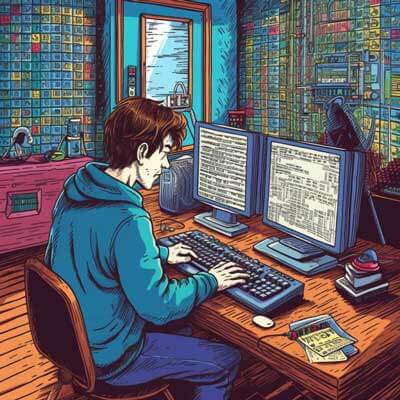
- The Importance of Anonymous Classes in Object-Oriented Programming
- Example 1: Creating an Anonymous Class
- Example 2: Using Anonymous Classes as Function Parameters
- Understanding TypeScript’s Type Annotations
- Example 1: Variable Type Annotation
- Example 2: Function Parameter and Return Type Annotations
- Exploring the Basics of Classes in TypeScript
- Example 1: Creating a Simple Class
- Example 2: Access Modifiers
- The Role of Inheritance in TypeScript
- Example 1: Creating a Base Class
- Example 2: Creating a Derived Class
- Creating and Using Anonymous Classes in TypeScript
- Example 1: Creating an Anonymous Class
- Example 2: Using Anonymous Classes as Function Parameters
- Benefits and Use Cases of Anonymous Classes
- Exploring Static Typing in TypeScript
- Example 1: Variable Type Annotation
- Example 2: Function Type Annotation
- Key Features and Differences Between TypeScript and JavaScript
- What is Object-Oriented Programming?
- How TypeScript Handles Type Annotations
- Advanced Techniques for Working with Anonymous Classes
- Example 1: Extending Functionality with Mixins
- Example 2: Implementing Decorators with Anonymous Classes
- Common Pitfalls and Best Practices for Anonymous Classes in TypeScript
- Pitfall 1: Overusing Anonymous Classes
- Pitfall 2: Lack of Reusability
- Pitfall 3: Difficulty in Debugging
- Best Practice 1: Use Named Classes for Reusable Code
- Best Practice 2: Limit the Use of Anonymous Classes
- Best Practice 3: Provide Meaningful Variable Names
- Using Anonymous Classes to Extend Functionality in TypeScript
- Example 1: Extending Functionality with Anonymous Classes
- Example 2: Overriding Methods with Anonymous Classes
- Code Snippet: Creating an Anonymous Class with Inheritance
- Code Snippet: Implementing Interfaces with Anonymous Classes
- Code Snippet: Using Anonymous Classes for Enhanced Modularity
- Code Snippet: Leveraging Anonymous Classes for Decorators
- Code Snippet: Applying Anonymous Classes in Real-World Scenarios
- External Sources
The Importance of Anonymous Classes in Object-Oriented Programming
Anonymous classes play a vital role in object-oriented programming (OOP) by allowing developers to define and instantiate a class in a single expression, without explicitly giving it a name. This concept is particularly useful when dealing with small, one-off classes that do not need to be reused or referenced elsewhere in the code.
Anonymous classes offer several benefits in OOP:
1. Code organization: By creating anonymous classes, developers can encapsulate related functionality within a single expression, making the code more concise and focused.
2. Improved readability: Anonymous classes can be used to define small, inline implementations of interfaces or abstract classes, making the code easier to read and understand by providing a clear context for the implementation.
3. Flexibility: Anonymous classes can be created on the fly, allowing for dynamic behavior and customization at runtime.
4. Encapsulation: Anonymous classes are typically used to implement interfaces or extend abstract classes, providing a level of abstraction and encapsulation that promotes modular and reusable code.
5. Reduced code duplication: By allowing developers to define and instantiate a class in a single expression, anonymous classes help reduce code duplication by eliminating the need to create separate classes for simple, one-off implementations.
Related Article: How to Get an Object Value by Dynamic Keys in TypeScript
Example 1: Creating an Anonymous Class
Here’s an example of how to create an anonymous class in TypeScript:
interface Greeting { greet(): void; } const greeter: Greeting = new class { greet() { console.log("Hello, world!"); } }(); greeter.greet(); // Output: Hello, world!
In this example, we define an anonymous class that implements the Greeting
interface. We then create an instance of the anonymous class and assign it to the greeter
variable. Finally, we call the greet
method on the greeter
instance to display the greeting.
Example 2: Using Anonymous Classes as Function Parameters
Anonymous classes can also be used as function parameters, allowing for dynamic behavior and customization. Here’s an example:
class Logger { log(message: string) { console.log(`[INFO] ${message}`); } } function processMessage(message: string, loggerClass: new () => Logger) { const logger = new loggerClass(); logger.log(message); } processMessage("Hello, world!", class { log(message: string) { console.log(`[CUSTOM] ${message}`); } });
In this example, we define a Logger
class with a log
method that prefixes the message with [INFO]
. The processMessage
function takes a message
parameter and a loggerClass
parameter, which represents an anonymous class that implements the Logger
interface. Inside the processMessage
function, we create an instance of the loggerClass
and call its log
method to display the message.
Understanding TypeScript’s Type Annotations
TypeScript is a statically typed superset of JavaScript that provides optional static typing, allowing developers to specify the types of variables, function parameters, and return values. Type annotations in TypeScript help catch errors early in the development process and improve code readability and maintainability.
Type annotations are used to define the types of variables, function parameters, and return values. They provide information to the TypeScript compiler about the expected types, enabling it to perform type checking and provide helpful error messages.
TypeScript supports several basic types, including number, string, boolean, null, undefined, and object, as well as more complex types such as arrays, tuples, enums, and interfaces. Additionally, TypeScript allows developers to create custom types using type aliases and union types.
Related Article: Using ESLint & eslint-config-standard-with-typescript
Example 1: Variable Type Annotation
Here’s an example of how to use type annotations for variables in TypeScript:
let count: number = 0; let message: string = "Hello, TypeScript"; let isActive: boolean = true; let data: any = { name: "John", age: 25 }; console.log(count, message, isActive, data);
In this example, we use type annotations to specify the types of the variables count
, message
, isActive
, and data
. The TypeScript compiler will check that the assigned values match the specified types and raise an error if there is a mismatch.
Example 2: Function Parameter and Return Type Annotations
Type annotations can also be used for function parameters and return values. Here’s an example:
function addNumbers(a: number, b: number): number { return a + b; } console.log(addNumbers(5, 3)); // Output: 8
In this example, we define a function addNumbers
that takes two parameters a
and b
, both of type number
, and returns a value of type number
. The type annotations for the function parameters and return value help the TypeScript compiler enforce type safety and provide better error checking.
Type annotations are a useful feature of TypeScript that enable developers to write more robust and maintainable code. By specifying the types of variables, function parameters, and return values, developers can catch errors early and benefit from better tooling and code documentation.
Exploring the Basics of Classes in TypeScript
Classes are an essential part of object-oriented programming in TypeScript. They provide a way to define objects with properties and methods, encapsulating related data and behavior into reusable entities.
In TypeScript, classes are defined using the class
keyword, followed by the class name and a set of curly braces containing the class members. Class members can include properties, methods, constructors, and access modifiers.
Related Article: Building a Rules Engine with TypeScript
Example 1: Creating a Simple Class
Here’s an example of how to create a simple class in TypeScript:
class Person { name: string; age: number; constructor(name: string, age: number) { this.name = name; this.age = age; } sayHello() { console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`); } } const john = new Person("John", 25); john.sayHello(); // Output: Hello, my name is John and I'm 25 years old.
In this example, we define a Person
class with two properties: name
of type string
and age
of type number
. We also define a constructor that takes the name
and age
as parameters and assigns them to the corresponding properties. The sayHello
method logs a greeting message using the name
and age
properties.
We then create an instance of the Person
class using the new
keyword and call the sayHello
method on the john
instance.
Example 2: Access Modifiers
Access modifiers in TypeScript allow developers to control the visibility and accessibility of class members. TypeScript supports three access modifiers: public
, private
, and protected
.
Here’s an example that demonstrates the use of access modifiers:
class BankAccount { private balance: number; constructor(initialBalance: number) { this.balance = initialBalance; } deposit(amount: number) { this.balance += amount; } withdraw(amount: number) { if (amount <= this.balance) { this.balance -= amount; } else { console.log("Insufficient funds"); } } getBalance() { return this.balance; } } const account = new BankAccount(1000); account.deposit(500); account.withdraw(200); console.log(account.getBalance()); // Output: 1300
In this example, we define a BankAccount
class with a private balance
property. The deposit
, withdraw
, and getBalance
methods can access and modify the balance
property because they are part of the same class. However, external code cannot directly access the balance
property due to its private
access modifier.
The Role of Inheritance in TypeScript
Inheritance is a fundamental concept of object-oriented programming that allows classes to inherit properties and methods from other classes. In TypeScript, classes can inherit from a single base class using the extends
keyword, enabling code reuse and promoting a hierarchical structure in object-oriented designs.
Related Article: How to Implement ETL Processes with TypeScript
Example 1: Creating a Base Class
Here’s an example of how to create a base class in TypeScript:
class Vehicle { speed: number; constructor(speed: number) { this.speed = speed; } move() { console.log("Moving at a speed of", this.speed, "km/h"); } } const vehicle = new Vehicle(100); vehicle.move(); // Output: Moving at a speed of 100 km/h
In this example, we define a Vehicle
class with a speed
property and a move
method. The move
method logs a message indicating the current speed of the vehicle.
We then create an instance of the Vehicle
class using the new
keyword and call the move
method on the vehicle
instance.
Example 2: Creating a Derived Class
To create a derived class that inherits from a base class, we use the extends
keyword. The derived class can add additional properties and methods or override the ones inherited from the base class.
Here’s an example:
class Car extends Vehicle { brand: string; constructor(speed: number, brand: string) { super(speed); this.brand = brand; } displayBrand() { console.log("Brand:", this.brand); } } const car = new Car(120, "Toyota"); car.move(); // Output: Moving at a speed of 120 km/h car.displayBrand(); // Output: Brand: Toyota
In this example, we define a Car
class that extends the Vehicle
class. The Car
class has an additional brand
property and a displayBrand
method.
We create an instance of the Car
class using the new
keyword and call the move
method inherited from the Vehicle
class. We also call the displayBrand
method defined in the Car
class.
Inheritance allows us to reuse code from a base class and build upon it, promoting code reuse and enhancing the extensibility of our programs.
Creating and Using Anonymous Classes in TypeScript
Anonymous classes in TypeScript provide a way to define and instantiate a class without explicitly giving it a name. They are particularly useful when we need a small, one-off class that doesn’t need to be reused or referenced elsewhere in the code.
To create an anonymous class in TypeScript, we can use the new
keyword followed by the class
keyword and a set of curly braces containing the class members.
Related Article: TypeScript ETL (Extract, Transform, Load) Tutorial
Example 1: Creating an Anonymous Class
Here’s an example of creating an anonymous class in TypeScript:
const greeter = new class { greet() { console.log("Hello, world!"); } }(); greeter.greet(); // Output: Hello, world!
In this example, we define an anonymous class with a single method greet
that logs a greeting message. We then create an instance of the anonymous class using the new
keyword and assign it to the greeter
variable. Finally, we call the greet
method on the greeter
instance to display the greeting.
Anonymous classes are useful when we need to define a class on the fly, without the need for reuse or reference.
Example 2: Using Anonymous Classes as Function Parameters
Anonymous classes can also be used as function parameters, allowing for dynamic behavior and customization. Here’s an example:
class Logger { log(message: string) { console.log(`[INFO] ${message}`); } } function processMessage(message: string, loggerClass: new () => Logger) { const logger = new loggerClass(); logger.log(message); } processMessage("Hello, world!", class { log(message: string) { console.log(`[CUSTOM] ${message}`); } });
In this example, we define a Logger
class with a log
method that prefixes the message with [INFO]
. The processMessage
function takes a message
parameter and a loggerClass
parameter, which represents an anonymous class that implements the Logger
interface. Inside the processMessage
function, we create an instance of the loggerClass
and call its log
method to display the message.
Benefits and Use Cases of Anonymous Classes
Anonymous classes in TypeScript offer several benefits and can be used in various scenarios to enhance code organization and flexibility. Here are some of the benefits and use cases of anonymous classes:
1. Code organization: Anonymous classes allow developers to encapsulate related functionality within a single expression, making the code more concise and focused.
2. Improved readability: Anonymous classes can be used to define small, inline implementations of interfaces or abstract classes, making the code easier to read and understand by providing a clear context for the implementation.
3. Flexibility: Anonymous classes can be created on the fly, allowing for dynamic behavior and customization at runtime.
4. Encapsulation: Anonymous classes are typically used to implement interfaces or extend abstract classes, providing a level of abstraction and encapsulation that promotes modular and reusable code.
5. Reduced code duplication: By allowing developers to define and instantiate a class in a single expression, anonymous classes help reduce code duplication by eliminating the need to create separate classes for simple, one-off implementations.
Anonymous classes can be useful in scenarios where we need to define a class that is only used in a specific context or situation. They provide a way to create small, self-contained pieces of code without the need for separate class definitions.
Related Article: Tutorial on Circuit Breaker Pattern in TypeScript
Exploring Static Typing in TypeScript
Static typing is a key feature of TypeScript that allows developers to specify the types of variables, function parameters, and return values at compile-time. This enables the TypeScript compiler to perform type checking and provide helpful error messages, leading to more reliable and maintainable code.
In TypeScript, static typing is achieved through the use of type annotations. Type annotations specify the expected types of variables, function parameters, and return values, providing valuable information to the TypeScript compiler.
Example 1: Variable Type Annotation
Here’s an example of using static typing with variable type annotations in TypeScript:
let count: number = 0; let message: string = "Hello, TypeScript"; let isActive: boolean = true; let data: any = { name: "John", age: 25 }; console.log(count, message, isActive, data);
In this example, we use type annotations to specify the types of the variables count
, message
, isActive
, and data
. The TypeScript compiler will check that the assigned values match the specified types and raise an error if there is a mismatch.
Static typing helps catch errors early in the development process and provides better tooling support, such as code completion and type inference, which can improve productivity and code quality.
Example 2: Function Type Annotation
Static typing can also be applied to function parameters and return values. Here’s an example:
function addNumbers(a: number, b: number): number { return a + b; } console.log(addNumbers(5, 3)); // Output: 8
In this example, we define a function addNumbers
that takes two parameters a
and b
, both of type number
, and returns a value of type number
. The type annotations for the function parameters and return value help the TypeScript compiler enforce type safety and provide better error checking.
Static typing in TypeScript promotes code reliability and maintainability by catching type-related errors early in the development process. It also improves code documentation and provides better tooling support, helping developers write cleaner and more robust code.
Key Features and Differences Between TypeScript and JavaScript
TypeScript is a superset of JavaScript that adds optional static typing, advanced tooling, and additional language features to JavaScript. Here are some key features and differences between TypeScript and JavaScript:
1. Static typing: TypeScript allows developers to specify the types of variables, function parameters, and return values, enabling static type checking and catching type-related errors at compile-time. JavaScript, on the other hand, is dynamically typed and performs type checking at runtime.
2. Enhanced tooling: TypeScript provides better tooling support compared to JavaScript, including features such as code completion, type inference, and refactoring tools. These tools help developers write cleaner and more maintainable code.
3. Language features: TypeScript introduces several new language features that are not available in JavaScript, such as classes, interfaces, modules, and decorators. These features promote code organization, encapsulation, and reusability.
4. Compatibility with JavaScript: TypeScript is a superset of JavaScript, which means that any valid JavaScript code is also valid TypeScript code. This allows developers to gradually introduce TypeScript into existing JavaScript projects without the need for a complete rewrite.
5. Compilation step: TypeScript code needs to be compiled to JavaScript before it can be executed in a browser or a Node.js environment. This compilation step is performed by the TypeScript compiler, which transforms TypeScript code into standard JavaScript code.
TypeScript provides developers with the benefits of static typing, enhanced tooling, and additional language features, while maintaining compatibility with existing JavaScript codebases. It is a popular choice for large-scale projects and teams that value code reliability and maintainability.
What is Object-Oriented Programming?
Object-oriented programming (OOP) is a programming paradigm that organizes code into objects, which are instances of classes. It focuses on modeling real-world entities as objects, encapsulating data and behavior into reusable entities, and promoting code reusability and maintainability.
OOP is based on four main principles:
1. Encapsulation: Encapsulation is the process of bundling data and related behavior into a single unit, called an object. The data is hidden from the outside world and can only be accessed through methods or properties defined by the object. This promotes code organization and protects data integrity.
2. Inheritance: Inheritance allows classes to inherit properties and methods from other classes, promoting code reuse and creating a hierarchical structure. Inheritance enables the creation of more specialized classes that inherit the characteristics of a base class, allowing for code extension and customization.
3. Polymorphism: Polymorphism allows objects to take on multiple forms or behaviors. It enables the use of a single interface to represent different types of objects, promoting code flexibility and adaptability. Polymorphism is typically achieved through method overriding and interfaces.
4. Abstraction: Abstraction is the process of simplifying complex systems by modeling them at a higher level of abstraction. It involves identifying the essential features and ignoring the irrelevant details. Abstraction allows developers to focus on the relevant aspects of a problem and create reusable, modular code.
OOP provides several benefits, including code organization, code reusability, maintainability, and scalability. It allows for the creation of modular and extensible codebases, promotes a clear separation of concerns, and facilitates collaboration among developers.
How TypeScript Handles Type Annotations
TypeScript is a statically typed superset of JavaScript that allows developers to specify the types of variables, function parameters, and return values. Type annotations in TypeScript provide information about the expected types, enabling the TypeScript compiler to perform type checking and provide helpful error messages.
Type annotations in TypeScript are optional, which means that developers can choose to add them or rely on type inference, where the TypeScript compiler infers the types based on the assigned values.
When TypeScript encounters a type annotation, it checks that the assigned value matches the specified type. If there is a type mismatch, the TypeScript compiler raises an error.
TypeScript supports several basic types, including number, string, boolean, null, undefined, and object, as well as more complex types such as arrays, tuples, enums, and interfaces. Additionally, TypeScript allows developers to create custom types using type aliases and union types.
TypeScript also provides type inference, which means that the compiler can automatically infer the types of variables, function parameters, and return values based on the context and assigned values. This reduces the need for explicit type annotations in many cases.
Here’s an example that demonstrates how TypeScript handles type annotations:
let count: number = 0; let message: string = "Hello, TypeScript"; let isActive: boolean = true; let data: any = { name: "John", age: 25 }; console.log(count, message, isActive, data);
In this example, we use type annotations to specify the types of the variables count
, message
, isActive
, and data
. The TypeScript compiler will check that the assigned values match the specified types and raise an error if there is a mismatch.
TypeScript’s handling of type annotations helps catch errors early in the development process and improves code documentation and maintainability. It provides developers with a useful tool for writing more reliable and robust code.
Advanced Techniques for Working with Anonymous Classes
Working with anonymous classes in TypeScript opens up a range of possibilities for writing flexible and modular code. In addition to the basic usage of creating and using anonymous classes, there are several advanced techniques that can be employed to take advantage of their dynamic nature.
Example 1: Extending Functionality with Mixins
One way to enhance the functionality of anonymous classes is by using mixins. Mixins are a way of adding additional behavior to a class without inheriting from it. They allow for the composition of functionality from multiple sources, providing a flexible and reusable approach to code organization.
Here’s an example of using mixins with anonymous classes:
function withLogger(Base: T) { return class extends Base { log(message: string) { console.log(`[LOG] ${message}`); } }; } class Person { name: string; constructor(name: string) { this.name = name; } sayHello() { console.log(`Hello, my name is ${this.name}`); } } const LoggedPerson = withLogger(Person); const john = new LoggedPerson("John"); john.sayHello(); // Output: Hello, my name is John john.log("Logging from John"); // Output: [LOG] Logging from John
In this example, we define a withLogger
mixin that adds a log
method to the class it is applied to. The mixin takes a base class as a parameter and returns an anonymous class that extends the base class and adds the log
method.
We then use the withLogger
mixin to create a new class LoggedPerson
that extends the Person
class and includes the logging functionality. We create an instance of the LoggedPerson
class and call both the sayHello
and log
methods.
Mixins provide a useful way to extend the functionality of anonymous classes and promote code reuse and modularity.
Example 2: Implementing Decorators with Anonymous Classes
Another advanced technique for working with anonymous classes is using them in decorators. Decorators are a language feature in TypeScript that allows developers to modify the behavior of classes, methods, or properties at runtime.
Here’s an example of using anonymous classes in decorators:
function logAccess(target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value; descriptor.value = function (...args: any[]) { console.log(`Accessing method ${propertyKey}`); return originalMethod.apply(this, args); }; return descriptor; } class Person { name: string; constructor(name: string) { this.name = name; } @logAccess sayHello() { console.log(`Hello, my name is ${this.name}`); } } const john = new Person("John"); john.sayHello(); // Output: Accessing method sayHello // Hello, my name is John
In this example, we define a decorator function logAccess
that logs a message whenever a method is accessed. The decorator takes three parameters: target
, propertyKey
, and descriptor
. We extract the original method from the descriptor and replace it with an anonymous function that logs the access before calling the original method.
We then apply the logAccess
decorator to the sayHello
method of the Person
class. When we create an instance of the Person
class and call the sayHello
method, the decorator intercepts the method access and logs a message before executing the original method.
Decorators provide a useful way to modify the behavior of classes, methods, or properties at runtime, and anonymous classes can be used to define the modifications in a concise and modular way.
Common Pitfalls and Best Practices for Anonymous Classes in TypeScript
While working with anonymous classes in TypeScript can be beneficial, there are several common pitfalls to be aware of. By understanding these pitfalls and following best practices, developers can avoid potential issues and write cleaner and more maintainable code.
Pitfall 1: Overusing Anonymous Classes
One common pitfall is overusing anonymous classes where they are not necessary. While anonymous classes can be useful in certain situations, excessive use can lead to code that is difficult to understand and maintain. It is important to evaluate whether an anonymous class is the best approach or if a named class would be more appropriate.
Pitfall 2: Lack of Reusability
Another pitfall is the lack of reusability when using anonymous classes. Anonymous classes are typically used for small, one-off implementations that do not need to be reused elsewhere in the code. If there is a need for reuse or reference, it is recommended to use named classes instead.
Pitfall 3: Difficulty in Debugging
Debugging code that uses anonymous classes can be more challenging compared to named classes. Since anonymous classes do not have a distinct name, it can be harder to identify and debug issues related to them. Proper code documentation and meaningful variable names can help mitigate this pitfall.
Best Practice 1: Use Named Classes for Reusable Code
When creating code that is intended to be reused or referenced elsewhere, it is generally best to use named classes instead of anonymous classes. Named classes provide better code organization and maintainability, as well as improved debugging capabilities.
Best Practice 2: Limit the Use of Anonymous Classes
While anonymous classes can be handy in certain situations, it is important to limit their use to cases where they provide clear benefits. Overusing anonymous classes can lead to code that is difficult to understand and maintain. Consider whether a named class would be more appropriate for the specific use case.
Best Practice 3: Provide Meaningful Variable Names
When using anonymous classes, it is important to provide meaningful variable names to improve code readability and maintainability. Since anonymous classes do not have a distinct name, descriptive variable names can help clarify the purpose and functionality of the anonymous class.
Following these best practices can help developers avoid common pitfalls and write cleaner and more maintainable code when working with anonymous classes in TypeScript.
Using Anonymous Classes to Extend Functionality in TypeScript
Anonymous classes in TypeScript can be used to extend the functionality of existing classes, allowing for dynamic behavior and customization. By creating and instantiating anonymous classes, developers can add new methods or override existing ones, providing a flexible and modular approach to code extension.
Example 1: Extending Functionality with Anonymous Classes
Here’s an example of using anonymous classes to extend the functionality of an existing class:
class Calculator { add(a: number, b: number): number { return a + b; } } const extendedCalculator = new class extends Calculator { subtract(a: number, b: number): number { return a - b; } }(); console.log(extendedCalculator.add(5, 3)); // Output: 8 console.log(extendedCalculator.subtract(5, 3)); // Output: 2
In this example, we have a Calculator
class with an add
method that adds two numbers. We then create an anonymous class that extends the Calculator
class and adds a subtract
method that subtracts two numbers.
Example 2: Overriding Methods with Anonymous Classes
Anonymous classes can also be used to override existing methods in a base class. Here’s an example:
class Vehicle { move() { console.log("Moving..."); } } const enhancedVehicle = new class extends Vehicle { move() { console.log("Moving faster..."); } }(); enhancedVehicle.move(); // Output: Moving faster...
In this example, we have a Vehicle
class with a move
method that logs a generic message. We then create an anonymous class that extends the Vehicle
class and overrides the move
method with a more specific message.
Using anonymous classes to extend functionality provides a flexible and modular approach to code extension. It allows developers to add new methods or override existing ones without modifying the original implementation, promoting code reuse and maintainability.
Code Snippet: Creating an Anonymous Class with Inheritance
Here’s a code snippet that demonstrates how to create an anonymous class with inheritance in TypeScript:
class Animal { speak() { console.log("Animal speaks"); } } const dog = new Animal(); dog.speak(); // Output: Animal speaks const cat = new (class extends Animal { speak() { console.log("Cat speaks"); } })(); cat.speak(); // Output: Cat speaks
In this code snippet, we have a base class Animal
with a speak
method that logs a generic message. We then create an anonymous class that extends the Animal
class and overrides the speak
method with a more specific message.
Creating anonymous classes with inheritance allows for dynamic behavior and customization at runtime, providing a flexible and modular approach to code extension.
Code Snippet: Implementing Interfaces with Anonymous Classes
Here’s a code snippet that demonstrates how to implement interfaces using anonymous classes in TypeScript:
interface Greeting { greet(): void; } const greeter: Greeting = new (class implements Greeting { greet() { console.log("Hello, world!"); } })(); greeter.greet(); // Output: Hello, world!
In this code snippet, we define an interface
called Greeting
with a greet
method. We then create an anonymous class that implements the Greeting
interface and provides an implementation for the greet
method.
Implementing interfaces with anonymous classes allows for concise and inline implementations, making the code easier to read and understand.
Code Snippet: Using Anonymous Classes for Enhanced Modularity
Here’s a code snippet that demonstrates how to use anonymous classes for enhanced modularity in TypeScript:
function createLogger(): { log(message: string): void; } { return new (class { log(message: string) { console.log(`[LOG] ${message}`); } })(); } const logger = createLogger(); logger.log("Logging message"); // Output: [LOG] Logging message
In this code snippet, we define a function createLogger
that returns an object with a log
method. Inside the createLogger
function, we create an anonymous class that implements the log
method and returns an instance of the anonymous class.
Using anonymous classes for enhanced modularity allows for the creation of self-contained and reusable pieces of code, promoting code organization and maintainability.
Code Snippet: Leveraging Anonymous Classes for Decorators
Here’s a code snippet that demonstrates how to leverage anonymous classes for decorators in TypeScript:
function logAccess(target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value; descriptor.value = new (class { log(message: string) { console.log(`[LOG] ${message}`); } invoke(...args: any[]) { this.log(`Accessing method ${propertyKey}`); return originalMethod.apply(target, args); } })().invoke; return descriptor; } class Person { name: string; constructor(name: string) { this.name = name; } @logAccess sayHello() { console.log(`Hello, my name is ${this.name}`); } } const john = new Person("John"); john.sayHello(); // Output: [LOG] Accessing method sayHello // Hello, my name is John
In this code snippet, we define a decorator function logAccess
that logs a message whenever a method is accessed. The decorator takes three parameters: target
, propertyKey
, and descriptor
. We extract the original method from the descriptor and replace it with an anonymous class that adds a log
method and an invoke
method. The invoke
method logs the access message before calling the original method.
We then apply the logAccess
decorator to the sayHello
method of the Person
class. When we create an instance of the Person
class and call the sayHello
method, the decorator intercepts the method access, logs a message, and executes the original method.
Leveraging anonymous classes for decorators provides a useful and modular approach to modifying the behavior of classes, methods, or properties at runtime.
Code Snippet: Applying Anonymous Classes in Real-World Scenarios
Here’s a code snippet that demonstrates how anonymous classes can be applied in real-world scenarios:
interface Shape { area(): number; perimeter(): number; } function calculateMetrics(shape: Shape) { const calculatedArea = shape.area(); const calculatedPerimeter = shape.perimeter(); console.log("Calculated area:", calculatedArea); console.log("Calculated perimeter:", calculatedPerimeter); } const rectangle = { width: 5, height: 10, area() { return this.width * this.height; }, perimeter() { return 2 * (this.width + this.height); } }; calculateMetrics(rectangle);
In this code snippet, we define an interface
called Shape
with area
and perimeter
methods. We then define an anonymous class representing a rectangle object with properties width
and height
, as well as area
and perimeter
methods.
We then call the calculateMetrics
function with the rectangle
object as an argument. The function expects an object that implements the Shape
interface. The calculateMetrics
function calculates the area and perimeter of the shape and logs the results.
This example demonstrates how anonymous classes can be used to represent objects that adhere to a specific interface, allowing for dynamic behavior and customization.
External Sources
– TypeScript Handbook
– TypeScript Deep Dive
– MDN Web Docs: Classes