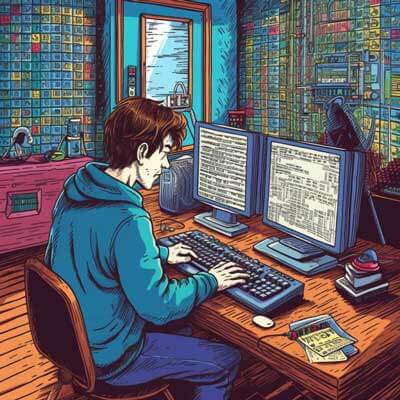
- What is ESLint?
- What is a config file in ESLint?
- What is the purpose of eslint-config-standard-with-typescript?
- How does eslint-config-standard-with-typescript differ from eslint-config-standard?
- How to install eslint-config-standard-with-typescript?
- How to configure eslint-config-standard-with-typescript?
- What are some common ESLint rules for TypeScript?
- How does ESLint improve code quality?
- What are the benefits of using eslint-config-standard-with-typescript in a project?
- Can eslint-config-standard-with-typescript be used with other ESLint configurations?
- External Sources
What is ESLint?
ESLint is a popular open-source JavaScript linting tool that helps developers maintain code quality and enforce coding conventions. It identifies and reports code patterns that may lead to bugs, security vulnerabilities, or poor code readability. ESLint supports a wide range of customizable rules and can be integrated into various development environments, including IDEs and build systems.
Related Article: How to Get an Object Value by Dynamic Keys in TypeScript
What is a config file in ESLint?
In ESLint, a config file is a JavaScript or JSON file that specifies the configuration options for the ESLint tool. It allows developers to define which rules should be enabled or disabled and how they should be applied to their codebase. The config file provides a way to establish a consistent coding style across a project or an organization.
Here’s an example of a .eslintrc.js config file:
module.exports = { root: true, env: { node: true, es6: true, }, extends: [ 'eslint:recommended', 'plugin:@typescript-eslint/recommended', ], parser: '@typescript-eslint/parser', parserOptions: { ecmaVersion: 2020, sourceType: 'module', }, plugins: [ '@typescript-eslint', ], rules: { // Custom rules can be defined here }, };
This config file enables ESLint’s recommended rules and extends the configuration provided by the @typescript-eslint/recommended
plugin. It also specifies that TypeScript code should be parsed using the @typescript-eslint/parser
parser.
What is the purpose of eslint-config-standard-with-typescript?
The purpose of eslint-config-standard-with-typescript
is to provide a pre-configured ESLint configuration specifically tailored for projects that use TypeScript. It combines the rules from the eslint-config-standard
configuration (which enforces the JavaScript Standard Style) with additional rules and configurations for TypeScript.
How does eslint-config-standard-with-typescript differ from eslint-config-standard?
While both eslint-config-standard
and eslint-config-standard-with-typescript
provide ESLint configurations based on the JavaScript Standard Style, the latter includes additional rules and configurations specific to TypeScript. The main differences between the two configurations are:
1. TypeScript Support: eslint-config-standard-with-typescript
includes rules and configurations that are optimized for TypeScript code, such as type checking rules and rules for handling TypeScript-specific syntax.
2. Parser: eslint-config-standard
uses the default ESLint parser for JavaScript, while eslint-config-standard-with-typescript
uses the @typescript-eslint/parser
parser specifically designed for TypeScript.
3. Plugin: eslint-config-standard-with-typescript
includes the @typescript-eslint
plugin, which provides additional rules and utilities for TypeScript.
Related Article: How to Work with Anonymous Classes in TypeScript
How to install eslint-config-standard-with-typescript?
To install eslint-config-standard-with-typescript
, you need to follow these steps:
1. Make sure you have Node.js and npm (Node Package Manager) installed on your system.
2. Create a new project or navigate to the root directory of your existing project.
3. Open your terminal or command prompt and run the following command:
npm install eslint eslint-config-standard eslint-plugin-standard eslint-plugin-promise @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-config-standard-with-typescript --save-dev
4. This command installs ESLint, the required plugins, and the eslint-config-standard-with-typescript
package as development dependencies in your project.
5. Once the installation is complete, you can proceed with configuring ESLint to use the eslint-config-standard-with-typescript
configuration.
How to configure eslint-config-standard-with-typescript?
To configure ESLint to use the eslint-config-standard-with-typescript
configuration, follow these steps:
1. Create an ESLint config file (e.g., .eslintrc.js
) in the root directory of your project if you haven’t already.
2. Open the config file in a text editor and add the following content:
module.exports = { extends: 'standard-with-typescript', parserOptions: { project: './tsconfig.json', }, };
3. The extends
option specifies that you want to use the standard-with-typescript
configuration.
4. The parserOptions
option specifies the path to your TypeScript configuration file (tsconfig.json
). This allows ESLint to analyze your TypeScript code correctly.
5. Save the config file.
Now, when you run ESLint, it will use the eslint-config-standard-with-typescript
configuration and apply the rules and settings specific to TypeScript.
What are some common ESLint rules for TypeScript?
ESLint provides a wide range of rules for TypeScript to enforce coding standards and catch potential issues. Here are some common ESLint rules for TypeScript:
1. @typescript-eslint/explicit-function-return-type
: Requires function return types to be explicitly specified.
2. @typescript-eslint/no-unused-vars
: Flags unused variables and parameters.
3. @typescript-eslint/no-explicit-any
: Disallows the use of the any
type, encouraging type safety.
4. @typescript-eslint/no-non-null-assertion
: Disallows the use of non-null assertions, which can lead to runtime errors.
5. @typescript-eslint/semi
: Requires semicolons at the end of statements.
These rules help ensure type safety, reduce potential bugs, and maintain code consistency in TypeScript projects.
Related Article: Building a Rules Engine with TypeScript
How does ESLint improve code quality?
ESLint improves code quality in several ways:
1. Consistent Coding Style: ESLint enforces coding conventions and style guidelines, ensuring that the codebase follows a consistent and readable style. This improves code readability and maintainability.
2. Early Bug Detection: ESLint catches common programming errors and potential bugs, such as unused variables, undefined variables, missing return statements, and more. This helps identify and fix issues before they manifest as runtime errors.
3. Code Consistency: ESLint ensures that the codebase adheres to a set of predefined rules, reducing inconsistencies and making it easier for multiple developers to work on the same codebase.
4. Maintainability: By enforcing best practices and coding standards, ESLint improves the overall quality and maintainability of the codebase. It helps identify potential code smells and anti-patterns, making it easier to refactor and improve the codebase over time.
5. Code Review Assistance: ESLint provides automatic code analysis, highlighting potential issues during code reviews. This speeds up the review process and helps ensure that code changes meet the required quality standards.
What are the benefits of using eslint-config-standard-with-typescript in a project?
Using eslint-config-standard-with-typescript
in a project offers several benefits:
1. TypeScript-Specific Rules: eslint-config-standard-with-typescript
includes rules optimized for TypeScript code. It helps catch common mistakes, enforce best practices, and ensure type safety in TypeScript projects.
2. JavaScript Standard Style: eslint-config-standard-with-typescript
is based on the JavaScript Standard Style, which is a widely adopted coding style guide. It promotes clean and consistent code across the project.
3. Easy Integration: eslint-config-standard-with-typescript
can be easily integrated into existing projects. By extending the configuration and adding the required dependencies, developers can quickly set up their ESLint environment for TypeScript.
4. Customizability: The ESLint configuration provided by eslint-config-standard-with-typescript
is highly customizable. Developers can add or override rules to suit their specific project requirements.
5. Improved Code Quality: By enforcing coding conventions, catching potential bugs, and promoting best practices, eslint-config-standard-with-typescript
helps improve the overall code quality of the project. This leads to more maintainable and reliable code.
Can eslint-config-standard-with-typescript be used with other ESLint configurations?
Yes, eslint-config-standard-with-typescript
can be used with other ESLint configurations. It is designed to be extendable, allowing developers to combine it with other ESLint configurations and customize it based on their project’s needs.
To use eslint-config-standard-with-typescript
with other configurations, you can extend multiple configurations in your ESLint config file. Here’s an example:
module.exports = { extends: [ 'standard-with-typescript', 'eslint-config-other', ], parserOptions: { project: './tsconfig.json', }, // Other configuration options };
In this example, the extends
option includes both the standard-with-typescript
configuration and another custom configuration named 'eslint-config-other'
. This allows you to combine the rules and settings from both configurations in your project.
Related Article: How to Implement ETL Processes with TypeScript
External Sources
– ESLint Documentation
– ESLint Configuring Rules
– TypeScript ESLint