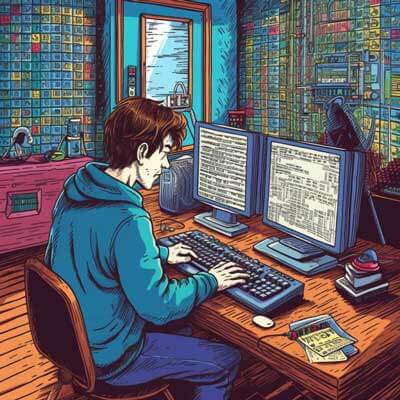
- Returning a Value in TypeScript
- Exiting a TypeScript Process
- Defining an Exit Function in TypeScript
- Understanding Exit Codes in TypeScript
- Exiting a TypeScript Process Gracefully
- Handling Exit Events in TypeScript
- Exiting a TypeScript Application
- Exiting a TypeScript Script
- Exiting a TypeScript Script with an Error
- Handling Errors and Exits in TypeScript
- External Sources
Returning a Value in TypeScript
In TypeScript, functions can return values using the return
keyword. The return type of a function is specified after the closing parenthesis of the function parameters.
Here’s an example of a TypeScript function that returns a string:
function greet(name: string): string { return "Hello, " + name; } console.log(greet("John")); // Output: Hello, John
In this example, the greet
function takes a name
parameter of type string and returns a string. The console.log
statement calls the greet
function with the argument “John” and logs the returned value to the console.
Related Article: How to Implement and Use Generics in Typescript
Exiting a TypeScript Process
In TypeScript, exiting a process refers to terminating the execution of a program or script. When a process exits, all resources allocated by the program are released, and the operating system regains control.
There are several ways to exit a TypeScript process, depending on the context in which the code is running. In a browser environment, you can simply close the browser tab or window to exit the process. In a Node.js environment, you can use the process.exit
method to exit the process programmatically.
Defining an Exit Function in TypeScript
To define an exit function in TypeScript, you can use the process.exit
method provided by the Node.js runtime. The process.exit
method accepts an optional exit code parameter, which indicates the reason for the process exit.
Here’s an example of defining an exit function in TypeScript:
function exitProcess(exitCode: number): void { process.exit(exitCode); } exitProcess(0); // Exit with code 0 (success)
In this example, the exitProcess
function takes an exitCode
parameter of type number and calls the process.exit
method with the provided exit code.
Understanding Exit Codes in TypeScript
Exit codes are used to indicate the outcome of a process execution. In TypeScript, exit codes can be used to communicate success, failure, or other specific conditions to the calling environment.
Commonly used exit codes in TypeScript include:
– 0
: Indicates successful process execution.
– 1
: Indicates a generic error condition.
– 2
: Indicates a misuse of the command line or incorrect arguments.
Exit codes can be used to implement error handling or to provide information about the execution status of a process.
Related Article: How to Check If a String is in an Enum in TypeScript
Exiting a TypeScript Process Gracefully
When exiting a TypeScript process, it is often desirable to perform cleanup tasks or notify other components of the imminent exit. Graceful process termination involves handling exit events and ensuring that all necessary cleanup operations are completed before the process exits.
To exit a TypeScript process gracefully, you can listen for the beforeExit
event emitted by the process
object in Node.js. This event is emitted when the Node.js event loop is about to exit.
Here’s an example of exiting a TypeScript process gracefully:
process.on("beforeExit", () => { console.log("Performing cleanup tasks before exit..."); // Perform cleanup tasks here }); console.log("Exiting process..."); process.exit(0);
In this example, the beforeExit
event is registered using the process.on
method. Inside the event handler, you can perform any necessary cleanup tasks before the process exits.
Handling Exit Events in TypeScript
In addition to the beforeExit
event, the Node.js process
object provides other exit-related events that can be useful for handling process termination.
– exit
: This event is emitted when the process is about to exit. It is triggered after the beforeExit
event and can be used to perform final cleanup tasks or log exit information.
process.on("exit", (exitCode) => { console.log(`Process exited with code ${exitCode}`); });
In this example, the exit
event is registered using the process.on
method. The event handler receives the exit code as a parameter and can perform any necessary cleanup or logging tasks.
Exiting a TypeScript Application
To exit a TypeScript application in a Node.js environment, you can use the process.exit
method as mentioned earlier. The process.exit
method terminates the Node.js process with the specified exit code.
Here’s an example of exiting a TypeScript application:
function exitApplication(): void { // Perform necessary cleanup tasks here process.exit(0); } exitApplication();
In this example, the exitApplication
function performs any necessary cleanup tasks before calling process.exit
with an exit code of 0
to indicate a successful exit.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Exiting a TypeScript Script
When working with TypeScript scripts, you can exit the script by calling the process.exit
method, just like in a TypeScript application.
Here’s an example of exiting a TypeScript script:
function exitScript(): void { // Perform necessary cleanup tasks here process.exit(0); } exitScript();
In this example, the exitScript
function performs any necessary cleanup tasks before calling process.exit
with an exit code of 0
to indicate a successful exit.
Exiting a TypeScript Script with an Error
In some cases, you may need to exit a TypeScript script with an error condition. This can be achieved by calling the process.exit
method with a non-zero exit code.
Here’s an example of exiting a TypeScript script with an error:
function exitWithError(): void { console.error("An error occurred. Exiting script..."); process.exit(1); } exitWithError();
In this example, the exitWithError
function logs an error message to the console before calling process.exit
with an exit code of 1
to indicate an error condition.
Handling Errors and Exits in TypeScript
Error handling and proper process termination are essential in TypeScript applications to ensure stability and reliability. TypeScript provides various mechanisms for handling errors and exits.
One approach is to use try-catch blocks to catch and handle errors. Additionally, you can use the process.on
method to listen for unhandled exceptions and handle them accordingly.
Here’s an example of handling errors and exits in TypeScript:
try { // Code that may throw an error throw new Error("Something went wrong"); } catch (error) { console.error("Caught an error:", error); process.exit(1); } process.on("uncaughtException", (error) => { console.error("Uncaught exception:", error); process.exit(1); });
In this example, the try-catch block is used to catch any thrown errors and log them to the console before calling process.exit
with an exit code of 1
. Additionally, the uncaughtException
event is registered using the process.on
method to handle uncaught exceptions and exit the process.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript