } current.next = newNode; } } print(): void { let current = this.head; while (current !== null) { console.log(current.value); current = current.next; } } } let list = new LinkedList(); list.add(1); list.add(2); list.add(3); list.print(); // Output: 1 2 3
In this example, we define a ListNode
class that represents a node in the linked list. The class has a generic type parameter T
to represent the type of the value stored in the node. We then create a LinkedList
class that manages the nodes and provides methods for adding and printing the values. We create an instance of the LinkedList
class with the number
type parameter and add some numbers to the list.
Related Article: Tutorial on Typescript ts-ignore
Example: Binary Search Tree
A binary search tree is a commonly used data structure for efficient searching and sorting operations. Generics can be used to create a binary search tree implementation that can store elements of any type. Here's an example:
class BinaryTreeNode { constructor( public value: T, public left: BinaryTreeNode | null = null, public right: BinaryTreeNode | null = null ) {} } class BinarySearchTree { private root: BinaryTreeNode | null = null; add(value: T): void { const newNode = new BinaryTreeNode(value); if (this.root === null) { this.root = newNode; } else { let current = this.root; while (true) { if (value < current.value) { if (current.left === null) { current.left = newNode; break; } current = current.left; } else { if (current.right === null) { current.right = newNode; break; } current = current.right; } } } } contains(value: T): boolean { let current = this.root; while (current !== null) { if (value === current.value) { return true; } else if (value < current.value) { current = current.left; } else { current = current.right; } } return false; } } let tree = new BinarySearchTree(); tree.add(5); tree.add(3); tree.add(7); console.log(tree.contains(3)); // Output: true console.log(tree.contains(8)); // Output: false
In this example, we define a BinaryTreeNode
class that represents a node in the binary search tree. The class has a generic type parameter T
to represent the type of the value stored in the node. We then create a BinarySearchTree
class that manages the nodes and provides methods for adding and searching values. We create an instance of the BinarySearchTree
class with the number
type parameter and add some numbers to the tree.
Example: Graph
A graph is a versatile data structure that represents a collection of interconnected nodes. Generics can be used to create a generic graph implementation that can represent different types of nodes and edges. Here's an example:
class GraphNode { constructor(public value: T, public neighbors: GraphNode[] = []) {} } class Graph { private nodes: GraphNode[] = []; addNode(value: T): void { const newNode = new GraphNode(value); this.nodes.push(newNode); } addEdge(from: T, to: T): void { const fromNode = this.findNode(from); const toNode = this.findNode(to); if (fromNode && toNode) { fromNode.neighbors.push(toNode); } } private findNode(value: T): GraphNode | undefined { return this.nodes.find(node => node.value === value); } } let graph = new Graph(); graph.addNode("A"); graph.addNode("B"); graph.addNode("C"); graph.addEdge("A", "B"); graph.addEdge("B", "C"); console.log(graph);
In this example, we define a GraphNode
class that represents a node in the graph. The class has a generic type parameter T
to represent the type of the value stored in the node. We then create a Graph
class that manages the nodes and provides methods for adding nodes and edges. We create an instance of the Graph
class with the string
type parameter and add some nodes and edges to the graph.
Real World Examples: Generics in API Design
Generics are commonly used in API design to create flexible and reusable interfaces. Here are some real-world examples of how generics are used in API design:
Example: Array.prototype.map
The Array.prototype.map
method in JavaScript is a common example of using generics in API design. It allows developers to apply a transformation function to each element of an array and returns a new array with the transformed values. Here's an example:
let numbers: number[] = [1, 2, 3, 4, 5]; let doubled = numbers.map(number => number * 2); console.log(doubled); // Output: [2, 4, 6, 8, 10]
In this example, we use the map
method on the numbers
array. The map
method takes a generic type parameter to represent the type of the transformed values. The transformation function passed to map
returns the doubled value of each number in the array.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
Example: React Props and State
The React library uses generics extensively in its API design to provide type safety and reusability. For example, the Props
and State
generics are used in React components to define the types of the properties and internal state. Here's an example:
interface User { id: number; name: string; } class UserComponent extends React.Component<UserProps, UserState> { // Component implementation } interface UserProps { user: User; } interface UserState { isLoading: boolean; }
In this example, we define a User
interface that represents a user object. We then create a UserComponent
class that extends React.Component
with UserProps
as the props type and UserState
as the state type. The UserProps
interface specifies that the component expects a user
prop of type User
, and the UserState
interface specifies that the component has an isLoading
state property of type boolean
.
Example: Express Middleware
The Express.js framework uses generics in its API design to create flexible and reusable middleware functions. Middleware functions in Express can accept generic types to specify the types of the request, response, and next function. Here's an example:
import { Request, Response, NextFunction } from "express"; function loggerMiddleware( req: T, res: Response, next: NextFunction ): void { console.log(`${req.method} ${req.url}`); next(); } app.use(loggerMiddleware);
In this example, we define a loggerMiddleware
function that logs the HTTP method and URL of each incoming request. The function uses generics to specify the type of the req
parameter as T extends Request
, where T
represents the specific type of the request. We then use the app.use
method to register the loggerMiddleware
function as middleware in an Express application.
Limitations and Workarounds of Generics
While generics provide powerful abstractions, there are some limitations and workarounds to be aware of. Here are some common limitations and possible workarounds when working with generics:
Limitation: Type Erasure
TypeScript generics are subject to type erasure at runtime, meaning that the type information is not available during runtime. This can limit the ability to perform runtime checks or access the type information in certain scenarios.
Workaround: Use Type Predicates or Type Guards to perform runtime checks or use instanceof
to check the type of an object at runtime.
Related Article: Tutorial: Checking if a String is a Number in TypeScript
Limitation: Inference Limitations
TypeScript's type inference for generics may not always infer the desired types correctly, especially in complex scenarios. This can result in the need to provide explicit type annotations for generic functions or classes.
Workaround: Provide explicit type annotations for generic functions or classes to ensure the desired types are inferred correctly.
Limitation: Lack of Generic Overloads
TypeScript does not currently support generic overloads, which means that it's not possible to have different behavior based on the type parameter in an overload set.
Workaround: Use function overloads without generics to provide different behavior based on the runtime type of the arguments.
Performance Considerations: Benchmarking Generics
When working with generics, it's important to consider the performance implications, especially in performance-critical scenarios. Here are some tips for benchmarking generics:
Tip: Measure Performance Impact
When using generics, measure the performance impact of the generic code compared to non-generic alternatives. Use tools like performance profilers or benchmarking libraries to measure the execution time and resource usage of your code.
Related Article: TypeScript ETL (Extract, Transform, Load) Tutorial
Tip: Compare Generic and Non-Generic Implementations
Compare the performance of your generic implementation with a non-generic implementation to determine if the performance overhead of generics is acceptable for your use case. Sometimes, using more specific types or non-generic alternatives can provide better performance.
Tip: Optimize Generic Code
If you identify performance bottlenecks in your generic code, consider optimizing the code to reduce the performance impact. This can include techniques like caching, memoization, or using more specific types to avoid unnecessary type checks or boxing/unboxing operations.
Performance Considerations: Memory Management with Generics
When working with generics, it's important to consider memory management, especially when dealing with large data structures. Here are some tips for memory management with generics:
Tip: Avoid Unnecessary Boxing and Unboxing
Generics may introduce boxing and unboxing operations, which can increase memory usage and impact performance. Avoid unnecessary boxing and unboxing by using more specific types or non-generic alternatives where possible.
Related Article: How to Use the Record Type in TypeScript
Tip: Be Mindful of Object Size
When working with large data structures or collections, be mindful of the size of the objects stored in the generic types. Storing large objects in generic types can increase memory usage and impact performance. Consider using more specific types or non-generic alternatives to reduce memory overhead.
Tip: Dispose of Unused Objects
If your generic code creates temporary objects or data structures that are no longer needed, make sure to dispose of them properly to free up memory. Use techniques like object pooling or manual memory management to optimize memory usage.
Performance Considerations: Optimization Tips for Generics
When working with generics, there are some optimization tips that can help improve performance. Here are some tips for optimizing generics:
Tip: Use Specific Types When Possible
When working with generics, consider using specific types instead of generic types where possible. Using specific types can avoid unnecessary type checks and improve performance.
Related Article: Tutorial: Readonly vs Const in TypeScript
Tip: Minimize Type Parameter Usage
Minimize the usage of type parameters in generic functions or classes. Excessive usage of type parameters can lead to code bloat and slower compilation times. Use type parameters only when necessary for the functionality of your code.
Tip: Use Narrower Type Constraints
When defining generic types, use narrower type constraints to limit the possible types that can be used as type arguments. This can help the TypeScript compiler infer more specific types and reduce the impact of type erasure.
Advanced Techniques: Using Mapped Types with Generics
Mapped types in TypeScript allow us to create new types by transforming the properties of an existing type. When combined with generics, mapped types can create powerful abstractions. Here's an example:
type Readonly = { readonly [K in keyof T]: T[K]; }; interface User { id: number; name: string; } let readonlyUser: Readonly = { id: 1, name: "John", }; readonlyUser.id = 2; // Error: Cannot assign to 'id' because it is a read-only property.
In this example, we define a mapped type Readonly
that transforms all the properties of a type T
to be readonly. We then use this mapped type to create a readonlyUser
object where all the properties of User
are readonly.
Advanced Techniques: Conditional Types and Generics
Conditional types in TypeScript allow us to define types that depend on a condition. When combined with generics, conditional types can create more precise and flexible types. Here's an example:
type NonNullable = T extends null | undefined ? never : T; function processValue(value: NonNullable): void { // Process the non-null and non-undefined value } processValue("Hello"); // No error processValue(null); // Error: Argument of type 'null' is not assignable to parameter of type 'never'
In this example, we define a conditional type NonNullable
that checks if the generic type T
is assignable to null
or undefined
. If it is, the type is set to never
, indicating an error. We then create a function processValue
that takes a generic value of type NonNullable
, ensuring that the value is neither null
nor undefined
.
Related Article: How to Check If a String is in an Enum in TypeScript
Advanced Techniques: Using Type Guards with Generics
Type guards in TypeScript allow us to narrow down the type of a value based on a runtime check. When combined with generics, type guards can create more precise type checks. Here's an example:
function processValue(value: T): void { if (typeof value === "string") { // Process the string value } else if (Array.isArray(value)) { // Process the array value } else { // Process other types } }
In this example, we define a generic function processValue
that takes a value of type T
and performs different actions based on the runtime type of the value. We use type guards (typeof
and Array.isArray
) to narrow down the type and enable specific processing logic for different types.