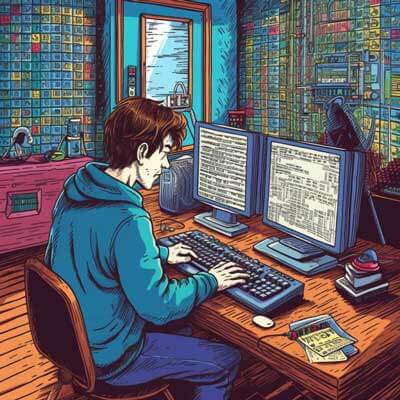
- Syntax to Omit a Property in TypeScript
- Removing a Specific Property from an Object in TypeScript
- Excluding a Key from an Interface in TypeScript
- Syntax to Exclude a Field from a Type in TypeScript
- Excluding an Attribute from a Class in TypeScript
- Excluding a Member from a Type in TypeScript
- Syntax to Exclude a Property from an Interface in TypeScript
- Excluding a Property from a Type Definition in TypeScript
- Excluding a Property from an Object Literal in TypeScript
- External Sources
Syntax to Omit a Property in TypeScript
In TypeScript, the Omit
utility type allows you to exclude a specific property from an object type. The syntax for using Omit
is as follows:
type NewType = Omit<OldType, 'propertyToExclude'>;
Here, OldType
represents the original type with the property you want to exclude, and 'propertyToExclude'
is the name of the property you want to omit. The resulting type NewType
will be the same as OldType
, but without the specified property.
Let’s consider an example where we have an object type Person
with properties name
, age
, and address
, and we want to exclude the address
property:
type Person = { name: string; age: number; address: string; }; type PersonWithoutAddress = Omit<Person, 'address'>; const person: PersonWithoutAddress = { name: 'John Doe', age: 30, };
In the above example, the PersonWithoutAddress
type is created using Omit<Person, 'address'>
, which excludes the address
property from the Person
type. The resulting type is then used to define the person
object, which only has the name
and age
properties.
Related Article: How to Implement and Use Generics in Typescript
Removing a Specific Property from an Object in TypeScript
In TypeScript, you can also remove a specific property from an object directly using the delete
keyword. The syntax for removing a property is as follows:
delete object.property;
Let’s consider an example where we have an object person
with properties name
, age
, and address
, and we want to remove the address
property:
const person = { name: 'John Doe', age: 30, address: '123 Main St', }; delete person.address; console.log(person);
The output of the above code will be:
{ name: 'John Doe', age: 30 }
In the above example, we use delete person.address
to remove the address
property from the person
object. The resulting object only has the name
and age
properties.
Excluding a Key from an Interface in TypeScript
In TypeScript, you can exclude a specific key from an interface by creating a new interface that extends the original interface and omits the desired key. The syntax for excluding a key from an interface is as follows:
interface NewInterface extends Omit<OldInterface, 'keyToExclude'> {}
Here, OldInterface
represents the original interface with the key you want to exclude, and 'keyToExclude'
is the name of the key you want to omit. The resulting NewInterface
will be the same as OldInterface
, but without the specified key.
Let’s consider an example where we have an interface Person
with keys name
, age
, and address
, and we want to exclude the address
key:
interface Person { name: string; age: number; address: string; } interface PersonWithoutAddress extends Omit<Person, 'address'> {} const person: PersonWithoutAddress = { name: 'John Doe', age: 30, };
In the above example, the PersonWithoutAddress
interface is created by extending Omit<Person, 'address'>
, which excludes the address
key from the Person
interface. The resulting interface can then be used to define objects that don’t have the address
key.
Syntax to Exclude a Field from a Type in TypeScript
In TypeScript, you can exclude a specific field from a type using the Exclude
utility type. The syntax for using Exclude
is as follows:
type NewType = Exclude<FieldType, 'fieldToExclude'>;
Here, FieldType
represents the original type with the field you want to exclude, and 'fieldToExclude'
is the name of the field you want to omit. The resulting type NewType
will be the same as FieldType
, but without the specified field.
Let’s consider an example where we have a type Person
with fields name
, age
, and address
, and we want to exclude the address
field:
type Person = { name: string; age: number; address: string; }; type PersonWithoutAddress = Exclude<Person, 'address'>; const person: PersonWithoutAddress = { name: 'John Doe', age: 30, };
In the above example, the PersonWithoutAddress
type is created using Exclude<Person, 'address'>
, which excludes the address
field from the Person
type. The resulting type is then used to define the person
object, which only has the name
and age
fields.
Related Article: How to Check If a String is in an Enum in TypeScript
Excluding an Attribute from a Class in TypeScript
In TypeScript, you can exclude an attribute from a class by extending a base class and omitting the desired attribute. The syntax for excluding an attribute from a class is as follows:
class NewClass extends BaseClass { constructor(...args: any[]) { super(...args); } }
Here, NewClass
represents the new class that extends the BaseClass
, and the constructor
method is used to call the constructor of the BaseClass
using the super
keyword.
Let’s consider an example where we have a base class Person
with attributes name
, age
, and address
, and we want to exclude the address
attribute:
class Person { name: string; age: number; address: string; constructor(name: string, age: number, address: string) { this.name = name; this.age = age; this.address = address; } } class PersonWithoutAddress extends Person { constructor(name: string, age: number) { super(name, age, ''); } } const person: PersonWithoutAddress = new PersonWithoutAddress('John Doe', 30);
In the above example, the PersonWithoutAddress
class is created by extending the Person
class and overriding the constructor to exclude the address
attribute. The resulting class can then be used to create objects without the address
attribute.
Excluding a Member from a Type in TypeScript
In TypeScript, you can exclude a specific member from a type using the Exclude
utility type. The syntax for using Exclude
is as follows:
type NewType = Exclude<OldType, 'memberToExclude'>;
Here, OldType
represents the original type with the member you want to exclude, and 'memberToExclude'
is the name of the member you want to omit. The resulting type NewType
will be the same as OldType
, but without the specified member.
Let’s consider an example where we have a type Person
with members name
, age
, and address
, and we want to exclude the address
member:
type Person = { name: string; age: number; address: string; }; type PersonWithoutAddress = Exclude<Person, 'address'>; const person: PersonWithoutAddress = { name: 'John Doe', age: 30, };
In the above example, the PersonWithoutAddress
type is created using Exclude<Person, 'address'>
, which excludes the address
member from the Person
type. The resulting type is then used to define the person
object, which only has the name
and age
members.
Syntax to Exclude a Property from an Interface in TypeScript
In TypeScript, you can exclude a specific property from an interface using the Omit
utility type. The syntax for using Omit
is as follows:
type NewInterface = Omit<OldInterface, 'propertyToExclude'>;
Here, OldInterface
represents the original interface with the property you want to exclude, and 'propertyToExclude'
is the name of the property you want to omit. The resulting interface NewInterface
will be the same as OldInterface
, but without the specified property.
Let’s consider an example where we have an interface Person
with properties name
, age
, and address
, and we want to exclude the address
property:
interface Person { name: string; age: number; address: string; } type PersonWithoutAddress = Omit<Person, 'address'>; const person: PersonWithoutAddress = { name: 'John Doe', age: 30, };
In the above example, the PersonWithoutAddress
type is created using Omit<Person, 'address'>
, which excludes the address
property from the Person
interface. The resulting interface can then be used to define objects that don’t have the address
property.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Excluding a Property from a Type Definition in TypeScript
In TypeScript, you can exclude a specific property from a type definition using the Omit
utility type. The syntax for using Omit
is as follows:
type NewType = Omit<OldType, 'propertyToExclude'>;
Here, OldType
represents the original type with the property you want to exclude, and 'propertyToExclude'
is the name of the property you want to omit. The resulting type NewType
will be the same as OldType
, but without the specified property.
Let’s consider an example where we have a type definition Person
with properties name
, age
, and address
, and we want to exclude the address
property:
type Person = { name: string; age: number; address: string; }; type PersonWithoutAddress = Omit<Person, 'address'>; const person: PersonWithoutAddress = { name: 'John Doe', age: 30, };
In the above example, the PersonWithoutAddress
type is created using Omit<Person, 'address'>
, which excludes the address
property from the Person
type. The resulting type is then used to define the person
object, which only has the name
and age
properties.
Excluding a Property from an Object Literal in TypeScript
In TypeScript, you can exclude a specific property from an object literal by using the Omit
utility type in combination with the Pick
utility type. The Pick
type allows you to select specific properties from an object type, while the Omit
type allows you to exclude specific properties from an object type.
The syntax for excluding a property from an object literal is as follows:
const newObj: Omit<OldObj, 'propertyToExclude'> = { ...oldObj };
Here, OldObj
represents the original object with the property you want to exclude, and 'propertyToExclude'
is the name of the property you want to omit. The resulting newObj
will be the same as oldObj
, but without the specified property.
Let’s consider an example where we have an object literal person
with properties name
, age
, and address
, and we want to exclude the address
property:
const person = { name: 'John Doe', age: 30, address: '123 Main St', }; const personWithoutAddress: Omit = { ...person }; console.log(personWithoutAddress);
The output of the above code will be:
{ name: 'John Doe', age: 30 }
In the above example, we use Omit
to create the personWithoutAddress
object by excluding the address
property from the person
object literal. The resulting object only has the name
and age
properties.