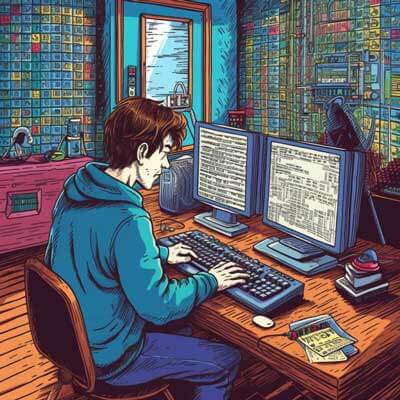
- HTMLButtonElement Overview
- Properties
- Methods
- Creating a Button Element in TypeScript
- Adding a Button to the HTML using TypeScript
- Styling a Button Element in TypeScript
- Disabling a Button using TypeScript
- Adding an Event Listener to a Button Element in TypeScript
- Handling Click Events on a Button in TypeScript
- Common Attributes of a Button Element in HTML
- Changing the Text of a Button Element in TypeScript
- Dynamically Creating and Appending a Button Element in TypeScript
- Removing a Button Element from the DOM using TypeScript
- External Sources
HTMLButtonElement Overview
The HTMLButtonElement interface represents a button element in the HTML document object model (DOM). It inherits properties and methods from the HTMLElement interface and provides additional functionality specific to buttons.
Buttons are widely used in web development to trigger actions or submit forms. The HTMLButtonElement interface allows you to access and manipulate button elements using TypeScript.
To work with HTMLButtonElement in TypeScript, you need to understand its properties and methods. Let’s explore some of the key ones:
Related Article: Tutorial: Extending the Window Object in TypeScript
Properties
– autofocus
: A boolean property that specifies whether the button should automatically have focus when the page loads. The default value is false.
– disabled
: A boolean property that determines whether the button is disabled. A disabled button cannot be clicked or receive focus. The default value is false.
– form: A readonly property that returns the form element associated with the button, if any.
– name
: A string property that represents the name of the button, which is submitted with the form data when the button is clicked.
– type
: A string property that specifies the type of button. Possible values are “submit”, “reset”, and “button”. The default value is “submit”.
Methods
– click()
: A method that simulates a mouse click on the button element. This can be useful for triggering button actions programmatically.
Now that we have an overview of the HTMLButtonElement interface, let’s see how to create and work with button elements in TypeScript.
Creating a Button Element in TypeScript
To create a button element in TypeScript, you can use the createElement
method of the document object. Here’s an example:
const button = document.createElement('button');
In this example, we create a new button element and assign it to the variable button
. The createElement
method takes the tag name of the element as a parameter.
Related Article: How to Use the MouseEventHandlers in TypeScript
Adding a Button to the HTML using TypeScript
Once you have created a button element, you can add it to the HTML document using the appendChild
method. Here’s an example:
const button = document.createElement('button'); button.textContent = 'Click me'; document.body.appendChild(button);
In this example, we set the text content of the button element to “Click me” using the textContent
property. Then, we append the button element to the body
element of the HTML document using the appendChild
method.
Styling a Button Element in TypeScript
To style a button element in TypeScript, you can use the style
property of the button element. Here’s an example:
const button = document.createElement('button'); button.textContent = 'Click me'; button.style.backgroundColor = 'blue'; button.style.color = 'white';
In this example, we set the background color of the button to blue and the text color to white using the style
property. You can apply various CSS styles to the button element using this property.
Disabling a Button using TypeScript
To disable a button element in TypeScript, you can set the disabled
property to true. Here’s an example:
const button = document.createElement('button'); button.textContent = 'Click me'; button.disabled = true;
In this example, we set the disabled
property of the button element to true, which disables the button. A disabled button cannot be clicked or receive focus.
Adding an Event Listener to a Button Element in TypeScript
To add an event listener to a button element in TypeScript, you can use the addEventListener
method. Here’s an example:
const button = document.createElement('button'); button.textContent = 'Click me'; button.addEventListener('click', () => { console.log('Button clicked'); });
In this example, we add a click event listener to the button element using the addEventListener
method. When the button is clicked, the callback function is executed, and it logs “Button clicked” to the console.
Handling Click Events on a Button in TypeScript
When a button is clicked, you can perform various actions or execute a specific function. Here’s an example of handling click events on a button in TypeScript:
const button = document.createElement('button'); button.textContent = 'Click me'; button.addEventListener('click', () => { // Perform action or call a function });
In this example, we add a click event listener to the button element and define the callback function to perform the desired action or call a specific function.
Common Attributes of a Button Element in HTML
In addition to the properties and methods provided by the HTMLButtonElement interface, a button element in HTML can have various attributes that define its behavior and appearance. Here are some common attributes of a button element in HTML:
– id
: Specifies a unique identifier for the button element.
– class
: Specifies one or more class names for the button element, which can be used for styling purposes.
– value
: Specifies the initial value of the button element, which is submitted with the form data when the button is clicked.
These attributes can be accessed and modified using the corresponding properties of the HTMLButtonElement interface in TypeScript.
Changing the Text of a Button Element in TypeScript
To change the text content of a button element in TypeScript, you can simply assign a new value to the textContent
property. Here’s an example:
const button = document.createElement('button'); button.textContent = 'Click me'; // Change the text content button.textContent = 'New text';
In this example, we create a button element with the initial text content “Click me”. We then assign a new value “New text” to the textContent
property, which changes the text displayed on the button.
Dynamically Creating and Appending a Button Element in TypeScript
In some cases, you may need to dynamically create and append button elements based on certain conditions or user interactions. Here’s an example of dynamically creating and appending a button element in TypeScript:
function createButton(text: string) { const button = document.createElement('button'); button.textContent = text; document.body.appendChild(button); } // Usage createButton('Click me');
In this example, we define a function createButton
that takes a string parameter text
. Inside the function, we create a button element, set its text content to the provided text
value, and then append it to the body
element of the HTML document. You can call this function with different text values to create and append button elements dynamically.
Removing a Button Element from the DOM using TypeScript
To remove a button element from the DOM (Document Object Model) using TypeScript, you can use the removeChild
method of the parent element. Here’s an example:
const button = document.createElement('button'); button.textContent = 'Click me'; document.body.appendChild(button); // Remove the button element document.body.removeChild(button);
In this example, we create a button element and append it to the body
element of the HTML document. Then, we use the removeChild
method of the body
element to remove the button element from the DOM.
External Sources
Here are some external sources that provide further information on working with HTMLButtonElement in TypeScript:
– MDN Web Docs: HTMLButtonElement
– TypeScript Handbook: DOM Manipulation