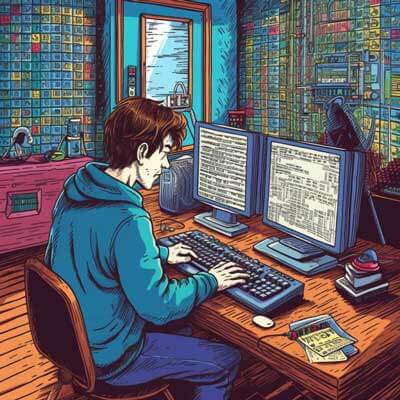
- What is Go?
- Go Example Code
- What is TypeScript?
- TypeScript Example Code
- Static Typing Definition
- Example of Using Static Typing with Go
- Static Typing & TypeScript
- Exploring Type Safety
- Example with Go
- Example with TypeScript
- Comparing Transpiler and Compiler
- Go & TypeScript Examples
- Type Annotations in Go and TypeScript
- Go Example
- TypeScript Example
- Type Inference in Go and TypeScript
- Go Example
- TypeScript Example
- Type Checking in Go and TypeScript
- Go Example
- TypeScript Example
- Handling Runtime Errors in Go
- Go Example
- Development Speed in Go and TypeScript
- Examples
- Comparing Go and TypeScript
- Web Development: Go vs. TypeScript
- Advantages of TypeScript over Go
- TypeScript as a Replacement for Go
- Projects Suited for Go instead of TypeScript
- Benefits of Go’s Concurrency Model
- Example
- Runtime Error Handling in TypeScript vs. Go
- TypeScript Example
- Go Example
- Performance Differences: Go vs. TypeScript
- Development Speed in Go vs. TypeScript
- External Sources
What is Go?
Go, also known as Golang, is an open-source programming language developed by Google. It was created by Robert Griesemer, Rob Pike, and Ken Thompson in 2007 and was released to the public in 2009. Go is designed to be simple, efficient, and easy to use for developing scalable and concurrent applications.
One of the key features of Go is its focus on simplicity and readability. The creators of Go aimed to create a programming language that could be easily understood and maintained by developers. Go achieves this by providing a small and concise set of features and a minimalistic syntax.
Go is statically typed, which means that variable types are checked at compile-time. It also supports garbage collection, memory safety, and built-in support for concurrent programming. These features make Go a popular choice for developing high-performance applications, especially in areas such as networking, distributed systems, and web development.
Related Article: How to Work with Anonymous Classes in TypeScript
Go Example Code
package main import "fmt" func main() { fmt.Println("Hello, world!") }
In this example, we have a simple Go program that prints “Hello, world!” to the console. The fmt
package provides functions for formatted I/O, and the Println
function is used to print the text.
What is TypeScript?
TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. It was developed by Microsoft and first released in 2012. TypeScript adds optional static typing to JavaScript, which allows developers to catch errors and bugs at compile-time rather than runtime.
One of the main advantages of TypeScript is its ability to provide static type checking. This means that variables, function parameters, and return types can be explicitly declared with their respective types. TypeScript’s type system allows developers to catch common mistakes and provides better tooling support, such as code completion and refactoring.
TypeScript also introduces other features not present in JavaScript, such as classes, interfaces, and modules. These features enable developers to write more structured and maintainable code, especially in large-scale applications.
TypeScript Example Code
function greet(name: string): string { return "Hello, " + name + "!"; } console.log(greet("world"));
In this example, we have a TypeScript function greet
that takes a name
parameter of type string
and returns a string. The name
parameter is explicitly declared with its type, allowing the TypeScript compiler to perform type checking. The function then concatenates the name
parameter with the string “Hello, ” and returns the result. Finally, the console.log
function is used to print the result to the console.
Static Typing Definition
Static typing is a feature of programming languages that requires variables to have their types declared at compile-time. This means that variable types are checked by the compiler before the program is executed. Static typing helps catch errors and bugs early in the development process.
In Go, static typing is enforced by the compiler. Variables must have their types declared explicitly, and the compiler performs type checking to ensure that variables are used correctly. This helps eliminate runtime errors and improves code quality.
Example of Using Static Typing with Go
package main import "fmt" func main() { var name string = "John" var age int = 30 fmt.Println("Name:", name) fmt.Println("Age:", age) }
In this example, we have two variables, name
and age
, with their types declared explicitly. The name
variable has the type string
, and the age
variable has the type int
. The compiler checks that the variables are assigned values of the correct types.
In TypeScript, static typing is also enforced by the compiler. Variables can have their types declared explicitly or inferred by the compiler based on their assigned values. TypeScript’s static typing helps catch errors and provides better tooling support.
Static Typing & TypeScript
let name: string = "John"; let age: number = 30; console.log("Name:", name); console.log("Age:", age);
In this example, we have two variables, name
and age
, with their types declared explicitly. The name
variable has the type string
, and the age
variable has the type number
. The TypeScript compiler checks that the variables are assigned values of the correct types.
Exploring Type Safety
Type safety is a concept in programming languages that ensures that operations are performed only on values of the correct types. Type-safe languages prevent common errors and bugs that can occur when values of incompatible types are mixed.
In Go, type safety is enforced by the compiler. Variables must have their types declared explicitly, and the compiler performs type checking to ensure that variables are used correctly. This helps prevent type-related errors and improves code reliability.
Example with Go
package main import "fmt" func main() { var age int = 30 var name string = "John" var result = age + name // Error: invalid operation: age + name (mismatched types int and string) fmt.Println(result) }
In this example, we have an error because the +
operator is used to add an int
variable (age
) with a string
variable (name
). This operation is not allowed in Go because the types are incompatible. The compiler detects this error during compilation and prevents the program from running.
In TypeScript, type safety is also enforced by the compiler. Variables can have their types declared explicitly or inferred by the compiler based on their assigned values. TypeScript’s type system helps catch type-related errors and provides better tooling support.
Example with TypeScript
let age: number = 30; let name: string = "John"; let result = age + name; // Error: Operator '+' cannot be applied to types 'number' and 'string'. console.log(result);
In this example, we have an error because the +
operator is used to add a number
variable (age
) with a string
variable (name
). This operation is not allowed in TypeScript because the types are incompatible. The TypeScript compiler detects this error during compilation and prevents the program from running.
Comparing Transpiler and Compiler
Both Go and TypeScript are compiled languages, but they differ in their approach to compilation.
Go is a compiled language, which means that the source code is compiled directly into machine code that can be executed by the target platform. The Go compiler, called “gc”, translates Go source code into machine code for a specific operating system and architecture. The resulting binary can be executed directly without the need for an interpreter or runtime environment.
On the other hand, TypeScript is a transpiled language. It is transpiled into JavaScript, which can be executed by any JavaScript runtime environment. The TypeScript compiler, called “tsc”, translates TypeScript source code into JavaScript, preserving the static types and other language features. The resulting JavaScript code can be executed in web browsers or server-side environments.
The main difference between a compiler and a transpiler is the target output. A compiler produces machine code or bytecode for a specific platform, while a transpiler produces code in a different language, typically one that is more widely supported.
Go & TypeScript Examples
package main import "fmt" func main() { fmt.Println("Hello, world!") }
This is a simple Go program. To compile it, we can use the go build
command:
$ go build main.go
This command will generate an executable file named main
, which can be executed on the target platform.
function greet(name: string): string { return "Hello, " + name + "!"; } console.log(greet("world"));
This is a simple TypeScript program. To transpile it into JavaScript, we can use the tsc
command:
$ tsc main.ts
This command will generate a JavaScript file named main.js
, which can be executed in a JavaScript runtime environment.
Type Annotations in Go and TypeScript
Type annotations are used to explicitly declare the types of variables, function parameters, and return values. They provide additional information to the compiler or transpiler and help catch errors early in the development process.
In Go, type annotations are required for variables, function parameters, and return values. Types are declared using the var
keyword followed by the variable name and the type. Function parameters and return values are declared with the type before the variable name.
Go Example
package main import "fmt" func main() { var name string = "John" var age int = 30 fmt.Println("Name:", name) fmt.Println("Age:", age) } func add(a int, b int) int { return a + b }
In this example, the name
variable is declared with the type string
, and the age
variable is declared with the type int
. The add
function takes two parameters, a
and b
, both of type int
, and returns an int
value.
In TypeScript, type annotations are optional thanks to type inference. The compiler can often infer the types based on the assigned values. However, type annotations can still be used to provide explicit type information or when type inference is not possible.
TypeScript Example
let name: string = "John"; let age: number = 30; console.log("Name:", name); console.log("Age:", age); function add(a: number, b: number): number { return a + b; }
In this example, the name
variable is declared with the type string
, and the age
variable is declared with the type number
. The add
function takes two parameters, a
and b
, both of type number
, and returns a number
value.
Type Inference in Go and TypeScript
Type inference is a feature of programming languages that allows the compiler or transpiler to automatically determine the types of variables based on the assigned values. This eliminates the need for explicit type annotations in many cases and makes the code more concise and readable.
In Go, type inference is limited. Variables must have their types declared explicitly using type annotations. The Go compiler does not perform extensive type inference like some other languages.
Go Example
package main import "fmt" func main() { var name = "John" // type string (inferred) var age = 30 // type int (inferred) fmt.Println("Name:", name) fmt.Println("Age:", age) }
In this example, the types of the name
and age
variables are inferred by the Go compiler based on their assigned values. The name
variable is inferred as a string
, and the age
variable is inferred as an int
.
In TypeScript, type inference is more useful. The TypeScript compiler can infer types based on the assigned values, function return types, and other contextual information. This allows for more concise code without sacrificing type safety.
TypeScript Example
let name = "John"; // type string (inferred) let age = 30; // type number (inferred) console.log("Name:", name); console.log("Age:", age); function add(a: number, b: number) { return a + b; // return type number (inferred) }
In this example, the types of the name
and age
variables are inferred by the TypeScript compiler based on their assigned values. The name
variable is inferred as a string
, and the age
variable is inferred as a number
. The add
function’s return type is also inferred as a number
based on the return statement.
Type Checking in Go and TypeScript
Type checking is the process of verifying that variables, function parameters, and return values have the correct types. It is performed by the compiler or transpiler to ensure type safety and catch errors early in the development process.
In Go, type checking is performed by the Go compiler. It checks that variables are assigned values of the correct types, function parameters are passed values of the correct types, and function return values match the declared types.
Go Example
package main import "fmt" func main() { var name string = "John" var age int = 30 fmt.Println("Name:", name) fmt.Println("Age:", age) var result = add(10, 20) // Error: cannot use 10 (type untyped int) as type string in argument to add fmt.Println("Result:", result) } func add(a int, b int) int { return a + b }
In this example, the Go compiler detects an error because the add
function is called with two arguments of type int
, but the function expects two arguments of type string
. This error is caught during compilation, preventing the program from running.
In TypeScript, type checking is also performed by the TypeScript compiler. It checks that variables are assigned values of the correct types, function parameters are passed values of the correct types, and function return values match the declared types.
TypeScript Example
let name: string = "John"; let age: number = 30; console.log("Name:", name); console.log("Age:", age); let result = add(10, 20); // Error: Argument of type 'number' is not assignable to parameter of type 'string'. console.log("Result:", result); function add(a: string, b: string): string { return a + b; }
In this example, the TypeScript compiler detects an error because the add
function is called with two arguments of type number
, but the function expects two arguments of type string
. This error is caught during compilation, preventing the program from running.
Handling Runtime Errors in Go
Runtime errors are errors that occur while a program is running. They can be caused by a variety of factors, such as invalid input, division by zero, or accessing an array out of bounds. Handling runtime errors is important to ensure that the program behaves correctly and does not crash.
In Go, runtime errors are typically handled using error values. Functions can return an additional error value to indicate whether an operation was successful or encountered an error. This allows the calling code to handle the error appropriately.
Go Example
package main import ( "fmt" "os" ) func main() { file, err := os.Open("file.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() // Code to read and process the file }
In this example, the os.Open
function is used to open a file named “file.txt”. If the file cannot be opened for any reason, the function returns an error value. The calling code checks if the error value is not nil
, indicating that an error occurred. If an error is detected, it is printed to the console and the program exits early. The defer
statement is used to ensure that the file is closed before the function returns, even in the case of an error.
Development Speed in Go and TypeScript
Development speed is an important factor in software development. The speed at which developers can write, test, and deploy code directly impacts the time it takes to deliver software to users. Both Go and TypeScript offer features that can improve development speed.
In Go, the focus on simplicity and minimalistic syntax allows developers to write code quickly and concisely. Go’s standard library provides a wide range of pre-built packages that can be used to quickly develop common functionality. Additionally, the Go ecosystem offers a variety of third-party libraries and frameworks that can further accelerate development.
In TypeScript, the ability to catch errors at compile-time and provide better tooling support helps improve development speed. The type system helps prevent common mistakes and provides auto-completion, code navigation, and refactoring tools that can save time during development. TypeScript’s integration with modern IDEs and development tools enhances the developer experience and speeds up the development process.
Examples
package main import "fmt" func main() { fmt.Println("Hello, world!") }
This is a simple Go program that prints “Hello, world!” to the console. The code is concise, and the Go compiler quickly verifies that there are no syntax errors.
function greet(name: string): string { return "Hello, " + name + "!"; } console.log(greet("world"));
This is a simple TypeScript program that greets the provided name. The code is concise, and the TypeScript compiler quickly verifies that there are no type errors.
Comparing Go and TypeScript
Go and TypeScript are both popular programming languages, but they have different strengths and use cases. Understanding the differences between the two can help developers choose the right tool for their projects.
Go is designed for systems programming and is well-suited for building high-performance, concurrent, and scalable applications. It has a minimalistic syntax and a small set of features, which makes it easy to learn and use. Go’s focus on simplicity and efficiency has made it a popular choice for networked and distributed systems, as well as other performance-critical applications.
TypeScript, on the other hand, is a superset of JavaScript and is primarily used for web development. It adds optional static typing to JavaScript, which helps catch errors and provides better tooling support. TypeScript’s type system allows for more structured and maintainable code, especially in large-scale applications. It also offers features not present in Go, such as classes, interfaces, and modules.
When choosing between Go and TypeScript, it is important to consider the specific requirements of the project. If performance and concurrency are crucial, Go may be the better choice. If web development and type safety are the main concerns, TypeScript may be a more suitable option.
Web Development: Go vs. TypeScript
Web development is a broad field, and both Go and TypeScript have their strengths and weaknesses when it comes to building web applications. Understanding the differences between the two can help developers choose the right tool for their web development projects.
Go excels at building high-performance web servers and APIs. It has a built-in HTTP server library that makes it easy to handle incoming requests and serve responses. Go’s efficient concurrency model allows it to handle large numbers of concurrent connections without sacrificing performance. Go’s simplicity and minimalistic syntax also make it easy to write clean and maintainable server-side code.
TypeScript, on the other hand, is widely used for front-end web development. It can be used to build complex web applications using modern frameworks like Angular or React. TypeScript’s static typing provides better tooling support and enables developers to write more structured and maintainable code. TypeScript’s integration with popular web development tools and frameworks makes it a popular choice for building interactive and scalable web applications.
When choosing between Go and TypeScript for web development, it is important to consider the specific requirements of the project. If building high-performance APIs or server-side applications is the main goal, Go may be the better choice. If front-end web development or building complex web applications is the focus, TypeScript may be more suitable.
Advantages of TypeScript over Go
While both Go and TypeScript are useful programming languages, TypeScript offers several advantages over Go, especially in the context of web development.
The main advantage of TypeScript is its static typing. TypeScript’s type system allows developers to catch errors at compile-time and provides better tooling support. With static typing, developers can find and fix errors early in the development process, which can save time and improve code quality. TypeScript’s type system also enables code completion, code navigation, and refactoring tools that enhance the developer experience.
Another advantage of TypeScript is its integration with popular web development frameworks and libraries. TypeScript can be used with frameworks like Angular, React, and Vue.js, which provide a rich set of tools and components for building modern web applications. The combination of TypeScript and these frameworks offers a seamless development experience and allows for more structured and maintainable code.
TypeScript also benefits from the large JavaScript ecosystem. There are countless libraries and packages available for JavaScript, and TypeScript can use these libraries directly. This allows developers to leverage existing code and solutions, saving time and effort in development.
TypeScript as a Replacement for Go
While TypeScript offers many advantages for web development, it may not be a suitable replacement for Go in all cases. Go’s focus on simplicity, efficiency, and concurrency makes it a useful choice for building high-performance, scalable, and concurrent applications.
If performance and concurrency are critical requirements for a project, Go may be a better choice. Go’s efficient concurrency model and low-level control make it well-suited for systems programming, networked applications, and other performance-critical tasks.
However, if web development or front-end development is the main focus, TypeScript can be a strong alternative to Go. TypeScript’s static typing, tooling support, and integration with popular web frameworks make it a useful choice for building complex and scalable web applications.
Ultimately, the choice between TypeScript and Go depends on the specific requirements and goals of the project. It is important to consider factors such as performance, concurrency, type safety, tooling support, and the existing skill set of the development team.
Projects Suited for Go instead of TypeScript
While TypeScript is a useful language for web development, there are certain types of projects that are better suited for Go. Go’s focus on simplicity, efficiency, and concurrency makes it a strong choice for specific use cases.
Projects that require high performance, scalability, and concurrency are well-suited for Go. Go’s efficient concurrency model and low-level control allow developers to build highly concurrent applications without sacrificing performance. Examples of projects that benefit from Go’s concurrency model include networked applications, distributed systems, and real-time applications.
Go is also a good choice for system-level programming and infrastructure development. Its simplicity and minimalistic syntax make it easy to write clean and maintainable code, even for complex systems. Go’s standard library provides a wide range of packages for system-level tasks, such as file I/O, networking, and cryptography.
Additionally, Go’s built-in support for cross-compilation and static linking makes it ideal for building standalone executables that can be deployed on different platforms without additional dependencies. This makes Go a popular choice for developing command-line tools, utilities, and microservices.
Benefits of Go’s Concurrency Model
Go’s concurrency model is one of its most useful features. It provides a simple and efficient way to write concurrent programs, allowing developers to take full advantage of modern multi-core processors and distributed systems.
Go’s concurrency model is based on goroutines and channels. Goroutines are lightweight threads of execution that can be created easily and efficiently. Channels are communication primitives that allow goroutines to send and receive values. Goroutines and channels provide a convenient and safe way to share data and synchronize the execution of concurrent tasks.
The benefits of Go’s concurrency model include:
– Efficient use of resources: Goroutines are lightweight and have a small memory footprint. They can be created and destroyed quickly, allowing for the efficient use of system resources.
– Simplified synchronization: Channels provide a safe and efficient way to synchronize the execution of concurrent tasks. They eliminate the need for low-level synchronization primitives, such as locks and condition variables, which can be error-prone and hard to reason about.
– Simplified error handling: Go’s error handling mechanism, based on the error
type and the defer
statement, works seamlessly with goroutines and channels. Errors can be propagated through channels, allowing for centralized error handling and graceful shutdown of concurrent tasks.
– Scalability: Go’s concurrency model is designed to scale with the number of available CPU cores. Goroutines can be easily spawned and scheduled across multiple cores, allowing for efficient parallelism.
– Distributed systems: Go’s concurrency model is well-suited for building distributed systems. Goroutines and channels can be used to communicate between different parts of a distributed system, making it easy to coordinate the execution of tasks across multiple machines.
Example:
package main import ( "fmt" "time" ) func main() { // Create a channel for communication between goroutines ch := make(chan int) // Start a goroutine that sends values to the channel go func() { for i := 0; i < 10; i++ { ch <- i time.Sleep(time.Second) } close(ch) }() // Start a goroutine that receives values from the channel go func() { for value := range ch { fmt.Println("Received:", value) } }() // Wait for the goroutines to finish time.Sleep(5 * time.Second) }
In this example, two goroutines are created: one that sends values to a channel (ch
) and one that receives values from the channel. The sender goroutine sends values from 0 to 9 to the channel, with a delay of one second between each value. The receiver goroutine prints the received values to the console. The main goroutine waits for five seconds before exiting.
Go’s concurrency model makes it easy to write concurrent programs that can take advantage of modern multi-core processors and distributed systems. It simplifies the process of writing scalable and efficient concurrent code, making Go a useful choice for performance-critical applications.
Runtime Error Handling in TypeScript vs. Go
Runtime errors are errors that occur while a program is running. Handling runtime errors correctly is important to ensure that the program behaves correctly and does not crash. Both TypeScript and Go provide mechanisms for handling runtime errors.
In TypeScript, runtime errors can be handled using try-catch blocks. The try block contains the code that may throw an error, and the catch block handles the error if it occurs. TypeScript’s error handling mechanism is based on JavaScript’s try-catch-finally syntax.
TypeScript Example
try { // Code that may throw an error let result = 1 / 0; console.log(result); } catch (error) { // Handling the error console.log("Error:", error); }
In this example, the code inside the try block attempts to divide 1 by 0, which will result in a runtime error (division by zero). The catch block catches the error and prints it to the console.
In Go, runtime errors can be handled using error values. Functions can return an additional error value to indicate whether an operation was successful or encountered an error. The calling code can then check the error value and handle the error appropriately.
Go Example
package main import ( "fmt" "os" ) func main() { file, err := os.Open("file.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() // Code to read and process the file }
In this example, the os.Open
function is used to open a file named “file.txt”. If the file cannot be opened for any reason, the function returns an error value. The calling code checks if the error value is not nil
, indicating that an error occurred. If an error is detected, it is printed to the console.
Both TypeScript and Go provide mechanisms for handling runtime errors, allowing developers to handle errors gracefully and prevent program crashes. TypeScript’s try-catch syntax is familiar to developers coming from JavaScript, while Go’s error handling mechanism provides a flexible and explicit way to handle errors. The choice between the two depends on the specific requirements and preferences of the project.
Performance Differences: Go vs. TypeScript
Performance is an important consideration when choosing a programming language for a specific project. Both Go and TypeScript offer good performance, but there are some differences that developers should be aware of.
Go is designed for performance and efficiency. Its minimalistic syntax and efficient garbage collector make it a good choice for high-performance applications. Go’s built-in support for concurrency and its efficient scheduler allow it to handle large numbers of concurrent connections without sacrificing performance. Go’s compiled nature also contributes to its performance, as it can generate machine code that is optimized for the target platform.
TypeScript, on the other hand, is a superset of JavaScript and runs on JavaScript engines. While TypeScript itself does not provide any performance optimizations, it benefits from the performance improvements made to JavaScript engines over the years. JavaScript engines have become highly optimized, and modern browsers can execute JavaScript code very efficiently. Additionally, TypeScript’s static typing can help catch type-related errors at compile-time, which can improve performance by preventing runtime errors.
When it comes to performance, the choice between Go and TypeScript depends on the specific requirements of the project. If performance is a critical factor and the project involves high-performance applications, Go may be the better choice. If the project is web-focused and performance is not the primary concern, TypeScript’s performance, combined with its type safety and tooling support, can be a good fit.
Development Speed in Go vs. TypeScript
Development speed is an important factor in software development. The speed at which developers can write, test, and deploy code directly impacts the time it takes to deliver software to users. Both Go and TypeScript offer features that can improve development speed.
Go’s focus on simplicity and minimalistic syntax allows developers to write code quickly and concisely. Go’s standard library provides a wide range of pre-built packages that can be used to quickly develop common functionality. Additionally, the Go ecosystem offers a variety of third-party libraries and frameworks that can further accelerate development.
TypeScript’s static typing provides better tooling support and enables developers to write more structured and maintainable code. TypeScript’s integration with modern IDEs and development tools enhances the developer experience and speeds up the development process. Additionally, TypeScript’s type system helps catch errors at compile-time, reducing the time spent debugging and fixing issues.
When it comes to development speed, the choice between Go and TypeScript depends on the specific requirements of the project. If simplicity and performance are the main concerns, Go may be the better choice. If web development and type safety are the focus, TypeScript can accelerate development and improve code quality.
External Sources
– Go Official Website: https://golang.org/
– TypeScript Official Website: https://www.typescriptlang.org/