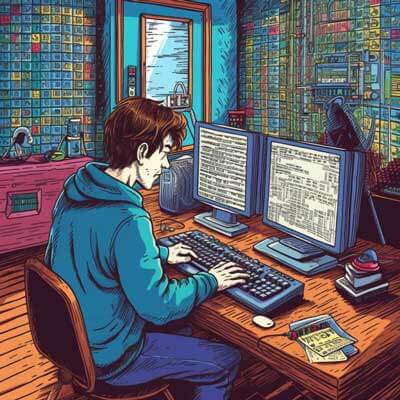
- Introduction to Printf Method
- Code Snippet: Basic Formatting with Printf Method
- Code Snippet: Numeric Formatting with Printf Method
- Printf Method Syntax
- Code Snippet: String Formatting with Printf Method
- Code Snippet: Date Formatting with Printf Method
- Printf Method Arguments
- Code Snippet: Custom Object Formatting with Printf Method
- Code Snippet: Numeric Formatting with Printf Method
- Printf Method Flags
- Code Snippet: Width and Precision with Printf Method
- Code Snippet: Width and Alignment with Printf Method
- Printf Method: Use Case 1
- Code Snippet: Generating Log Messages with Printf Method
- Code Snippet: Generating Log Messages with Printf Method (Alternative Syntax)
- Printf Method: Use Case 2
- Code Snippet: Formatting Data Output with Printf Method
- Code Snippet: Formatting Data Output with Printf Method (Additional Formatting)
- Printf Method: Use Case 3
- Code Snippet: Generating Formatted Data with Printf Method
- Code Snippet: Generating Formatted Data with Printf Method (Concatenation)
- Best Practices: Printf Method
- Use Descriptive Format Specifiers
- Avoid Ambiguous Format Specifiers
- Real World Example: Printf Method in Data Formatting
- Code Snippet: Formatting Data for Output
- Code Snippet: Formatting Data for Storage
- Real World Example: Printf Method in Log Generation
- Code Snippet: Generating Log Messages
- Code Snippet: Generating Log Messages with Exception Information
- Performance Considerations: Printf Method vs Concatenation
- Code Snippet: Comparing Performance of Printf Method and Concatenation
- Performance Considerations: Printf Method and Memory Usage
- Code Snippet: Memory Usage of Printf Method
- Advanced Technique: Printf Method with Custom Formatter
- Code Snippet: Custom Formatter for Binary Numbers
- Advanced Technique: Printf Method with Locale Settings
- Code Snippet: Formatting Numbers with Locale Settings
- Error Handling with Printf Method
- Code Snippet: Error Handling with Printf Method
- Performance Considerations: Printf Method and Exception Handling
- Code Snippet: Performance Considerations with Exception Handling
Introduction to Printf Method
The printf method in Java is a powerful tool for formatting and displaying text output. It allows you to create formatted strings by specifying placeholders for values that will be inserted into the final output. This method is commonly used for generating well-formatted log messages, displaying formatted data, and more.
Related Article: How To Parse JSON In Java
Code Snippet: Basic Formatting with Printf Method
String name = "John"; int age = 25; System.out.printf("Name: %s, Age: %d%n", name, age);
The above code snippet demonstrates the basic usage of the printf method. The placeholders %s
and %d
are used to specify the positions where the values of name
and age
will be inserted, respectively. The %n
is a platform-independent newline character.
Code Snippet: Numeric Formatting with Printf Method
double price = 19.99; System.out.printf("Price: %.2f%n", price);
In this example, the %f
placeholder is used to format the price
value as a floating-point number. The .2
specifies that the number should be displayed with two decimal places.
Printf Method Syntax
The syntax of the printf method is straightforward. It starts with the string that contains the format specifier, followed by the values to be inserted into the placeholders. The format specifier consists of a %
character followed by one or more conversion characters that define the type and formatting of the values.
Related Article: How To Convert Array To List In Java
Code Snippet: String Formatting with Printf Method
String name = "Alice"; System.out.printf("Hello, %s!%n", name);
In this example, the %s
placeholder is used to insert the value of the name
variable as a string.
Code Snippet: Date Formatting with Printf Method
import java.time.LocalDate; LocalDate today = LocalDate.now(); System.out.printf("Today is %tB %te, %tY%n", today, today, today);
In this code snippet, the %t
placeholder is used to format the today
date object. The B
represents the full month name, the e
represents the day of the month, and the Y
represents the four-digit year.
Printf Method Arguments
The printf method can accept a variable number of arguments, allowing you to format and display multiple values in a single call.
Related Article: How To Iterate Over Entries In A Java Map
Code Snippet: Custom Object Formatting with Printf Method
class Person { String name; int age; public Person(String name, int age) { this.name = name; this.age = age; } @Override public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + '}'; } } Person person1 = new Person("Jane", 30); Person person2 = new Person("Bob", 35); System.out.printf("Person 1: %s, Person 2: %s%n", person1, person2);
In this example, the %s
placeholder is used to insert the Person
objects person1
and person2
. Since the Person
class overrides the toString()
method, the formatted output will display the values of the name
and age
fields.
Code Snippet: Numeric Formatting with Printf Method
int number1 = 10; int number2 = 20; System.out.printf("Number 1: %d, Number 2: %d%n", number1, number2);
In this code snippet, the %d
placeholder is used to insert the values of number1
and number2
as integers.
Printf Method Flags
Flags in the printf method allow you to modify the formatting behavior. They can be used to add leading zeros, specify minimum field widths, control the alignment of values, and more.
Related Article: How To Split A String In Java
Code Snippet: Width and Precision with Printf Method
double value = 123.456; System.out.printf("Value: %10.2f%n", value);
In this example, the 10
specifies the minimum field width, ensuring that the output is at least 10 characters wide. The .2
specifies the precision, limiting the number of decimal places to two.
Code Snippet: Width and Alignment with Printf Method
String name = "Alice"; System.out.printf("Name: %-10s%n", name);
In this code snippet, the -
flag specifies left alignment, ensuring that the output is left-justified within the field width of 10 characters.
Printf Method: Use Case 1
One common use case for the printf method is generating well-formatted log messages. By using format specifiers, you can easily include variable values in the log message without the need for string concatenation.
Related Article: How To Convert Java Objects To JSON With Jackson
Code Snippet: Generating Log Messages with Printf Method
String message = "Error"; int errorCode = 500; System.out.printf("[%tF %tT] %s: %d%n", LocalDate.now(), LocalTime.now(), message, errorCode);
In this example, the %tF
and %tT
placeholders are used to insert the current date and time, respectively. The %s
and %d
placeholders are used to insert the values of message
and errorCode
.
Code Snippet: Generating Log Messages with Printf Method (Alternative Syntax)
String message = "Error"; int errorCode = 500; System.out.printf("[%1$tF %1$tT] %2$s: %3$d%n", LocalDate.now(), message, errorCode);
This code snippet demonstrates an alternative syntax for referencing the same argument multiple times. The numbers inside the %
characters specify the argument index to be used. In this case, %1$tF
refers to the first argument (LocalDate.now()
), %2$s
refers to the second argument (message
), and %3$d
refers to the third argument (errorCode
).
Printf Method: Use Case 2
Another use case for the printf method is displaying formatted data to the user. By using format specifiers, you can control the appearance of the output and provide a better user experience.
Related Article: Storing Contact Information in Java Data Structures
Code Snippet: Formatting Data Output with Printf Method
String product = "Widget"; double price = 9.99; int quantity = 3; double total = price * quantity; System.out.printf("Product: %s%n", product); System.out.printf("Price: $%.2f%n", price); System.out.printf("Quantity: %d%n", quantity); System.out.printf("Total: $%.2f%n", total);
In this example, the %s
placeholder is used to insert the product
value as a string. The %f
placeholder is used to format the price
and total
values as floating-point numbers with two decimal places. The %d
placeholder is used to insert the quantity
value as an integer.
Code Snippet: Formatting Data Output with Printf Method (Additional Formatting)
String product = "Widget"; double price = 9.99; double discount = 0.1; int quantity = 3; double total = price * quantity * (1 - discount); System.out.printf("Product: %20s%n", product); System.out.printf("Price: $%10.2f%n", price); System.out.printf("Discount: %.0f%%%n", discount * 100); System.out.printf("Quantity: %5d%n", quantity); System.out.printf("Total: $%10.2f%n", total);
This code snippet demonstrates additional formatting options. The numbers inside the %
characters specify the minimum field widths. By specifying a width, you can ensure consistent alignment of values in the output.
Printf Method: Use Case 3
A third use case for the printf method is generating formatted data for further processing or storage. By using format specifiers, you can create well-formatted strings that can be easily parsed or manipulated.
Related Article: How to Convert JSON String to Java Object
Code Snippet: Generating Formatted Data with Printf Method
String name = "Alice"; int age = 30; String formattedData = String.format("Name: %s, Age: %d", name, age); System.out.println(formattedData);
In this example, the String.format
method is used to generate a formatted string. The placeholders %s
and %d
are replaced with the values of name
and age
, respectively. The resulting string can then be stored or further processed as needed.
Code Snippet: Generating Formatted Data with Printf Method (Concatenation)
String name = "Alice"; int age = 30; String formattedData = "Name: " + name + ", Age: " + age; System.out.println(formattedData);
This code snippet demonstrates an alternative approach using string concatenation. While concatenation can be used to achieve similar results, the printf method provides a more concise and readable way to format data.
Best Practices: Printf Method
When using the printf method, it’s important to follow some best practices to ensure clean and maintainable code.
Related Article: How to Retrieve Current Date and Time in Java
Use Descriptive Format Specifiers
Choose format specifiers that accurately represent the intended data type and format. For example, use %d
for integers, %f
for floating-point numbers, %s
for strings, and %t
for dates.
Avoid Ambiguous Format Specifiers
Be mindful of format specifiers that can be easily confused. For example, %d
and %f
both represent numbers, but %d
is used for integers while %f
is used for floating-point numbers.
Real World Example: Printf Method in Data Formatting
The printf method is commonly used in data formatting tasks, where well-formatted data is crucial for readability and analysis.
Related Article: How to Reverse a String in Java
Code Snippet: Formatting Data for Output
double temperature = 25.5; double humidity = 0.65; System.out.printf("Temperature: %.1f°C%n", temperature); System.out.printf("Humidity: %.0f%%%n", humidity * 100);
In this example, the temperature and humidity values are formatted for output. The %f
placeholder is used to format the temperature value with one decimal place, and the humidity value is converted to a percentage using the %
character.
Code Snippet: Formatting Data for Storage
int year = 2022; int month = 9; int day = 15; String formattedDate = String.format("%d-%02d-%02d", year, month, day); System.out.println(formattedDate);
In this code snippet, the year, month, and day values are formatted for storage or further processing. The %d
placeholder is used for integers, and the %02d
specifier ensures that the month and day values are padded with leading zeros if necessary.
Real World Example: Printf Method in Log Generation
The printf method is widely used in log generation, where well-formatted log messages are essential for debugging and monitoring purposes.
Related Article: How to Generate Random Integers in a Range in Java
Code Snippet: Generating Log Messages
String level = "INFO"; String message = "Operation completed successfully"; System.out.printf("[%tF %tT] %s: %s%n", LocalDate.now(), LocalTime.now(), level, message);
In this example, the %tF
and %tT
placeholders are used to insert the current date and time. The %s
placeholders are used to insert the level
and message
values. This format ensures that log messages are consistently formatted with timestamps and relevant information.
Code Snippet: Generating Log Messages with Exception Information
String level = "ERROR"; String message = "An exception occurred"; Exception exception = new RuntimeException("Something went wrong"); System.out.printf("[%tF %tT] %s: %s%n%s%n", LocalDate.now(), LocalTime.now(), level, message, exception);
In this code snippet, an exception object is included in the log message. The %s
placeholder is used to insert the exception, which is automatically formatted using the toString()
method of the exception class.
Performance Considerations: Printf Method vs Concatenation
When it comes to performance, there is a trade-off between using the printf method and string concatenation. While the printf method provides more flexibility and readability, concatenation can be more efficient in certain scenarios.
Related Article: Java Equals Hashcode Tutorial
Code Snippet: Comparing Performance of Printf Method and Concatenation
long startTime = System.nanoTime(); for (int i = 0; i < 10000; i++) { String result = "Value: " + i; // or // String result = String.format("Value: %d", i); } long endTime = System.nanoTime(); long elapsedTime = endTime - startTime; System.out.println("Elapsed Time: " + elapsedTime + " nanoseconds");
In this example, we measure the elapsed time for performing 10,000 iterations of string concatenation and printf formatting. By comparing the elapsed times, you can get an idea of the performance difference between the two approaches.
Performance Considerations: Printf Method and Memory Usage
In addition to performance considerations, it’s important to be mindful of memory usage when using the printf method. The format string and the resulting formatted strings can consume significant memory, especially when working with large datasets.
Code Snippet: Memory Usage of Printf Method
String format = "%s: %d"; String result = String.format(format, "Value", 12345); System.out.println("Memory Usage: " + result.length() + " characters");
In this code snippet, we measure the memory usage of a formatted string. By checking the length of the resulting string, you can estimate the memory consumption. Keep in mind that the actual memory usage may vary depending on the JVM implementation and other factors.
Related Article: How To Convert String To Int In Java
Advanced Technique: Printf Method with Custom Formatter
The printf method supports custom formatters, allowing you to define your own format specifiers for specific types or formatting requirements.
Code Snippet: Custom Formatter for Binary Numbers
import java.util.Formatter; public class BinaryFormatter implements Formatter.BigDecimalLayoutForm { @Override public String layout(BigDecimal value, int scale, int precision) { return Integer.toBinaryString(value.intValue()); } } public class Main { public static void main(String[] args) { Formatter formatter = new Formatter(System.out); formatter.format(new BinaryFormatter(), "Binary: %b%n", new BigDecimal(10)); } }
In this example, a custom formatter BinaryFormatter
is created to format decimal numbers as binary strings. The layout
method is overridden to perform the binary conversion using Integer.toBinaryString()
. The custom formatter is then used with the printf method to display the binary representation of the decimal value.
Advanced Technique: Printf Method with Locale Settings
The printf method also supports locale settings, allowing you to format numbers, dates, and other values according to specific language and cultural conventions.
Related Article: Java Composition Tutorial
Code Snippet: Formatting Numbers with Locale Settings
import java.util.Locale; double value = 1234.56; System.out.printf(Locale.FRANCE, "Value: %.2f%n", value); System.out.printf(Locale.US, "Value: %.2f%n", value);
In this example, the Locale.FRANCE
and Locale.US
settings are used to format the value
according to French and US conventions, respectively. The %f
placeholder is used to format the value as a floating-point number with two decimal places.
Error Handling with Printf Method
When using the printf method, it’s important to handle any potential format or runtime errors that may occur during the formatting process.
Code Snippet: Error Handling with Printf Method
try { int invalidValue = Integer.parseInt("abc"); System.out.printf("Invalid Value: %d%n", invalidValue); } catch (NumberFormatException e) { System.err.println("Invalid number format"); e.printStackTrace(); }
In this example, the parseInt
method is used to parse a non-numeric string, which results in a NumberFormatException
. The exception is caught, and an error message is printed to the standard error stream. The exception stack trace is also printed for debugging purposes.
Related Article: Java Hashmap Tutorial
Performance Considerations: Printf Method and Exception Handling
When using the printf method, it’s important to be aware of the performance impact of exception handling. Exception handling can introduce overhead and should be used judiciously in performance-critical code.
Code Snippet: Performance Considerations with Exception Handling
long startTime = System.nanoTime(); try { for (int i = 0; i < 10000; i++) { String result = String.format("Value: %d", i); // or // System.out.printf("Value: %d%n", i); } } catch (Exception e) { e.printStackTrace(); } long endTime = System.nanoTime(); long elapsedTime = endTime - startTime; System.out.println("Elapsed Time: " + elapsedTime + " nanoseconds");
In this example, we measure the elapsed time for performing 10,000 iterations of string formatting with exception handling. By comparing the elapsed times with and without exception handling, you can assess the performance impact of exception handling in your specific use case.