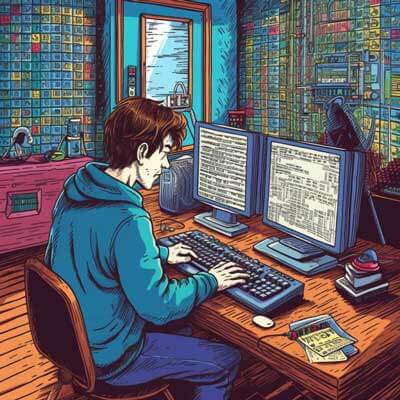
- Type Checking in TypeScript
- Static Typing in TypeScript
- Type Inference in TypeScript
- Type Annotations in TypeScript
- Type System in TypeScript
- Type Compatibility in TypeScript
- Type Assertions in TypeScript
- Type Declaration in TypeScript
- Type Alias in TypeScript
- Type Narrowing in TypeScript
- Type Checking in TypeScript – Explained
- Static Typing in TypeScript – Explained
- How Type Inference Works in TypeScript
- Understanding Type Annotations in TypeScript
- What is a Type System in TypeScript?
- Type Compatibility in TypeScript – Explained
- What are Type Assertions in TypeScript?
- Declaring Types in TypeScript
- Type Alias in TypeScript – Explained
Type Checking in TypeScript
TypeScript is a statically typed superset of JavaScript that adds static typing capabilities to the language. Type checking is an essential feature of TypeScript that helps catch errors and bugs at compile-time rather than runtime. It ensures that variables, functions, and objects are used correctly based on their declared types.
Let’s take a look at an example to understand how type checking works in TypeScript:
// Declaring a variable with a specific type let message: string = "Hello, TypeScript!"; // Trying to assign a number to a string variable message = 42; // Error: Type 'number' is not assignable to type 'string'
In this example, we declare a variable message
with the type string
. When we try to assign a number to this variable, TypeScript throws an error at compile-time, indicating that the types are not compatible.
TypeScript uses static type checking, which means that type checks are performed during the compilation process rather than at runtime. This allows developers to catch potential errors early in the development cycle and improve the overall reliability of their code.
Related Article: How to Implement and Use Generics in Typescript
Static Typing in TypeScript
Static typing is a fundamental feature of TypeScript that allows developers to specify types explicitly for variables, function parameters, and return values. By providing static types, developers can catch errors and bugs at compile-time and benefit from improved code readability and maintainability.
Let’s consider the following example to understand static typing in TypeScript:
// Declaring a function with static typing function addNumbers(a: number, b: number): number { return a + b; } // Calling the function with correct types const result = addNumbers(10, 20); // result will be inferred as number // Calling the function with incorrect types const errorResult = addNumbers(10, "20"); // Error: Argument of type 'string' is not assignable to parameter of type 'number'
In this example, we define a function addNumbers
that takes two parameters a
and b
, both of type number
, and returns a value of type number
. When we call this function with correct types, TypeScript infers the return type as number
. However, if we call the function with incorrect types, TypeScript throws an error at compile-time.
Static typing in TypeScript helps catch errors and bugs early in the development process and provides better tooling support, such as autocompletion and code navigation, by leveraging the type information.
Type Inference in TypeScript
Type inference is a useful feature of TypeScript that enables the compiler to automatically determine the types of variables, function parameters, and return values based on their usage. This eliminates the need for explicit type annotations in many cases and reduces the verbosity of the code.
Let’s look at an example to understand how type inference works in TypeScript:
// Type inference for primitive types const message = "Hello, TypeScript!"; // Type: string const count = 42; // Type: number const isValid = true; // Type: boolean // Type inference for arrays const numbers = [1, 2, 3]; // Type: number[] // Type inference for objects const person = { name: "John", age: 30 }; // Type: { name: string, age: number } // Type inference for function return values function addNumbers(a: number, b: number) { return a + b; // Type of return value: number }
In this example, we declare variables without explicitly specifying their types. TypeScript uses type inference to determine the types based on the assigned values. For example, the variable message
is inferred as a string
, count
as a number
, isValid
as a boolean
, numbers
as an array of number
, person
as an object with name
and age
properties, and the return value of the addNumbers
function as a number
.
Type inference helps reduce the amount of boilerplate code required in TypeScript and improves developer productivity. However, it’s important to note that type inference is not always perfect, and there may be cases where explicit type annotations are necessary to ensure correct typing.
Type Annotations in TypeScript
Type annotations are a way to explicitly specify the types of variables, function parameters, and return values in TypeScript. They provide additional clarity and help catch errors and bugs at compile-time.
Let’s consider the following example to understand type annotations in TypeScript:
// Type annotation for variable let message: string = "Hello, TypeScript!"; // Type annotation for function parameter and return value function greet(name: string): string { return `Hello, ${name}!`; } // Type annotation for object properties const person: { name: string; age: number } = { name: "John", age: 30 };
In this example, we use type annotations to explicitly specify the types of variables, function parameters, and object properties. The variable message
is annotated as a string
, the name
parameter of the greet
function is annotated as a string
, and the return value of the greet
function is annotated as a string
. Additionally, the person
object is annotated with the types of its properties, name
as a string
and age
as a number
.
Type annotations provide explicit typing information, which can improve code understanding, especially in cases where type inference may not be sufficient or clear. They also enable the TypeScript compiler to perform type checking and catch errors at compile-time.
Related Article: Tutorial: Navigating the TypeScript Exit Process
Type System in TypeScript
The type system in TypeScript is a set of rules and features that govern how types are defined, used, and checked in the language. It provides a way to enforce type safety and catch errors at compile-time, improving the reliability and maintainability of the codebase.
TypeScript’s type system includes various concepts and features, such as:
– Primitive types: TypeScript supports the same primitive types as JavaScript, including number
, string
, boolean
, null
, undefined
, and symbol
.
– Object types: TypeScript allows defining object types using interfaces or type aliases. Object types can have properties with specific types and optional or readonly modifiers.
– Function types: TypeScript supports defining function types, including the parameter types and return type. This enables type checking for function calls and function implementations.
– Union and intersection types: TypeScript allows combining multiple types using union or intersection operators. Union types represent values that can be of one of several types, while intersection types represent values that have properties from multiple types.
– Generics: TypeScript supports generics, which allow creating reusable components that can work with different types. Generics provide type parameters that can be used to define the types of function parameters, return values, and data structures.
– Type inference: TypeScript’s type system includes useful type inference capabilities that automatically determine the types of variables, function parameters, and return values based on their usage. This reduces the need for explicit type annotations in many cases.
The type system in TypeScript helps catch errors and bugs at compile-time, provides better tooling support, and improves code readability and maintainability. It allows developers to write more reliable and robust code by enforcing type safety throughout the development process.
Type Compatibility in TypeScript
TypeScript has a useful type compatibility system that determines whether one type can be assigned to another. This feature allows for more flexible and reusable code, especially when working with libraries or codebases that may have different typing conventions.
Let’s consider the following example to understand type compatibility in TypeScript:
interface Animal { name: string; } interface Dog { name: string; breed: string; } function greetAnimal(animal: Animal) { console.log(`Hello, ${animal.name}!`); } const dog: Dog = { name: "Buddy", breed: "Labrador" }; greetAnimal(dog); // Valid: Dog is compatible with Animal
In this example, we define two interfaces, Animal
and Dog
. The Dog
interface extends the Animal
interface and adds a breed
property. We also define a function greetAnimal
that takes an Animal
parameter and logs a greeting message.
Even though the greetAnimal
function expects an Animal
parameter, we can pass a Dog
object to it, as Dog
is compatible with Animal
. This is because Dog
has all the properties required by Animal
and satisfies the type contract. TypeScript’s type compatibility system allows for this kind of flexibility and ensures that compatible types can be used interchangeably.
Type compatibility in TypeScript is based on structural typing rather than nominal typing. This means that types are compared based on their structure and shape, rather than their names or declarations. This allows for more robust and flexible code, as types can be reused and composed more easily.
Type Assertions in TypeScript
Type assertions, also known as type casts, are a way to tell the TypeScript compiler that you know more about a value’s type than it does. They allow you to override the static type checking and treat a value as a different type.
Type assertions are useful in situations where the type of a value cannot be determined by the TypeScript compiler through type inference or explicit type annotations. They provide a mechanism to explicitly tell the compiler about the intended type of a value.
Let’s consider the following example to understand type assertions in TypeScript:
// Type assertion to treat a value as a specific type let value: any = "Hello, TypeScript!"; let length: number = (value as string).length; // Type assertion with angle bracket syntax let anotherValue: any = "Hello, TypeScript!"; let anotherLength: number = (anotherValue).length;
In this example, we have a variable value
of type any
, which means it can hold values of any type. We use a type assertion (value as string)
to treat the value as a string
and access its length
property. Similarly, we use another type assertion anotherValue
to achieve the same result.
Type assertions are a way to tell the TypeScript compiler that you are confident about the type of a value, even if it cannot be verified through the usual type checking mechanisms. However, it’s important to be cautious when using type assertions, as they bypass the type checking and may lead to runtime errors if the types are not compatible.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Type Declaration in TypeScript
Type declaration in TypeScript refers to the process of defining the shape and structure of custom types, including interfaces, type aliases, and classes. Type declarations allow developers to create reusable type definitions and enforce type safety throughout their codebase.
Let’s look at some examples of type declarations in TypeScript:
// Interface declaration interface Person { name: string; age: number; } // Type alias declaration type Point = { x: number; y: number; }; // Class declaration class Rectangle { width: number; height: number; constructor(width: number, height: number) { this.width = width; this.height = height; } getArea(): number { return this.width * this.height; } }
In this example, we define an interface Person
that represents the shape of a person object, with name
and age
properties. We also define a type alias Point
that represents a point in a two-dimensional coordinate system, with x
and y
properties. Additionally, we define a class Rectangle
that represents a rectangle object, with width
, height
, and getArea
method.
Type declarations provide a way to define the structure and behavior of custom types, making the code more expressive and self-documenting. They enable the TypeScript compiler to perform type checking and ensure type safety throughout the development process.
Type Alias in TypeScript
Type aliases in TypeScript allow developers to create custom names for existing types, including primitive types, object types, and union or intersection types. They provide a way to create reusable type definitions and improve code readability and maintainability.
Let’s consider the following example to understand type aliases in TypeScript:
// Type alias for a function type type MathOperation = (a: number, b: number) => number; // Type alias for an object type type Person = { name: string; age: number; }; // Type alias for a union type type Result = number | string; // Type alias for an intersection type type Coordinate = { x: number } & { y: number };
In this example, we define a type alias MathOperation
for a function type that takes two parameters of type number
and returns a value of type number
. We also define a type alias Person
for an object type with name
and age
properties. Additionally, we define type aliases Result
and Coordinate
for a union type and an intersection type, respectively.
Type aliases provide a way to create descriptive and expressive names for types, making the code more self-documenting and easier to understand. They also enable the reuse of type definitions, reducing duplication and improving code maintainability.
Type Narrowing in TypeScript
Type narrowing in TypeScript refers to the process of narrowing down the type of a value within a conditional statement based on certain conditions. It allows developers to write more precise and type-safe code by leveraging the control flow analysis performed by the TypeScript compiler.
Let’s look at an example to understand type narrowing in TypeScript:
function printValue(value: string | number) { if (typeof value === "string") { console.log(value.toUpperCase()); // Valid: value is narrowed down to string } else if (typeof value === "number") { console.log(value.toFixed(2)); // Valid: value is narrowed down to number } else { console.log("Invalid value!"); // Valid: value is narrowed down to never } }
In this example, we define a function printValue
that takes a parameter value
of type string
or number
. Within the function, we use conditional statements to narrow down the type of value
based on its typeof. If value
is a string
, we can safely call string-specific methods like toUpperCase()
. If value
is a number
, we can call number-specific methods like toFixed()
. If value
is neither a string
nor a number
, it is narrowed down to the never
type.
Type narrowing in TypeScript allows developers to write code that is more type-safe and avoids potential runtime errors. It enables the TypeScript compiler to perform static type checking and catch type-related issues at compile-time.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
Type Checking in TypeScript – Explained
TypeScript’s type checking is a process that the TypeScript compiler performs to ensure that variables, functions, and objects are used correctly based on their declared types. It helps catch errors and bugs at compile-time rather than runtime, improving code reliability and maintainability.
When the TypeScript compiler encounters a variable declaration, function call, or object usage, it checks if the operations and assignments are compatible with the declared types. It performs various checks, such as:
– Type inference: TypeScript uses type inference to automatically determine the types of variables, function parameters, and return values based on their usage. It leverages the context and available type information to infer the most appropriate types.
– Type annotations: Developers can explicitly specify the types of variables, function parameters, and return values using type annotations. These annotations provide additional clarity and help catch errors at compile-time.
– Type compatibility: TypeScript’s type system includes rules for determining whether one type can be assigned to another. It allows for more flexible and reusable code, especially when working with libraries or codebases that may have different typing conventions.
– Type narrowing: Type narrowing is the process of narrowing down the type of a value within a conditional statement based on certain conditions. It allows for more precise and type-safe code by leveraging the control flow analysis performed by the TypeScript compiler.
TypeScript’s type checking is a useful feature that brings the benefits of static typing to JavaScript, enabling developers to write more reliable and robust code.
Static Typing in TypeScript – Explained
Static typing is a fundamental feature of TypeScript that allows developers to specify types explicitly for variables, function parameters, and return values. It provides compile-time type checking, catching errors and bugs before the code is executed, and improving code reliability and maintainability.
In static typing, types are checked during the compilation process rather than at runtime. The TypeScript compiler analyzes the code, examines the type annotations and inferred types, and verifies if the operations and assignments are compatible with the declared types.
– Early error detection: Static typing helps catch errors and bugs at compile-time, before the code is executed. This allows developers to address issues early in the development process and reduces the number of runtime errors.
– Improved code readability: Explicitly specifying types using type annotations enhances code readability and self-documentation. It makes the code more understandable, especially for developers who are new to the codebase.
– Enhanced tooling support: Static typing enables better tooling support, such as autocompletion, code navigation, and refactoring. IDEs and text editors can leverage the type information to provide more accurate suggestions and help developers write code more efficiently.
– Code maintainability: Static typing helps enforce type safety throughout the codebase, making it easier to maintain and refactor the code. It ensures that changes to one part of the code don’t introduce type-related issues in other parts.
However, it’s important to note that static typing in TypeScript is optional. Developers can choose to use dynamic typing, where types are not explicitly specified, by using the any
type or relying solely on type inference.
Static typing in TypeScript strikes a balance between the flexibility and expressiveness of JavaScript and the reliability and maintainability of static typing. It enables developers to write more reliable and robust code while still enjoying the benefits of a dynamic scripting language.
How Type Inference Works in TypeScript
Type inference is a useful feature of TypeScript that allows the compiler to automatically determine the types of variables, function parameters, and return values based on their usage. It eliminates the need for explicit type annotations in many cases and reduces the verbosity of the code.
Type inference in TypeScript works by analyzing the context and available type information to infer the most appropriate types. It considers various factors, such as the assigned values, function arguments, and return statements, to determine the types.
Let’s look at some examples to understand how type inference works in TypeScript:
// Type inference for primitive types const message = "Hello, TypeScript!"; // Type: string const count = 42; // Type: number const isValid = true; // Type: boolean // Type inference for arrays const numbers = [1, 2, 3]; // Type: number[] // Type inference for objects const person = { name: "John", age: 30 }; // Type: { name: string, age: number } // Type inference for function return values function addNumbers(a: number, b: number) { return a + b; // Type of return value: number }
In these examples, we declare variables without explicitly specifying their types. TypeScript uses type inference to determine the types based on the assigned values and usage.
For example, in the first example, the variable message
is inferred as a string
because it is assigned a string value. Similarly, the variables count
and isValid
are inferred as number
and boolean
, respectively, based on their assigned values.
In the second example, the variable numbers
is inferred as an array of number
because it is assigned an array containing only numbers.
In the third example, the variable person
is inferred as an object with name
and age
properties. TypeScript infers the types of the properties based on the assigned values.
In the fourth example, the return value of the addNumbers
function is inferred as a number
because the function body performs addition on two numbers.
Type inference in TypeScript is a useful mechanism that reduces the need for explicit type annotations and improves developer productivity. However, it’s important to note that type inference is not always perfect, and there may be cases where explicit type annotations are necessary to ensure correct typing.
Related Article: Tutorial on Exact Type in TypeScript
Understanding Type Annotations in TypeScript
Type annotations in TypeScript allow developers to explicitly specify the types of variables, function parameters, and return values. They provide additional clarity and help catch errors and bugs at compile-time.
Type annotations in TypeScript follow a syntax where the variable or parameter name is followed by a colon :
and the desired type. Here are some examples of type annotations in TypeScript:
// Type annotation for variable let message: string = "Hello, TypeScript!"; // Type annotation for function parameter and return value function greet(name: string): string { return `Hello, ${name}!`; } // Type annotation for object properties const person: { name: string; age: number } = { name: "John", age: 30 };
In the first example, we declare a variable message
and annotate it with the type string
. This specifies that the variable can only hold string values.
In the second example, we define a function greet
with a parameter name
annotated as string
. This indicates that the function expects a string value as the argument. The return value of the function is also annotated as string
, specifying that the function will return a string value.
In the third example, we declare a constant person
and annotate it with an object type. The object type specifies the types of the name
and age
properties, both of which are annotated as string
and number
, respectively.
Type annotations provide explicit typing information, which can improve code understanding and catch errors at compile-time. They also enable the TypeScript compiler to perform type checking and ensure type safety throughout the development process.
What is a Type System in TypeScript?
A type system in TypeScript is a set of rules and features that govern how types are defined, used, and checked in the language. It provides a way to enforce type safety and catch errors at compile-time, improving the reliability and maintainability of the codebase.
TypeScript’s type system includes various concepts and features, including:
– Primitive types: TypeScript supports the same primitive types as JavaScript, including number
, string
, boolean
, null
, undefined
, and symbol
. These types represent basic values in the language.
– Object types: TypeScript allows defining object types using interfaces or type aliases. Object types can have properties with specific types and optional or readonly modifiers. They represent structured data in the language.
– Function types: TypeScript supports defining function types, including the parameter types and return type. This enables type checking for function calls and function implementations. Function types can be used to specify the types of variables or function parameters.
– Union and intersection types: TypeScript allows combining multiple types using union or intersection operators. Union types represent values that can be of one of several types, while intersection types represent values that have properties from multiple types. Union and intersection types provide flexibility and composability in type definitions.
– Generics: TypeScript supports generics, which allow creating reusable components that can work with different types. Generics provide type parameters that can be used to define the types of function parameters, return values, and data structures. Generics enable type-safe abstraction and code reuse.
– Type inference: TypeScript’s type system includes useful type inference capabilities that automatically determine the types of variables, function parameters, and return values based on their usage. Type inference reduces the need for explicit type annotations and improves developer productivity.
The type system in TypeScript helps catch errors and bugs at compile-time, provides better tooling support, and improves code readability and maintainability. It allows developers to write more reliable and robust code by enforcing type safety throughout the development process.
Type Compatibility in TypeScript – Explained
Type compatibility in TypeScript refers to the rules and mechanisms that determine whether one type can be assigned to another. It allows for more flexible and reusable code, especially when working with libraries or codebases that may have different typing conventions.
TypeScript’s type compatibility is based on structural typing rather than nominal typing. This means that types are compared based on their structure and shape, rather than their names or declarations. If two types have compatible structures, they are considered compatible, even if they have different names or were defined separately.
Let’s consider the following example to understand type compatibility in TypeScript:
interface Animal { name: string; } interface Dog { name: string; breed: string; } function greetAnimal(animal: Animal) { console.log(`Hello, ${animal.name}!`); } const dog: Dog = { name: "Buddy", breed: "Labrador" }; greetAnimal(dog); // Valid: Dog is compatible with Animal
In this example, we define two interfaces, Animal
and Dog
. The Dog
interface extends the Animal
interface and adds a breed
property. We also define a function greetAnimal
that takes an Animal
parameter and logs a greeting message.
Even though the greetAnimal
function expects an Animal
parameter, we can pass a Dog
object to it, as Dog
is compatible with Animal
. This is because Dog
has all the properties required by Animal
and satisfies the type contract. TypeScript’s type compatibility system allows for this kind of flexibility and ensures that compatible types can be used interchangeably.
Type compatibility in TypeScript enables code reuse and interoperability by allowing types with compatible structures to be used interchangeably. It promotes flexibility and extensibility while maintaining type safety throughout the codebase.
Related Article: How to Convert Strings to Booleans in TypeScript
What are Type Assertions in TypeScript?
Type assertions, also known as type casts, are a way to tell the TypeScript compiler that you know more about a value’s type than it does. They allow you to override the static type checking and treat a value as a different type.
Type assertions are useful in situations where the type of a value cannot be determined by the TypeScript compiler through type inference or explicit type annotations. They provide a mechanism to explicitly tell the compiler about the intended type of a value.
Let’s consider the following example to understand type assertions in TypeScript:
// Type assertion to treat a value as a specific type let value: any = "Hello, TypeScript!"; let length: number = (value as string).length; // Type assertion with angle bracket syntax let anotherValue: any = "Hello, TypeScript!"; let anotherLength: number = (anotherValue).length;
In this example, we have a variable value
of type any
, which means it can hold values of any type. We use a type assertion (value as string)
to treat the value as a string
and access its length
property. Similarly, we use another type assertion anotherValue
to achieve the same result.
Type assertions are a way to tell the TypeScript compiler that you are confident about the type of a value, even if it cannot be verified through the usual type checking mechanisms. They are particularly useful when working with values that have a more general or dynamic type, such as any
.
However, it’s important to be cautious when using type assertions, as they bypass the type checking and may lead to runtime errors if the types are not compatible. It’s recommended to use type assertions sparingly and only when necessary.
Declaring Types in TypeScript
In TypeScript, types can be declared using various mechanisms, such as interfaces, type aliases, classes, and enums. These mechanisms provide a way to define the shape and behavior of custom types, enabling type checking and ensuring type safety throughout the codebase.
Let’s look at some examples of declaring types in TypeScript:
// Interface declaration interface Person { name: string; age: number; } // Type alias declaration type Point = { x: number; y: number; }; // Class declaration class Rectangle { width: number; height: number; constructor(width: number, height: number) { this.width = width; this.height = height; } getArea(): number { return this.width * this.height; } } // Enum declaration enum Color { Red = "red", Green = "green", Blue = "blue", }
In this example, we declare an interface Person
that represents the shape of a person object, with name
and age
properties. We also define a type alias Point
that represents a point in a two-dimensional coordinate system, with x
and y
properties. Additionally, we declare a class Rectangle
that represents a rectangle object, with width
, height
, and a method getArea
. Finally, we declare an enum Color
that represents a set of colors.
These type declarations allow developers to define the structure and behavior of custom types, making the code more expressive and self-documenting. They enable the TypeScript compiler to perform type checking and ensure type safety throughout the development process.
Type Alias in TypeScript – Explained
Type aliases in TypeScript allow developers to create custom names for existing types, including primitive types, object types, and union or intersection types. They provide a way to create reusable type definitions and improve code readability and maintainability.
To create a type alias in TypeScript, we use the type
keyword followed by the desired alias name and the type definition. Here are some examples of type aliases in TypeScript:
// Type alias for a function type type MathOperation = (a: number, b: number) => number; // Type alias for an object type type Person = { name: string; age: number; }; // Type alias for a union type type Result = number | string; // Type alias for an intersection type type Coordinate = { x: number } & { y: number };
In this example, we define a type alias MathOperation
for a function type that takes two parameters of type number
and returns a value of type number
. We also define a type alias Person
for an object type with name
and age
properties. Additionally, we define type aliases Result
and Coordinate
for a union type and an intersection type, respectively.
Type aliases provide a way to create descriptive and expressive names for types, making the code more self-documenting and easier to understand. They also enable the reuse of type definitions, reducing duplication and improving code maintainability.
Type aliases are particularly useful in scenarios where multiple types need to be combined or when defining complex types that are used in multiple places. They provide a convenient way to create aliases for commonly used types and improve the readability of the code.