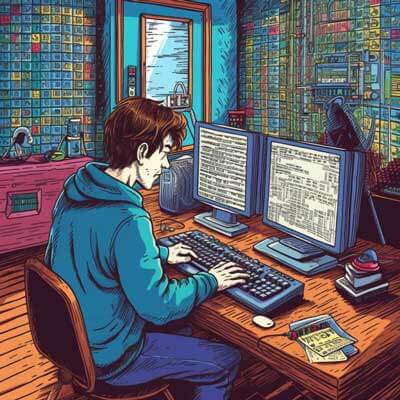
- Introduction to Typescript and Ts-Node
- Setting Up Your Development Environment with Ts-Node
- Running Ts-Node in the Context of Data Handling
- Use Cases: Querying Data with Ts-Node
- Use Cases: Updating Data with Typescript and Ts-Node
- Use Cases: Typescript and Ts-Node for Data Migration Tasks
- Best Practices: Coding Conventions in Typescript and Ts-Node
- Best Practices: Efficient Data Retrieval with Ts-Node
- Real World Examples: Using Ts-Node in Data-Intensive Web Applications
- Real World Examples: Ts-Node in Data Analysis Applications
- Performance Considerations: Ts-Node and Query Speed
- Performance Considerations: Memory Management with Typescript and Ts-Node
- Advanced Techniques: Debugging Ts-Node Applications
- Advanced Techniques: Using Ts-Node with ORM Tools
- Code Snippet: Connect to a Data Source with Ts-Node
- Code Snippet: Execute a Query with Ts-Node
- Code Snippet: Error Handling in Typescript with Ts-Node
- Code Snippet: Handle Query Results with Ts-Node
- Code Snippet: Close a Connection with Ts-Node
- Error Handling: Managing Connection Errors with Ts-Node
- Error Handling: Handling Query Errors with Typescript and Ts-Node
Introduction to Typescript and Ts-Node
Typescript is a strongly typed superset of JavaScript that compiles to plain JavaScript code. It adds static types to JavaScript, allowing developers to catch errors and improve code quality during the development process. Ts-Node is a popular tool for running TypeScript code directly in Node.js without the need for transpiling to JavaScript.
To get started with Typescript and Ts-Node, you will need to have Node.js and Typescript installed on your machine. Once installed, you can create a new Typescript project and configure Ts-Node as the runtime for executing your Typescript code.
Here’s an example of how to set up a basic Typescript project with Ts-Node:
1. Create a new directory for your project and navigate to it using the command line:
mkdir my-project cd my-project
2. Initialize a new Node.js project:
npm init -y
3. Install the required dependencies:
npm install typescript ts-node
4. Create a new file named index.ts
and add some sample Typescript code:
// index.ts const message: string = 'Hello, world!'; console.log(message);
5. Open the package.json
file and add a new script to execute the Typescript code using Ts-Node:
"scripts": { "start": "ts-node index.ts" }
6. Run the Typescript code using Ts-Node:
npm start
Related Article: Tutorial: Using React-Toastify with TypeScript
Setting Up Your Development Environment with Ts-Node
Setting up a development environment with Ts-Node involves configuring the necessary tools and libraries to streamline your coding workflow. This includes setting up a code editor, installing relevant plugins or extensions, and configuring Ts-Node to work seamlessly with your project.
Here’s an example of how to set up your development environment with Ts-Node:
1. Choose a code editor that supports Typescript. Some popular options include Visual Studio Code, Atom, and WebStorm.
2. Install the necessary plugins or extensions for your code editor to provide support for Typescript. These plugins usually include features like syntax highlighting, code completion, and error checking.
3. Open your project in your chosen code editor.
4. Configure your code editor to use Ts-Node as the default runtime for executing Typescript code. This ensures that your code editor can run your Typescript code directly without the need for transpiling.
5. Configure any additional build tools or linters to work with Ts-Node. For example, if you are using a task runner like Gulp or Grunt, make sure to update your build scripts to use Ts-Node.
By setting up your development environment with Ts-Node, you can enjoy the benefits of running Typescript code directly in Node.js without the hassle of transpiling. This allows for a more efficient and streamlined development process.
Running Ts-Node in the Context of Data Handling
Running Ts-Node in the context of data handling involves utilizing Ts-Node to interact with databases and manipulate data. Ts-Node provides a convenient way to write and execute Typescript code that communicates with databases using various database drivers or ORMs.
Here’s an example of how to run Ts-Node in the context of data handling:
1. Install the necessary database driver or ORM library for your preferred database. For example, if you are using PostgreSQL, you can install the pg
library:
npm install pg
2. Import the necessary modules and configure the database connection in your Typescript code:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); // Example query pool.query('SELECT * FROM users', (error, results) => { if (error) { console.error('Error executing query:', error); } else { console.log('Query results:', results.rows); } });
3. Run your Typescript code using Ts-Node:
ts-node index.ts
By running Ts-Node in the context of data handling, you can easily write Typescript code to interact with databases and perform various data manipulation tasks.
Use Cases: Querying Data with Ts-Node
Querying data is a common use case when working with databases. Ts-Node provides a straightforward way to execute SQL queries or use query builders to retrieve data from databases.
Here’s an example of how to query data with Ts-Node:
1. Install the necessary database driver or ORM library for your preferred database. For example, if you are using MySQL, you can install the mysql2
library:
npm install mysql2
2. Import the necessary modules and configure the database connection in your Typescript code:
import { createConnection } from 'mysql2/promise'; async function runQuery() { const connection = await createConnection({ host: 'your-hostname', user: 'your-username', password: 'your-password', database: 'your-database', }); try { const [rows] = await connection.execute('SELECT * FROM users'); console.log('Query results:', rows); } catch (error) { console.error('Error executing query:', error); } finally { connection.end(); } } runQuery();
3. Run your Typescript code using Ts-Node:
ts-node index.ts
By utilizing Ts-Node for querying data, you can easily retrieve information from databases and process it in your Typescript code.
Use Cases: Updating Data with Typescript and Ts-Node
Updating data in databases is another common use case. Ts-Node allows you to execute SQL update statements or use query builders to modify data in databases.
Here’s an example of how to update data with Typescript and Ts-Node:
1. Install the necessary database driver or ORM library for your preferred database. For example, if you are using MongoDB, you can install the mongodb
library:
npm install mongodb
2. Import the necessary modules and configure the database connection in your Typescript code:
import { MongoClient } from 'mongodb'; async function updateData() { const client = new MongoClient('mongodb://your-hostname:27017'); try { await client.connect(); const db = client.db('your-database'); const collection = db.collection('your-collection'); const result = await collection.updateOne({ name: 'John' }, { $set: { age: 30 } }); console.log('Update result:', result); } catch (error) { console.error('Error updating data:', error); } finally { client.close(); } } updateData();
3. Run your Typescript code using Ts-Node:
ts-node index.ts
By leveraging Typescript and Ts-Node for updating data, you can easily modify information in databases based on your specific requirements.
Use Cases: Typescript and Ts-Node for Data Migration Tasks
Data migration is a crucial aspect of managing databases. Ts-Node can be a valuable tool for automating data migration tasks by allowing you to write Typescript scripts that handle the migration process.
Here’s an example of how to use Typescript and Ts-Node for data migration tasks:
1. Install the necessary database driver or ORM library for your preferred database. For example, if you are using SQLite, you can install the sqlite3
library:
npm install sqlite3
2. Import the necessary modules and configure the database connection in your Typescript code:
import sqlite3 from 'sqlite3'; const db = new sqlite3.Database('your-database.sqlite'); // Example migration script db.serialize(() => { db.run('CREATE TABLE IF NOT EXISTS users (id INT PRIMARY KEY, name TEXT)'); const stmt = db.prepare('INSERT INTO users (id, name) VALUES (?, ?)'); for (let i = 1; i <= 10; i++) { stmt.run(i, `User ${i}`); } stmt.finalize(); }); db.close();
3. Run your Typescript code using Ts-Node:
ts-node migration.ts
By utilizing Typescript and Ts-Node for data migration tasks, you can automate the process of altering database structures and populating data, ensuring consistency and efficiency in your database management.
Best Practices: Coding Conventions in Typescript and Ts-Node
Following coding conventions is essential for maintaining clean and readable code. When working with Typescript and Ts-Node, it’s important to adhere to best practices to ensure consistency and collaboration within your development team.
Here are some coding conventions to consider when using Typescript and Ts-Node:
1. Use meaningful variable and function names: Choose descriptive names that accurately convey the purpose and functionality of your code elements. Avoid using single-letter variable names or cryptic abbreviations.
2. Follow consistent indentation: Use a consistent indentation style, such as two spaces or four spaces, to enhance code readability. Avoid mixing spaces and tabs for indentation.
3. Use type annotations: Explicitly define the types of your variables, function parameters, and return values using type annotations. This helps catch errors during development and improves code understanding for other developers.
4. Avoid unnecessary type assertions: Only use type assertions when necessary. Type assertions should be used sparingly and only when you are confident about the type. Instead, rely on type inference as much as possible.
5. Use interfaces and types for complex data structures: Define interfaces or types for complex data structures to provide clarity and ensure consistency in your codebase.
6. Utilize TypeScript’s strict mode: Enable TypeScript’s strict mode by setting the strict
flag to true
in your tsconfig.json
file. This enforces stricter type checking and helps catch potential errors at compile-time.
7. Organize your code into modules: Use TypeScript’s module system to structure your code into logical modules. This improves code maintainability and allows for better code reusability.
Here’s an example of adhering to coding conventions in Typescript and Ts-Node:
interface User { id: number; name: string; age?: number; } function greetUser(user: User): void { console.log(`Hello, ${user.name}!`); } const user: User = { id: 1, name: 'John Doe', }; greetUser(user);
Best Practices: Efficient Data Retrieval with Ts-Node
Efficient data retrieval is crucial for achieving optimal performance when working with databases. When using Ts-Node for data retrieval, there are several best practices to consider to improve the efficiency of your code.
Here are some best practices for efficient data retrieval with Ts-Node:
1. Limit the number of returned rows: When querying data, specify the maximum number of rows to retrieve using the LIMIT
clause. This reduces unnecessary data transfer and improves query performance.
2. Optimize your database queries: Analyze your queries and ensure they are properly optimized. Use appropriate indexes, avoid unnecessary joins, and consider denormalizing your data when necessary.
3. Use pagination for large result sets: When dealing with large result sets, implement pagination to retrieve data in smaller chunks. This prevents memory overload and improves the responsiveness of your application.
4. Utilize caching mechanisms: Implement caching mechanisms to store frequently accessed data in memory. This reduces the need for repetitive database queries and improves overall performance.
5. Use asynchronous operations: Leverage asynchronous operations when retrieving data to avoid blocking the event loop. This allows your application to handle concurrent requests efficiently.
6. Minimize data transfer: Only retrieve the necessary columns and fields from the database. Avoid retrieving unused or unnecessary data to reduce network latency and improve query performance.
Here’s an example of efficient data retrieval with Ts-Node using pagination:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); const pageSize = 10; // Number of rows to retrieve per page const pageNumber = 1; // Page number to retrieve const offset = (pageNumber - 1) * pageSize; pool.query(`SELECT * FROM users LIMIT ${pageSize} OFFSET ${offset}`, (error, results) => { if (error) { console.error('Error executing query:', error); } else { console.log('Query results:', results.rows); } }); pool.end();
Real World Examples: Using Ts-Node in Data-Intensive Web Applications
Ts-Node can be effectively utilized in data-intensive web applications to handle complex data processing, database interactions, and performance optimizations. Here are some real-world examples of how Ts-Node can be used in data-intensive web applications:
1. Real-time Analytics Dashboard: Ts-Node can be used to process and analyze large volumes of real-time data from various sources. It can handle complex data transformations and calculations, and interact with databases to store and retrieve analytics data.
2. E-commerce Inventory Management: Ts-Node can be used to manage e-commerce inventory systems, including real-time stock updates, order processing, and inventory tracking. It can interface with databases to update inventory quantities, manage product listings, and generate reports.
3. Social Media Monitoring: Ts-Node can be used to monitor social media platforms for specific keywords or hashtags, retrieve relevant data, and perform sentiment analysis. It can interact with databases to store and analyze social media data for insights and reporting.
4. Big Data Processing: Ts-Node can be used in big data processing pipelines to handle data ingestion, transformation, and analysis. It can interface with distributed data processing frameworks like Apache Spark or Apache Flink to process massive datasets efficiently.
These are just a few examples of how Ts-Node can be applied in data-intensive web applications. By leveraging Ts-Node’s flexibility and performance, developers can build robust and scalable solutions for handling complex data workflows.
Real World Examples: Ts-Node in Data Analysis Applications
Ts-Node can be used in data analysis applications to perform statistical analysis, generate visualizations, and derive insights from data. Here are some real-world examples of how Ts-Node can be used in data analysis applications:
1. Financial Data Analysis: Ts-Node can be used to analyze financial data, including stock prices, market trends, and portfolio performance. It can handle data manipulation, perform calculations, and generate visualizations to aid in financial decision-making.
2. Machine Learning Pipelines: Ts-Node can be utilized in machine learning pipelines for data preprocessing, model training, and evaluation. It can interface with machine learning libraries like TensorFlow or scikit-learn to process data and build predictive models.
3. Natural Language Processing: Ts-Node can be used in natural language processing tasks, including text classification, sentiment analysis, and named entity recognition. It can interact with NLP libraries like Natural or TensorFlow.js to process text data and extract meaningful insights.
4. Customer Behavior Analysis: Ts-Node can be employed in customer behavior analysis applications to analyze user interactions, identify patterns, and segment customers. It can interface with databases to retrieve and process customer data for analysis.
These examples demonstrate how Ts-Node can be a valuable tool in data analysis applications, enabling developers to efficiently process and derive insights from large and complex datasets.
Performance Considerations: Ts-Node and Query Speed
When working with databases and executing queries using Ts-Node, query speed is an important consideration for optimal performance. Here are some performance considerations to keep in mind when working with Ts-Node and query speed:
1. Indexing: Ensure that appropriate indexes are created on the relevant columns in your database tables. Indexing can significantly improve query performance by allowing the database engine to locate and retrieve data more efficiently.
2. Query Optimization: Analyze your query execution plans and identify areas for improvement. Consider using query optimization techniques such as rewriting queries, adding hints, or restructuring the database schema to enhance query performance.
3. Connection Pooling: Utilize connection pooling to reuse database connections instead of establishing a new connection for each query. Connection pooling reduces the overhead of establishing connections and improves overall query performance.
4. Caching: Implement caching mechanisms to store frequently accessed query results in memory. Caching can significantly reduce the need for repetitive database queries and improve query speed.
5. Batch Processing: Whenever possible, batch process multiple queries together instead of executing individual queries. Batch processing reduces the overhead of multiple round trips to the database and improves overall query performance.
6. Data Denormalization: Consider denormalizing your database schema for frequently accessed data to improve query performance. Denormalization involves duplicating data across multiple tables to eliminate the need for complex joins and improve query speed.
By considering these performance considerations when working with Ts-Node and executing queries, you can optimize query speed and improve the overall performance of your database operations.
Performance Considerations: Memory Management with Typescript and Ts-Node
Efficient memory management is crucial for optimizing the performance of your Typescript and Ts-Node applications, especially when dealing with large datasets or processing-intensive tasks. Here are some performance considerations for memory management:
1. Minimize Memory Footprint: Avoid unnecessary memory allocations by minimizing the creation of temporary objects or variables. Reuse existing objects or variables whenever possible to reduce memory usage.
2. Dispose of Unused Resources: Release any resources or memory that are no longer needed. This includes closing database connections, releasing file handles, or disposing of large data structures when they are no longer required.
3. Use Streams for Large Data Sets: When dealing with large datasets, consider using streams or iterators to process data in smaller chunks. This reduces the memory footprint by only loading a portion of the data into memory at a time.
4. Avoid Memory Leaks: Be mindful of potential memory leaks, such as circular references or long-lived objects that are no longer needed. Ensure that all resources are properly released and garbage collected to prevent memory leaks.
5. Analyze Memory Usage: Monitor and analyze the memory usage of your application using tools like heap profilers or memory profilers. Identify any memory-intensive areas of your code and optimize them for better memory management.
6. Use Weak References: Consider using weak references when dealing with caches or long-lived objects that may not be needed all the time. Weak references allow objects to be garbage collected when they are no longer strongly referenced.
By considering these performance considerations for memory management in Typescript and Ts-Node applications, you can optimize memory usage and improve the overall performance and stability of your code.
Advanced Techniques: Debugging Ts-Node Applications
Debugging Ts-Node applications is crucial for identifying and resolving issues in your code. Ts-Node provides several advanced debugging techniques that can help streamline the debugging process.
Here are some advanced debugging techniques for Ts-Node applications:
1. Debugging with breakpoints: Place breakpoints in your Typescript code to pause execution at specific lines. This allows you to inspect variables, step through code, and identify the source of issues.
2. Debugging with console.log: Use console.log statements strategically to log relevant information during runtime. This can help you understand the flow of your code and identify problematic areas.
3. Remote debugging: Ts-Node allows remote debugging by exposing a debugging port. This enables you to connect a remote debugger, such as the Chrome DevTools, to your running Ts-Node application for real-time debugging.
4. Debugging with VS Code: If you are using Visual Studio Code as your code editor, it provides excellent built-in support for debugging Ts-Node applications. You can set breakpoints, inspect variables, and step through code using the integrated debugger.
5. Debugging with source maps: Ts-Node generates source maps by default, which map the compiled JavaScript code back to the original Typescript code. This allows you to debug your Typescript code directly, even though it is running as JavaScript.
By utilizing these advanced debugging techniques, you can efficiently diagnose and resolve issues in your Ts-Node applications, improving the overall quality and stability of your code.
Advanced Techniques: Using Ts-Node with ORM Tools
Ts-Node can be seamlessly integrated with Object-Relational Mapping (ORM) tools to simplify database interactions and improve code maintainability. ORM tools provide a higher-level abstraction for working with databases, allowing you to work with objects instead of writing raw SQL queries.
Here are some popular ORM tools that can be used with Ts-Node:
1. TypeORM: TypeORM is a feature-rich ORM tool for Typescript and JavaScript. It supports a wide range of databases and provides a powerful query builder, entity management, and migration capabilities.
2. Sequelize: Sequelize is a popular ORM tool for Node.js that supports multiple databases. It provides a simple and intuitive API for querying, inserting, updating, and deleting data, as well as support for transactions and associations.
3. Prisma: Prisma is a modern ORM and database toolkit that offers type-safe database access, automatic migrations, and powerful query capabilities. It integrates seamlessly with Ts-Node and provides a developer-friendly API for working with databases.
Integrating Ts-Node with an ORM tool involves configuring the ORM tool to use Ts-Node as the runtime for executing Typescript code and defining database models and relationships using the ORM’s syntax.
Here’s an example of using TypeORM with Ts-Node:
1. Install TypeORM and the necessary database driver:
npm install typeorm pg
2. Create a tsconfig.json
file in your project directory with the following configuration:
{ "compilerOptions": { "target": "es6", "module": "commonjs", "moduleResolution": "node", "esModuleInterop": true, "outDir": "dist", "sourceMap": true } }
3. Create a typeorm.config.ts
file in your project directory with the following configuration:
module.exports = { type: 'postgres', host: 'your-hostname', port: 5432, username: 'your-username', password: 'your-password', database: 'your-database', synchronize: true, logging: true, entities: ['src/entities/**/*.ts'], migrations: ['src/migrations/**/*.ts'], subscribers: ['src/subscribers/**/*.ts'], cli: { entitiesDir: 'src/entities', migrationsDir: 'src/migrations', subscribersDir: 'src/subscribers', }, };
4. Create a User.ts
file in the src/entities
directory with the following code:
import { Entity, PrimaryGeneratedColumn, Column } from 'typeorm'; @Entity() export class User { @PrimaryGeneratedColumn() id: number; @Column() name: string; @Column() age: number; }
5. Write your business logic in a Typescript file, for example, index.ts
:
import { createConnection } from 'typeorm'; import { User } from './entities/User'; async function main() { const connection = await createConnection(); const userRepository = connection.getRepository(User); const users = await userRepository.find(); console.log('Users:', users); connection.close(); } main().catch((error) => { console.error('Error:', error); });
6. Run your Typescript code using Ts-Node:
ts-node src/index.ts
By integrating Ts-Node with an ORM tool, you can simplify database interactions, improve code maintainability, and leverage the advanced features provided by the ORM tool.
Code Snippet: Connect to a Data Source with Ts-Node
Connecting to a data source, such as a database, is a crucial step in working with Ts-Node. Ts-Node provides various libraries and modules that facilitate connecting to different data sources.
Here’s a code snippet that demonstrates how to connect to a PostgreSQL database using Ts-Node:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); pool.connect((error, client, release) => { if (error) { console.error('Error connecting to the database:', error); } else { console.log('Connected to the database'); // Perform database operations here release(); pool.end(); } });
In this code snippet, a connection pool is created using the pg
library, which is a popular PostgreSQL client for Node.js. The connection pool allows multiple connections to be established and reused, improving performance and scalability.
Once the connection is established, you can perform database operations using the client
object provided in the callback function. After completing the operations, remember to release the client and end the connection pool to free up resources.
By utilizing Ts-Node and the appropriate libraries, you can easily connect to various data sources and interact with them using Typescript.
Code Snippet: Execute a Query with Ts-Node
Executing queries is a common task when working with databases in Ts-Node. Ts-Node provides libraries and modules that simplify the process of executing queries and retrieving results.
Here’s a code snippet that demonstrates how to execute a SQL query using Ts-Node and the pg
library for PostgreSQL:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); pool.query('SELECT * FROM users', (error, result) => { if (error) { console.error('Error executing query:', error); } else { console.log('Query result:', result.rows); } pool.end(); });
In this code snippet, a SQL query is executed using the query
method provided by the pg
library. The result of the query is obtained in the callback function, where you can handle any errors and process the query result as needed.
By using Ts-Node and the appropriate libraries, you can easily execute queries and retrieve results from databases in your Typescript applications.
Code Snippet: Error Handling in Typescript with Ts-Node
Error handling is an essential aspect of writing robust and reliable applications. Ts-Node provides error handling mechanisms that allow you to catch and handle errors effectively in your Typescript code.
Here’s a code snippet that demonstrates basic error handling in Typescript with Ts-Node:
try { // Code that may throw an error const result = 10 / 0; console.log('Result:', result); } catch (error) { // Handle the error console.error('An error occurred:', error); }
In this code snippet, the try
block contains the code that may throw an error. If an error occurs, it is caught by the catch
block, where you can handle the error appropriately. The error object contains information about the error, such as the error message and stack trace.
By using try-catch blocks, you can gracefully handle errors and prevent your application from crashing or producing incorrect results.
Code Snippet: Handle Query Results with Ts-Node
Handling query results is an important part of working with databases in Ts-Node. Ts-Node provides mechanisms to process and manipulate query results in a convenient manner.
Here’s a code snippet that demonstrates how to handle query results using the pg
library for PostgreSQL in Ts-Node:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); pool.query('SELECT * FROM users', (error, result) => { if (error) { console.error('Error executing query:', error); } else { const rows = result.rows; console.log('Query result:', rows); // Process the query result here rows.forEach((row) => { console.log('User:', row.name); }); } pool.end(); });
In this code snippet, the query result is obtained in the callback function, where you can handle any errors and process the result as needed. The result.rows
property contains an array of rows returned by the query, which you can iterate over and process individually.
By using Ts-Node and the appropriate libraries, you can easily handle query results and perform further operations on the retrieved data.
Code Snippet: Close a Connection with Ts-Node
Closing a connection is an important step when working with databases in Ts-Node. Ts-Node provides mechanisms to gracefully close connections and release any resources used by the database client.
Here’s a code snippet that demonstrates how to close a connection using the pg
library for PostgreSQL in Ts-Node:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); pool.connect((error, client, release) => { if (error) { console.error('Error connecting to the database:', error); } else { console.log('Connected to the database'); // Perform database operations here release(); pool.end((error) => { if (error) { console.error('Error closing the connection pool:', error); } else { console.log('Connection pool closed'); } }); } });
In this code snippet, the connection is established using the connect
method provided by the pg
library. After performing the necessary database operations, the connection is released by calling the release
function. Finally, the connection pool is closed using the end
method, and any errors are handled appropriately.
By properly closing connections and releasing resources, you can prevent resource leaks and ensure efficient usage of database connections in your Ts-Node applications.
Error Handling: Managing Connection Errors with Ts-Node
Managing connection errors is crucial when working with databases in Ts-Node. Ts-Node provides error handling mechanisms that allow you to handle connection errors effectively and gracefully.
Here’s an example of managing connection errors with Ts-Node using the pg
library for PostgreSQL:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); pool.connect((error, client, release) => { if (error) { console.error('Error connecting to the database:', error); } else { console.log('Connected to the database'); // Perform database operations here release(); } }); pool.on('error', (error) => { console.error('Unexpected error on idle client:', error); process.exit(-1); });
In this example, the connection is established using the connect
method provided by the pg
library. If an error occurs during the connection process, it is caught in the callback function and an appropriate error message is logged.
Additionally, the connection pool listens for the ‘error’ event, which is emitted when an idle client encounters an error. In the event handler, the error is logged, and the application is terminated with an exit code of -1.
By effectively managing connection errors with Ts-Node, you can handle unexpected issues and ensure the stability and reliability of your database connections.
Error Handling: Handling Query Errors with Typescript and Ts-Node
Handling query errors is essential to ensure the reliability and stability of your Ts-Node applications. Ts-Node provides mechanisms to catch and handle query errors effectively.
Here’s an example of handling query errors with Typescript and Ts-Node using the pg
library for PostgreSQL:
import { Pool } from 'pg'; const pool = new Pool({ user: 'your-username', host: 'your-hostname', database: 'your-database', password: 'your-password', port: 5432, }); pool.query('SELECT * FROM non_existing_table', (error, result) => { if (error) { console.error('Error executing query:', error); // Handle the query error here } else { console.log('Query result:', result.rows); } pool.end(); });
In this example, a query is executed using the query
method provided by the pg
library. If an error occurs during the query execution, it is caught in the callback function, and an appropriate error message is logged.
You can handle the query error based on your application’s requirements. This may include displaying an error message to the user, logging the error for debugging purposes, or performing any necessary error recovery steps.
By effectively handling query errors with Typescript and Ts-Node, you can ensure the robustness and stability of your database interactions.