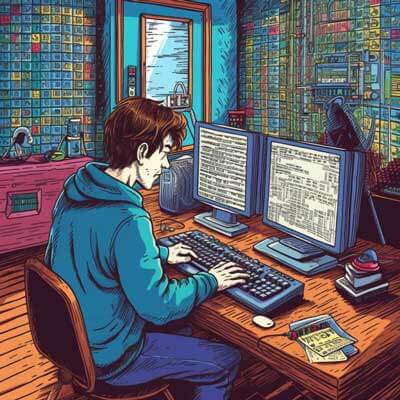
- Syntax for subtracting dates in TypeScript
- Calculating the difference between two dates in TypeScript
- Subtracting days from a date in TypeScript
- Useful date manipulation techniques in TypeScript
- Libraries and packages for date arithmetic in TypeScript
- Performing advanced date calculations in TypeScript
- Built-in functions for working with dates in TypeScript
- Utility functions or helpers for handling dates in TypeScript
- Common pitfalls when subtracting dates in TypeScript
- External Sources
Syntax for subtracting dates in TypeScript
In TypeScript, subtracting dates involves using the built-in Date
object and its methods. The basic syntax for subtracting dates in TypeScript is as follows:
const date1 = new Date(); const date2 = new Date(); const differenceInMilliseconds = date1.getTime() - date2.getTime();
In the example above, we create two Date
objects date1
and date2
. We then use the getTime()
method to get the time value in milliseconds for each date. Finally, we subtract the time values to get the difference in milliseconds.
Related Article: How to Implement and Use Generics in Typescript
Calculating the difference between two dates in TypeScript
To calculate the difference between two dates in TypeScript, we can subtract the time values in milliseconds, as shown in the previous example. However, this will give us the difference in milliseconds, which may not be very useful. To calculate the difference in a more meaningful format, we can use the following code:
const date1 = new Date(); const date2 = new Date(); const differenceInMilliseconds = date1.getTime() - date2.getTime(); const differenceInSeconds = differenceInMilliseconds / 1000; const differenceInMinutes = differenceInSeconds / 60; const differenceInHours = differenceInMinutes / 60; const differenceInDays = differenceInHours / 24;
In the example above, we calculate the difference in milliseconds between date1
and date2
. We then divide this difference by the appropriate conversion factor to get the difference in seconds, minutes, hours, and days.
Subtracting days from a date in TypeScript
To subtract days from a date in TypeScript, we can use the setDate()
method of the Date
object. Here’s an example:
const date = new Date(); const daysToSubtract = 7; date.setDate(date.getDate() - daysToSubtract);
In the example above, we create a Date
object date
, and specify the number of days to subtract in the daysToSubtract
variable. We then use the setDate()
method to subtract the specified number of days from the date.
Useful date manipulation techniques in TypeScript
In addition to basic date subtraction, TypeScript provides several useful techniques for date manipulation. Here are a few examples:
Formatting dates
To format dates in a specific way, TypeScript provides the toLocaleDateString()
method. Here’s an example:
const date = new Date(); const formattedDate = date.toLocaleDateString("en-US", { year: "numeric", month: "long", day: "numeric" });
In the example above, we create a Date
object date
and use the toLocaleDateString()
method to format the date according to the specified options. The resulting formattedDate
will be a string in the format “Month Day, Year”.
Adding or subtracting specific units of time
To add or subtract specific units of time, TypeScript provides methods like setFullYear()
, setMonth()
, setDate()
, setHours()
, setMinutes()
, setSeconds()
, and setMilliseconds()
. Here’s an example:
const date = new Date(); date.setFullYear(date.getFullYear() + 1); // Add 1 year date.setMonth(date.getMonth() - 6); // Subtract 6 months date.setDate(date.getDate() + 7); // Add 7 days
In the example above, we create a Date
object date
and use the appropriate methods to add or subtract specific units of time.
Related Article: How to Check If a String is in an Enum in TypeScript
Libraries and packages for date arithmetic in TypeScript
While TypeScript provides basic date arithmetic capabilities, there are also several libraries and packages available that offer more advanced and convenient features. Some popular options include:
– date-fns: A lightweight and modular library for date manipulation and formatting.
– Moment.js: A popular library for parsing, validating, manipulating, and formatting dates.
– Luxon: A library for working with dates and times in a friendly and modern way.
These libraries can simplify complex date calculations and provide additional functionality not available in the standard TypeScript Date
object.
Performing advanced date calculations in TypeScript
To perform advanced date calculations in TypeScript, you can combine the basic date arithmetic operations with additional logic and algorithms. Here’s an example of calculating the number of weekdays between two dates:
const startDate = new Date("2022-01-01"); const endDate = new Date("2022-01-31"); let currentDate = startDate; let weekdaysCount = 0; while (currentDate <= endDate) { const dayOfWeek = currentDate.getDay(); if (dayOfWeek !== 0 && dayOfWeek !== 6) { weekdaysCount++; } currentDate.setDate(currentDate.getDate() + 1); } console.log(weekdaysCount); // Output: 22
In the example above, we create Date
objects for the start and end dates. We then use a while
loop to iterate through each date between the start and end dates. For each date, we check if it’s a weekday (not Saturday or Sunday) and increment the weekdaysCount
variable accordingly. Finally, we log the total number of weekdays between the two dates.
Built-in functions for working with dates in TypeScript
In addition to the methods provided by the Date
object, TypeScript also offers built-in functions for working with dates. Some commonly used functions include:
– Date.now()
: Returns the current time value in milliseconds.
– Date.parse()
: Parses a date string and returns the time value in milliseconds.
– Date.UTC()
: Returns the time value in milliseconds for a specified UTC date and time.
Here’s an example of using the Date.now()
function:
const currentTime = Date.now(); console.log(currentTime); // Output: 1658657628364
In the example above, we use the Date.now()
function to get the current time value in milliseconds and store it in the currentTime
variable. We then log the value to the console.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Utility functions or helpers for handling dates in TypeScript
When working with dates in TypeScript, it can be helpful to create utility functions or helpers to simplify common operations. Here’s an example of a utility function that checks if a date is in the past:
function isPastDate(date: Date): boolean { const currentDate = new Date(); return date < currentDate; }
In the example above, we define a utility function isPastDate()
that takes a Date
object as a parameter. We create a new Date
object currentDate
representing the current date and compare it with the input date. If the input date is earlier than the current date, the function returns true
; otherwise, it returns false
.
Common pitfalls when subtracting dates in TypeScript
When subtracting dates in TypeScript, there are a few common pitfalls to be aware of:
1. Timezone differences: When working with dates, it’s important to consider the timezone of the dates involved. The Date
object in TypeScript operates based on the user’s local timezone. If you need to work with dates in a specific timezone, consider using a library like Luxon or Moment.js.
2. Daylight Saving Time: Daylight Saving Time can affect the accuracy of date subtraction, especially when dealing with time intervals spanning DST transitions. It’s important to account for DST changes when performing date calculations, as the duration of a day can vary.
3. Precision loss: When subtracting dates and performing calculations, there may be some precision loss due to the limited resolution of the Date
object. To mitigate this, consider using libraries or techniques that provide higher precision, such as using timestamps or working with UTC dates.