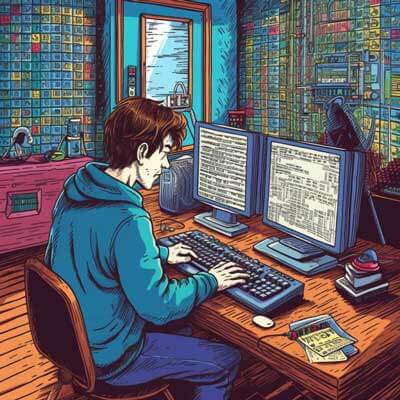
- Introduction to Lists
- List Types and Their Characteristics
- List Creation and Initialization
- Adding Elements to a List
- Removing Elements from a List
- Accessing List Elements
- List Iteration Techniques
- List Sorting Techniques
- Use Case: Managing Inventory with Lists
- Best Practice: Using Generics in Lists
- Real World Example: Using Lists for Data Manipulation
- Performance Consideration: List Size and Memory Usage
- Advanced Technique: Custom Comparators for List Sorting
- Code Snippet: Creating and Initializing a List
- Code Snippet: Adding Elements to a List
- Code Snippet: Removing Elements from a List
- Code Snippet: Accessing Elements in a List
- Code Snippet: Iterating Over a List
- Error Handling: Dealing with IndexOutOfBoundsException
Introduction to Lists
A list is a fundamental data structure in Java that represents an ordered collection of elements. It allows you to store and manipulate a group of related objects. Lists are commonly used in Java programming due to their flexibility and efficiency. They provide various methods and operations to add, remove, access, and manipulate elements.
In Java, the List interface is implemented by several classes, including ArrayList, LinkedList, and Vector. Each of these classes has its own characteristics and performance trade-offs, which we will explore in the next chapter.
Related Article: How To Parse JSON In Java
List Types and Their Characteristics
There are several types of lists available in Java, each with its own characteristics and use cases. Let’s take a closer look at some of the commonly used list types:
1. ArrayList: This class implements the List interface using a dynamic array. It provides fast random access and efficient element insertion and removal at the end of the list. However, inserting or removing elements in the middle of the list can be slower.
2. LinkedList: This class implements the List interface using a doubly-linked list. It provides efficient element insertion and removal at any position in the list. However, random access to elements can be slower compared to ArrayList.
3. Vector: This class is similar to ArrayList but is synchronized, making it thread-safe. It provides similar performance characteristics to ArrayList but with added synchronization overhead.
Each list type has its own advantages and trade-offs, so it’s important to choose the appropriate list type based on the specific requirements of your application.
List Creation and Initialization
To create a list in Java, you need to instantiate a class that implements the List interface. Here’s an example of creating an ArrayList and initializing it with some elements:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> names = new ArrayList<>(); names.add("John"); names.add("Alice"); names.add("Bob"); names.add("Emily"); // Print the list System.out.println(names); } }
In this example, we create an ArrayList called names
and add four elements to it. The add
method is used to append elements to the end of the list. Finally, we print the contents of the list using the println
method.
You can also initialize a list with predefined elements using the Arrays.asList
method:
import java.util.Arrays; import java.util.List; public class ListExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); // Print the list System.out.println(numbers); } }
In this example, we create a List called numbers
and initialize it with the elements 1, 2, 3, 4, and 5 using the Arrays.asList
method.
Adding Elements to a List
Adding elements to a list is a common operation in Java. You can add elements to a list using the add
method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Print the list System.out.println(fruits); } }
In this example, we create an ArrayList called fruits
and add three elements to it using the add
method. The elements are added to the end of the list. Finally, we print the contents of the list.
You can also insert elements at a specific position in the list using the add
method with an index parameter:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Blue"); colors.add(1, "Green"); // Print the list System.out.println(colors); } }
In this example, we create an ArrayList called colors
and add three elements to it. The element “Green” is inserted at index 1 using the add
method with the index parameter.
Related Article: How To Convert Array To List In Java
Removing Elements from a List
Removing elements from a list is another common operation in Java. You can remove elements from a list using the remove
method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Remove an element fruits.remove("Banana"); // Print the list System.out.println(fruits); } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then remove the element “Banana” using the remove
method. Finally, we print the contents of the list.
You can also remove elements by their index using the remove
method with an index parameter:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); // Remove an element by index colors.remove(1); // Print the list System.out.println(colors); } }
In this example, we create an ArrayList called colors
and add three elements to it. We then remove the element at index 1 (which is “Green”) using the remove
method with the index parameter.
Accessing List Elements
Accessing elements in a list is a common operation when working with lists in Java. You can access elements in a list using the get
method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Access an element String fruit = fruits.get(1); // Print the element System.out.println(fruit); } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then access the element at index 1 (which is “Banana”) using the get
method and store it in a variable called fruit
. Finally, we print the value of fruit
.
It’s important to note that list indices start from 0, so the first element is at index 0, the second element is at index 1, and so on.
You can also iterate over all the elements in a list using a loop. We will explore different list iteration techniques in the next chapter.
List Iteration Techniques
Iterating over the elements of a list is a common requirement in Java programming. There are several techniques you can use to iterate over a list. Let’s explore some of them:
1. Using a for loop: You can use a for loop to iterate over the elements of a list by using the list size as the loop condition and the get
method to access each element. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Iterate over the elements using a for loop for (int i = 0; i < fruits.size(); i++) { String fruit = fruits.get(i); System.out.println(fruit); } } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then use a for loop to iterate over the elements of the list. For each iteration, we use the get
method to access the element at the current index and print it.
2. Using an enhanced for loop: Java provides an enhanced for loop, also known as a for-each loop, that simplifies the process of iterating over the elements of a list. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Iterate over the elements using an enhanced for loop for (String fruit : fruits) { System.out.println(fruit); } } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then use an enhanced for loop to iterate over the elements of the list. For each iteration, the current element is assigned to the variable fruit
, which we can directly use in the loop body.
By using the appropriate iteration technique, you can efficiently process the elements of a list in your Java programs.
Related Article: How To Iterate Over Entries In A Java Map
List Sorting Techniques
Sorting elements in a list is a common requirement in Java programming. Java provides several techniques to sort the elements of a list. Let’s explore some of them:
1. Using the Collections.sort
method: The Collections
class in Java provides a sort
method that can be used to sort the elements of a list in ascending order. Here’s an example:
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class ListExample { public static void main(String[] args) { List<Integer> numbers = new ArrayList<>(); numbers.add(5); numbers.add(2); numbers.add(8); numbers.add(3); // Sort the list in ascending order Collections.sort(numbers); // Print the sorted list System.out.println(numbers); } }
In this example, we create an ArrayList called numbers
and add four elements to it. We then use the Collections.sort
method to sort the elements of the list in ascending order. Finally, we print the sorted list.
2. Using a custom comparator: If you need to sort a list in a custom order, you can implement a custom comparator and use it with the Collections.sort
method. Here’s an example:
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Sort the list using a custom comparator Collections.sort(fruits, new Comparator<String>() { @Override public int compare(String fruit1, String fruit2) { return fruit1.compareToIgnoreCase(fruit2); } }); // Print the sorted list System.out.println(fruits); } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then create a custom comparator using an anonymous inner class that compares the elements of the list in a case-insensitive manner. We use this custom comparator with the Collections.sort
method to sort the elements of the list. Finally, we print the sorted list.
By using the appropriate sorting technique, you can easily sort the elements of a list in your Java programs.
Use Case: Managing Inventory with Lists
Lists are commonly used to manage inventory in various applications. Let’s consider a use case where we need to manage the inventory of a store using lists.
To represent the inventory, we can create a class called Product
that has attributes such as id
, name
, price
, and quantity
. We can then create a list of Product
objects to store the inventory.
Here’s an example of managing inventory using lists in Java:
import java.util.ArrayList; import java.util.List; public class InventoryManager { private List<Product> inventory; public InventoryManager() { inventory = new ArrayList<>(); } public void addProduct(Product product) { inventory.add(product); } public void removeProduct(int productId) { for (Product product : inventory) { if (product.getId() == productId) { inventory.remove(product); break; } } } public void printInventory() { for (Product product : inventory) { System.out.println(product); } } public static void main(String[] args) { InventoryManager manager = new InventoryManager(); // Add products to the inventory manager.addProduct(new Product(1, "Apple", 0.99, 10)); manager.addProduct(new Product(2, "Banana", 0.49, 20)); manager.addProduct(new Product(3, "Orange", 0.79, 15)); // Remove a product from the inventory manager.removeProduct(2); // Print the inventory manager.printInventory(); } } class Product { private int id; private String name; private double price; private int quantity; public Product(int id, String name, double price, int quantity) { this.id = id; this.name = name; this.price = price; this.quantity = quantity; } // Getters and setters @Override public String toString() { return "Product [id=" + id + ", name=" + name + ", price=" + price + ", quantity=" + quantity + "]"; } }
In this example, we create an InventoryManager
class that manages the inventory using a list. We provide methods to add a product to the inventory, remove a product from the inventory based on its ID, and print the contents of the inventory.
We also create a Product
class that represents a product in the inventory. Each Product
object has attributes such as id
, name
, price
, and quantity
.
In the main
method, we demonstrate how to use the InventoryManager
to manage the inventory. We add three products to the inventory, remove one product, and then print the contents of the inventory.
By using lists, we can easily manage and manipulate inventory in our Java applications.
Best Practice: Using Generics in Lists
When working with lists in Java, it is considered a best practice to use generics to ensure type safety. Generics allow you to specify the type of elements that a list can hold, which helps prevent type-related errors at compile-time.
Here’s an example of using generics in a list:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> names = new ArrayList<>(); names.add("John"); names.add("Alice"); names.add("Bob"); // Print the list for (String name : names) { System.out.println(name); } } }
In this example, we create an ArrayList called names
and specify that it will hold elements of type String
by using the generic type parameter <String>
. This ensures that only String
objects can be added to the list, and the compiler will flag any attempts to add objects of other types.
Using generics in lists not only improves type safety but also makes the code more readable and maintainable by explicitly specifying the intended element type.
Related Article: How To Split A String In Java
Real World Example: Using Lists for Data Manipulation
Lists are widely used in real-world applications for data manipulation and processing. Let’s consider a real-world example where we need to manipulate a list of employee records.
Suppose we have a list of Employee
objects, where each Employee
object represents an employee’s record with attributes such as id
, name
, department
, and salary
. We want to calculate the average salary of all employees and find the highest-paid employee.
Here’s an example of using a list to manipulate employee records in Java:
import java.util.ArrayList; import java.util.List; public class EmployeeManager { private List<Employee> employees; public EmployeeManager() { employees = new ArrayList<>(); } public void addEmployee(Employee employee) { employees.add(employee); } public double getAverageSalary() { double totalSalary = 0; for (Employee employee : employees) { totalSalary += employee.getSalary(); } return totalSalary / employees.size(); } public Employee getHighestPaidEmployee() { Employee highestPaid = null; double maxSalary = Double.MIN_VALUE; for (Employee employee : employees) { if (employee.getSalary() > maxSalary) { maxSalary = employee.getSalary(); highestPaid = employee; } } return highestPaid; } public static void main(String[] args) { EmployeeManager manager = new EmployeeManager(); // Add employees manager.addEmployee(new Employee(1, "John Doe", "Engineering", 50000)); manager.addEmployee(new Employee(2, "Alice Smith", "Sales", 60000)); manager.addEmployee(new Employee(3, "Bob Johnson", "Marketing", 55000)); // Calculate the average salary double averageSalary = manager.getAverageSalary(); System.out.println("Average Salary: " + averageSalary); // Get the highest-paid employee Employee highestPaid = manager.getHighestPaidEmployee(); System.out.println("Highest-Paid Employee: " + highestPaid.getName()); } } class Employee { private int id; private String name; private String department; private double salary; public Employee(int id, String name, String department, double salary) { this.id = id; this.name = name; this.department = department; this.salary = salary; } // Getters and setters @Override public String toString() { return "Employee [id=" + id + ", name=" + name + ", department=" + department + ", salary=" + salary + "]"; } }
In this example, we create an EmployeeManager
class that manages employee records using a list. We provide methods to add an employee to the list, calculate the average salary of all employees, and find the highest-paid employee.
We also create an Employee
class that represents an employee’s record. Each Employee
object has attributes such as id
, name
, department
, and salary
.
In the main
method, we demonstrate how to use the EmployeeManager
to manipulate employee records. We add three employees to the list, calculate the average salary, and find the highest-paid employee.
By using lists for data manipulation, we can easily perform various operations on collections of objects in our Java applications.
Performance Consideration: List Size and Memory Usage
When working with lists in Java, it’s important to consider the size of the list and its impact on memory usage and performance. The size of a list can affect the time and memory required to perform various operations on the list.
For example, adding or removing elements at the end of an ArrayList is generally an efficient operation, as it has a constant time complexity of O(1). However, if the ArrayList needs to resize its underlying array to accommodate more elements, it incurs a performance penalty.
On the other hand, adding or removing elements in the middle of an ArrayList requires shifting the subsequent elements, which can be an expensive operation for large lists, as it has a time complexity of O(n).
LinkedList, on the other hand, provides efficient element insertion and removal at any position in the list, as it only requires updating the references between elements.
When dealing with large lists, it’s important to consider the memory usage. ArrayList, for example, allocates a contiguous block of memory for its elements, which can lead to increased memory usage if the list is large.
LinkedList, on the other hand, requires additional memory for storing the references between elements, which can also increase memory usage for large lists.
Therefore, if you anticipate frequent insertions or removals at arbitrary positions, LinkedList might be a better choice to avoid the performance penalty of shifting elements. However, if you primarily perform operations at the end of the list, ArrayList might be more efficient.
It’s also worth noting that the performance characteristics of lists can vary depending on the specific use case and the implementation of the Java runtime environment. It’s recommended to measure and profile your code to identify any performance bottlenecks and make informed decisions based on your specific requirements.
Advanced Technique: Custom Comparators for List Sorting
When sorting a list in Java, you can use custom comparators to define the sorting order based on specific criteria. A comparator is an object that compares two elements and determines their relative order.
Here’s an example of using a custom comparator to sort a list of objects based on a specific attribute:
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List; public class ListExample { public static void main(String[] args) { List<Employee> employees = new ArrayList<>(); employees.add(new Employee(1, "John Doe", 50000)); employees.add(new Employee(2, "Alice Smith", 60000)); employees.add(new Employee(3, "Bob Johnson", 55000)); // Sort the list based on salary in descending order Collections.sort(employees, new Comparator<Employee>() { @Override public int compare(Employee employee1, Employee employee2) { return Double.compare(employee2.getSalary(), employee1.getSalary()); } }); // Print the sorted list for (Employee employee : employees) { System.out.println(employee); } } } class Employee { private int id; private String name; private double salary; public Employee(int id, String name, double salary) { this.id = id; this.name = name; this.salary = salary; } // Getters and setters @Override public String toString() { return "Employee [id=" + id + ", name=" + name + ", salary=" + salary + "]"; } }
In this example, we create a list of Employee
objects and add three employees to it. We then use a custom comparator to sort the list based on the employees’ salaries in descending order.
The custom comparator is defined using an anonymous inner class that implements the Comparator
interface. The compare
method is overridden to compare two Employee
objects based on their salaries.
We use the Collections.sort
method to sort the list using the custom comparator. Finally, we print the sorted list.
By using custom comparators, we can easily define custom sorting orders for lists based on specific criteria.
Related Article: How To Convert Java Objects To JSON With Jackson
Code Snippet: Creating and Initializing a List
To create and initialize a list in Java, you need to instantiate a class that implements the List interface. Here’s an example of creating and initializing an ArrayList:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { // Create and initialize an ArrayList List<String> names = new ArrayList<>(); names.add("John"); names.add("Alice"); names.add("Bob"); // Print the list System.out.println(names); } }
In this example, we create an ArrayList called names
and add three elements to it using the add
method. The elements are added to the end of the list. Finally, we print the contents of the list.
You can also initialize a list with predefined elements using the Arrays.asList
method:
import java.util.Arrays; import java.util.List; public class ListExample { public static void main(String[] args) { // Create and initialize a List using Arrays.asList List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); // Print the list System.out.println(numbers); } }
In this example, we create a List called numbers
and initialize it with the elements 1, 2, 3, 4, and 5 using the Arrays.asList
method.
Code Snippet: Adding Elements to a List
Adding elements to a list is a common operation in Java. You can add elements to a list using the add
method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); // Add elements to the list fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Print the list System.out.println(fruits); } }
In this example, we create an ArrayList called fruits
and add three elements to it using the add
method. The elements are added to the end of the list. Finally, we print the contents of the list.
You can also insert elements at a specific position in the list using the add
method with an index parameter:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> colors = new ArrayList<>(); // Add elements to the list at specific positions colors.add("Red"); colors.add("Blue"); colors.add(1, "Green"); // Print the list System.out.println(colors); } }
In this example, we create an ArrayList called colors
and add three elements to it. The element “Green” is inserted at index 1 using the add
method with the index parameter.
Code Snippet: Removing Elements from a List
Removing elements from a list is another common operation in Java. You can remove elements from a list using the remove
method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Remove an element from the list fruits.remove("Banana"); // Print the list System.out.println(fruits); } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then remove the element “Banana” using the remove
method. Finally, we print the contents of the list.
You can also remove elements by their index using the remove
method with an index parameter:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); // Remove an element from the list by index colors.remove(1); // Print the list System.out.println(colors); } }
In this example, we create an ArrayList called colors
and add three elements to it. We then remove the element at index 1 (which is “Green”) using the remove
method with the index parameter.
Related Article: Storing Contact Information in Java Data Structures
Code Snippet: Accessing Elements in a List
Accessing elements in a list is a common operation when working with lists in Java. You can access elements in a list using the get
method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Access an element from the list String fruit = fruits.get(1); // Print the element System.out.println(fruit); } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then access the element at index 1 (which is “Banana”) using the get
method and store it in a variable called fruit
. Finally, we print the value of fruit
.
It’s important to note that list indices start from 0, so the first element is at index 0, the second element is at index 1, and so on.
You can also iterate over all the elements in a list using a loop. We will explore different list iteration techniques in the next chapter.
Code Snippet: Iterating Over a List
Iterating over the elements of a list is a common requirement in Java programming. There are several techniques you can use to iterate over a list. Let’s explore some of them:
1. Using a for loop: You can use a for loop to iterate over the elements of a list by using the list size as the loop condition and the get
method to access each element. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Iterate over the elements using a for loop for (int i = 0; i < fruits.size(); i++) { String fruit = fruits.get(i); System.out.println(fruit); } } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then use a for loop to iterate over the elements of the list. For each iteration, we use the get
method to access the element at the current index and print it.
2. Using an enhanced for loop: Java provides an enhanced for loop, also known as a for-each loop, that simplifies the process of iterating over the elements of a list. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Iterate over the elements using an enhanced for loop for (String fruit : fruits) { System.out.println(fruit); } } }
In this example, we create an ArrayList called fruits
and add three elements to it. We then use an enhanced for loop to iterate over the elements of the list. For each iteration, the current element is assigned to the variable fruit
, which we can directly use in the loop body.
By using the appropriate iteration technique, you can efficiently process the elements of a list in your Java programs.
Error Handling: Dealing with IndexOutOfBoundsException
When working with lists in Java, you need to be aware of the possibility of encountering an IndexOutOfBoundsException
if you try to access or remove an element with an invalid index.
The IndexOutOfBoundsException
is a runtime exception that is thrown when you try to access an element at an index that is outside the valid range of indices for the list.
To handle the IndexOutOfBoundsException
, you can use try-catch blocks to catch the exception and handle it gracefully. Here’s an example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); try { // Trying to access an element with an invalid index String fruit = fruits.get(2); System.out.println(fruit); } catch (IndexOutOfBoundsException e) { System.out.println("Invalid index. Please provide a valid index."); } } }
In this example, we create an ArrayList called fruits
and add two elements to it. We then try to access an element at index 2, which is an invalid index because the list has only two elements.
To handle this situation, we use a try-catch block to catch the IndexOutOfBoundsException
that is thrown when trying to access the element. In the catch block, we print an error message indicating that the index is invalid.
By handling the IndexOutOfBoundsException
, you can ensure that your program gracefully handles situations where the index provided is out of bounds for the list.