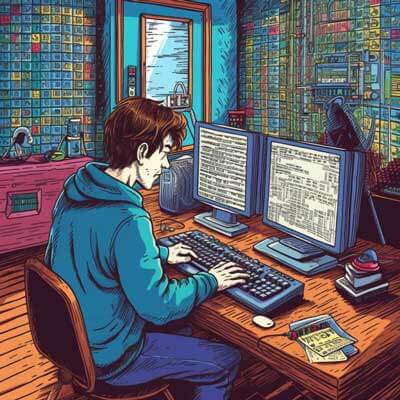
The Record type in TypeScript is a useful feature that allows you to define an object with dynamic keys and specific value types. It is particularly useful when you want to create dictionaries or maps with unknown or dynamic keys. In this guide, we will explore how to use the Record type effectively in TypeScript.
1. Basic Usage
To define a Record type in TypeScript, you can use the following syntax:
type MyRecord = Record<string, number>;
In this example, the MyRecord
type is defined as a dictionary with string keys and number values. You can replace string
and number
with any other TypeScript types to suit your needs.
Once you have defined the Record type, you can use it to create objects with dynamic keys:
const myObject: MyRecord = { key1: 10, key2: 20, };
In this case, myObject
is an object with keys key1
and key2
, both mapped to number values.
Related Article: How to Implement and Use Generics in Typescript
2. Readonly Record
You can also create a readonly version of a Record type by using the Readonly
utility type:
type MyReadonlyRecord = Readonly<Record<string, number>>;
With this definition, the properties of the object created from MyReadonlyRecord
cannot be modified:
const myReadonlyObject: MyReadonlyRecord = { key1: 10, key2: 20, }; myReadonlyObject.key1 = 30; // Error: Cannot assign to 'key1' because it is a read-only property.
3. Advanced Usage
The Record type can be used with more complex value types, such as interfaces or other custom types. For example, you can define a Record type with an interface as the value type:
interface Person { name: string; age: number; } type PersonRecord = Record<string, Person>;
In this case, the PersonRecord
type represents an object with dynamic keys, where each key maps to a Person
object.
You can also use the Record type in combination with other TypeScript features, such as mapped types. For instance, you can create a mapped type that makes all the properties of a given type optional:
type Optional = { [K in keyof T]?: T[K]; }; type OptionalPersonRecord = Record<string, Optional>;
In this example, the OptionalPersonRecord
type allows each property of the Person
object to be optional.
4. Best Practices
When using the Record type in TypeScript, consider the following best practices:
– Use descriptive key names: Since the keys in a Record type are dynamic, it is important to use descriptive names to make the code more readable and maintainable.
– Limit the use of dynamic keys: While the Record type provides flexibility with dynamic keys, it is generally recommended to limit their use to cases where the keys are truly unknown or dynamic. In most cases, using a more structured data structure, such as an array or a Map, may be more appropriate.
– Use mapped types and utility types: Take advantage of mapped types and utility types, such as Readonly, to further refine the behavior of Record types and make them more expressive.
– Consider immutability: Depending on your use case, you may want to consider making the properties of the Record object readonly or using immutability libraries like Immutable.js to ensure data integrity.
Related Article: How to Check If a String is in an Enum in TypeScript