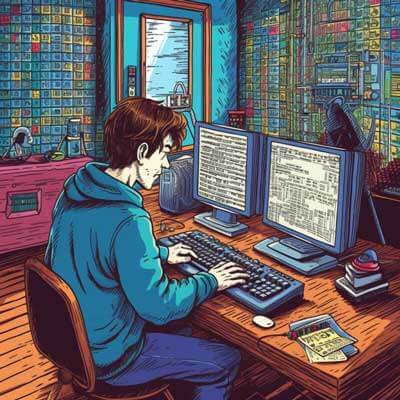
Table of Contents
Differentiating between string and number in TypeScript
One simple way to differentiate between a string and a number is to use the typeof
operator. The typeof
operator returns a string that represents the type of a value. For example, typeof "Hello"
returns "string"
and typeof 42
returns "number"
. Here is an example code snippet that demonstrates how to use the typeof
operator to differentiate between a string and a number:
function checkType(value: string | number) { if (typeof value === "string") { console.log("The value is a string"); } else if (typeof value === "number") { console.log("The value is a number"); } else { console.log("The value is neither a string nor a number"); } } checkType("Hello"); // Output: The value is a string checkType(42); // Output: The value is a number checkType(true); // Output: The value is neither a string nor a number
Another way to differentiate between a string and a number is to use the isNaN
function. The isNaN
function returns true
if the value is not a number, and false
otherwise. Here is an example code snippet that demonstrates how to use the isNaN
function to differentiate between a string and a number:
function checkType(value: string | number) { if (isNaN(Number(value))) { console.log("The value is a string"); } else { console.log("The value is a number"); } } checkType("Hello"); // Output: The value is a string checkType(42); // Output: The value is a number checkType(true); // Output: The value is a string
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
Using regular expressions to check if a string is numeric
Regular expressions provide a useful tool for pattern matching and can be used to check if a string is numeric in TypeScript. Regular expressions allow you to specify a pattern that a string must match. In the case of checking if a string is numeric, we can use a regular expression that matches any number.
Here is an example code snippet that demonstrates how to use a regular expression to check if a string is numeric:
function isNumeric(value: string) { return /^\d+$/.test(value); } console.log(isNumeric("123")); // Output: true console.log(isNumeric("abc")); // Output: false console.log(isNumeric("123abc")); // Output: false
In this code snippet, the regular expression ^\d+$
is used to match any sequence of one or more digits. The ^
character matches the start of the string, \d
matches any digit, and the +
quantifier specifies that the previous pattern should match one or more times. The $
character matches the end of the string. The test
method of the regular expression object is used to check if the pattern matches the value.
Checking if a string is an integer using regular expressions
Regular expressions can also be used to check if a string represents an integer in TypeScript. An integer is a whole number without a fractional or decimal part. We can use a regular expression that matches any sequence of one or more digits without any other characters.
Here is an example code snippet that demonstrates how to use a regular expression to check if a string is an integer:
function isInteger(value: string) { return /^\d+$/.test(value); } console.log(isInteger("123")); // Output: true console.log(isInteger("123.45")); // Output: false console.log(isInteger("abc")); // Output: false
In this code snippet, the regular expression ^\d+$
is used to match any sequence of one or more digits. The ^
character matches the start of the string, \d
matches any digit, and the +
quantifier specifies that the previous pattern should match one or more times. The $
character matches the end of the string. The test
method of the regular expression object is used to check if the pattern matches the value.
Validating a string as a number in TypeScript
Validating a string as a number in TypeScript involves checking if the string represents a valid numeric value. This means that the string can be converted to a number without any errors. TypeScript provides several methods to validate a string as a number.
One way to validate a string as a number is to use the isNaN
function. The isNaN
function returns true
if the value is not a number, and false
otherwise. By using the Number
constructor to convert the string to a number, we can determine if the string represents a valid numeric value.
Here is an example code snippet that demonstrates how to validate a string as a number using the isNaN
function:
function validateNumber(value: string) { return !isNaN(Number(value)); } console.log(validateNumber("123")); // Output: true console.log(validateNumber("123.45")); // Output: true console.log(validateNumber("abc")); // Output: false
In this code snippet, the isNaN
function is used to check if the value is not a number. The Number
constructor is used to convert the string to a number. If the conversion is successful and the value is a number, the isNaN
function will return false
. The !
operator is then used to negate the result, so that true
is returned if the value is a valid number.
Related Article: Tutorial on Exact Type in TypeScript
Validating a string as numeric using regular expressions
Regular expressions can also be used to validate a string as numeric in TypeScript. This involves checking if the string represents a valid numeric value according to a specified pattern. Regular expressions provide a flexible and useful way to define the pattern for validating numeric values.
Here is an example code snippet that demonstrates how to validate a string as numeric using a regular expression:
function validateNumeric(value: string) { return /^\d+(\.\d+)?$/.test(value); } console.log(validateNumeric("123")); // Output: true console.log(validateNumeric("123.45")); // Output: true console.log(validateNumeric("abc")); // Output: false
In this code snippet, the regular expression ^\d+(\.\d+)?$
is used to match any sequence of one or more digits, followed by an optional decimal part. The ^
character matches the start of the string, \d
matches any digit, and the +
quantifier specifies that the previous pattern should match one or more times. The (\.\d+)?
part matches an optional decimal part, consisting of a dot followed by one or more digits. The ?
quantifier makes the whole group optional. The $
character matches the end of the string. The test
method of the regular expression object is used to check if the pattern matches the value.
Validating a string as an integer in TypeScript
Validating a string as an integer in TypeScript involves checking if the string represents a valid whole number without a fractional or decimal part. This can be done using regular expressions or by converting the string to a number and checking if it is an integer.
Here is an example code snippet that demonstrates how to validate a string as an integer using regular expressions:
function validateInteger(value: string) { return /^\d+$/.test(value); } console.log(validateInteger("123")); // Output: true console.log(validateInteger("123.45")); // Output: false console.log(validateInteger("abc")); // Output: false
In this code snippet, the regular expression ^\d+$
is used to match any sequence of one or more digits. The ^
character matches the start of the string, \d
matches any digit, and the +
quantifier specifies that the previous pattern should match one or more times. The $
character matches the end of the string. The test
method of the regular expression object is used to check if the pattern matches the value.
Converting a string to a number in TypeScript
In TypeScript, you can convert a string to a number using the Number
constructor or by using type casting. The Number
constructor and type casting allow you to explicitly convert a string to a number.
Here is an example code snippet that demonstrates how to convert a string to a number using the Number
constructor:
let str = "42"; let num = Number(str); console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the Number
constructor is used to convert the string "42"
to a number. The resulting number is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Here is an example code snippet that demonstrates how to convert a string to a number using type casting:
let str = "42"; let num = +str; console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the +
operator is used for type casting the string "42"
to a number. The resulting number is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Converting a string to numeric data type in TypeScript
In TypeScript, you can convert a string to a numeric data type using the Number
constructor or by using type casting. The Number
constructor and type casting allow you to explicitly convert a string to a numeric data type.
Here is an example code snippet that demonstrates how to convert a string to a numeric data type using the Number
constructor:
let str = "42"; let num = Number(str); console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the Number
constructor is used to convert the string "42"
to a numeric data type. The resulting number is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Here is an example code snippet that demonstrates how to convert a string to a numeric data type using type casting:
let str = "42"; let num = +str; console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the +
operator is used for type casting the string "42"
to a numeric data type. The resulting number is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Related Article: How to Check if a String is in Enum in TypeScript: A Tutorial
Converting a string to an integer in TypeScript
In TypeScript, you can convert a string to an integer using the parseInt
function or by using type casting. The parseInt
function and type casting allow you to explicitly convert a string to an integer.
Here is an example code snippet that demonstrates how to convert a string to an integer using the parseInt
function:
let str = "42"; let num = parseInt(str); console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the parseInt
function is used to convert the string "42"
to an integer. The resulting integer is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Here is an example code snippet that demonstrates how to convert a string to an integer using type casting:
let str = "42"; let num = +str; console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the +
operator is used for type casting the string "42"
to an integer. The resulting integer is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
The isNaN function in TypeScript
The isNaN
function is a built-in function in TypeScript that is used to determine if a value is not a number. It returns true
if the value is not a number, and false
otherwise. The isNaN
function is useful when you need to check if a value is a valid number.
Here is an example code snippet that demonstrates how to use the isNaN
function:
console.log(isNaN(42)); // Output: false console.log(isNaN("42")); // Output: false console.log(isNaN("abc")); // Output: true
In this code snippet, the isNaN
function is used to check if the values 42
, "42"
, and "abc"
are not a number. The first two values are valid numbers, so isNaN
returns false
for them. The third value is not a valid number, so isNaN
returns true
for it.
Using the isNaN function to check if a string is not a number
The isNaN
function can be used to check if a string is not a number in TypeScript. By converting the string to a number using the Number
constructor, we can determine if the string is a valid numeric value. If the conversion fails, the isNaN
function will return true
, indicating that the string is not a number.
Here is an example code snippet that demonstrates how to use the isNaN
function to check if a string is not a number:
function isNotANumber(value: string) { return isNaN(Number(value)); } console.log(isNotANumber("123")); // Output: false console.log(isNotANumber("123.45")); // Output: false console.log(isNotANumber("abc")); // Output: true
In this code snippet, the isNaN
function is used to check if the values "123"
, "123.45"
, and "abc"
are not a number. The strings "123"
and "123.45"
can be successfully converted to numbers, so isNaN
returns false
for them. The string "abc"
cannot be converted to a number, so isNaN
returns true
for it.
Applying the isNaN function to validate a string as not a number
The isNaN
function can be applied to validate a string as not a number in TypeScript. By converting the string to a number using the Number
constructor, we can determine if the string is a valid numeric value. If the conversion fails, the isNaN
function will return true
, indicating that the string is not a number.
Here is an example code snippet that demonstrates how to apply the isNaN
function to validate a string as not a number:
function validateNotANumber(value: string) { return isNaN(Number(value)); } console.log(validateNotANumber("123")); // Output: false console.log(validateNotANumber("123.45")); // Output: false console.log(validateNotANumber("abc")); // Output: true
In this code snippet, the isNaN
function is used to validate the values "123"
, "123.45"
, and "abc"
as not a number. The strings "123"
and "123.45"
can be successfully converted to numbers, so isNaN
returns false
for them. The string "abc"
cannot be converted to a number, so isNaN
returns true
for it.
Related Article: Tutorial: Checking if a Value is in Enum in TypeScript
Implementing a custom isNaN function in TypeScript
Although TypeScript provides a built-in isNaN
function, you can also implement a custom isNaN
function to check if a value is not a number. This can be useful if you have specific requirements or if you want to extend the functionality of the built-in isNaN
function.
Here is an example code snippet that demonstrates how to implement a custom isNaN
function in TypeScript:
function customIsNaN(value: any) { return typeof value === "number" && isNaN(value); } console.log(customIsNaN(42)); // Output: false console.log(customIsNaN("42")); // Output: true console.log(customIsNaN("abc")); // Output: false
In this code snippet, the customIsNaN
function is implemented to check if the values 42
, "42"
, and "abc"
are not a number. The function first checks if the type of the value is "number"
using the typeof
operator. If the type is "number"
, the function calls the built-in isNaN
function to determine if the value is not a number. If the type is not "number"
, the function returns false
.
Using isNaN in TypeScript to check if a string is not numeric
The isNaN
function can be utilized in TypeScript to check if a string is not numeric. By converting the string to a number using the Number
constructor, we can determine if the string is a valid numeric value. If the conversion fails, the isNaN
function will return true
, indicating that the string is not numeric.
Here is an example code snippet that demonstrates how to utilize the isNaN
function to check if a string is not numeric:
function isNotNumeric(value: string) { return isNaN(Number(value)); } console.log(isNotNumeric("123")); // Output: false console.log(isNotNumeric("123.45")); // Output: false console.log(isNotNumeric("abc")); // Output: true
In this code snippet, the isNaN
function is utilized to check if the values "123"
, "123.45"
, and "abc"
are not numeric. The strings "123"
and "123.45"
can be successfully converted to numbers, so isNaN
returns false
for them. The string "abc"
cannot be converted to a number, so isNaN
returns true
for it.
Leveraging isNaN to validate a string as not numeric
The isNaN
function can be leveraged to validate a string as not numeric in TypeScript. By converting the string to a number using the Number
constructor, we can determine if the string is a valid numeric value. If the conversion fails, the isNaN
function will return true
, indicating that the string is not numeric.
Here is an example code snippet that demonstrates how to leverage the isNaN
function to validate a string as not numeric:
function validateNotNumeric(value: string) { return isNaN(Number(value)); } console.log(validateNotNumeric("123")); // Output: false console.log(validateNotNumeric("123.45")); // Output: false console.log(validateNotNumeric("abc")); // Output: true
In this code snippet, the isNaN
function is leveraged to validate the values "123"
, "123.45"
, and "abc"
as not numeric. The strings "123"
and "123.45"
can be successfully converted to numbers, so isNaN
returns false
for them. The string "abc"
cannot be converted to a number, so isNaN
returns true
for it.
Applying type casting to convert a string to a number in TypeScript
Type casting can be applied to convert a string to a number in TypeScript. Type casting allows you to explicitly specify the type of a value, in this case, converting the string to a number. By using the +
operator or the parseInt
function, you can perform type casting to convert the string to a number.
Here is an example code snippet that demonstrates how to apply type casting to convert a string to a number in TypeScript:
let str = "42"; let num = +str; console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the +
operator is used for type casting the string "42"
to a number. The resulting number is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Here is an example code snippet that demonstrates how to apply type casting using the parseInt
function to convert a string to a number in TypeScript:
let str = "42"; let num = parseInt(str); console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the parseInt
function is used for type casting the string "42"
to a number. The resulting number is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Related Article: Tutorial on Prisma Enum with TypeScript
Using the parseInt function to convert a string to an integer in TypeScript
The parseInt
function can be utilized to convert a string to an integer in TypeScript. By using the parseInt
function, you can explicitly convert the string to an integer, allowing you to perform mathematical operations or comparisons with the string as an integer value.
Here is an example code snippet that demonstrates how to utilize the parseInt
function to convert a string to an integer in TypeScript:
let str = "42"; let num = parseInt(str); console.log(typeof num); // Output: number console.log(num); // Output: 42
In this code snippet, the parseInt
function is utilized to convert the string "42"
to an integer. The resulting integer is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.
Here is an example code snippet that demonstrates how to utilize the parseInt
function with a radix parameter to convert a string to an integer in TypeScript:
let str = "1010"; let num = parseInt(str, 2); console.log(typeof num); // Output: number console.log(num); // Output: 10
In this code snippet, the parseInt
function is utilized with a radix parameter of 2
to convert the binary string "1010"
to an integer. The resulting integer is stored in the variable num
. The typeof
operator is used to check the type of num
, which is "number"
. The value of num
is then logged to the console.