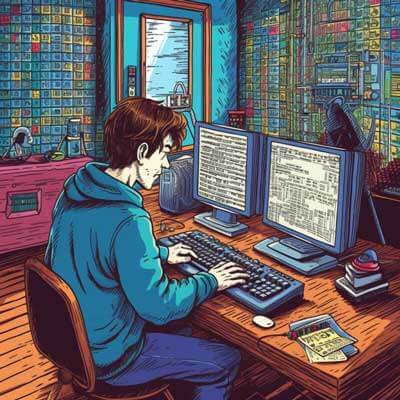
Table of Contents
In TypeScript, the ability to convert a boolean value to a string is an essential aspect of working with data and manipulating it in various ways. Converting a boolean to a string allows us to display or store the boolean value in a readable format, making it easier to work with in different scenarios. Whether you are building a user interface that requires displaying boolean values or manipulating data in a backend application, understanding how to convert boolean to string in TypeScript is crucial.
Different Approaches to Converting Boolean to String in TypeScript
There are several different approaches to converting a boolean value to a string in TypeScript. These approaches vary in their complexity, flexibility, and performance. In this section, we will explore some of the most common approaches to converting boolean to string in TypeScript.
Related Article: Tutorial: Working with Datetime Type in TypeScript
Using the toString() Method to Convert Boolean to String in TypeScript
One of the simplest and most straightforward ways to convert a boolean value to a string in TypeScript is by using the toString()
method. The toString()
method is a built-in function that is available on all JavaScript and TypeScript objects, including booleans.
Here is an example of how to use the toString()
method to convert a boolean value to a string in TypeScript:
const value: boolean = true; const stringValue: string = value.toString(); console.log(stringValue); // Output: "true"
In the example above, we define a boolean variable value
with the value true
. We then use the toString()
method to convert the boolean value to a string and assign it to the stringValue
variable. Finally, we log the stringValue
variable to the console, which will output the string representation of the boolean value, in this case, "true".
The toString()
method can also be used to convert false
to the string "false".
const value: boolean = false; const stringValue: string = value.toString(); console.log(stringValue); // Output: "false"
Using the toString()
method is a simple and effective way to convert a boolean value to a string in TypeScript. However, it is important to note that the toString()
method will always return the string "true" or "false" for boolean values, regardless of the language or locale settings.
Using Conditional Statements to Convert Boolean to String in TypeScript
Another approach to converting a boolean value to a string in TypeScript is by using conditional statements. Conditional statements allow us to check the value of a boolean and perform different actions based on its true or false state.
Here is an example of how to use conditional statements to convert a boolean value to a string in TypeScript:
const value: boolean = true; let stringValue: string; if (value) { stringValue = "true"; } else { stringValue = "false"; } console.log(stringValue); // Output: "true"
In the example above, we define a boolean variable value
with the value true
. We then declare a string variable stringValue
and use an if-else statement to check the value of value
. If value
is true, we assign the string "true" to stringValue
, otherwise, we assign the string "false". Finally, we log the value of stringValue
to the console, which will output "true".
Using conditional statements to convert a boolean to a string provides more flexibility than using the toString()
method. You can customize the string representation of the boolean value based on your specific requirements.
Using Template Literals to Convert Boolean to String in TypeScript
Template literals, introduced in ECMAScript 6, provide a useful way to create strings with embedded expressions. They can also be used to convert a boolean value to a string in TypeScript.
Here is an example of how to use template literals to convert a boolean value to a string in TypeScript:
const value: boolean = true; const stringValue: string = `${value}`; console.log(stringValue); // Output: "true"
In the example above, we define a boolean variable value
with the value true
. We then use a template literal, denoted by the backticks, to create a string that includes the value of value
. The expression ${value}
inside the template literal is evaluated and replaced with the string representation of the boolean value. Finally, we assign the resulting string to the stringValue
variable and log it to the console, which will output "true".
Using template literals provides a concise and readable way to convert a boolean value to a string in TypeScript. It also allows for more complex expressions and string manipulations if needed.
Related Article: Tutorial: Date Comparison in TypeScript
Handling Null or Undefined Boolean Values When Converting to String in TypeScript
In TypeScript, it is common to encounter null or undefined boolean values when working with data. When converting these values to a string, it is important to handle them appropriately to avoid unexpected behavior or errors.
Here is an example of how to handle null or undefined boolean values when converting to a string in TypeScript:
const value: boolean | null = null; const stringValue: string = value ? value.toString() : "null"; console.log(stringValue); // Output: "null"
In the example above, we define a variable value
with the type boolean | null
, which means it can either be a boolean value or null. We then use a conditional (ternary) operator to check if value
is null. If it is null, we assign the string "null" to stringValue
. Otherwise, we use the toString()
method to convert the boolean value to a string. Finally, we log the value of stringValue
to the console, which will output "null" in this case.
Understanding the Syntax for Converting Boolean to String in TypeScript
The syntax for converting a boolean value to a string in TypeScript depends on the approach chosen. Here is a summary of the syntax for the different approaches discussed in this article:
Using the toString()
method:
const value: boolean = true; const stringValue: string = value.toString();
Using conditional statements:
const value: boolean = true; let stringValue: string; if (value) { stringValue = "true"; } else { stringValue = "false"; }
Using template literals:
const value: boolean = true; const stringValue: string = `${value}`;
Handling null or undefined boolean values:
const value: boolean | null = null; const stringValue: string = value ? value.toString() : "null";
Understanding the syntax for converting a boolean to a string in TypeScript allows you to choose the approach that best fits your specific requirements and coding style.
Exploring Built-in Methods for Converting Boolean to String in TypeScript
In addition to the toString()
method, TypeScript provides several built-in methods that can be used to convert a boolean value to a string. These methods offer different functionalities and can be useful in various scenarios.
One such method is the String()
constructor. The String()
constructor creates a string object from the specified value. When called with a boolean value, it automatically converts the boolean to a string.
Here is an example of using the String()
constructor to convert a boolean value to a string in TypeScript:
const value: boolean = true; const stringValue: string = String(value); console.log(stringValue); // Output: "true"
In the example above, we use the String()
constructor to convert the boolean value true
to a string. The resulting string is assigned to the stringValue
variable, and when logged to the console, it outputs "true".
Another built-in method that can be used to convert a boolean to a string is the toLocaleString()
method. The toLocaleString()
method returns a string that represents the boolean value according to the system's locale settings.
const value: boolean = true; const stringValue: string = value.toLocaleString(); console.log(stringValue); // Output: "true"
In the example above, we use the toLocaleString()
method to convert the boolean value true
to a string. The resulting string is assigned to the stringValue
variable, and when logged to the console, it outputs "true".
These are just a few examples of the built-in methods available in TypeScript for converting boolean values to strings. Depending on your specific requirements, you can explore other built-in methods and choose the one that best suits your needs.
Using External Libraries for Converting Boolean to String in TypeScript
While TypeScript provides built-in methods and approaches for converting boolean values to strings, there may be situations where you need more advanced functionality or additional formatting options. In such cases, using external libraries can be a viable solution.
One popular library for working with boolean values and converting them to strings is the lodash
library. Lodash provides a wide range of utility functions, including functions for converting boolean values to strings.
To use the lodash
library for converting a boolean value to a string in TypeScript, you first need to install the library using npm or yarn:
npm install lodash
Once installed, you can import the necessary functions from the lodash
library and use them in your TypeScript code.
Here is an example of how to use the lodash
library to convert a boolean value to a string in TypeScript:
import { toString } from 'lodash'; const value: boolean = true; const stringValue: string = toString(value); console.log(stringValue); // Output: "true"
In the example above, we import the toString
function from the lodash
library and use it to convert the boolean value true
to a string. The resulting string is assigned to the stringValue
variable, and when logged to the console, it outputs "true".
Using external libraries like lodash
can provide additional functionality and flexibility when converting boolean values to strings in TypeScript. However, it is important to consider the size and performance implications of using external libraries in your project.
Related Article: Tutorial: Navigating the TypeScript Exit Process
Exploring Shorthand Syntax for Converting Boolean to String in TypeScript
In TypeScript, there is a shorthand syntax for converting a boolean value to a string. This shorthand syntax takes advantage of the fact that TypeScript can infer the type of a variable from its initial value.
Here is an example of how to use the shorthand syntax to convert a boolean value to a string in TypeScript:
const value = true; const stringValue = value.toString(); console.log(stringValue); // Output: "true"
In the example above, we omit the explicit type annotation for the value
and stringValue
variables. TypeScript infers the type of value
as boolean based on its initial value, and the type of stringValue
as string based on the result of the toString()
method. This shorthand syntax can make the code more concise and readable.
It is important to note that while the shorthand syntax can be convenient, it may not always be suitable for all situations. If you need to explicitly specify the type of a variable or if the initial value of the variable does not provide enough information for TypeScript to infer its type correctly, you should use explicit type annotations.
Possible String Representations for Boolean Values in TypeScript
When converting a boolean value to a string in TypeScript, there are two possible string representations: "true" and "false". These string representations correspond to the true and false states of a boolean value, respectively.
It is important to note that the string representations of boolean values are case-sensitive. The string "true" is not equal to the string "True", and the string "false" is not equal to the string "False".
Here are some examples of converting boolean values to strings in TypeScript:
const value1: boolean = true; const stringValue1: string = value1.toString(); console.log(stringValue1); // Output: "true" const value2: boolean = false; const stringValue2: string = value2.toString(); console.log(stringValue2); // Output: "false"
In the examples above, we convert the boolean values true
and false
to strings using the toString()
method. The resulting strings are assigned to the stringValue1
and stringValue2
variables, respectively. When logged to the console, stringValue1
outputs "true" and stringValue2
outputs "false".
Understanding the possible string representations for boolean values in TypeScript allows you to correctly handle and manipulate boolean values in your code.
External Sources