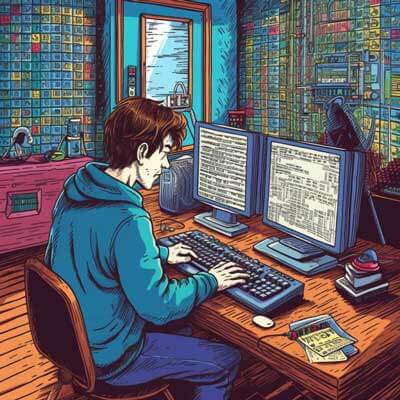
Iterating over entries in a Java Map allows you to access and process each key-value pair in the map. This can be useful in various scenarios, such as performing operations on the values, filtering the entries based on certain conditions, or transforming the entries into a different data structure. In this guide, we will explore different approaches to iterate over entries in a Java Map.
1. Using the entrySet() method
One common way to iterate over entries in a Java Map is by using the entrySet() method. The entrySet() method returns a Set view of the map, where each element is a Map.Entry object representing a key-value pair. You can then iterate over this set to access each entry.
Here’s an example that demonstrates how to iterate over entries using the entrySet() method:
Map map = new HashMap(); map.put("A", 1); map.put("B", 2); map.put("C", 3); for (Map.Entry entry : map.entrySet()) { String key = entry.getKey(); Integer value = entry.getValue(); System.out.println("Key: " + key + ", Value: " + value); }
In this example, we create a HashMap and populate it with some key-value pairs. We then use a for-each loop to iterate over the entry set of the map. For each entry, we retrieve the key and value using the getKey() and getValue() methods of the Map.Entry object. Finally, we print the key-value pair.
Related Article: How To Parse JSON In Java
2. Using the keySet() method
Another approach to iterate over entries in a Java Map is by using the keySet() method. The keySet() method returns a Set view of the keys in the map. You can then iterate over this set and retrieve the corresponding values from the map.
Here’s an example that demonstrates how to iterate over entries using the keySet() method:
Map map = new HashMap(); map.put("A", 1); map.put("B", 2); map.put("C", 3); for (String key : map.keySet()) { Integer value = map.get(key); System.out.println("Key: " + key + ", Value: " + value); }
In this example, we create a HashMap and populate it with some key-value pairs. We then use a for-each loop to iterate over the key set of the map. For each key, we retrieve the corresponding value using the get() method of the map. Finally, we print the key-value pair.
Why is this question asked?
The question of how to iterate over entries in a Java Map is commonly asked by Java developers who need to process or manipulate the data stored in a map. Understanding the various approaches to iterate over entries is essential for working with maps effectively and efficiently. By knowing how to iterate over entries, developers can perform operations on the values, filter the entries based on certain conditions, or transform the entries into a different data structure.
Potential reasons for iterating over entries in a Java Map
There are several potential reasons why you might need to iterate over entries in a Java Map:
– Processing the values: Iterating over entries allows you to access and process the values stored in the map. For example, you might need to perform calculations on the values or apply certain operations to them.
– Filtering entries: You may want to filter the entries based on certain conditions. For example, you might want to select only the entries with values greater than a specific threshold or entries that meet certain criteria.
– Transforming entries: Iterating over entries also allows you to transform the entries into a different data structure. For example, you might want to convert the map into a list of key-value pairs or extract specific information from the entries.
Related Article: How To Convert Array To List In Java
Suggestions and alternative ideas
While iterating over entries using the entrySet() or keySet() methods is a common and straightforward approach, there are alternative ideas and suggestions to consider:
– Using Java 8 streams: If you are using Java 8 or later, you can leverage the Stream API to perform operations on the entries of a map. For example, you can use the stream() method on the entrySet() or keySet() to obtain a stream of entries, and then use various stream operations to filter, map, or reduce the entries.
– Using forEach() method: If you are using Java 8 or later, you can also use the forEach() method introduced in the Map interface. This method allows you to iterate over the entries and apply a specified action to each entry.
Here’s an example that demonstrates how to use the forEach() method:
Map map = new HashMap(); map.put("A", 1); map.put("B", 2); map.put("C", 3); map.forEach((key, value) -> { System.out.println("Key: " + key + ", Value: " + value); });
In this example, we use the forEach() method on the map and provide a lambda expression that specifies the action to be performed on each entry. The lambda expression takes two parameters, key and value, and prints them.
It’s worth noting that the forEach() method is a default method in the Map interface, so it is available for all classes implementing the Map interface, such as HashMap, LinkedHashMap, and TreeMap.
Best practices for iterating over entries in a Java Map
When iterating over entries in a Java Map, it’s important to keep in mind some best practices to ensure efficient and correct code:
– Prefer the entrySet() method: If you need to access both the keys and values of the entries, it is generally more efficient to use the entrySet() method, as it avoids the extra lookup for values. Iterating over the entry set allows you to directly access the key and value of each entry without the need for additional lookups.
– Be mindful of the map implementation: The performance characteristics of iterating over entries may vary depending on the specific implementation of the Map interface. For example, HashMap provides constant-time performance for basic operations, while TreeMap provides logarithmic-time performance. Consider the specific requirements of your use case and choose the appropriate map implementation accordingly.
– Use the right data structure for the task: If you frequently need to iterate over entries or perform operations on the values, consider using a data structure specifically designed for these tasks, such as LinkedHashMap or TreeMap. These data structures provide additional guarantees or ordering that can be beneficial for certain use cases.
– Be cautious with concurrent maps: If you are working with a concurrent map implementation, such as ConcurrentHashMap, be aware of the concurrency implications when iterating over entries. Concurrent maps may allow concurrent modifications, and iterating over entries may not provide a consistent snapshot of the map’s state. Consider using appropriate synchronization mechanisms or concurrent iterators to ensure thread safety.
Overall, iterating over entries in a Java Map is a fundamental operation for working with map data structures. By understanding the different approaches, potential reasons, and best practices for iterating over entries, you can effectively process and manipulate the data stored in a map.