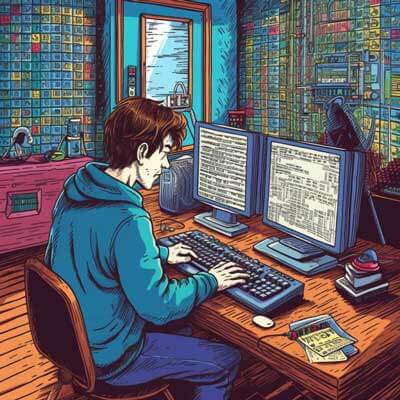
- Chapter 1: Introduction to Equals and Hashcode
- Example 1: Comparing Person Objects
- Example 2: Storing Person Objects in a HashSet
- Chapter 2: The Importance of Override in Equals and Hashcode
- Example: Storing Person Objects in a HashSet with Improper HashCode Implementation
- Chapter 3: Basics of Hashcode
- Chapter 4: Constructing the Equals Method
- Chapter 5: Constructing the Hashcode Method
- Chapter 6: Practical Use Case: Implementing Hashcode in a Class
- Example: Storing Book Objects in a HashSet
- Chapter 7: Practical Use Case: Implementing Equals in a Class
- Example: Comparing Point Objects
- Chapter 8: Best Practice: Consistency of Equals and Hashcode
- Chapter 9: Best Practice: Non-nullity of Equals and Hashcode
- Chapter 10: Real World Example: Hashcode in Complex Object
- Example: Storing Car Objects in a HashSet
- Chapter 11: Real World Example: Equals in Complex Object
- Example: Comparing Car Objects
- Chapter 12: Performance Consideration: Memory Impact of Hashcode
- Chapter 13: Performance Consideration: Time Complexity of Equals
- Chapter 14: Advanced Technique: Using Apache Commons Lang Library
- Chapter 15: Advanced Technique: Dealing with Inheritance
- Chapter 16: Code Snippet: Basic Hashcode Implementation
- Chapter 17: Code Snippet: Basic Equals Implementation
- Chapter 18: Code Snippet: Advanced Hashcode with Custom Objects
- Chapter 19: Code Snippet: Advanced Equals with Custom Objects
- Chapter 20: Code Snippet: Dealing with Null Values in Equals and Hashcode
- Chapter 21: Error Handling: Dealing with Unhandled Exceptions
- Chapter 22: Error Handling: Debugging Common Issues
Chapter 1: Introduction to Equals and Hashcode
In Java, the equals
and hashCode
methods are used to compare objects and determine their equality. The equals
method checks if two objects are equal, while the hashCode
method returns a unique identifier for an object. These methods are essential for proper functioning of collections like HashSet
and HashMap
.
To understand the concept of equals
and hashCode
, let’s consider an example. Suppose we have a Person
class with attributes like name
and age
. We want to compare two Person
objects based on their names. We can define the equals
method to check if the names are equal, and the hashCode
method to generate a hash code based on the name.
Here’s an example of the Person
class with the equals
and hashCode
methods:
public class Person { private String name; private int age; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Person person = (Person) obj; return Objects.equals(name, person.name); } @Override public int hashCode() { return Objects.hash(name); } }
In this example, the equals
method checks if the name
attribute is equal using the Objects.equals
method. The hashCode
method generates a hash code based on the name
attribute using the Objects.hash
method.
Related Article: How To Parse JSON In Java
Example 1: Comparing Person Objects
Now, let’s create two Person
objects and compare them using the equals
method:
Person person1 = new Person("John", 25); Person person2 = new Person("John", 30); System.out.println(person1.equals(person2)); // Output: true
In this example, the equals
method returns true
because the names of the Person
objects are equal.
Example 2: Storing Person Objects in a HashSet
The hashCode
method is used when storing objects in collections like HashSet
. Let’s see an example:
Set<Person> people = new HashSet<>(); Person person1 = new Person("John", 25); Person person2 = new Person("John", 30); people.add(person1); people.add(person2); System.out.println(people.size()); // Output: 1
In this example, the HashSet
ensures that only one Person
object with the same name is added, thanks to the hashCode
method. The equals
method is used to check if two objects are equal when adding them to the HashSet
.
Chapter 2: The Importance of Override in Equals and Hashcode
When overriding the equals
and hashCode
methods in Java, it is crucial to follow certain guidelines. The equals
method should have specific properties, such as reflexivity, symmetry, transitivity, and consistency. Similarly, the hashCode
method should return the same hash code for objects that are equal according to the equals
method.
Let’s consider an example to understand why it is important to override these methods properly.
Suppose we have a Person
class with attributes name
and age
, and we override the equals
method to compare objects based on their names only. If we don’t override the hashCode
method, the Person
objects will not work correctly when stored in collections like HashSet
or HashMap
.
Here’s an example of a Person
class with improper hashCode
implementation:
public class Person { private String name; private int age; // Constructor, getters, setters @Override public boolean equals(Object obj) { // Equals implementation } @Override public int hashCode() { return Objects.hash(name); // Improper hashCode implementation } }
In this example, the hashCode
method only takes into account the name
attribute. As a result, two Person
objects with the same name will have different hash codes, violating the contract between equals
and hashCode
.
Let’s see an example to understand the consequences of improper hashCode
implementation:
Related Article: How To Convert Array To List In Java
Example: Storing Person Objects in a HashSet with Improper HashCode Implementation
Set<Person> people = new HashSet<>(); Person person1 = new Person("John", 25); Person person2 = new Person("John", 30); people.add(person1); people.add(person2); System.out.println(people.size()); // Output: 2
In this example, the HashSet
considers the two Person
objects as different, even though their names are the same. This happens because the hashCode
method generates different hash codes for the objects, causing them to be stored separately in the HashSet
.
To fix this issue, we need to override the hashCode
method properly. We’ll cover this in the next chapter.
Chapter 3: Basics of Hashcode
The hashCode
method in Java returns a hash code value for an object. The hash code is an integer that is typically used to improve the performance of hash-based data structures like HashSet
and HashMap
.
The contract between the equals
and hashCode
methods states that if two objects are equal according to the equals
method, their hash codes must be equal as well. This means that if you override the equals
method, you must also override the hashCode
method to ensure consistency.
The hashCode
method should generate hash codes that distribute objects evenly across the hash-based data structure. Ideally, each object should have a unique hash code. However, due to the limited range of integers, collisions can occur, where different objects have the same hash code.
Java provides the Objects.hash
method to generate hash codes based on the attributes of an object. This method combines the hash codes of the attributes using a simple algorithm to minimize collisions.
Here’s an example of the hashCode
method using Objects.hash
:
@Override public int hashCode() { return Objects.hash(attribute1, attribute2, attribute3); }
In this example, the hashCode
method generates a hash code based on the values of attribute1
, attribute2
, and attribute3
.
It is important to note that the hashCode
method should only take into account the attributes used in the equals
method. If you include attributes that are not used for equality comparison, it may result in unequal objects having the same hash code, leading to incorrect behavior in hash-based data structures.
In the next chapter, we’ll see how to properly construct the equals
method.
Chapter 4: Constructing the Equals Method
The equals
method in Java is used to compare objects for equality. When overriding the equals
method, it is important to follow certain guidelines to ensure correct behavior.
The equals
method should have the following properties:
– Reflexivity: x.equals(x)
should always return true
.
– Symmetry: If x.equals(y)
returns true
, then y.equals(x)
should also return true
.
– Transitivity: If x.equals(y)
returns true
and y.equals(z)
returns true
, then x.equals(z)
should also return true
.
– Consistency: Multiple invocations of x.equals(y)
should consistently return the same result as long as the objects are not modified.
To construct the equals
method, follow these steps:
1. Check if the objects are identical: Use the ==
operator to check if the objects are the same instance. If they are, return true
.
2. Check if the object is null
or of a different class: Use the getClass
method and the null
check to ensure the object is of the same class. If it is not, return false
.
3. Cast the object to the appropriate class: Use the cast operator to cast the object to the appropriate class.
4. Compare the attributes for equality: Compare the attributes of the objects using the equals
method or appropriate comparison operators. Return false
if any of the attributes are not equal.
5. Return true
if all checks pass: If all checks pass, return true
.
Here’s an example of the equals
method for the Person
class:
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Person person = (Person) obj; return Objects.equals(attribute1, person.attribute1) && Objects.equals(attribute2, person.attribute2) && Objects.equals(attribute3, person.attribute3); }
In this example, we first check if the objects are identical using the ==
operator. Then, we check if the object is null
or of a different class. If both checks pass, we cast the object to the Person
class and compare the attributes using the Objects.equals
method.
In the next chapter, we’ll see how to construct the hashCode
method.
Related Article: How To Iterate Over Entries In A Java Map
Chapter 5: Constructing the Hashcode Method
The hashCode
method in Java is used to generate a hash code for an object. A hash code is an integer that is typically used in hash-based data structures like HashSet
and HashMap
to improve performance.
When overriding the hashCode
method, it is important to follow certain guidelines to ensure correctness and consistency.
To construct the hashCode
method, follow these steps:
1. Initialize a result variable to a prime number: Start by initializing a result variable to a prime number, typically 31.
2. Combine the hash codes of the attributes: Use the Objects.hash
method to generate a hash code for each attribute, and combine them using a simple algorithm. You can use addition, multiplication, or bitwise operators to combine the hash codes.
3. Return the final hash code: Return the final hash code as the result.
Here’s an example of the hashCode
method for the Person
class:
@Override public int hashCode() { return Objects.hash(attribute1, attribute2, attribute3); }
In this example, we use the Objects.hash
method to generate a hash code based on the attributes attribute1
, attribute2
, and attribute3
. The Objects.hash
method combines the hash codes of the attributes using a simple algorithm.
It is important to note that the attributes used in the hashCode
method should be the same attributes used in the equals
method. If you include additional attributes, it may result in unequal objects having the same hash code, leading to incorrect behavior in hash-based data structures.
In the next chapter, we’ll see a practical use case of implementing hashCode
in a class.
Chapter 6: Practical Use Case: Implementing Hashcode in a Class
Implementing the hashCode
method in a class allows objects of that class to be stored and retrieved efficiently from hash-based data structures like HashSet
and HashMap
. In this chapter, we’ll explore a practical use case of implementing the hashCode
method in a class.
Suppose we have a Book
class with attributes like title
, author
, and ISBN
. We want to compare Book
objects based on their title
and author
attributes. To ensure correct behavior in hash-based data structures, we need to override the hashCode
method.
Here’s an example of the Book
class with the equals
and hashCode
methods:
public class Book { private String title; private String author; private String isbn; // Constructor, getters, setters @Override public boolean equals(Object obj) { // Equals implementation } @Override public int hashCode() { return Objects.hash(title, author); } }
In this example, the equals
method compares the title
and author
attributes using the Objects.equals
method. The hashCode
method generates a hash code based on the title
and author
attributes using the Objects.hash
method.
Let’s see an example of storing Book
objects in a HashSet
:
Example: Storing Book Objects in a HashSet
Set<Book> books = new HashSet<>(); Book book1 = new Book("Title 1", "Author 1", "ISBN 1"); Book book2 = new Book("Title 2", "Author 2", "ISBN 2"); Book book3 = new Book("Title 1", "Author 1", "ISBN 3"); books.add(book1); books.add(book2); books.add(book3); System.out.println(books.size()); // Output: 2
In this example, the HashSet
ensures that only two Book
objects are added because book1
and book3
have the same title
and author
. The hashCode
method is used to determine if objects are equal and avoid duplicates in the HashSet
.
By properly implementing the hashCode
method, we can achieve efficient and correct behavior when storing and retrieving objects from hash-based data structures.
In the next chapter, we’ll see a practical use case of implementing the equals
method in a class.
Related Article: How To Split A String In Java
Chapter 7: Practical Use Case: Implementing Equals in a Class
Implementing the equals
method in a class allows objects of that class to be compared for equality. In this chapter, we’ll explore a practical use case of implementing the equals
method in a class.
Let’s consider a Point
class with x
and y
coordinates. We want to compare Point
objects based on their coordinates. To ensure correct behavior, we need to override the equals
method.
Here’s an example of the Point
class with the equals
method:
public class Point { private int x; private int y; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Point point = (Point) obj; return x == point.x && y == point.y; } }
In this example, the equals
method checks if the x
and y
coordinates of two Point
objects are equal.
Let’s see an example of comparing Point
objects:
Example: Comparing Point Objects
Point point1 = new Point(1, 2); Point point2 = new Point(1, 2); Point point3 = new Point(3, 4); System.out.println(point1.equals(point2)); // Output: true System.out.println(point1.equals(point3)); // Output: false
In this example, the equals
method returns true
for point1
and point2
because their coordinates are equal. It returns false
for point1
and point3
because their coordinates are different.
By properly implementing the equals
method, we can compare objects for equality based on specific attributes or criteria.
In the next chapter, we’ll discuss best practices for ensuring consistency and non-nullity of the equals
and hashCode
methods.
Chapter 8: Best Practice: Consistency of Equals and Hashcode
To ensure correct behavior and avoid unexpected issues, it is important to follow best practices when implementing the equals
and hashCode
methods in Java.
One important best practice is to ensure consistency between the equals
and hashCode
methods. According to the contract between these methods, if two objects are equal according to the equals
method, their hash codes must be equal as well. In other words, if obj1.equals(obj2)
returns true
, then obj1.hashCode()
should equal obj2.hashCode()
.
To achieve consistency, make sure that the attributes used in the equals
method are also used in the hashCode
method. This ensures that two equal objects have the same hash code, which is crucial for correct behavior in hash-based data structures like HashSet
and HashMap
.
Here’s an example of consistent equals
and hashCode
methods for a Person
class:
public class Person { private String name; private int age; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Person person = (Person) obj; return Objects.equals(name, person.name) && age == person.age; } @Override public int hashCode() { return Objects.hash(name, age); } }
In this example, the equals
method compares both the name
and age
attributes, while the hashCode
method generates a hash code based on both attributes. This ensures consistency between the two methods.
In the next chapter, we’ll discuss another best practice: non-nullity of the equals
and hashCode
methods.
Related Article: How To Convert Java Objects To JSON With Jackson
Chapter 9: Best Practice: Non-nullity of Equals and Hashcode
Another best practice when implementing the equals
and hashCode
methods in Java is to ensure non-nullity. According to the contract between these methods, the equals
method should return false
if the argument is null
.
To ensure non-nullity, include a null check at the beginning of the equals
method. If the argument is null
, return false
immediately. This prevents possible NullPointerExceptions
and ensures correct behavior when comparing objects.
Here’s an example of the equals
method with a null check for a Person
class:
public class Person { private String name; private int age; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Person person = (Person) obj; return Objects.equals(name, person.name) && age == person.age; } @Override public int hashCode() { return Objects.hash(name, age); } }
In this example, the equals
method first checks if the argument is null
. If it is, it returns false
. This ensures non-nullity and prevents possible NullPointerExceptions
.
In the next chapter, we’ll explore a real-world example of implementing the hashCode
method in a complex object.
Chapter 10: Real World Example: Hashcode in Complex Object
Implementing the hashCode
method becomes more challenging when dealing with complex objects that contain other objects as attributes. In this chapter, we’ll explore a real-world example of implementing the hashCode
method in a complex object.
Suppose we have a Car
class with attributes like make
, model
, and an object of the Engine
class. We want to compare Car
objects based on all their attributes. To ensure correct behavior in hash-based data structures, we need to override the hashCode
method.
Here’s an example of the Car
class with the equals
and hashCode
methods:
public class Car { private String make; private String model; private Engine engine; // Constructor, getters, setters @Override public boolean equals(Object obj) { // Equals implementation } @Override public int hashCode() { return Objects.hash(make, model, engine); } }
In this example, the equals
method compares all the attributes using the Objects.equals
method. The hashCode
method generates a hash code based on the make
, model
, and engine
attributes using the Objects.hash
method.
Let’s see an example of storing Car
objects in a HashSet
:
Example: Storing Car Objects in a HashSet
Set<Car> cars = new HashSet<>(); Car car1 = new Car("Make 1", "Model 1", new Engine("Engine 1")); Car car2 = new Car("Make 2", "Model 2", new Engine("Engine 2")); Car car3 = new Car("Make 1", "Model 1", new Engine("Engine 1")); cars.add(car1); cars.add(car2); cars.add(car3); System.out.println(cars.size()); // Output: 2
In this example, the HashSet
ensures that only two Car
objects are added because car1
and car3
have the same attributes. The hashCode
method is used to determine if objects are equal and avoid duplicates in the HashSet
.
By properly implementing the hashCode
method, we can achieve correct behavior when comparing complex objects.
In the next chapter, we’ll explore a real-world example of implementing the equals
method in a complex object.
Related Article: Storing Contact Information in Java Data Structures
Chapter 11: Real World Example: Equals in Complex Object
Implementing the equals
method in a complex object becomes more challenging when dealing with objects that contain other objects as attributes. In this chapter, we’ll explore a real-world example of implementing the equals
method in a complex object.
Suppose we have a Car
class with attributes like make
, model
, and an object of the Engine
class. We want to compare Car
objects based on all their attributes. To ensure correct behavior, we need to override the equals
method.
Here’s an example of the Car
class with the equals
and hashCode
methods:
public class Car { private String make; private String model; private Engine engine; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Car car = (Car) obj; return Objects.equals(make, car.make) && Objects.equals(model, car.model) && Objects.equals(engine, car.engine); } @Override public int hashCode() { return Objects.hash(make, model, engine); } }
In this example, the equals
method checks if all the attributes are equal using the Objects.equals
method. The hashCode
method generates a hash code based on all the attributes using the Objects.hash
method.
Let’s see an example of comparing Car
objects:
Example: Comparing Car Objects
Car car1 = new Car("Make 1", "Model 1", new Engine("Engine 1")); Car car2 = new Car("Make 2", "Model 2", new Engine("Engine 2")); Car car3 = new Car("Make 1", "Model 1", new Engine("Engine 1")); System.out.println(car1.equals(car2)); // Output: false System.out.println(car1.equals(car3)); // Output: true
In this example, the equals
method returns false
for car1
and car2
because their attributes are different. It returns true
for car1
and car3
because their attributes are equal.
By properly implementing the equals
method, we can compare complex objects for equality based on all their attributes.
In the next chapter, we’ll discuss the memory impact of the hashCode
method.
Chapter 12: Performance Consideration: Memory Impact of Hashcode
The hashCode
method in Java is used to generate a hash code for an object. While hash codes improve the performance of hash-based data structures, they also have a memory impact.
The memory impact of the hashCode
method depends on the size of the attributes used to generate the hash code. If the attributes have large memory footprints, the hash code generation process can consume significant memory.
To minimize the memory impact, follow these guidelines:
1. Use a limited number of attributes: Include only the necessary attributes in the hashCode
method. Avoid including attributes with large memory footprints, such as large arrays or collections.
2. Consider using smaller data types: If possible, use smaller data types for attributes to reduce the memory footprint. For example, use int
instead of long
if the attribute values fit within the range of an int
.
3. Use a balanced combination of attributes: If multiple attributes contribute to the hash code, try to use a balanced combination to distribute objects evenly across hash-based data structures. This helps avoid collisions and improves performance.
It is important to strike a balance between memory impact and the distribution of objects in hash-based data structures. Choosing the right attributes and data types can help achieve this balance.
In the next chapter, we’ll discuss the time complexity of the equals
method.
Related Article: How to Convert JSON String to Java Object
Chapter 13: Performance Consideration: Time Complexity of Equals
The equals
method in Java is used to compare objects for equality. While the equals
method is essential for correct behavior, it is also important to consider its time complexity.
The time complexity of the equals
method depends on the number of attributes being compared. If the class has a large number of attributes, the time complexity of the equals
method can be significant.
To minimize the time complexity, follow these guidelines:
1. Use a limited number of attributes: Include only the necessary attributes in the equals
method. Avoid comparing attributes that are not essential for equality.
2. Optimize attribute comparison: If certain attributes are more likely to be unequal, compare them first. This can help avoid unnecessary attribute comparisons if the first attributes already indicate inequality.
3. Use short-circuiting: If the equals
method involves multiple comparisons, use short-circuiting to exit early if inequality is detected. For example, use the &&
operator to combine attribute comparisons, as it stops evaluating if the first comparison is false
.
By considering the time complexity and optimizing the equals
method, you can improve the performance of object comparisons.
In the next chapter, we’ll explore an advanced technique: using the Apache Commons Lang library.
Chapter 14: Advanced Technique: Using Apache Commons Lang Library
The Apache Commons Lang library provides a convenient utility class, EqualsBuilder
, that simplifies the implementation of the equals
method. This library reduces the boilerplate code required to compare attributes and follow best practices.
To use the Apache Commons Lang library, follow these steps:
1. Add the Apache Commons Lang library as a dependency in your project.
2. Import the necessary classes from the library, such as EqualsBuilder
.
3. Use the EqualsBuilder
class to compare attributes and construct the equals
method.
Here’s an example of using the Apache Commons Lang library in the equals
method for a Person
class:
import org.apache.commons.lang3.builder.EqualsBuilder; public class Person { private String name; private int age; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Person person = (Person) obj; return new EqualsBuilder() .append(name, person.name) .append(age, person.age) .isEquals(); } }
In this example, we use the EqualsBuilder
class from the Apache Commons Lang library to compare the name
and age
attributes. The isEquals
method of the EqualsBuilder
class returns the final result of the equals
method.
Using the Apache Commons Lang library can simplify the implementation of the equals
method and ensure adherence to best practices.
In the next chapter, we’ll explore an advanced technique for dealing with inheritance in the equals
and hashCode
methods.
Chapter 15: Advanced Technique: Dealing with Inheritance
When dealing with inheritance in the equals
and hashCode
methods, it is important to consider the behavior of the superclass and ensure correct comparisons.
To properly handle inheritance, follow these guidelines:
1. Call the superclass’s equals
method: In the equals
method of a subclass, call the superclass’s equals
method using the super.equals(obj)
syntax. This ensures that the superclass’s attributes are compared correctly.
2. Include superclass attributes in hashCode
: When generating the hash code in the hashCode
method of a subclass, include the superclass’s attributes using the Objects.hash
method. This ensures that the superclass’s attributes contribute to the hash code.
3. Override superclass’s equals
and hashCode
if necessary: If the superclass’s equals
and hashCode
methods do not meet the requirements of the subclass, override them in the subclass.
Here’s an example of a subclass Child
inheriting from a superclass Parent
:
public class Parent { private String name; private int age; // Constructor, getters, setters @Override public boolean equals(Object obj) { // Equals implementation } @Override public int hashCode() { return Objects.hash(name, age); } } public class Child extends Parent { private String address; // Constructor, getters, setters @Override public boolean equals(Object obj) { if (!super.equals(obj)) { return false; } Child child = (Child) obj; return Objects.equals(address, child.address); } @Override public int hashCode() { return Objects.hash(super.hashCode(), address); } }
In this example, the Child
class calls the superclass’s equals
method using super.equals(obj)
and includes the superclass’s hash code in the hashCode
method using super.hashCode()
. The Child
class then compares the address
attribute and includes it in the hash code.
By properly handling inheritance, you can ensure correct comparisons and hash code generation in subclasses.
In the next chapter, we’ll provide a code snippet for a basic hashCode
implementation.
Related Article: How to Retrieve Current Date and Time in Java
Chapter 16: Code Snippet: Basic Hashcode Implementation
Implementing the hashCode
method in Java follows certain guidelines to ensure correctness and consistency. Here’s a code snippet for a basic hashCode
implementation:
@Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + Objects.hashCode(attribute1); result = prime * result + Objects.hashCode(attribute2); result = prime * result + Objects.hashCode(attribute3); return result; }
In this code snippet, we use a prime number (31) and a result variable to generate the hash code. We multiply the result by the prime number and add the hash codes of the attributes using the Objects.hashCode
method. Finally, we return the result as the hash code.
This basic implementation ensures consistency and minimizes collisions when using hash-based data structures.
In the next chapter, we’ll provide a code snippet for a basic equals
implementation.
Chapter 17: Code Snippet: Basic Equals Implementation
Implementing the equals
method in Java follows certain guidelines to ensure correctness and consistency. Here’s a code snippet for a basic equals
implementation:
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } final MyClass other = (MyClass) obj; return Objects.equals(attribute1, other.attribute1) && Objects.equals(attribute2, other.attribute2) && Objects.equals(attribute3, other.attribute3); }
In this code snippet, we first check if the objects are identical using the ==
operator. Then, we check if the object is null
or of a different class. If both checks pass, we cast the object to the appropriate class and compare the attributes using the Objects.equals
method. Finally, we return the result of the attribute comparisons.
This basic implementation ensures correctness and consistency when comparing objects for equality.
In the next chapter, we’ll provide a code snippet for advanced hashCode
implementation with custom objects.
Chapter 18: Code Snippet: Advanced Hashcode with Custom Objects
Implementing the hashCode
method for objects that contain other objects as attributes requires special consideration. Here’s a code snippet for an advanced hashCode
implementation with custom objects:
@Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + Objects.hashCode(attribute1); result = prime * result + Objects.hashCode(attribute2); result = prime * result + Objects.hashCode(attribute3); result = prime * result + customObject1.hashCode(); result = prime * result + customObject2.hashCode(); return result; }
In this code snippet, we use a prime number (31) and a result variable to generate the hash code. We multiply the result by the prime number and add the hash codes of the attributes using the Objects.hashCode
method. For custom objects, we call their hashCode
methods directly. Finally, we return the result as the hash code.
By including the hash codes of custom objects, we ensure correct hash code generation for objects with complex attributes.
In the next chapter, we’ll provide a code snippet for advanced equals
implementation with custom objects.
Related Article: How to Reverse a String in Java
Chapter 19: Code Snippet: Advanced Equals with Custom Objects
Implementing the equals
method for objects that contain other objects as attributes requires special consideration. Here’s a code snippet for an advanced equals
implementation with custom objects:
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } final MyClass other = (MyClass) obj; return Objects.equals(attribute1, other.attribute1) && Objects.equals(attribute2, other.attribute2) && Objects.equals(attribute3, other.attribute3) && Objects.equals(customObject1, other.customObject1) && Objects.equals(customObject2, other.customObject2); }
In this code snippet, we first check if the objects are identical using the ==
operator. Then, we check if the object is null
or of a different class. If both checks pass, we cast the object to the appropriate class and compare the attributes using the Objects.equals
method. For custom objects, we call their equals
methods directly. Finally, we return the result of the attribute comparisons.
By including the comparisons of custom objects, we ensure correct equality comparisons for objects with complex attributes.
In the next chapter, we’ll explore how to deal with null values in the equals
and hashCode
methods.
Chapter 20: Code Snippet: Dealing with Null Values in Equals and Hashcode
When implementing the equals
and hashCode
methods, it is important to handle null values properly to avoid NullPointerExceptions
and ensure correct behavior. Here’s a code snippet for dealing with null values:
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } final MyClass other = (MyClass) obj; return Objects.equals(attribute1, other.attribute1) && Objects.equals(attribute2, other.attribute2) && Objects.equals(attribute3, other.attribute3) && Objects.equals(customObject1, other.customObject1) && Objects.equals(customObject2, other.customObject2); } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + Objects.hashCode(attribute1); result = prime * result + Objects.hashCode(attribute2); result = prime * result + Objects.hashCode(attribute3); result = prime * result + (customObject1 != null ? customObject1.hashCode() : 0); result = prime * result + (customObject2 != null ? customObject2.hashCode() : 0); return result; }
In this code snippet, we use the Objects.equals
method to compare attributes for equality, which handles null values correctly. When generating the hash code, we use the conditional operator (?
) to check for null values and call their hashCode
methods only if they are not null.
By handling null values properly, we ensure correct behavior in the equals
and hashCode
methods.
In the next chapter, we’ll discuss error handling when dealing with unhandled exceptions.
Chapter 21: Error Handling: Dealing with Unhandled Exceptions
When implementing the equals
and hashCode
methods, it is important to handle unhandled exceptions properly to ensure correct behavior and avoid unexpected issues. Here are some error handling strategies:
1. Catch and handle exceptions: If an attribute comparison or hash code generation can throw an exception, catch the exception and handle it appropriately. You can return a default value or throw a custom exception to indicate the error.
2. Document potential exceptions: If an attribute comparison or hash code generation is known to throw exceptions, document it in the method’s documentation or comments. This helps other developers understand the potential issues and handle them properly.
Here’s an example of catching and handling exceptions in the equals
method:
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } final MyClass other = (MyClass) obj; try { // Attribute comparisons that can throw exceptions } catch (Exception e) { // Handle the exception or return a default value return false; } return true; }
In this example, we catch any exceptions that may occur during attribute comparisons and handle them appropriately. By handling unhandled exceptions, we ensure error-free execution of the equals
and hashCode
methods.
In the next chapter, we’ll discuss error handling strategies for debugging common issues.
Related Article: How to Generate Random Integers in a Range in Java
Chapter 22: Error Handling: Debugging Common Issues
When implementing the equals
and hashCode
methods, it is important to handle common issues and debug them effectively. Here are some error handling strategies:
1. Use logging and debugging tools: Utilize logging frameworks and debugging tools to identify and debug common issues. Log relevant information and use breakpoints to step through the code and inspect variables.
2. Test with different scenarios: Test the equals
and hashCode
methods with different scenarios and edge cases to identify and fix common issues. Use test frameworks and assertions to automate the testing process.
3. Consult documentation and online resources: Refer to official documentation and online resources to understand common issues and their solutions. Communities and forums can provide valuable insights and guidance.
By using effective error handling strategies, you can debug common issues and ensure correct behavior in the equals
and hashCode
methods.