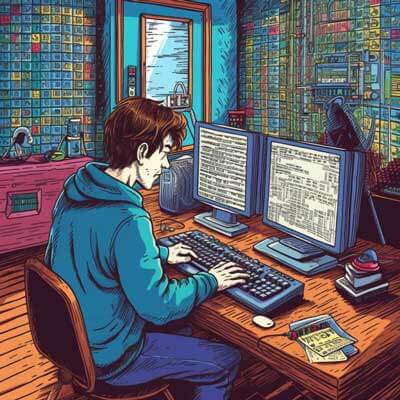
- Introduction to String Arrays
- Example: Creating a String Array
- Example: Modifying String Array Elements
- Defining and Declaring String Arrays
- Example: Declaring a String Array
- Example: Declaring and Initializing a String Array
- Adding Elements to String Arrays
- Example: Adding Elements to a String Array
- Example: Adding Elements to a String Array using a Loop
- Chapter 4: Accessing String Array Elements
- Example: Accessing String Array Elements
- Modifying String Array Elements
- Example: Modifying String Array Elements
- Removing String Array Elements
- Example: Removing String Array Elements
- Iterating Over String Arrays
- Example: Iterating Over a String Array using a for Loop
- Example: Iterating Over a String Array using a foreach Loop
- String Array Use Case: Data Validation
- Example: Data Validation using a String Array
- String Array Use Case: Text Processing
- Example: Splitting a String into an Array of Words
- Example: Extracting Specific Parts of a String using a Delimiter
- String Array Best Practice: Avoid Null Elements
- Example: Avoiding Null Elements in a String Array
- String Array Best Practice: Using the Length Property
- Example: Using the Length Property of a String Array
- Real World Example: String Arrays in File Handling
- Example: Reading Data from a File into a String Array
- Real World Example: String Arrays in User Input Handling
- Example: Handling User Input using a String Array
- Performance Consideration: Memory Usage of String Arrays
- Performance Consideration: Speed of Operations on String Arrays
- Advanced Technique: Nested String Arrays
- Example: Nested String Arrays
- Advanced Technique: Multidimensional String Arrays
- Example: Multidimensional String Arrays
- Code Snippet Idea: Sorting String Arrays
- Example: Sorting a String Array
- Code Snippet Idea: Searching in String Arrays
- Example: Linear Search in a String Array
- Code Snippet Idea: Converting a String to String Array
- Example: Converting a String to a String Array
- Code Snippet Idea: Converting a String Array to List
- Example: Converting a String Array to a List
- Code Snippet Idea: Comparing String Arrays
- Example: Comparing String Arrays
- Error Handling: NullPointer Exception with String Arrays
- Example: Handling NullPointer Exception with String Arrays
- Error Handling: ArrayIndexOutOfBoundsException with String Arrays
- Example: Handling ArrayIndexOutOfBoundsException with String Arrays
Introduction to String Arrays
A string array in Java is an array that stores multiple strings. It allows you to group related strings together and perform various operations on them. String arrays are useful when you need to work with a collection of strings, such as storing names, addresses, or any other text-based data.
To declare a string array, you specify the type of the array (String) followed by the name of the array and square brackets ([]). For example:
String[] names;
This declares a string array variable named “names”. However, at this point, the array is not yet initialized and does not contain any elements.
To initialize a string array and assign values to its elements, you can use the following syntax:
String[] names = {"John", "Jane", "Alice"};
In this example, the string array “names” is initialized with three elements: “John”, “Jane”, and “Alice”. The number of elements in the array is determined by the number of values specified within the curly braces.
Related Article: How To Parse JSON In Java
Example: Creating a String Array
Here’s an example that demonstrates how to create and initialize a string array:
String[] fruits = {"Apple", "Banana", "Orange"}; // Accessing elements of the string array System.out.println(fruits[0]); // Output: Apple System.out.println(fruits[1]); // Output: Banana System.out.println(fruits[2]); // Output: Orange
In this example, we declare a string array “fruits” and initialize it with three fruit names. We then access and print the elements of the array using their indices (0, 1, 2).
Example: Modifying String Array Elements
You can modify the elements of a string array by assigning new values to individual elements. Here’s an example:
String[] colors = {"Red", "Green", "Blue"}; // Modifying an element of the string array colors[1] = "Yellow"; // Accessing and printing modified element System.out.println(colors[1]); // Output: Yellow
In this example, we modify the second element of the “colors” array by assigning it a new value (“Yellow”). We then access and print the modified element to verify the change.
Defining and Declaring String Arrays
To define and declare a string array, you need to specify the type of the array (String) followed by the name of the array and square brackets ([]). You can declare a string array without initializing it, or you can declare and initialize it in the same statement.
Related Article: How To Convert Array To List In Java
Example: Declaring a String Array
To declare a string array without initializing it, you can use the following syntax:
String[] names;
In this example, we declare a string array variable named “names” without assigning any values to it. The array is not yet initialized and does not contain any elements.
Example: Declaring and Initializing a String Array
To declare and initialize a string array in the same statement, you can use the following syntax:
String[] names = {"John", "Jane", "Alice"};
In this example, we declare a string array variable named “names” and initialize it with three elements: “John”, “Jane”, and “Alice”. The number of elements in the array is determined by the number of values specified within the curly braces.
Adding Elements to String Arrays
To add elements to a string array, you need to assign values to individual elements or use a loop to assign values to multiple elements.
Related Article: How To Iterate Over Entries In A Java Map
Example: Adding Elements to a String Array
To add elements to a string array by assigning values to individual elements, you can use the following syntax:
String[] fruits = new String[3]; fruits[0] = "Apple"; fruits[1] = "Banana"; fruits[2] = "Orange";
In this example, we declare a string array “fruits” with a size of three elements. We then assign values to each element using the assignment operator (=).
Example: Adding Elements to a String Array using a Loop
To add elements to a string array using a loop, you can use the following syntax:
String[] weekdays = new String[7]; String[] daysOfWeek = {"Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"}; for (int i = 0; i < weekdays.length; i++) { weekdays[i] = daysOfWeek[i]; }
In this example, we declare a string array “weekdays” with a size of seven elements. We also have another string array “daysOfWeek” that contains the names of the days of the week. We then use a for loop to assign the values from “daysOfWeek” to “weekdays” element by element.
Chapter 4: Accessing String Array Elements
To access individual elements of a string array, you use the index number of the element within square brackets ([]).
Related Article: How To Split A String In Java
Example: Accessing String Array Elements
To access and print the elements of a string array, you can use the following syntax:
String[] fruits = {"Apple", "Banana", "Orange"}; System.out.println(fruits[0]); // Output: Apple System.out.println(fruits[1]); // Output: Banana System.out.println(fruits[2]); // Output: Orange
In this example, we declare a string array “fruits” with three elements. We then access and print each element using its index number (0, 1, 2).
Modifying String Array Elements
You can modify the elements of a string array by assigning new values to individual elements.
Example: Modifying String Array Elements
To modify an element of a string array, you can use the following syntax:
String[] colors = {"Red", "Green", "Blue"}; colors[1] = "Yellow"; System.out.println(colors[1]); // Output: Yellow
In this example, we declare a string array “colors” with three elements. We then modify the second element of the array by assigning it a new value (“Yellow”). Finally, we access and print the modified element to verify the change.
Related Article: How To Convert Java Objects To JSON With Jackson
Removing String Array Elements
In Java, you cannot directly remove elements from an array. However, you can simulate the removal of elements by creating a new array with the desired elements.
Example: Removing String Array Elements
To remove an element from a string array, you can use the following steps:
1. Find the index of the element you want to remove.
2. Create a new array with a size of one less than the original array.
3. Copy the elements from the original array to the new array, excluding the element to be removed.
Here’s an example:
String[] colors = {"Red", "Green", "Blue"}; int indexToRemove = 1; String[] newColors = new String[colors.length - 1]; for (int i = 0, j = 0; i < colors.length; i++) { if (i != indexToRemove) { newColors[j++] = colors[i]; } } System.out.println(Arrays.toString(newColors)); // Output: [Red, Blue]
In this example, we have a string array “colors” with three elements. We want to remove the element at index 1 (which is “Green”). We create a new array “newColors” with a size of one less than the original array. We then copy the elements from the original array to the new array, excluding the element at the specified index. Finally, we print the contents of the new array to verify that the element has been removed.
Iterating Over String Arrays
To iterate over a string array, you can use a loop, such as a for loop or a foreach loop, to access and process each element of the array.
Related Article: Storing Contact Information in Java Data Structures
Example: Iterating Over a String Array using a for Loop
To iterate over a string array using a for loop, you can use the following syntax:
String[] fruits = {"Apple", "Banana", "Orange"}; for (int i = 0; i < fruits.length; i++) { System.out.println(fruits[i]); }
In this example, we declare a string array “fruits” with three elements. We then use a for loop to iterate over the array and print each element.
Example: Iterating Over a String Array using a foreach Loop
To iterate over a string array using a foreach loop, you can use the following syntax:
String[] fruits = {"Apple", "Banana", "Orange"}; for (String fruit : fruits) { System.out.println(fruit); }
In this example, we declare a string array “fruits” with three elements. We then use a foreach loop to iterate over the array and print each element.
String Array Use Case: Data Validation
String arrays can be used for data validation, where you need to check if a given value matches any of the valid values in the array.
Related Article: How to Convert JSON String to Java Object
Example: Data Validation using a String Array
String[] validUserRoles = {"Admin", "Editor", "User"}; String userRole = "Editor"; boolean isValidRole = false; for (String role : validUserRoles) { if (role.equals(userRole)) { isValidRole = true; break; } } if (isValidRole) { System.out.println("Valid user role"); } else { System.out.println("Invalid user role"); }
In this example, we have a string array “validUserRoles” that contains the valid roles for a user. We also have a variable “userRole” that represents the role of a user. We iterate over the array using a foreach loop and check if the “userRole” matches any of the valid roles. If a match is found, we set the “isValidRole” variable to true. Finally, we print whether the user role is valid or invalid based on the value of “isValidRole”.
String Array Use Case: Text Processing
String arrays can be used for text processing tasks, such as splitting a string into an array of words or extracting specific parts of a string based on a delimiter.
Example: Splitting a String into an Array of Words
String sentence = "Hello, how are you?"; String[] words = sentence.split(" "); for (String word : words) { System.out.println(word); }
In this example, we have a string “sentence” that contains a sentence. We use the “split” method of the String class to split the sentence into an array of words using a space (” “) as the delimiter. We then iterate over the array using a foreach loop and print each word.
Related Article: How to Retrieve Current Date and Time in Java
Example: Extracting Specific Parts of a String using a Delimiter
String data = "John,Doe,30,New York"; String[] parts = data.split(","); String firstName = parts[0]; String lastName = parts[1]; int age = Integer.parseInt(parts[2]); String city = parts[3]; System.out.println("First Name: " + firstName); System.out.println("Last Name: " + lastName); System.out.println("Age: " + age); System.out.println("City: " + city);
In this example, we have a string “data” that contains comma-separated values representing different parts of a person’s information. We use the “split” method to split the string into an array of parts using a comma (“,”) as the delimiter. We then access each part of the array and assign it to the corresponding variables (firstName, lastName, age, city). Finally, we print the extracted parts of the string.
String Array Best Practice: Avoid Null Elements
It is a best practice to avoid having null elements in a string array. Null elements can cause NullPointerExceptions and make your code more error-prone.
Example: Avoiding Null Elements in a String Array
String[] names = {"John", "Jane", null, "Alice"}; for (String name : names) { if (name != null) { System.out.println(name); } }
In this example, we have a string array “names” that contains four elements, including a null element. Before printing each name, we check if it is not null using the != operator. This avoids NullPointerExceptions and ensures that only non-null elements are processed.
Related Article: How to Reverse a String in Java
String Array Best Practice: Using the Length Property
When working with string arrays, it is a best practice to use the length property to determine the size or length of the array instead of hardcoding a specific value.
Example: Using the Length Property of a String Array
String[] fruits = {"Apple", "Banana", "Orange"}; for (int i = 0; i < fruits.length; i++) { System.out.println(fruits[i]); }
In this example, we have a string array “fruits” with three elements. We use the length property (fruits.length) in the condition of the for loop to iterate over the array. This ensures that the loop iterates exactly the number of times as the length of the array, regardless of the specific number of elements.
Real World Example: String Arrays in File Handling
String arrays can be used in file handling tasks, such as reading data from a file into an array or writing data from an array to a file.
Related Article: How to Generate Random Integers in a Range in Java
Example: Reading Data from a File into a String Array
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class FileExample { public static void main(String[] args) { try { File file = new File("data.txt"); Scanner scanner = new Scanner(file); String[] lines = new String[3]; int index = 0; while (scanner.hasNextLine()) { String line = scanner.nextLine(); lines[index++] = line; } scanner.close(); for (String line : lines) { System.out.println(line); } } catch (FileNotFoundException e) { System.out.println("File not found"); } } }
In this example, we have a file named “data.txt” that contains three lines of text. We create a File object and a Scanner object to read the contents of the file. We also create a string array “lines” with a size of three elements. We use a while loop to read each line from the file using the “nextLine” method of the Scanner class and assign it to the corresponding element in the “lines” array. Finally, we close the scanner and print the contents of the array.
Real World Example: String Arrays in User Input Handling
String arrays can be used in user input handling to store and process multiple input values.
Example: Handling User Input using a String Array
import java.util.Scanner; public class UserInputExample { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the number of students: "); int numberOfStudents = scanner.nextInt(); scanner.nextLine(); String[] students = new String[numberOfStudents]; for (int i = 0; i < numberOfStudents; i++) { System.out.print("Enter the name of student " + (i + 1) + ": "); students[i] = scanner.nextLine(); } scanner.close(); System.out.println("Students: "); for (String student : students) { System.out.println(student); } } }
In this example, we prompt the user to enter the number of students and store it in the variable “numberOfStudents”. We then create a string array “students” with a size equal to the number of students. We use a for loop to iterate over the array and prompt the user to enter the name of each student, which we store in the corresponding element of the array. Finally, we print the names of the students.
Related Article: Java Equals Hashcode Tutorial
Performance Consideration: Memory Usage of String Arrays
When working with large string arrays, it is important to consider the memory usage. String arrays can consume a significant amount of memory, especially if the strings are long or if the array contains a large number of elements.
Performance Consideration: Speed of Operations on String Arrays
The speed of operations on string arrays can vary depending on the size of the array and the specific operation being performed. Generally, accessing and modifying individual elements of a string array has a constant time complexity of O(1), while iterating over the array has a linear time complexity of O(n), where n is the number of elements in the array.
Advanced Technique: Nested String Arrays
In Java, you can create nested string arrays, where each element of an array is another array. This allows you to create multi-level data structures or represent more complex data.
Related Article: How To Convert String To Int In Java
Example: Nested String Arrays
String[][] matrix = {{"1", "2", "3"}, {"4", "5", "6"}, {"7", "8", "9"}}; System.out.println(matrix[0][0]); // Output: 1 System.out.println(matrix[1][1]); // Output: 5 System.out.println(matrix[2][2]); // Output: 9
In this example, we create a nested string array “matrix” with three rows and three columns. Each element of the array is itself an array of strings. We can access individual elements of the nested array by specifying both the row index and the column index.
Advanced Technique: Multidimensional String Arrays
In addition to nested arrays, Java also supports multidimensional string arrays, where each element of the array can have more than one dimension.
Example: Multidimensional String Arrays
String[][][] cube = { {{"1", "2"}, {"3", "4"}}, {{"5", "6"}, {"7", "8"}} }; System.out.println(cube[0][0][0]); // Output: 1 System.out.println(cube[1][1][1]); // Output: 8
In this example, we create a multidimensional string array “cube” with two layers of nesting. Each element of the array is itself a two-dimensional array of strings. We can access individual elements of the multidimensional array by specifying the indices for each dimension.
Related Article: Java Composition Tutorial
Code Snippet Idea: Sorting String Arrays
Sorting a string array in ascending or descending order can be useful in various scenarios, such as alphabetizing a list of names or arranging words in a dictionary order.
Example: Sorting a String Array
import java.util.Arrays; String[] fruits = {"Orange", "Apple", "Banana"}; Arrays.sort(fruits); System.out.println(Arrays.toString(fruits)); // Output: [Apple, Banana, Orange]
In this example, we have a string array “fruits” with three elements. We use the “sort” method of the Arrays class to sort the array in ascending order. Finally, we print the sorted array using the “toString” method of the Arrays class.
Code Snippet Idea: Searching in String Arrays
Searching for a specific value in a string array can be done using various techniques, such as linear search or binary search, depending on the characteristics of the array and the requirements of the task.
Related Article: Java Hashmap Tutorial
Example: Linear Search in a String Array
String[] fruits = {"Apple", "Banana", "Orange"}; String searchItem = "Banana"; boolean found = false; for (String fruit : fruits) { if (fruit.equals(searchItem)) { found = true; break; } } if (found) { System.out.println("Item found"); } else { System.out.println("Item not found"); }
In this example, we have a string array “fruits” with three elements. We want to search for the value “Banana” in the array. We use a foreach loop to iterate over the array and compare each element with the search item. If a match is found, we set the “found” variable to true. Finally, we print whether the item was found or not based on the value of “found”.
Code Snippet Idea: Converting a String to String Array
Converting a string to a string array can be useful when you need to split a string into individual words or characters for further processing.
Example: Converting a String to a String Array
String sentence = "Hello, how are you?"; String[] words = sentence.split(" "); System.out.println(Arrays.toString(words));
In this example, we have a string “sentence” that contains a sentence. We use the “split” method of the String class to split the sentence into an array of words using a space (” “) as the delimiter. Finally, we print the string array using the “toString” method of the Arrays class.
Related Article: Popular Data Structures Asked in Java Interviews
Code Snippet Idea: Converting a String Array to List
Converting a string array to a list can be useful when you need to use list-specific operations or when you want to pass the array as a list to a method that expects a list as an argument.
Example: Converting a String Array to a List
import java.util.Arrays; import java.util.List; String[] fruits = {"Apple", "Banana", "Orange"}; List<String> fruitList = Arrays.asList(fruits); System.out.println(fruitList);
In this example, we have a string array “fruits” with three elements. We use the “asList” method of the Arrays class to convert the array to a list. Finally, we print the list.
Code Snippet Idea: Comparing String Arrays
Comparing string arrays can be done by comparing individual elements of the arrays or by using the Arrays.equals() method to compare the entire arrays.
Related Article: Java List Tutorial
Example: Comparing String Arrays
import java.util.Arrays; String[] fruits1 = {"Apple", "Banana", "Orange"}; String[] fruits2 = {"Apple", "Banana", "Orange"}; boolean areEqual = Arrays.equals(fruits1, fruits2); System.out.println("Are the arrays equal? " + areEqual);
In this example, we have two string arrays “fruits1” and “fruits2” with the same elements. We use the Arrays.equals() method to compare the arrays and store the result in the “areEqual” variable. Finally, we print whether the arrays are equal or not based on the value of “areEqual”.
Error Handling: NullPointer Exception with String Arrays
When working with string arrays, it is important to handle and prevent NullPointerExceptions, which can occur when trying to access or modify null elements in the array.
Example: Handling NullPointer Exception with String Arrays
String[] names = {"John", null, "Alice"}; for (String name : names) { if (name != null) { System.out.println(name.length()); } }
In this example, we have a string array “names” that contains three elements, including a null element. Before accessing the length of each name, we check if it is not null using the != operator. This prevents a NullPointerException from occurring and ensures that only non-null elements are processed.
Related Article: Java Set Tutorial
Error Handling: ArrayIndexOutOfBoundsException with String Arrays
When working with string arrays, it is important to handle and prevent ArrayIndexOutOfBoundsExceptions, which can occur when trying to access elements using invalid indices.
Example: Handling ArrayIndexOutOfBoundsException with String Arrays
String[] fruits = {"Apple", "Banana", "Orange"}; for (int i = 0; i <= fruits.length; i++) { try { System.out.println(fruits[i]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Invalid index"); } }
In this example, we have a string array “fruits” with three elements. We use a for loop to iterate over the array and access each element using the loop variable “i”. We intentionally try to access an element using an invalid index (fruits.length) to demonstrate the ArrayIndexOutOfBoundsException. We catch the exception using a try-catch block and print a message indicating an invalid index.