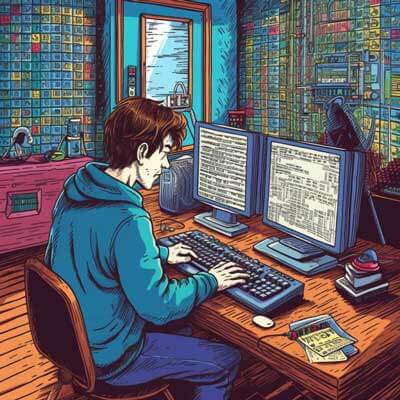
- The Purpose of a Circuit Breaker in TypeScript
- How TypeScript Handles Fault Tolerance
- Benefits of Using a Circuit Breaker in Microservices Architecture
- Error Handling with Circuit Breaker in TypeScript
- Retry Mechanism in Circuit Breaker for TypeScript
- Fallback Mechanism in TypeScript’s Circuit Breaker
- Tracking Circuit State in Circuit Breaker for TypeScript
- Role of Timeout in Circuit Breaker Implementation in TypeScript
- Usage of Circuit Breaker in Other Programming Languages
- Popular TypeScript Libraries/Frameworks for Circuit Breaker Implementation
- External Sources
The Purpose of a Circuit Breaker in TypeScript
A Circuit Breaker is a design pattern that helps in handling and preventing cascading failures in distributed systems. It acts as a safety net by monitoring the calls made to remote systems and preventing them from overwhelming the system in case of failures or timeouts. In TypeScript, the Circuit Breaker pattern can be implemented to ensure fault tolerance and resilience in microservices architectures.
To understand the purpose of a Circuit Breaker in TypeScript, let’s consider a scenario where a microservice makes multiple API calls to external services. If one of the external services becomes slow or unresponsive, the microservice could end up making repeated requests, which can lead to a degradation of the overall system performance. The Circuit Breaker pattern helps in mitigating this issue by introducing a mechanism to detect failures and temporarily halt the requests to the failing service.
In TypeScript, a Circuit Breaker can be implemented using various techniques such as timeouts, error thresholds, and fallback mechanisms. By monitoring the success and failure rates of API calls, the Circuit Breaker can dynamically open or close the circuit to protect the system from further failures.
Let’s take a look at an example of implementing a Circuit Breaker in TypeScript:
class CircuitBreaker { private isOpen: boolean; private failureCount: number; constructor(private maxFailures: number, private timeout: number) { this.isOpen = false; this.failureCount = 0; } public async executeRequest(request: () => Promise): Promise { if (this.isOpen) { throw new Error('Circuit Breaker is open'); } try { const result = await Promise.race([ request(), new Promise((_, reject) => setTimeout(() => reject(new Error('Timeout')), this.timeout) ), ]); this.reset(); return result; } catch (error) { this.failureCount++; if (this.failureCount >= this.maxFailures) { this.isOpen = true; setTimeout(() => this.reset(), this.timeout); } throw error; } } private reset() { this.failureCount = 0; this.isOpen = false; } } const circuitBreaker = new CircuitBreaker(3, 5000); const makeApiCall = () => { return new Promise((resolve, reject) => { // Simulating a slow API call setTimeout(() => { const randomNumber = Math.random(); if (randomNumber < 0.5) { resolve('API call successful'); } else { reject('API call failed'); } }, 2000); }); }; async function main() { try { const response = await circuitBreaker.executeRequest(makeApiCall); console.log(response); } catch (error) { console.log(error); } } main();
In this example, we have a CircuitBreaker
class that takes in the maximum number of failures allowed (maxFailures
) and a timeout duration (timeout
). The executeRequest
method is responsible for executing the API call wrapped inside the Circuit Breaker logic. If the Circuit Breaker is open (i.e., too many failures have occurred), it throws an error. Otherwise, it makes the API call and waits for the result. If the API call takes longer than the specified timeout, it throws a timeout error. If the API call fails, the failure count is incremented. If the failure count exceeds the maximum allowed failures, the Circuit Breaker is opened for a duration equal to the timeout. After the timeout period, the Circuit Breaker is reset and subsequent requests can be made.
Related Article: How to Get an Object Value by Dynamic Keys in TypeScript
How TypeScript Handles Fault Tolerance
TypeScript is a statically-typed superset of JavaScript that provides a robust type system and compile-time error checking. While TypeScript itself does not have built-in fault tolerance mechanisms, it provides a strong foundation for building fault-tolerant systems.
One way TypeScript helps in handling fault tolerance is through its type system. By enforcing type safety at compile-time, TypeScript helps catch many potential errors before they even occur. This reduces the likelihood of runtime errors and improves the overall reliability of the codebase. Additionally, TypeScript’s type system enables developers to express complex data structures and function signatures, making it easier to reason about the behavior of the code.
Another way TypeScript handles fault tolerance is by leveraging modern JavaScript runtime features. For example, TypeScript can take advantage of JavaScript’s built-in exception handling mechanism to handle errors gracefully. By using try-catch blocks, developers can catch and handle exceptions, preventing them from crashing the entire application. This allows for better error handling and recovery strategies, improving fault tolerance.
Furthermore, TypeScript’s support for asynchronous programming with Promises and async/await syntax enables developers to write more resilient code. By using Promises and async/await, developers can easily handle and propagate errors in asynchronous operations, making it easier to handle failures and implement retry or fallback mechanisms.
Let’s look at an example of how TypeScript can handle fault tolerance using Promises and async/await:
async function fetchData(): Promise { const response = await fetch('https://api.example.com/data'); if (!response.ok) { throw new Error('Failed to fetch data'); } return response.json(); } async function main() { try { const data = await fetchData(); console.log(data); } catch (error) { console.log(error); // Handle error and implement fallback logic } } main();
In this example, we have an async
function fetchData()
that fetches data from an API using the fetch
function. If the response is not ok (indicating an error), an error is thrown. The main()
function calls fetchData()
and handles any errors that occur. By using try-catch
blocks, we can catch errors and implement fallback logic, such as displaying an error message or retrying the operation.
Overall, TypeScript’s type system, exception handling mechanisms, and support for asynchronous programming provide developers with the tools they need to build fault-tolerant systems. By leveraging these features effectively, developers can write more reliable and resilient code.
Benefits of Using a Circuit Breaker in Microservices Architecture
Microservices architecture has gained popularity in recent years due to its scalability, maintainability, and flexibility. However, with the increasing complexity of distributed systems, it becomes challenging to handle failures and maintain system stability. This is where the Circuit Breaker pattern comes in, providing several benefits for microservices architectures:
1. Fault Isolation: The Circuit Breaker pattern helps in isolating failures and preventing them from cascading throughout the system. By detecting failures in external services or dependencies, the Circuit Breaker can temporarily block requests to the failing service, allowing other parts of the system to continue functioning without being affected.
2. Improved Resilience: By implementing a Circuit Breaker, microservices can handle failures and recover gracefully. The Circuit Breaker can introduce strategies such as retries, fallback mechanisms, and timeouts to handle failures and prevent them from impacting the overall system performance.
3. Reduced Latency: When a Circuit Breaker detects a failing service, it can quickly respond and prevent unnecessary requests from being made. This reduces the latency of the system by avoiding repeated calls to the failing service. Instead, the Circuit Breaker can return cached responses or fallback data, improving the overall responsiveness of the system.
4. Graceful Degradation: In scenarios where external services are experiencing high load or congestion, the Circuit Breaker can handle the situation gracefully. Instead of overwhelming the failing service with requests, the Circuit Breaker can return fallback responses or implement alternative strategies to ensure smooth operation of the system.
5. Visibility and Monitoring: Circuit Breakers often provide monitoring and metrics that give insights into the health and performance of the system. By monitoring the success and failure rates of requests, developers can identify potential issues and take proactive measures to improve the overall system reliability.
Let’s consider an example to illustrate the benefits of using a Circuit Breaker in a microservices architecture:
class UserService { private circuitBreaker: CircuitBreaker; constructor() { this.circuitBreaker = new CircuitBreaker(3, 5000); } public async getUser(userId: string): Promise { const request = async () => { const response = await fetch(`https://api.example.com/users/${userId}`); if (!response.ok) { throw new Error('Failed to fetch user'); } return response.json(); }; return this.circuitBreaker.executeRequest(request); } } const userService = new UserService(); async function main() { try { const user = await userService.getUser('123'); console.log(user); } catch (error) { console.log(error); // Handle error and implement fallback logic } } main();
In this example, we have a UserService
class that uses a Circuit Breaker to handle requests to an external user service API. The getUser
method makes an API call to fetch a user by ID. The API call is wrapped inside the Circuit Breaker’s executeRequest
method. If the Circuit Breaker detects failures or timeouts, it throws an error, allowing the caller to handle the error and implement fallback logic as needed.
Error Handling with Circuit Breaker in TypeScript
Error handling is a critical aspect of building robust and reliable applications. In TypeScript, error handling can be implemented effectively with the Circuit Breaker pattern to handle failures and ensure fault tolerance. The Circuit Breaker pattern provides mechanisms to detect and handle errors in a controlled manner, preventing failures from propagating throughout the system.
When using a Circuit Breaker in TypeScript, there are several ways to handle errors:
1. Throwing Errors: When an error occurs, such as a failed API call or a timeout, the Circuit Breaker can throw an error. This allows the caller to catch and handle the error appropriately. Throwing errors can be useful for implementing fallback logic or retry strategies.
2. Fallback Mechanism: In case of failures, the Circuit Breaker can provide a fallback mechanism to return default or cached data instead of the failing result. This helps in maintaining system availability and providing a graceful degradation of functionality.
3. Retry Mechanism: The Circuit Breaker can implement a retry mechanism to automatically retry failed operations. This can be useful in scenarios where transient failures occur, such as network issues or temporary unavailability of services. By retrying the operation after a certain delay, the Circuit Breaker can increase the chances of success.
4. Timeout Handling: Timeouts are a common source of errors in distributed systems. The Circuit Breaker can handle timeouts by waiting for a specified duration and then throwing an error if the operation does not complete within the given time. This ensures that the system does not get stuck indefinitely waiting for a response.
Let’s see an example of error handling with a Circuit Breaker in TypeScript:
class CircuitBreaker { private isOpen: boolean; private failureCount: number; constructor(private maxFailures: number, private timeout: number) { this.isOpen = false; this.failureCount = 0; } public async executeRequest(request: () => Promise): Promise { if (this.isOpen) { throw new Error('Circuit Breaker is open'); } try { const result = await Promise.race([ request(), new Promise((_, reject) => setTimeout(() => reject(new Error('Timeout')), this.timeout) ), ]); this.reset(); return result; } catch (error) { this.failureCount++; if (this.failureCount >= this.maxFailures) { this.isOpen = true; setTimeout(() => this.reset(), this.timeout); } throw error; } } private reset() { this.failureCount = 0; this.isOpen = false; } } const circuitBreaker = new CircuitBreaker(3, 5000); const makeApiCall = () => { return new Promise((resolve, reject) => { // Simulating a failed API call setTimeout(() => { reject(new Error('API call failed')); }, 2000); }); }; async function main() { try { const response = await circuitBreaker.executeRequest(makeApiCall); console.log(response); } catch (error) { console.log(error); // Handle error and implement fallback logic or retry strategy } } main();
In this example, we have a CircuitBreaker
class that handles errors using the Circuit Breaker pattern. The executeRequest
method wraps the API call inside a try-catch block. If the API call fails, the failure count is incremented. If the failure count exceeds the maximum allowed failures, the Circuit Breaker is opened, preventing further requests to the failing service. After a timeout period, the Circuit Breaker resets and subsequent requests can be made.
Related Article: Using ESLint & eslint-config-standard-with-typescript
Retry Mechanism in Circuit Breaker for TypeScript
The retry mechanism is an essential component of the Circuit Breaker pattern, allowing for automatic retries of failed operations. In TypeScript, the retry mechanism can be implemented within the Circuit Breaker to handle transient failures, such as network issues or temporary unavailability of services.
When a failure occurs, the retry mechanism in the Circuit Breaker can automatically retry the operation after a specified delay. This can increase the chances of success, especially in scenarios where failures are intermittent or short-lived.
To implement a retry mechanism in a Circuit Breaker for TypeScript, we can modify the executeRequest
method to include a retry logic. The retry mechanism can be implemented using a loop and a delay between retries.
Let’s take a look at an example of implementing a retry mechanism in a Circuit Breaker for TypeScript:
class CircuitBreaker { private isOpen: boolean; private failureCount: number; constructor( private maxFailures: number, private timeout: number, private retryCount: number, private retryDelay: number ) { this.isOpen = false; this.failureCount = 0; } public async executeRequest(request: () => Promise): Promise { if (this.isOpen) { throw new Error('Circuit Breaker is open'); } let retryAttempts = 0; while (retryAttempts < this.retryCount) { try { const result = await Promise.race([ request(), new Promise((_, reject) => setTimeout(() => reject(new Error('Timeout')), this.timeout) ), ]); this.reset(); return result; } catch (error) { this.failureCount++; if (this.failureCount >= this.maxFailures) { this.isOpen = true; setTimeout(() => this.reset(), this.timeout); throw error; } retryAttempts++; await new Promise((resolve) => setTimeout(resolve, this.retryDelay)); } } throw new Error('Maximum retry attempts exceeded'); } private reset() { this.failureCount = 0; this.isOpen = false; } } const circuitBreaker = new CircuitBreaker(3, 5000, 3, 1000); const makeApiCall = () => { return new Promise((resolve, reject) => { // Simulating a 50% chance of failure const randomNumber = Math.random(); if (randomNumber < 0.5) { resolve('API call successful'); } else { reject(new Error('API call failed')); } }); }; async function main() { try { const response = await circuitBreaker.executeRequest(makeApiCall); console.log(response); } catch (error) { console.log(error); // Handle error and implement fallback logic } } main();
In this example, we have extended the CircuitBreaker
class to include additional properties for the retry mechanism: retryCount
and retryDelay
. The executeRequest
method now includes a loop that attempts the operation multiple times. If the operation fails, the failure count is incremented. If the failure count exceeds the maximum allowed failures, the Circuit Breaker is opened as before. Within each iteration of the loop, a delay is introduced using setTimeout
before the next retry attempt.
Fallback Mechanism in TypeScript’s Circuit Breaker
The fallback mechanism is a crucial feature of the Circuit Breaker pattern, providing an alternative response or behavior when an operation fails. In TypeScript’s Circuit Breaker, the fallback mechanism can be implemented to mitigate the impact of failures and ensure graceful degradation.
When a failure occurs, the fallback mechanism in the Circuit Breaker can return default or cached data instead of the failing result. This helps maintain system availability and provides a seamless user experience even in the presence of failures.
To implement a fallback mechanism in TypeScript’s Circuit Breaker, we can modify the executeRequest
method to include a fallback logic. The fallback logic can be a separate function or a default value that is returned when the operation fails.
Let’s take a look at an example of implementing a fallback mechanism in TypeScript’s Circuit Breaker:
class CircuitBreaker { private isOpen: boolean; private failureCount: number; constructor(private maxFailures: number, private timeout: number) { this.isOpen = false; this.failureCount = 0; } public async executeRequest( request: () => Promise, fallback: () => any ): Promise { if (this.isOpen) { return fallback(); } try { const result = await Promise.race([ request(), new Promise((_, reject) => setTimeout(() => reject(new Error('Timeout')), this.timeout) ), ]); this.reset(); return result; } catch (error) { this.failureCount++; if (this.failureCount >= this.maxFailures) { this.isOpen = true; setTimeout(() => this.reset(), this.timeout); return fallback(); } throw error; } } private reset() { this.failureCount = 0; this.isOpen = false; } } const circuitBreaker = new CircuitBreaker(3, 5000); const makeApiCall = () => { return new Promise((resolve, reject) => { // Simulating a failed API call setTimeout(() => { reject(new Error('API call failed')); }, 2000); }); }; const fallbackFunction = () => { return 'Fallback data'; }; async function main() { try { const response = await circuitBreaker.executeRequest( makeApiCall, fallbackFunction ); console.log(response); } catch (error) { console.log(error); // Handle error and implement additional fallback logic if needed } } main();
In this example, we have modified the executeRequest
method of the CircuitBreaker
class to include a fallback
parameter. The fallback
parameter is a function that is called when the Circuit Breaker is open or when the operation fails. The executeRequest
method first checks if the Circuit Breaker is open and returns the result of the fallback
function. If the Circuit Breaker is not open, it attempts the operation and returns the result. If the operation fails, the failure count is incremented, and if the failure count exceeds the maximum allowed failures, the Circuit Breaker is opened, and the fallback
function is called.
Tracking Circuit State in Circuit Breaker for TypeScript
Tracking the state of the Circuit Breaker is an important aspect of implementing the Circuit Breaker pattern in TypeScript. By monitoring the state of the Circuit Breaker, we can make informed decisions about whether to allow or block requests to a failing service.
In TypeScript’s Circuit Breaker, we can track the state of the Circuit Breaker by maintaining a set of properties that represent the current state. These properties can include the failure count, the maximum allowed failures, the open/closed state, and any additional metrics or metadata that can help in monitoring and managing the Circuit Breaker.
Let’s take a look at an example of tracking the state of the Circuit Breaker in TypeScript:
class CircuitBreaker { private isOpen: boolean; private failureCount: number; constructor(private maxFailures: number, private timeout: number) { this.isOpen = false; this.failureCount = 0; } public async executeRequest(request: () => Promise): Promise { if (this.isOpen) { throw new Error('Circuit Breaker is open'); } try { const result = await Promise.race([ request(), new Promise((_, reject) => setTimeout(() => reject(new Error('Timeout')), this.timeout) ), ]); this.reset(); return result; } catch (error) { this.failureCount++; if (this.failureCount >= this.maxFailures) { this.isOpen = true; setTimeout(() => this.reset(), this.timeout); } throw error; } } public isOpen(): boolean { return this.isOpen; } public getFailureCount(): number { return this.failureCount; } private reset() { this.failureCount = 0; this.isOpen = false; } } const circuitBreaker = new CircuitBreaker(3, 5000); const makeApiCall = () => { return new Promise((resolve, reject) => { // Simulating a failed API call setTimeout(() => { reject(new Error('API call failed')); }, 2000); }); }; async function main() { try { const response = await circuitBreaker.executeRequest(makeApiCall); console.log(response); } catch (error) { console.log(error); // Handle error and implement fallback logic } console.log(`Circuit Breaker state: ${circuitBreaker.isOpen() ? 'open' : 'closed'}`); console.log(`Failure count: ${circuitBreaker.getFailureCount()}`); } main();
In this example, we have added two additional methods to the CircuitBreaker
class: isOpen()
and getFailureCount()
. The isOpen()
method returns a boolean indicating whether the Circuit Breaker is open or closed. The getFailureCount()
method returns the current failure count. These methods allow us to track the state of the Circuit Breaker and obtain metrics about its behavior.
Related Article: How to Work with Anonymous Classes in TypeScript
Role of Timeout in Circuit Breaker Implementation in TypeScript
Timeout plays a critical role in the implementation of the Circuit Breaker pattern in TypeScript. It helps in detecting and handling slow or unresponsive operations, preventing them from causing performance degradation or system failures.
In TypeScript’s Circuit Breaker, the timeout value specifies the maximum time allowed for an operation to complete. If the operation exceeds the timeout duration, it is considered a failure, and appropriate actions can be taken, such as throwing an error or triggering a fallback mechanism.
To implement a timeout in TypeScript’s Circuit Breaker, we can use the setTimeout
function to create a timer that triggers an action after a specified duration. This action can be used to handle the timeout scenario and take the necessary steps to handle the failure.
Let’s take a look at an example of implementing a timeout in a Circuit Breaker in TypeScript:
class CircuitBreaker { private isOpen: boolean; private failureCount: number; constructor(private maxFailures: number, private timeout: number) { this.isOpen = false; this.failureCount = 0; } public async executeRequest(request: () => Promise): Promise { if (this.isOpen) { throw new Error('Circuit Breaker is open'); } try { const result = await Promise.race([ request(), new Promise((_, reject) => setTimeout(() => reject(new Error('Timeout')), this.timeout) ), ]); this.reset(); return result; } catch (error) { this.failureCount++; if (this.failureCount >= this.maxFailures) { this.isOpen = true; setTimeout(() => this.reset(), this.timeout); } throw error; } } private reset() { this.failureCount = 0; this.isOpen = false; } } const circuitBreaker = new CircuitBreaker(3, 5000); const makeApiCall = () => { return new Promise((resolve, reject) => { // Simulating a slow API call setTimeout(() => { const randomNumber = Math.random(); if (randomNumber < 0.5) { resolve('API call successful'); } else { reject('API call failed'); } }, 2000); }); }; async function main() { try { const response = await circuitBreaker.executeRequest(makeApiCall); console.log(response); } catch (error) { console.log(error); // Handle error and implement fallback logic or retry strategy } } main();
In this example, we have modified the CircuitBreaker
class to include a timeout mechanism. The executeRequest
method uses Promise.race
to race between the API request and a timer created with setTimeout
. If the API request completes first, the result is returned. If the timer triggers first, a timeout error is thrown. The timeout duration is specified by the timeout
parameter passed to the CircuitBreaker
constructor.
Usage of Circuit Breaker in Other Programming Languages
The Circuit Breaker pattern is not exclusive to TypeScript or any specific programming language. It is a widely adopted pattern that can be implemented in various programming languages to handle failures and ensure fault tolerance in distributed systems.
Let’s take a look at the usage of Circuit Breaker in other programming languages:
1. Java: In Java, the Circuit Breaker pattern can be implemented using libraries such as Netflix Hystrix or Resilience4j. These libraries provide comprehensive support for implementing fault tolerance mechanisms, including Circuit Breakers, retries, fallbacks, and timeouts.
2. C#: In C#, the Circuit Breaker pattern can be implemented using libraries such as Polly or Microsoft.Extensions.Http. These libraries provide useful features for handling failures and implementing resilient communication with external services.
3. Python: In Python, the Circuit Breaker pattern can be implemented using libraries such as hystrix-python or CircuitBreaker. These libraries provide similar functionalities to other languages, allowing developers to handle failures and improve the resilience of their applications.
4. Ruby: In Ruby, the Circuit Breaker pattern can be implemented using libraries such as circuit_breaker or resilience. These libraries provide mechanisms for handling failures and implementing fault tolerance in Ruby applications.
5. Go: In Go, the Circuit Breaker pattern can be implemented using libraries such as github.com/afex/hystrix-go or github.com/sony/gobreaker. These libraries provide Circuit Breaker implementations specifically designed for Go applications.
These are just a few examples of how the Circuit Breaker pattern can be implemented in other programming languages. Each language may have its own set of libraries and frameworks that provide Circuit Breaker functionality. By leveraging these libraries, developers can improve the resilience and fault tolerance of their applications in various programming languages.
Popular TypeScript Libraries/Frameworks for Circuit Breaker Implementation
When implementing the Circuit Breaker pattern in TypeScript, there are several popular libraries and frameworks available that provide ready-to-use Circuit Breaker implementations. These libraries and frameworks can greatly simplify the implementation process and provide additional features for handling failures and improving fault tolerance.
Let’s take a look at some popular TypeScript libraries and frameworks for Circuit Breaker implementation:
1. Opossum: Opossum is a popular Circuit Breaker library for TypeScript and JavaScript. It provides a simple and lightweight implementation of the Circuit Breaker pattern with support for timeouts, fallbacks, and metrics. Opossum is widely used and well-documented, making it a popular choice for implementing Circuit Breakers in TypeScript applications.
2. Brakes: Brakes is another popular Circuit Breaker library for TypeScript and JavaScript. It offers a feature-rich implementation of the Circuit Breaker pattern with support for timeouts, fallbacks, retries, and circuit state tracking. Brakes provides a flexible and extensible API, allowing developers to customize the Circuit Breaker behavior according to their specific requirements.
3. CircuitBreaker-js: CircuitBreaker-js is a lightweight Circuit Breaker library for TypeScript and JavaScript. It focuses on simplicity and ease of use, providing a straightforward API for implementing Circuit Breakers. CircuitBreaker-js supports timeouts, fallbacks, and circuit state tracking, making it suitable for basic Circuit Breaker needs.
4. Cockatiel: Cockatiel is a Circuit Breaker library for TypeScript and JavaScript that emphasizes simplicity and configurability. It provides a concise API for implementing Circuit Breakers with support for timeouts, fallbacks, and circuit state tracking. Cockatiel offers a flexible configuration system, allowing developers to fine-tune the behavior of the Circuit Breaker.
5. Async-Circuit-Breaker: Async-Circuit-Breaker is a TypeScript library that provides a Circuit Breaker implementation specifically designed for asynchronous operations. It supports timeouts, retries, and fallbacks, making it suitable for handling failures in asynchronous contexts. Async-Circuit-Breaker offers a simple and intuitive API, making it easy to integrate into TypeScript applications.
These are just a few examples of popular TypeScript libraries and frameworks for Circuit Breaker implementation. Each library has its own set of features and trade-offs, so it’s important to evaluate them based on your specific requirements and project needs.
Related Article: Building a Rules Engine with TypeScript
External Sources
– Netflix Hystrix
– Resilience4j
– Polly
– CircuitBreaker
– github.com/afex/hystrix-go
– github.com/sony/gobreaker
– Opossum
– Brakes
– Cockatiel