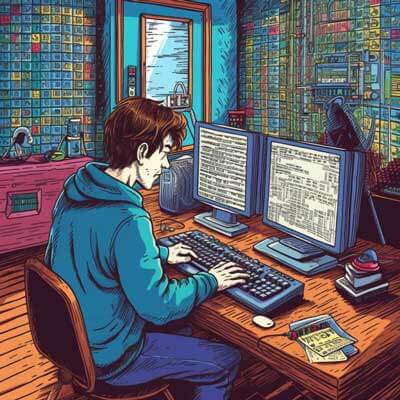
- Introduction to Class Loaders
- Code Snippet: Creating a Custom Class Loader
- Code Snippet: Loading a Class using a Class Loader
- Class Loader Hierarchy
- Code Snippet: Inspecting Class Loader Hierarchy
- Types of Class Loaders
- Code Snippet: Finding Loaded Classes
- Code Snippet: Unloading a Class
- Working Mechanism of Class Loaders
- Code Snippet: Inspecting Class Files
- How to Use Class Loaders
- Code Snippet: Dynamic Class Loading
- Code Snippet: Reloading Classes at Runtime
- Use Case: Isolating Application Modules
- Code Snippet: Creating Module Class Loaders
- Best Practice: Avoiding ClassLoader Leaks
- Code Snippet: Avoiding ClassLoader Leaks
- Best Practice: Ensuring Class Unloading
- Code Snippet: Ensuring Class Unloading
- Real World Example: Custom Class Loader for a Plugin System
- Code Snippet: Custom Class Loader for a Plugin System
- Real World Example: Class Loading in Application Servers
- Performance Consideration: Class Loading Overhead
- Performance Consideration: Impact on Startup Time
- Advanced Technique: Implementing a Network Class Loader
- Advanced Technique: Overriding Class Loading Behavior
- Code Snippet: Overriding Class Loading Behavior
- Error Handling: Dealing with ClassNotFoundException
- Error Handling: Resolving NoClassDefFoundError
- Error Handling: Troubleshooting LinkageError
Introduction to Class Loaders
In Java, a Class Loader is responsible for loading Java classes into the Java Virtual Machine (JVM) at runtime. It is an integral part of the Java Runtime Environment (JRE) and plays a crucial role in the dynamic nature of Java applications. The Class Loader reads the bytecode of a class file and creates an instance of the java.lang.Class class, which represents the loaded class.
To understand the importance of Class Loaders, let’s consider a scenario where an application needs to load a class dynamically based on certain conditions. Without a Class Loader, the application would need to include all possible classes at compile-time, resulting in a bloated and inflexible application. With a Class Loader, the application can load classes dynamically at runtime, enabling greater flexibility and modularity.
Related Article: How To Parse JSON In Java
Code Snippet: Creating a Custom Class Loader
public class CustomClassLoader extends ClassLoader { @Override public Class<?> findClass(String name) throws ClassNotFoundException { // Implement custom logic to find and load the class bytecode byte[] bytecode = loadClassBytecode(name); return defineClass(name, bytecode, 0, bytecode.length); } }
Code Snippet: Loading a Class using a Class Loader
public class Main { public static void main(String[] args) throws ClassNotFoundException { // Create an instance of the custom class loader ClassLoader classLoader = new CustomClassLoader(); // Load the class dynamically Class<?> loadedClass = classLoader.loadClass("com.example.MyClass"); // Create an instance of the loaded class Object instance = loadedClass.newInstance(); } }
Class Loader Hierarchy
In Java, Class Loaders are organized in a hierarchical structure known as the Class Loader Hierarchy. The hierarchy consists of multiple Class Loaders, each responsible for loading classes from a specific source or location.
At the top of the hierarchy is the Bootstrap Class Loader, which is responsible for loading essential Java classes from the bootstrap classpath. It is implemented in native code and is not represented by a specific Java class.
Below the Bootstrap Class Loader, there are several other Class Loaders, such as the Extension Class Loader and the System Class Loader. The Extension Class Loader loads classes from the extension classpath, while the System Class Loader loads classes from the application classpath.
Related Article: How To Convert Array To List In Java
Code Snippet: Inspecting Class Loader Hierarchy
public class Main { public static void main(String[] args) { // Get the Class Loader of the current class ClassLoader classLoader = Main.class.getClassLoader(); // Traverse the Class Loader hierarchy while (classLoader != null) { System.out.println(classLoader); classLoader = classLoader.getParent(); } } }
Types of Class Loaders
In Java, there are different types of Class Loaders, each designed to load classes from specific sources or locations. Understanding the types of Class Loaders can help in designing modular and extensible applications.
1. Bootstrap Class Loader: The Bootstrap Class Loader is responsible for loading essential Java classes from the bootstrap classpath. It is implemented in native code and is not represented by a specific Java class.
2. Extension Class Loader: The Extension Class Loader loads classes from the extension classpath. It is a child of the Bootstrap Class Loader and is implemented by the sun.misc.Launcher$ExtClassLoader class.
3. System Class Loader: The System Class Loader, also known as the Application Class Loader, loads classes from the application classpath. It is a child of the Extension Class Loader and is implemented by the sun.misc.Launcher$AppClassLoader class.
4. Custom Class Loaders: Custom Class Loaders can be created to load classes from custom sources or locations. By extending the java.lang.ClassLoader class, developers can implement their own logic for loading classes. Custom Class Loaders are useful in scenarios where classes need to be loaded dynamically or from non-standard locations.
Code Snippet: Finding Loaded Classes
public class Main { public static void main(String[] args) { // Get the Class Loader of the current class ClassLoader classLoader = Main.class.getClassLoader(); // Find loaded classes in the current Class Loader Class<?>[] loadedClasses = classLoader.getLoadedClasses(); // Print the names of the loaded classes for (Class<?> loadedClass : loadedClasses) { System.out.println(loadedClass.getName()); } } }
Related Article: How To Iterate Over Entries In A Java Map
Code Snippet: Unloading a Class
public class MyClass { // Class implementation } public class Main { public static void main(String[] args) throws Exception { // Create an instance of the custom class loader ClassLoader classLoader = new CustomClassLoader(); // Load the class dynamically Class<?> loadedClass = classLoader.loadClass("com.example.MyClass"); // Create an instance of the loaded class Object instance = loadedClass.newInstance(); // Unload the class ((CustomClassLoader) classLoader).unloadClass(loadedClass); } } public class CustomClassLoader extends ClassLoader { // Other methods and implementation public void unloadClass(Class<?> loadedClass) throws Exception { // Perform cleanup or release any resources associated with the class // Note: Unloading a class is not directly supported in Java and requires careful handling // This is just a simplified example and may not work in all scenarios } }
Working Mechanism of Class Loaders
The working mechanism of Class Loaders involves a series of steps to locate, load, and define classes within the JVM. When a class is requested, the Class Loader follows a set of rules to locate and load the class bytecode.
1. Name Resolution: The Class Loader receives the name of the class to be loaded and resolves it to a binary name using the Java Naming and Directory Interface (JNDI).
2. Classpath Search: The Class Loader searches for the class bytecode in a specific classpath or set of locations. The search order is typically determined by the Class Loader implementation and can include directories, JAR files, or other resources.
3. Bytecode Loading: Once the class bytecode is found, the Class Loader reads the bytecode and creates an instance of the java.lang.Class class, which represents the loaded class. The Class object contains information about the class, such as its methods, fields, and annotations.
4. Class Definition: The Class Loader defines the loaded class within the JVM by creating a java.lang.Class object. This object is then used by the JVM to instantiate objects, invoke methods, and perform other operations related to the loaded class.
Code Snippet: Inspecting Class Files
public class Main { public static void main(String[] args) throws IOException { // Get the Class Loader of the current class ClassLoader classLoader = Main.class.getClassLoader(); // Get the URL of the class file URL classUrl = classLoader.getResource("com/example/MyClass.class"); // Read the class file bytecode byte[] bytecode = Files.readAllBytes(Paths.get(classUrl.toURI())); // Print the bytecode as a hexadecimal string for (byte b : bytecode) { System.out.printf("%02X ", b); } } }
Related Article: How To Split A String In Java
How to Use Class Loaders
When working with Class Loaders, it is important to understand how to use them effectively to achieve specific goals. Here are some common use cases for Class Loaders:
1. Dynamic Class Loading: Class Loaders can be used to load classes dynamically at runtime, enabling greater flexibility and modularity in an application. This can be useful in scenarios where the set of classes to be loaded is determined dynamically, such as plugins or modules.
2. Reloading Classes at Runtime: Class Loaders can be used to reload classes at runtime, allowing for hot-reloading of code without restarting the application. This can be useful during development or in situations where code changes need to be applied without interrupting the application’s operation.
3. Isolating Application Modules: Class Loaders can be used to create isolated modules within an application, where each module has its own set of classes and resources. This can be useful in large applications with multiple components or in situations where different parts of the application need to use different versions of the same library.
Code Snippet: Dynamic Class Loading
public class PluginManager { private List<Class<?>> loadedClasses = new ArrayList<>(); public void loadPlugin(String pluginClassName) throws ClassNotFoundException { // Create an instance of the custom class loader ClassLoader classLoader = new CustomClassLoader(); // Load the plugin class dynamically Class<?> pluginClass = classLoader.loadClass(pluginClassName); // Add the loaded class to the list loadedClasses.add(pluginClass); } public void invokePlugins() { for (Class<?> pluginClass : loadedClasses) { try { // Create an instance of the plugin class Object pluginInstance = pluginClass.newInstance(); // Invoke the plugin's methods // ... } catch (InstantiationException | IllegalAccessException e) { // Handle exceptions } } } }
Code Snippet: Reloading Classes at Runtime
public class Reloader { private ClassLoader classLoader; public Reloader() { // Create an instance of the custom class loader classLoader = new CustomClassLoader(); } public void reloadClass(String className) throws ClassNotFoundException, InstantiationException, IllegalAccessException { // Load the class dynamically using the class loader Class<?> reloadedClass = classLoader.loadClass(className); // Create an instance of the reloaded class Object instance = reloadedClass.newInstance(); // Perform any necessary operations with the reloaded class // ... } }
Related Article: How To Convert Java Objects To JSON With Jackson
Use Case: Isolating Application Modules
In large applications, isolating modules can help manage complexity and ensure that different parts of the application remain independent. Class Loaders can be used to achieve module isolation by creating separate Class Loaders for each module.
By using separate Class Loaders, each module can have its own classpath, allowing it to load and use its own set of classes and resources. This prevents conflicts between modules and allows for different versions of the same library to be used in different modules.
The isolation of application modules can be particularly useful in scenarios where the application needs to support different versions of a library or when different modules have conflicting dependencies.
Code Snippet: Creating Module Class Loaders
public class ModuleManager { private Map<String, ClassLoader> moduleLoaders = new HashMap<>(); public void loadModule(String moduleName, List<String> classpath) { // Create a new class loader for the module ClassLoader moduleLoader = new URLClassLoader(classpath.toArray(new String[0])); // Store the module loader moduleLoaders.put(moduleName, moduleLoader); } public ClassLoader getModuleClassLoader(String moduleName) { // Get the class loader for the specified module return moduleLoaders.get(moduleName); } }
Best Practice: Avoiding ClassLoader Leaks
ClassLoader leaks occur when a Class Loader is not garbage collected due to references to loaded classes or resources. This can lead to memory leaks and other performance issues in long-running applications.
To avoid ClassLoader leaks, it is important to follow these best practices:
1. Avoid static references to classes or resources loaded by a Class Loader. Static references prevent the garbage collector from collecting the Class Loader and its associated classes.
2. Ensure that all classes and resources loaded by a Class Loader are released when they are no longer needed. This can be done by explicitly nullifying references or using weak references.
3. Avoid using custom Class Loaders unnecessarily. Custom Class Loaders should only be used when dynamic loading or isolation is required. Using custom Class Loaders unnecessarily can complicate the application and increase the risk of leaks.
Related Article: Storing Contact Information in Java Data Structures
Code Snippet: Avoiding ClassLoader Leaks
public class MyClass { private static final Set<ClassLoader> loadedClassLoaders = new HashSet<>(); public static void loadClass(String className) throws ClassNotFoundException { // Create an instance of the custom class loader ClassLoader classLoader = new CustomClassLoader(); // Load the class dynamically Class<?> loadedClass = classLoader.loadClass(className); // Add the class loader to the set loadedClassLoaders.add(classLoader); // ... } public static void unloadClassLoaders() { Iterator<ClassLoader> iterator = loadedClassLoaders.iterator(); while (iterator.hasNext()) { ClassLoader classLoader = iterator.next(); iterator.remove(); // Perform cleanup or release any resources associated with the class loader // ... } } }
Best Practice: Ensuring Class Unloading
In Java, classes are typically loaded into the JVM and remain in memory until the JVM shuts down. However, in certain situations, it may be necessary to unload classes from memory to free up resources or to allow for dynamic reloading of code.
To ensure class unloading, it is important to follow these best practices:
1. Avoid creating strong references to classes or objects loaded by a Class Loader. Strong references prevent the garbage collector from collecting the associated class and its resources.
2. Release all references to classes or objects loaded by a Class Loader when they are no longer needed. This can be done by setting references to null or using weak references.
3. Use custom Class Loaders with caution, as unloading classes is not directly supported in Java and requires careful handling. Implementing custom logic for unloading classes can help ensure that resources are released properly.
Code Snippet: Ensuring Class Unloading
public class MyClass { private static final Map<String, WeakReference<Class<?>>> loadedClasses = new HashMap<>(); public static void loadClass(String className) throws ClassNotFoundException { // Create an instance of the custom class loader ClassLoader classLoader = new CustomClassLoader(); // Load the class dynamically Class<?> loadedClass = classLoader.loadClass(className); // Add the loaded class to the map with a weak reference loadedClasses.put(className, new WeakReference<>(loadedClass)); // ... } public static void unloadClass(String className) { WeakReference<Class<?>> classReference = loadedClasses.get(className); if (classReference != null) { Class<?> loadedClass = classReference.get(); if (loadedClass != null) { // Perform cleanup or release any resources associated with the class // ... // Remove the class from the map loadedClasses.remove(className); } } } }
Related Article: How to Convert JSON String to Java Object
Real World Example: Custom Class Loader for a Plugin System
Many applications allow for extensibility through plugins or modules. A common approach to implementing a plugin system is to use a custom Class Loader that can dynamically load and manage plugins.
A custom Class Loader for a plugin system typically follows these steps:
1. Specify a classpath or set of locations where plugins can be found.
2. Implement a mechanism to discover and load plugins dynamically.
3. Create a separate instance of the custom Class Loader for each plugin to ensure isolation and prevent conflicts between plugins.
4. Provide methods to manage and interact with the loaded plugins.
Code Snippet: Custom Class Loader for a Plugin System
public class PluginManager { private Map<String, Plugin> loadedPlugins = new HashMap<>(); public void loadPlugins(String pluginDirectory) throws IOException { // Get the list of plugin JAR files from the specified directory List<File> pluginFiles = getPluginFiles(pluginDirectory); for (File pluginFile : pluginFiles) { // Create a separate class loader for each plugin ClassLoader pluginClassLoader = new URLClassLoader(new URL[]{pluginFile.toURI().toURL()}); // Load the plugin class dynamically Class<?> pluginClass = pluginClassLoader.loadClass("com.example.Plugin"); // Create an instance of the plugin Plugin plugin = (Plugin) pluginClass.newInstance(); // Initialize the plugin plugin.init(); // Add the loaded plugin to the map loadedPlugins.put(plugin.getName(), plugin); } } public Plugin getPlugin(String name) { return loadedPlugins.get(name); } }
Real World Example: Class Loading in Application Servers
Application servers, such as Apache Tomcat or JBoss, use Class Loaders extensively to manage the loading and execution of web applications. Class loading in application servers follows a specific hierarchy and set of rules to ensure the isolation and proper functioning of multiple web applications.
In an application server environment, each web application is deployed as a separate entity with its own classpath and resources. The Class Loader hierarchy is typically organized to allow for the sharing of common libraries and resources while ensuring the isolation of each web application.
Understanding class loading in application servers is essential for developing and deploying Java web applications effectively.
Related Article: How to Retrieve Current Date and Time in Java
Performance Consideration: Class Loading Overhead
Class loading in Java incurs a certain level of overhead due to the runtime resolution and verification of classes. This overhead can impact the performance of an application, especially in scenarios where a large number of classes need to be loaded.
To minimize the performance impact of class loading, consider the following:
1. Optimize the classpath: Minimize the number of directories or JAR files in the classpath. Having a large classpath can increase the time required for class loading.
2. Use class preloading: Preload frequently used classes during application startup to reduce the time required for class loading during runtime.
3. Utilize class caching: Cache loaded classes to avoid redundant class loading. This can be particularly useful in scenarios where classes are loaded multiple times.
4. Use shared libraries: Utilize shared libraries or frameworks to reduce the number of classes that need to be loaded. This can help reduce the overall class loading overhead.
Performance Consideration: Impact on Startup Time
Class loading is a significant factor in the overall startup time of a Java application. The time required to load and initialize classes can impact the perceived performance and user experience of an application.
To minimize the impact of class loading on startup time, consider the following:
1. Optimize the classpath: Ensure that the classpath includes only the necessary classes and resources. Removing unnecessary entries from the classpath can reduce the time required for class loading.
2. Utilize lazy loading: Delay the loading of non-essential classes until they are actually needed. This can be done using techniques like lazy initialization or dynamic class loading.
3. Optimize class loading order: Load classes in an order that minimizes dependencies and maximizes parallelism. Loading independent classes in parallel can help reduce the overall startup time.
4. Utilize class caching: Cache loaded classes to avoid redundant class loading during application startup. This can be particularly useful in scenarios where classes are loaded multiple times.
Advanced Technique: Implementing a Network Class Loader
In some scenarios, it may be necessary to load classes from a remote location, such as a network server or a distributed file system. This can be achieved by implementing a custom Network Class Loader that can fetch class bytecode from the remote location and load it into the JVM.
Implementing a Network Class Loader involves the following steps:
1. Establish a connection to the remote location where the classes are stored.
2. Fetch the bytecode of the requested class from the remote location.
3. Define the class within the JVM using the fetched bytecode.
4. Handle any errors or exceptions that may occur during the network class loading process.
Related Article: How to Reverse a String in Java
Advanced Technique: Overriding Class Loading Behavior
In certain scenarios, it may be necessary to override the default behavior of the Class Loader to achieve specific requirements. This can be done by implementing a custom Class Loader and overriding its methods to provide the desired behavior.
Some common scenarios where overriding class loading behavior may be required include:
1. Loading classes from non-standard locations, such as a database or a remote server.
2. Modifying the bytecode of classes before they are defined within the JVM.
3. Implementing custom class resolution or resource loading logic.
When overriding class loading behavior, it is important to carefully consider the implications and potential risks associated with modifying the default behavior of the Class Loader.
Code Snippet: Overriding Class Loading Behavior
public class CustomClassLoader extends ClassLoader { @Override protected Class<?> findClass(String name) throws ClassNotFoundException { // Implement custom logic to find and load the class bytecode byte[] bytecode = loadClassBytecode(name); return defineClass(name, bytecode, 0, bytecode.length); } @Override public URL getResource(String name) { // Implement custom resource loading logic return findResource(name); } }
Error Handling: Dealing with ClassNotFoundException
The ClassNotFoundException is thrown when the Class Loader is unable to find and load the requested class. This can occur if the class is not in the classpath or if the class is not accessible by the Class Loader.
To handle ClassNotFoundException, consider the following:
1. Check the classpath: Ensure that the class is present in the classpath. If the class is missing, add the necessary JAR file or directory to the classpath.
2. Verify class accessibility: Ensure that the class is accessible by the Class Loader. If the class is in a different package or module, check if the necessary access permissions are granted.
3. Handle the exception gracefully: Catch the ClassNotFoundException and handle it appropriately. This may involve logging an error message, displaying a user-friendly error, or taking corrective actions.
Related Article: How to Generate Random Integers in a Range in Java
Error Handling: Resolving NoClassDefFoundError
The NoClassDefFoundError is thrown when the JVM cannot find the definition of a class that was previously available at compile-time. This can occur if a class that is referenced by another class is no longer available in the classpath.
To resolve NoClassDefFoundError, consider the following:
1. Check the classpath: Ensure that the class that is missing at runtime is present in the classpath. If the class is missing, add the necessary JAR file or directory to the classpath.
2. Verify class dependencies: Check if the class has any dependencies on other classes or libraries. If the missing class depends on another class, ensure that the required class is also available in the classpath.
3. Handle the error gracefully: Catch the NoClassDefFoundError and handle it appropriately. This may involve logging an error message, displaying a user-friendly error, or taking corrective actions.
Error Handling: Troubleshooting LinkageError
LinkageError is a generic superclass for errors that occur during the linking phase of class loading. It indicates that there is a problem with the consistency or compatibility of classes or interfaces being linked.
To troubleshoot LinkageError, consider the following:
1. Check class dependencies: Ensure that all classes and interfaces being linked are compatible with each other. If there are any incompatible classes or interfaces, fix the compatibility issues or update the dependencies.
2. Verify class versions: Check if the versions of the classes being linked are compatible with the JVM. If the classes were compiled with a different version of Java, update the classes or update the JVM to a compatible version.
3. Analyze error messages: Examine the error messages provided by the JVM to understand the specific cause of the LinkageError. This can provide valuable information for diagnosing and resolving the problem.
4. Handle the error gracefully: Catch the LinkageError and handle it appropriately. This may involve logging an error message, displaying a user-friendly error, or taking corrective actions.