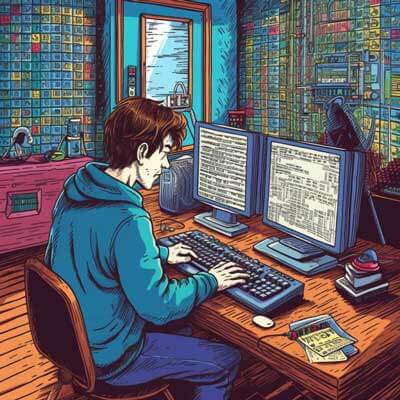
- Overview
- Writing to a File
- Appending to a File
- All File Handling Operations in Java
- Overview
- Creating a File
- Deleting a File
- Handling All File Types in Java
- Overview
- Processing Image Files in Java
- Processing Binary Files in Java
- Parsing CSV Files in Java
- Overview
- Reading CSV Files
- Writing to CSV Files
- Parsing XML Files in Java
- Overview
- Reading XML Files
- Writing to XML Files
- Advanced File Handling Use Cases in Java
- Overview
- Set the Last Modified Date of a File
- Get the Creation Date of a File
- Get the Owner of a File
- Get the Permissions of a File
- Get the File Attributes (Read-Only, Hidden, etc.)
- Check if a File is a Directory
- Check if a File is a Symbolic Link
- Handle File Not Found Exception
- Handle File Access Denied Exception
- Handle File Read or Write Error
- Handle File Permission Denied Exception
- Handle File Open Failure Exception
- Handle Disk Full Exception
- Handle Invalid File Path Exception
- Handle File Format Mismatch Exception
- Best Practices for Java File Handling
- Overview
- Close File Resources
- Use try-with-resources
- Handle Exceptions Appropriately
- Use Buffered I/O for Better Performance
- Handle Large Files with Memory Constraints
- Real World Examples of Java File Handling
- Overview
- Example 1: Log File Analysis
- Example 2: File Synchronization
- Performance Considerations for Java File Handling
- Overview
- Use Buffered I/O for Large Files
- Consider File Channel and Memory-Mapped I/O
- Avoid Redundant File Operations
- Parallelize File Operations
- Consider Asynchronous I/O
- Advanced Techniques for Java File Handling
- Overview
- Working with File Attributes
- Monitoring File System Events
- Working with Symbolic Links
- Working with File Locks
- Code Snippet Ideas for Java File Handling (1 of 5)
- Overview
- Code Snippet 1: Read Text File Line by Line
- Code Snippet 2: Write Text File Line by Line
- Code Snippet 3: Read Binary File
- Code Snippet 4: Write Binary File
- Code Snippet 5: Copy File
Overview
In Java, writing to a file is a common operation when working with file handling. It allows you to store data or modify existing data in a file. This chapter will cover various techniques and methods for performing write operations on files in Java.
Related Article: How To Parse JSON In Java
Writing to a File
To write data to a file in Java, you can use the FileWriter class. Here’s an example that demonstrates how to write a string to a file:
import java.io.FileWriter; import java.io.IOException; public class WriteToFileExample { public static void main(String[] args) { String data = "Hello, world!"; try { FileWriter writer = new FileWriter("output.txt"); writer.write(data); writer.close(); System.out.println("Data written to the file successfully."); } catch (IOException e) { System.out.println("An error occurred while writing to the file."); e.printStackTrace(); } } }
In the above example, we create a FileWriter object and provide the name of the file (“output.txt”) as an argument. We then call the write()
method of the FileWriter object to write the string data
to the file. Finally, we close the FileWriter using the close()
method.
Appending to a File
If you want to append data to an existing file, rather than overwriting it, you can use the FileWriter constructor that takes a boolean parameter indicating whether to append to the file. Here’s an example:
import java.io.FileWriter; import java.io.IOException; public class AppendToFileExample { public static void main(String[] args) { String data = "Hello, world again!"; try { FileWriter writer = new FileWriter("output.txt", true); writer.write(data); writer.close(); System.out.println("Data appended to the file successfully."); } catch (IOException e) { System.out.println("An error occurred while appending to the file."); e.printStackTrace(); } } }
In this example, we pass true
as the second argument to the FileWriter constructor, indicating that we want to append to the file rather than overwrite it.
All File Handling Operations in Java
Related Article: How To Convert Array To List In Java
Overview
Java provides a rich set of APIs for performing various file handling operations. This chapter will cover all the essential file handling operations that you can perform in Java.
Creating a File
To create a new file in Java, you can use the File class. Here’s an example:
import java.io.File; import java.io.IOException; public class CreateFileExample { public static void main(String[] args) { File file = new File("newfile.txt"); try { if (file.createNewFile()) { System.out.println("File created successfully."); } else { System.out.println("File already exists."); } } catch (IOException e) { System.out.println("An error occurred while creating the file."); e.printStackTrace(); } } }
In this example, we create a File object with the name “newfile.txt”. We then call the createNewFile()
method of the File object to create the file. If the file doesn’t exist, it will be created, and the method will return true
. If the file already exists, the method will return false
.
Deleting a File
To delete a file in Java, you can use the delete()
method of the File class. Here’s an example:
import java.io.File; public class DeleteFileExample { public static void main(String[] args) { File file = new File("fileToDelete.txt"); if (file.delete()) { System.out.println("File deleted successfully."); } else { System.out.println("Failed to delete the file."); } } }
In this example, we create a File object with the name “fileToDelete.txt”. We then call the delete()
method of the File object to delete the file. If the file is deleted successfully, the method will return true
. Otherwise, it will return false
.
Related Article: How To Iterate Over Entries In A Java Map
Handling All File Types in Java
Overview
Java provides support for handling various file types, including text files, binary files, image files, CSV files, and XML files. This chapter will demonstrate how to handle different file types in Java.
Processing Image Files in Java
To process image files in Java, you can use the ImageIO class from the javax.imageio package. Here’s an example that reads an image file and saves it in a different format:
import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; public class ImageProcessingExample { public static void main(String[] args) { try { File inputFile = new File("input.jpg"); BufferedImage image = ImageIO.read(inputFile); File outputFile = new File("output.png"); ImageIO.write(image, "png", outputFile); System.out.println("Image processed successfully."); } catch (IOException e) { System.out.println("An error occurred while processing the image."); e.printStackTrace(); } } }
In this example, we read an image file (“input.jpg”) using the ImageIO.read()
method and store it in a BufferedImage object. We then create an output file (“output.png”) and use the ImageIO.write()
method to save the image in PNG format.
Related Article: How To Split A String In Java
Processing Binary Files in Java
To process binary files in Java, you can use the FileInputStream and FileOutputStream classes. Here’s an example that copies a binary file:
import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; public class BinaryFileProcessingExample { public static void main(String[] args) { try { FileInputStream inputFile = new FileInputStream("input.bin"); FileOutputStream outputFile = new FileOutputStream("output.bin"); int data; while ((data = inputFile.read()) != -1) { outputFile.write(data); } inputFile.close(); outputFile.close(); System.out.println("Binary file processed successfully."); } catch (IOException e) { System.out.println("An error occurred while processing the binary file."); e.printStackTrace(); } } }
In this example, we create a FileInputStream object to read the input binary file (“input.bin”) and a FileOutputStream object to write the output binary file (“output.bin”). We then read data from the input file byte by byte using the read()
method and write it to the output file using the write()
method.
Parsing CSV Files in Java
Overview
CSV (Comma-Separated Values) files are a common format for storing tabular data. Java provides libraries that make it easy to parse CSV files and extract data. This chapter will demonstrate how to parse CSV files in Java.
Related Article: How To Convert Java Objects To JSON With Jackson
Reading CSV Files
To read data from a CSV file in Java, you can use a library like OpenCSV or Apache Commons CSV. Here’s an example using the OpenCSV library:
import com.opencsv.CSVReader; import java.io.FileReader; import java.io.IOException; public class CSVFileParsingExample { public static void main(String[] args) { try { CSVReader reader = new CSVReader(new FileReader("data.csv")); String[] line; while ((line = reader.readNext()) != null) { for (String value : line) { System.out.print(value + " "); } System.out.println(); } reader.close(); } catch (IOException e) { System.out.println("An error occurred while parsing the CSV file."); e.printStackTrace(); } } }
In this example, we use the CSVReader class from the OpenCSV library to read the CSV file (“data.csv”). We read each line of the file using the readNext()
method, which returns an array of strings representing the values in each line. We then print the values to the console.
Writing to CSV Files
To write data to a CSV file in Java, you can use the CSVWriter class from the OpenCSV library. Here’s an example:
import com.opencsv.CSVWriter; import java.io.FileWriter; import java.io.IOException; public class CSVFileWritingExample { public static void main(String[] args) { try { CSVWriter writer = new CSVWriter(new FileWriter("data.csv")); String[] line1 = {"Name", "Age", "Email"}; String[] line2 = {"John Doe", "25", "johndoe@example.com"}; String[] line3 = {"Jane Smith", "30", "janesmith@example.com"}; writer.writeNext(line1); writer.writeNext(line2); writer.writeNext(line3); writer.close(); System.out.println("Data written to the CSV file successfully."); } catch (IOException e) { System.out.println("An error occurred while writing to the CSV file."); e.printStackTrace(); } } }
In this example, we use the CSVWriter class to write data to the CSV file (“data.csv”). We create string arrays representing each line of data and pass them to the writeNext()
method of the CSVWriter object. Finally, we close the writer.
Parsing XML Files in Java
Related Article: Storing Contact Information in Java Data Structures
Overview
XML (eXtensible Markup Language) is a popular format for storing structured data. Java provides libraries that make it easy to parse XML files and extract data. This chapter will demonstrate how to parse XML files in Java.
Reading XML Files
To read data from an XML file in Java, you can use libraries like DOM (Document Object Model) or SAX (Simple API for XML). Here’s an example using the DOM library:
import org.w3c.dom.*; import javax.xml.parsers.*; import java.io.File; public class XMLFileParsingExample { public static void main(String[] args) { try { File inputFile = new File("data.xml"); DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse(inputFile); Element root = document.getDocumentElement(); NodeList nodeList = root.getElementsByTagName("person"); for (int i = 0; i < nodeList.getLength(); i++) { Node node = nodeList.item(i); if (node.getNodeType() == Node.ELEMENT_NODE) { Element element = (Element) node; String name = element.getElementsByTagName("name").item(0).getTextContent(); String age = element.getElementsByTagName("age").item(0).getTextContent(); String email = element.getElementsByTagName("email").item(0).getTextContent(); System.out.println("Name: " + name); System.out.println("Age: " + age); System.out.println("Email: " + email); System.out.println(); } } } catch (Exception e) { System.out.println("An error occurred while parsing the XML file."); e.printStackTrace(); } } }
In this example, we use the DocumentBuilder class to parse the XML file (“data.xml”) and obtain a Document object. We then access the root element of the document using the getDocumentElement()
method. From the root element, we can navigate the XML tree using various methods like getElementsByTagName()
to extract the desired data.
Writing to XML Files
To write data to an XML file in Java, you can use libraries like DOM or JAXB (Java Architecture for XML Binding). Here’s an example using the DOM library:
import org.w3c.dom.*; import javax.xml.parsers.*; import javax.xml.transform.*; import javax.xml.transform.dom.DOMSource; import javax.xml.transform.stream.StreamResult; import java.io.File; public class XMLFileWritingExample { public static void main(String[] args) { try { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.newDocument(); Element rootElement = document.createElement("bookstore"); document.appendChild(rootElement); Element bookElement = document.createElement("book"); rootElement.appendChild(bookElement); Element titleElement = document.createElement("title"); titleElement.appendChild(document.createTextNode("Java Programming")); bookElement.appendChild(titleElement); Element authorElement = document.createElement("author"); authorElement.appendChild(document.createTextNode("John Doe")); bookElement.appendChild(authorElement); Element priceElement = document.createElement("price"); priceElement.appendChild(document.createTextNode("29.99")); bookElement.appendChild(priceElement); TransformerFactory transformerFactory = TransformerFactory.newInstance(); Transformer transformer = transformerFactory.newTransformer(); DOMSource source = new DOMSource(document); StreamResult result = new StreamResult(new File("data.xml")); transformer.transform(source, result); System.out.println("Data written to the XML file successfully."); } catch (Exception e) { System.out.println("An error occurred while writing to the XML file."); e.printStackTrace(); } } }
In this example, we create a new XML document using the DocumentBuilder class. We then create various elements using the createElement()
method and add them to the document hierarchy using the appendChild()
method. Finally, we transform the document into an XML file using the Transformer class and the transform()
method.
Related Article: How to Convert JSON String to Java Object
Advanced File Handling Use Cases in Java
Overview
In addition to basic file handling operations, Java provides advanced features for handling files, such as manipulating file attributes, handling exceptions, and implementing best practices. This chapter will explore advanced file handling use cases in Java.
Set the Last Modified Date of a File
To set the last modified date of a file in Java, you can use the setLastModified()
method of the File class. Here’s an example:
import java.io.File; public class SetLastModifiedDateExample { public static void main(String[] args) { File file = new File("file.txt"); long timestamp = System.currentTimeMillis(); if (file.setLastModified(timestamp)) { System.out.println("Last modified date set successfully."); } else { System.out.println("Failed to set the last modified date."); } } }
In this example, we create a File object representing the file “file.txt”. We then obtain the current timestamp using System.currentTimeMillis()
and pass it to the setLastModified()
method of the File object. If the last modified date is set successfully, the method will return true
. Otherwise, it will return false
.
Related Article: How to Retrieve Current Date and Time in Java
Get the Creation Date of a File
Java does not provide a built-in method to directly retrieve the creation date of a file. However, you can obtain the creation date indirectly by retrieving the file’s attributes. Here’s an example using the BasicFileAttributes class from the java.nio.file package:
import java.nio.file.*; import java.io.IOException; public class GetCreationDateExample { public static void main(String[] args) { Path path = Paths.get("file.txt"); try { BasicFileAttributes attributes = Files.readAttributes(path, BasicFileAttributes.class); FileTime creationTime = attributes.creationTime(); System.out.println("Creation date: " + creationTime); } catch (IOException e) { System.out.println("An error occurred while retrieving the creation date."); e.printStackTrace(); } } }
In this example, we create a Path object representing the file “file.txt”. We then use the Files.readAttributes()
method to obtain the BasicFileAttributes object for the file. From the BasicFileAttributes object, we can access the creation time using the creationTime()
method.
Get the Owner of a File
To get the owner of a file in Java, you can use the Files class from the java.nio.file package. Here’s an example:
import java.nio.file.*; import java.io.IOException; public class GetFileOwnerExample { public static void main(String[] args) { Path path = Paths.get("file.txt"); try { FileOwnerAttributeView ownerAttributeView = Files.getFileAttributeView(path, FileOwnerAttributeView.class); UserPrincipal owner = ownerAttributeView.getOwner(); System.out.println("File owner: " + owner.getName()); } catch (IOException e) { System.out.println("An error occurred while retrieving the file owner."); e.printStackTrace(); } } }
In this example, we create a Path object representing the file “file.txt”. We then use the Files.getFileAttributeView()
method to obtain the FileOwnerAttributeView object for the file. From the FileOwnerAttributeView object, we can retrieve the owner of the file using the getOwner()
method.
Get the Permissions of a File
To get the permissions of a file in Java, you can use the Files class from the java.nio.file package. Here’s an example:
import java.nio.file.*; import java.io.IOException; import java.nio.file.attribute.*; public class GetFilePermissionsExample { public static void main(String[] args) { Path path = Paths.get("file.txt"); try { PosixFileAttributes attributes = Files.readAttributes(path, PosixFileAttributes.class); Set<PosixFilePermission> permissions = attributes.permissions(); System.out.println("File permissions: " + permissions); } catch (IOException e) { System.out.println("An error occurred while retrieving the file permissions."); e.printStackTrace(); } } }
In this example, we create a Path object representing the file “file.txt”. We then use the Files.readAttributes()
method to obtain the PosixFileAttributes object for the file, which contains the file’s permissions. We retrieve the permissions using the permissions()
method.
Related Article: How to Reverse a String in Java
Get the File Attributes (Read-Only, Hidden, etc.)
To get the file attributes (such as read-only or hidden) of a file in Java, you can use the Files class from the java.nio.file package. Here’s an example:
import java.nio.file.*; import java.io.IOException; import java.nio.file.attribute.*; public class GetFileAttributesExample { public static void main(String[] args) { Path path = Paths.get("file.txt"); try { BasicFileAttributes attributes = Files.readAttributes(path, BasicFileAttributes.class); System.out.println("Is read-only: " + attributes.isReadOnly()); System.out.println("Is hidden: " + attributes.isHidden()); System.out.println("Is archive: " + attributes.isArchive()); System.out.println("Is system: " + attributes.isSystem()); } catch (IOException e) { System.out.println("An error occurred while retrieving the file attributes."); e.printStackTrace(); } } }
In this example, we create a Path object representing the file “file.txt”. We then use the Files.readAttributes()
method to obtain the BasicFileAttributes object for the file. We can then access various attributes of the file using methods like isReadOnly()
, isHidden()
, isArchive()
, and isSystem()
.
Check if a File is a Directory
To check if a file is a directory in Java, you can use the isDirectory() method of the File class. Here’s an example:
import java.io.File; public class CheckIfDirectoryExample { public static void main(String[] args) { File file = new File("directory"); if (file.isDirectory()) { System.out.println("The file is a directory."); } else { System.out.println("The file is not a directory."); } } }
In this example, we create a File object representing a file named “directory”. We then use the isDirectory()
method to check if it is a directory. If the file is a directory, the method will return true
. Otherwise, it will return false
.
Check if a File is a Symbolic Link
To check if a file is a symbolic link in Java, you can use the isSymbolicLink() method of the Files class from the java.nio.file package. Here’s an example:
import java.nio.file.*; import java.io.IOException; public class CheckIfSymbolicLinkExample { public static void main(String[] args) { Path path = Paths.get("symlink"); try { boolean isSymbolicLink = Files.isSymbolicLink(path); if (isSymbolicLink) { System.out.println("The file is a symbolic link."); } else { System.out.println("The file is not a symbolic link."); } } catch (IOException e) { System.out.println("An error occurred while checking if the file is a symbolic link."); e.printStackTrace(); } } }
In this example, we create a Path object representing a file named “symlink”. We then use the Files.isSymbolicLink()
method to check if it is a symbolic link. If the file is a symbolic link, the method will return true
. Otherwise, it will return false
.
Related Article: How to Generate Random Integers in a Range in Java
Handle File Not Found Exception
When working with files in Java, it’s important to handle exceptions that may occur. One common exception is the FileNotFoundException, which is thrown when a file is not found. Here’s an example of how to handle this exception:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class HandleFileNotFoundExceptionExample { public static void main(String[] args) { try { File file = new File("nonexistent.txt"); Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); System.out.println(line); } scanner.close(); } catch (FileNotFoundException e) { System.out.println("The file was not found."); e.printStackTrace(); } } }
In this example, we create a File object representing a non-existent file (“nonexistent.txt”). We then create a Scanner object to read the file. Since the file doesn’t exist, a FileNotFoundException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Handle File Access Denied Exception
Another common exception when working with files in Java is the AccessDeniedException, which is thrown when access to a file is denied. Here’s an example of how to handle this exception:
import java.nio.file.*; import java.io.IOException; public class HandleAccessDeniedExceptionExample { public static void main(String[] args) { try { Path path = Paths.get("protected.txt"); Files.delete(path); } catch (AccessDeniedException e) { System.out.println("Access to the file is denied."); e.printStackTrace(); } catch (IOException e) { System.out.println("An error occurred while deleting the file."); e.printStackTrace(); } } }
In this example, we create a Path object representing a file (“protected.txt”) that we don’t have permission to delete. When we attempt to delete the file using Files.delete()
, an AccessDeniedException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Handle File Read or Write Error
When reading or writing files in Java, it’s important to handle errors that may occur during the process. One common error is an IOException, which can be thrown for various reasons, such as disk errors or insufficient permissions. Here’s an example of how to handle this error:
import java.io.*; public class HandleFileReadOrWriteErrorExample { public static void main(String[] args) { try { FileReader reader = new FileReader("file.txt"); FileWriter writer = new FileWriter("output.txt"); int data; while ((data = reader.read()) != -1) { writer.write(data); } reader.close(); writer.close(); } catch (IOException e) { System.out.println("An error occurred while reading or writing the file."); e.printStackTrace(); } } }
In this example, we create a FileReader object to read from a file (“file.txt”) and a FileWriter object to write to an output file. Inside the while loop, we read data from the input file and write it to the output file. If an IOException occurs, we catch it and handle it by printing a message and the stack trace.
Related Article: Java Equals Hashcode Tutorial
Handle File Permission Denied Exception
When working with files in Java, it’s essential to handle exceptions related to file permissions. One such exception is the FileSystemException, which can be thrown when permission to perform a file operation is denied. Here’s an example of how to handle this exception:
import java.nio.file.*; import java.io.IOException; public class HandlePermissionDeniedExceptionExample { public static void main(String[] args) { try { Path path = Paths.get("protected.txt"); Files.delete(path); } catch (FileSystemException e) { System.out.println("Permission to delete the file is denied."); e.printStackTrace(); } catch (IOException e) { System.out.println("An error occurred while deleting the file."); e.printStackTrace(); } } }
In this example, we create a Path object representing a file (“protected.txt”) that we don’t have permission to delete. When we attempt to delete the file using Files.delete()
, a FileSystemException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Handle File Open Failure Exception
When working with files in Java, it’s crucial to handle exceptions that may occur when opening a file. One such exception is the FileAlreadyExistsException, which is thrown when a file cannot be opened because it already exists as a directory. Here’s an example of how to handle this exception:
import java.io.*; import java.nio.file.*; public class HandleFileOpenFailureExceptionExample { public static void main(String[] args) { try { FileOutputStream fos = new FileOutputStream("directory"); fos.close(); } catch (FileAlreadyExistsException e) { System.out.println("A directory with the same name already exists."); e.printStackTrace(); } catch (IOException e) { System.out.println("An error occurred while opening the file."); e.printStackTrace(); } } }
In this example, we create a FileOutputStream object to open a file named “directory”. However, a directory with the same name already exists, so a FileAlreadyExistsException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Handle Disk Full Exception
When working with files in Java, it’s important to handle exceptions that may occur due to disk full conditions. One such exception is the FileSystemException, which can be thrown when there is insufficient space on the disk. Here’s an example of how to handle this exception:
import java.io.*; import java.nio.file.*; public class HandleDiskFullExceptionExample { public static void main(String[] args) { try { FileOutputStream fos = new FileOutputStream("file.txt"); byte[] data = new byte[1000000000]; fos.write(data); fos.close(); } catch (FileSystemException e) { System.out.println("The disk is full."); e.printStackTrace(); } catch (IOException e) { System.out.println("An error occurred while writing to the file."); e.printStackTrace(); } } }
In this example, we create a FileOutputStream object to write to a file (“file.txt”). We then write a large amount of data to the file using the write()
method. If the disk is full, a FileSystemException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Related Article: How To Convert String To Int In Java
Handle Invalid File Path Exception
When working with files in Java, it’s essential to handle exceptions related to invalid file paths. One such exception is the InvalidPathException, which can be thrown when a file path is invalid. Here’s an example of how to handle this exception:
import java.nio.file.*; import java.io.IOException; public class HandleInvalidFilePathExceptionExample { public static void main(String[] args) { try { Path path = Paths.get("file.txt\\invalid"); Files.createFile(path); } catch (InvalidPathException e) { System.out.println("The file path is invalid."); e.printStackTrace(); } catch (IOException e) { System.out.println("An error occurred while creating the file."); e.printStackTrace(); } } }
In this example, we create a Path object with an invalid file path (“file.txt\invalid”). When we attempt to create a file using Files.createFile()
, an InvalidPathException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Handle File Format Mismatch Exception
When working with files in Java, it’s important to handle exceptions related to file format mismatches. One such exception is the InvalidClassException, which can be thrown when deserializing an object from a file if the class of the serialized object does not match the class of the object being deserialized. Here’s an example of how to handle this exception:
import java.io.*; public class HandleFileFormatMismatchExceptionExample { public static void main(String[] args) { try { FileInputStream fis = new FileInputStream("data.ser"); ObjectInputStream ois = new ObjectInputStream(fis); Object obj = ois.readObject(); MyClass myObj = (MyClass) obj; ois.close(); } catch (InvalidClassException e) { System.out.println("The file format does not match the class definition."); e.printStackTrace(); } catch (IOException | ClassNotFoundException e) { System.out.println("An error occurred while deserializing the object."); e.printStackTrace(); } } } class MyClass implements Serializable { // class definition }
In this example, we create a FileInputStream and an ObjectInputStream to read an object from a file (“data.ser”). We then attempt to cast the deserialized object to a MyClass object. If the file format does not match the class definition, an InvalidClassException is thrown. We catch the exception and handle it by printing a message and the stack trace.
Best Practices for Java File Handling
Related Article: Java Composition Tutorial
Overview
When working with files in Java, it’s important to follow best practices to ensure efficient and reliable file handling. This chapter will discuss some best practices for Java file handling.
Close File Resources
After reading from or writing to a file, it’s essential to close the file resources to release system resources and prevent resource leaks. You can use the close()
method of the respective file handling classes, such as FileReader, FileWriter, FileInputStream, FileOutputStream, etc. Here’s an example:
import java.io.*; public class CloseFileResourcesExample { public static void main(String[] args) { try { FileReader reader = new FileReader("file.txt"); // Perform file operations reader.close(); } catch (IOException e) { System.out.println("An error occurred while reading the file."); e.printStackTrace(); } } }
In this example, we create a FileReader object to read from a file (“file.txt”). After performing the necessary file operations, we call the close()
method to close the file resource.
Use try-with-resources
To ensure that file resources are automatically closed, even in the event of an exception, you can use the try-with-resources statement introduced in Java 7. This statement automatically closes the declared resources at the end of the block. Here’s an example:
import java.io.*; public class TryWithResourcesExample { public static void main(String[] args) { try (FileReader reader = new FileReader("file.txt")) { // Perform file operations } catch (IOException e) { System.out.println("An error occurred while reading the file."); e.printStackTrace(); } } }
In this example, we create a FileReader object within the try-with-resources statement. The FileReader resource is automatically closed at the end of the block, whether an exception occurs or not.
Related Article: Java Hashmap Tutorial
Handle Exceptions Appropriately
When working with files, it’s important to handle exceptions appropriately to provide meaningful error messages and avoid unexpected program termination. You can catch specific exceptions related to file handling operations and handle them accordingly. Here’s an example:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class HandleExceptionsExample { public static void main(String[] args) { try { File file = new File("nonexistent.txt"); Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); System.out.println(line); } scanner.close(); } catch (FileNotFoundException e) { System.out.println("The file was not found."); } catch (Exception e) { System.out.println("An error occurred while reading the file."); e.printStackTrace(); } } }
In this example, we catch the FileNotFoundException specifically to handle the case when the file is not found. We catch the more general Exception to handle any other unexpected exceptions. We provide appropriate error messages based on the type of exception.
Use Buffered I/O for Better Performance
When working with large files or performing frequent read/write operations, it’s recommended to use buffered I/O to improve performance. Buffered I/O reduces the number of physical disk reads and writes by buffering data in memory. Here’s an example:
import java.io.*; public class BufferedIOExample { public static void main(String[] args) { try (BufferedReader reader = new BufferedReader(new FileReader("file.txt")); BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) { String line; while ((line = reader.readLine()) != null) { writer.write(line); writer.newLine(); } } catch (IOException e) { System.out.println("An error occurred while reading or writing the file."); e.printStackTrace(); } } }
In this example, we use BufferedReader and BufferedWriter to read from and write to files, respectively. These classes provide buffering capabilities, which can improve performance when reading or writing large amounts of data.
Handle Large Files with Memory Constraints
When working with large files that may exceed available memory, it’s important to handle them without causing memory constraints. One approach is to process the file in smaller chunks or use streaming APIs to read/write data incrementally. Here’s an example using the java.nio.file package:
import java.nio.file.*; import java.io.IOException; import java.util.stream.Stream; public class HandleLargeFilesExample { public static void main(String[] args) { Path path = Paths.get("largefile.txt"); try (Stream<String> lines = Files.lines(path)) { // Process each line of the file lines.forEach(System.out::println); } catch (IOException e) { System.out.println("An error occurred while processing the file."); e.printStackTrace(); } } }
In this example, we use the Files.lines() method to obtain a stream of lines from a large file (“largefile.txt”). We can then process each line using the stream’s forEach() method. This approach allows us to handle large files without loading the entire file into memory.
Related Article: Popular Data Structures Asked in Java Interviews
Real World Examples of Java File Handling
Overview
Java’s file handling capabilities are widely used in real-world scenarios. This chapter will explore some practical examples of Java file handling in various domains.
Example 1: Log File Analysis
In many applications, log files are used to record important events and information. Java file handling can be leveraged to analyze log files and extract useful insights. Here’s an example that counts the number of occurrences of a specific event in a log file:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class LogFileAnalysisExample { public static void main(String[] args) { String logFile = "application.log"; String eventToCount = "ERROR"; int count = 0; try (BufferedReader reader = new BufferedReader(new FileReader(logFile))) { String line; while ((line = reader.readLine()) != null) { if (line.contains(eventToCount)) { count++; } } } catch (IOException e) { System.out.println("An error occurred while reading the log file."); e.printStackTrace(); } System.out.println("Number of occurrences of " + eventToCount + ": " + count); } }
In this example, we read each line of a log file (“application.log”) and check if it contains a specific event (“ERROR”). We increment a counter whenever the event is found. Finally, we display the count of occurrences of the event.
Related Article: Java List Tutorial
Example 2: File Synchronization
In distributed systems or collaborative environments, file synchronization is a common requirement. Java file handling can be used to synchronize files across multiple locations or devices. Here’s an example that demonstrates file synchronization between a local directory and a remote server:
import java.io.*; import org.apache.commons.io.FileUtils; public class FileSynchronizationExample { public static void main(String[] args) { File localDirectory = new File("local"); File remoteDirectory = new File("remote"); try { FileUtils.copyDirectory(localDirectory, remoteDirectory); System.out.println("Files synchronized successfully."); } catch (IOException e) { System.out.println("An error occurred while synchronizing files."); e.printStackTrace(); } } }
In this example, we use the FileUtils.copyDirectory()
method from the Apache Commons IO library to synchronize a local directory (“local”) with a remote directory (“remote”). The method copies all files and subdirectories from the source directory to the destination directory, effectively synchronizing the files.
Performance Considerations for Java File Handling
Overview
When working with files in Java, it’s important to consider performance implications, especially when dealing with large files or performing frequent file operations. This chapter will discuss some performance considerations for Java file handling.
Related Article: Java Set Tutorial
Use Buffered I/O for Large Files
When handling large files, it’s recommended to use buffered I/O to improve performance. Buffered I/O reduces the number of physical disk reads and writes by buffering data in memory. Here’s an example:
import java.io.*; public class BufferedIOForLargeFilesExample { public static void main(String[] args) { try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream("largefile.txt")); BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("output.txt"))) { byte[] buffer = new byte[8192]; int bytesRead; while ((bytesRead = bis.read(buffer)) != -1) { bos.write(buffer, 0, bytesRead); } } catch (IOException e) { System.out.println("An error occurred while reading or writing the files."); e.printStackTrace(); } } }
In this example, we use BufferedInputStream and BufferedOutputStream to read from and write to files, respectively. We use a buffer size of 8192 bytes (8 KB), but you can adjust the buffer size based on your specific requirements and system capabilities.
Consider File Channel and Memory-Mapped I/O
For certain use cases, using FileChannel and memory-mapped I/O can provide better performance compared to traditional file handling methods. FileChannel allows direct access to the underlying file and supports various operations such as reading, writing, and memory mapping. Here’s an example:
import java.io.*; import java.nio.*; import java.nio.channels.*; public class FileChannelExample { public static void main(String[] args) { try (RandomAccessFile file = new RandomAccessFile("file.txt", "rw"); FileChannel channel = file.getChannel()) { MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_WRITE, 0, channel.size()); buffer.putChar('H'); buffer.putChar('i'); // ... buffer.force(); // Flush changes to the file System.out.println("Data written to the file using FileChannel."); } catch (IOException e) { System.out.println("An error occurred while reading or writing the file."); e.printStackTrace(); } } }
In this example, we use FileChannel to create a memory-mapped buffer that is directly mapped to the file “file.txt”. We can then perform read and write operations on the buffer, which will be reflected in the file. The force()
method is used to flush any changes made to the buffer to the file.
Avoid Redundant File Operations
Performing redundant file operations can significantly impact performance, especially when working with large files or in resource-constrained environments. It’s important to minimize unnecessary file operations and optimize the use of file handling APIs. Consider caching frequently accessed data or using efficient data structures to avoid redundant I/O operations.
Related Article: Java Classloader: How to Load Classes in Java
Parallelize File Operations
When dealing with multiple files or performing independent file operations, parallelizing the operations can improve performance by utilizing multiple threads or processes. You can leverage Java’s concurrency framework, such as the ExecutorService and Fork/Join framework, to parallelize file operations. However, be cautious when parallelizing file operations as concurrent access to the same file may lead to synchronization issues or conflicts.
Consider Asynchronous I/O
For certain scenarios, especially when dealing with non-blocking I/O operations, asynchronous I/O can offer better performance compared to synchronous I/O. Java provides the AsynchronousFileChannel class, which allows you to perform asynchronous read and write operations on files. Asynchronous I/O can be particularly useful when working with network-related file operations or when you need to handle a large number of concurrent file operations efficiently.
Advanced Techniques for Java File Handling
Related Article: Java Do-While Loop Tutorial
Overview
Java’s file handling capabilities offer various advanced techniques for handling files with increased flexibility and efficiency. This chapter will explore some advanced techniques for Java file handling.
Working with File Attributes
Java provides APIs to access and manipulate file attributes, such as file permissions, ownership, and timestamps. The java.nio.file.attribute package contains classes and interfaces for working with file attributes. Here’s an example that demonstrates how to retrieve and modify file attributes:
import java.nio.file.*; import java.io.IOException; import java.nio.file.attribute.*; public class FileAttributesExample { public static void main(String[] args) { Path path = Paths.get("file.txt"); try { BasicFileAttributes attributes = Files.readAttributes(path, BasicFileAttributes.class); System.out.println("Creation Time: " + attributes.creationTime()); System.out.println("Last Modified Time: " + attributes.lastModifiedTime()); System.out.println("Is Directory: " + attributes.isDirectory()); FileOwnerAttributeView ownerAttributeView = Files.getFileAttributeView(path, FileOwnerAttributeView.class); UserPrincipal owner = ownerAttributeView.getOwner(); System.out.println("Owner: " + owner.getName()); PosixFileAttributes posixAttributes = Files.readAttributes(path, PosixFileAttributes.class); System.out.println("Permissions: " + posixAttributes.permissions()); } catch (IOException e) { System.out.println("An error occurred while reading the file attributes."); e.printStackTrace(); } } }
In this example, we use the Files.readAttributes() method to retrieve the BasicFileAttributes and PosixFileAttributes for a file (“file.txt”). We can access various attributes, such as creation time, last modified time, file type, owner, and permissions. Note that the availability of certain attributes may depend on the underlying file system.
Monitoring File System Events
Java provides the WatchService API for monitoring file system events, such as file creation, modification, and deletion. This can be useful for building file synchronization tools, monitoring file changes, or triggering actions based on file system events. Here’s an example that demonstrates how to use WatchService to monitor file system events:
import java.nio.file.*; import java.io.IOException; public class FileSystemEventsExample { public static void main(String[] args) { try { WatchService watchService = FileSystems.getDefault().newWatchService(); Path directory = Paths.get("path/to/directory"); directory.register(watchService, StandardWatchEventKinds.ENTRY_CREATE, StandardWatchEventKinds.ENTRY_MODIFY, StandardWatchEventKinds.ENTRY_DELETE); while (true) { WatchKey key = watchService.take(); for (WatchEvent<?> event : key.pollEvents()) { WatchEvent.Kind<?> kind = event.kind(); if (kind == StandardWatchEventKinds.OVERFLOW) { continue; } Path filePath = (Path) event.context(); System.out.println("Event: " + kind + ", File: " + filePath); } key.reset(); } } catch (IOException | InterruptedException e) { System.out.println("An error occurred while monitoring file system events."); e.printStackTrace(); } } }
In this example, we create a WatchService using FileSystems.getDefault().newWatchService(). We then register the directory to be monitored using the register() method. We specify the types of events to be monitored, such as file creation, modification, and deletion. The program enters an infinite loop and waits for events using the watchService.take() method. When an event occurs, we retrieve the event kind and the corresponding file path.
Related Article: Tutorial: Best Practices for Java Singleton Design Pattern
Working with Symbolic Links
Java provides APIs to work with symbolic links, which are special types of files that act as references to other files or directories. The java.nio.file package contains classes and interfaces for working with symbolic links. Here’s an example that demonstrates how to create a symbolic link:
import java.nio.file.*; import java.io.IOException; public class SymbolicLinkExample { public static void main(String[] args) { Path source = Paths.get("original.txt"); Path target = Paths.get("symbolic.txt"); try { Files.createSymbolicLink(target, source); System.out.println("Symbolic link created successfully."); } catch (IOException e) { System.out.println("An error occurred while creating the symbolic link."); e.printStackTrace(); } } }
In this example, we create a symbolic link named “symbolic.txt” that points to the file “original.txt”. We use the Files.createSymbolicLink() method to create the symbolic link. Note that creating symbolic links requires appropriate operating system permissions.
Working with File Locks
Java provides APIs for file locking, which allows multiple processes or threads to coordinate access to a file. File locks can be used to prevent concurrent modifications to a file or to synchronize access to shared file resources. Here’s an example that demonstrates how to acquire an exclusive file lock:
import java.io.*; import java.nio.channels.*; public class FileLockExample { public static void main(String[] args) { try (RandomAccessFile file = new RandomAccessFile("file.txt", "rw"); FileChannel channel = file.getChannel()) { FileLock lock = channel.lock(); // Perform operations on the locked file lock.release(); // Release the lock System.out.println("File lock released."); } catch (IOException e) { System.out.println("An error occurred while acquiring or releasing the lock."); e.printStackTrace(); } } }
In this example, we create a RandomAccessFile and obtain its FileChannel. We then use the lock()
method of the FileChannel to acquire an exclusive lock on the file. We can perform operations on the locked file within the locked region. Finally, we release the lock using the release()
method.
Code Snippet Ideas for Java File Handling (1 of 5)
Related Article: How to Use Public Static Void Main String Args in Java
Overview
This chapter provides code snippet ideas for Java file handling. Each code snippet focuses on a specific aspect of file handling and demonstrates how to perform various file operations efficiently.
Code Snippet 1: Read Text File Line by Line
import java.io.*; public class ReadTextFileLineByLineExample { public static void main(String[] args) { try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.out.println("An error occurred while reading the file."); e.printStackTrace(); } } }
In this code snippet, we use a BufferedReader to efficiently read a text file line by line. We read each line using the readLine()
method and print it to the console. The try-with-resources statement ensures that the file resource is automatically closed after reading.
Code Snippet 2: Write Text File Line by Line
import java.io.*; public class WriteTextFileLineByLineExample { public static void main(String[] args) { try (BufferedWriter writer = new BufferedWriter(new FileWriter("file.txt"))) { writer.write("Line 1"); writer.newLine(); writer.write("Line 2"); writer.newLine(); writer.write("Line 3"); } catch (IOException e) { System.out.println("An error occurred while writing to the file."); e.printStackTrace(); } } }
In this code snippet, we use a BufferedWriter to efficiently write lines of text to a file. We use the write()
method to write each line and the newLine()
method to insert a newline character after each line. The try-with-resources statement ensures that the file resource is automatically closed after writing.
Related Article: Java Printf Method Tutorial
Code Snippet 3: Read Binary File
import java.io.*; public class ReadBinaryFileExample { public static void main(String[] args) { try (FileInputStream fis = new FileInputStream("file.bin")) { byte[] buffer = new byte[8192]; int bytesRead; while ((bytesRead = fis.read(buffer)) != -1) { // Process the byte data } } catch (IOException e) { System.out.println("An error occurred while reading the file."); e.printStackTrace(); } } }
In this code snippet, we use a FileInputStream to efficiently read a binary file. We read the file in chunks using a byte array buffer. The read()
method returns the number of bytes read, which allows us to process the data efficiently. The try-with-resources statement ensures that the file resource is automatically closed after reading.
Code Snippet 4: Write Binary File
import java.io.*; public class WriteBinaryFileExample { public static void main(String[] args) { try (FileOutputStream fos = new FileOutputStream("file.bin")) { byte[] data = {0x48, 0x65, 0x6C, 0x6C, 0x6F}; fos.write(data); } catch (IOException e) { System.out.println("An error occurred while writing to the file."); e.printStackTrace(); } } }
In this code snippet, we use a FileOutputStream to efficiently write binary data to a file. We create a byte array containing the data we want to write and use the write()
method to write the data to the file. The try-with-resources statement ensures that the file resource is automatically closed after writing.
Code Snippet 5: Copy File
import java.io.*; public class CopyFileExample { public static void main(String[] args) { try (FileInputStream fis = new FileInputStream("source.txt"); FileOutputStream fos = new FileOutputStream("destination.txt")) { byte[] buffer = new byte[8192]; int bytesRead; while ((bytesRead = fis.read(buffer)) != -1) { fos.write(buffer, 0, bytesRead); } } catch (IOException e) { System.out.println("An error occurred while copying the file."); e.printStackTrace(); } } }
In this code snippet, we use a FileInputStream to read data from a source file and a FileOutputStream to write the data to a destination file. We read the data in chunks using a byte array buffer and write the data to the destination file using the write()
method. The try-with-resources statement ensures that the file resources are automatically closed after reading and writing.