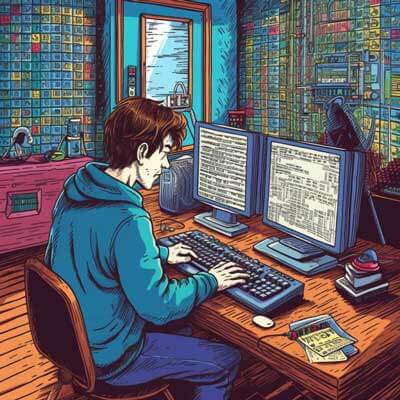
- Introduction to Regular Expressions
- Syntax of Regular Expressions
- Working with Patterns and Matchers
- Code Snippet: Digit Recognition
- Code Snippet: Word Boundary Matching
- Code Snippet: Email Validation
- Code Snippet: Password Strength Verification
- Code Snippet: URL Parsing
- Real World Use Case: Log File Analysis
- Real World Use Case: Data Scrubbing
- Real World Use Case: Text Parsing
- Best Practice: Using Precompiled Patterns
- Best Practice: Avoiding Catastrophic Backtracking
- Best Practice: Using Non-Capturing Groups
- Performance Consideration: Time Complexity
- Performance Consideration: Space Complexity
- Advanced Technique: Lookahead Assertions
- Advanced Technique: Lookbehind Assertions
- Error Handling: Common Regular Expression Errors
- Error Handling: Debugging Regular Expressions
Introduction to Regular Expressions
Regular expressions, often referred to as regex, provide a powerful and flexible way to search, match, and manipulate text. In Java, regular expressions are supported through the java.util.regex package. By using regex, you can perform complex pattern matching operations on strings, making it an essential tool for tasks such as data validation, text parsing, and data extraction.
Related Article: How To Parse JSON In Java
Syntax of Regular Expressions
Regular expressions are made up of characters and metacharacters that define a pattern to be matched. Here are some commonly used metacharacters in Java regex:
– .
: Matches any character except a newline.
– ^
: Matches the beginning of a line.
– $
: Matches the end of a line.
– *
: Matches zero or more occurrences of the preceding character or group.
– +
: Matches one or more occurrences of the preceding character or group.
– ?
: Matches zero or one occurrence of the preceding character or group.
– \
: Escapes special characters, allowing them to be treated as literals.
For example, the regular expression \d+
matches one or more digits. The backslash escapes the d
metacharacter to treat it as a literal digit.
Working with Patterns and Matchers
To use regular expressions in Java, you need to work with the Pattern
and Matcher
classes. The Pattern
class represents a compiled regex pattern, while the Matcher
class provides methods for matching patterns against input strings.
Here’s an example that demonstrates how to use Pattern
and Matcher
:
import java.util.regex.*; String input = "Hello, World!"; String regex = "World"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found!"); } else { System.out.println("No match found."); }
In this example, we create a Pattern
object by compiling the regex pattern “World”. We then create a Matcher
object and use the find()
method to search for a match in the input string. If a match is found, we print “Match found!”; otherwise, we print “No match found.”.
Code Snippet: Digit Recognition
Here’s an example of using regular expressions to recognize digits in a string:
import java.util.regex.*; String input = "The number is 123."; String regex = "\\d+"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(input); while (matcher.find()) { System.out.println("Digit found: " + matcher.group()); }
In this example, the regex pattern \\d+
matches one or more digits. The find()
method is used in a loop to find all occurrences of digits in the input string. The group()
method returns the matched digits.
Related Article: How To Convert Array To List In Java
Code Snippet: Word Boundary Matching
Word boundary matching can be useful when you want to match whole words in a text. Here’s an example:
import java.util.regex.*; String input = "Java is a programming language."; String regex = "\\bJava\\b"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found!"); } else { System.out.println("No match found."); }
In this example, the regex pattern \\bJava\\b
matches the word “Java” surrounded by word boundaries. The find()
method is used to search for a match in the input string. If a match is found, we print “Match found!”; otherwise, we print “No match found.”.
Code Snippet: Email Validation
Validating email addresses is a common use case for regular expressions. Here’s an example that demonstrates email validation:
import java.util.regex.*; String email = "test@example.com"; String regex = "^[A-Za-z0-9+_.-]+@(.+)$"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(email); if (matcher.matches()) { System.out.println("Valid email address."); } else { System.out.println("Invalid email address."); }
In this example, the regex pattern ^[A-Za-z0-9+_.-]+@(.+)$
matches a valid email address. The matches()
method is used to check if the entire input string matches the pattern. If it does, we print “Valid email address.”; otherwise, we print “Invalid email address.”.
Code Snippet: Password Strength Verification
Regular expressions can also be used to verify the strength of passwords. Here’s an example:
import java.util.regex.*; String password = "P@ssw0rd"; String regex = "^(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=])(?=\\S+$).{8,}$"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(password); if (matcher.matches()) { System.out.println("Strong password."); } else { System.out.println("Weak password."); }
In this example, the regex pattern ^(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=])(?=\S+$).{8,}$
matches a strong password that must contain at least one digit, one lowercase letter, one uppercase letter, one special character, and be at least 8 characters long. The matches()
method is used to check if the entire input string matches the pattern. If it does, we print “Strong password.”; otherwise, we print “Weak password.”.
Related Article: How To Iterate Over Entries In A Java Map
Code Snippet: URL Parsing
Regular expressions can be helpful for parsing URLs and extracting specific components. Here’s an example:
import java.util.regex.*; String url = "https://www.example.com/path/to/resource"; String regex = "^(https?)://([^/]+)(/.*)?$"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(url); if (matcher.matches()) { String protocol = matcher.group(1); String domain = matcher.group(2); String path = matcher.group(3); System.out.println("Protocol: " + protocol); System.out.println("Domain: " + domain); System.out.println("Path: " + path); } else { System.out.println("Invalid URL."); }
In this example, the regex pattern ^(https?)://([^/]+)(/.*)?$
matches a valid URL and captures the protocol, domain, and path components. The matches()
method is used to check if the entire input string matches the pattern. If it does, we use the group()
method to retrieve the captured components and print them out; otherwise, we print “Invalid URL.”.
Real World Use Case: Log File Analysis
Regular expressions are often used for log file analysis, where specific patterns need to be extracted from log entries. For example, suppose we have a log file with entries in the following format:
2022-01-01 10:00:00 INFO: Login successful for user: john_doe 2022-01-01 10:01:00 ERROR: File not found: /path/to/file.txt
We can use regular expressions to extract information such as the timestamp, log level, and relevant details from each log entry.
import java.util.regex.*; String logEntry = "2022-01-01 10:00:00 INFO: Login successful for user: john_doe"; String regex = "^(\\d{4}-\\d{2}-\\d{2} \\d{2}:\\d{2}:\\d{2}) (\\w+): (.+)$"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(logEntry); if (matcher.matches()) { String timestamp = matcher.group(1); String logLevel = matcher.group(2); String details = matcher.group(3); System.out.println("Timestamp: " + timestamp); System.out.println("Log Level: " + logLevel); System.out.println("Details: " + details); } else { System.out.println("Invalid log entry."); }
In this example, the regex pattern ^(\\d{4}-\\d{2}-\\d{2} \\d{2}:\\d{2}:\\d{2}) (\\w+): (.+)$
matches a log entry and captures the timestamp, log level, and details. The matches()
method is used to check if the entire input string matches the pattern. If it does, we use the group()
method to retrieve the captured components and print them out; otherwise, we print “Invalid log entry.”.
Real World Use Case: Data Scrubbing
Data scrubbing involves removing or replacing sensitive information from a dataset. Regular expressions can be used to identify and sanitize sensitive data such as credit card numbers, social security numbers, or email addresses. Here’s an example:
import java.util.regex.*; String input = "Please make a payment of $100 to 1234-5678-9012-3456."; String regex = "\\d{4}-\\d{4}-\\d{4}-\\d{4}"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(input); String sanitizedInput = matcher.replaceAll("[REDACTED]"); System.out.println("Sanitized Input: " + sanitizedInput);
In this example, the regex pattern \\d{4}-\\d{4}-\\d{4}-\\d{4}
matches a credit card number in the format XXXX-XXXX-XXXX-XXXX. The replaceAll()
method is used to replace the matched credit card number with the string “[REDACTED]”. The sanitized input is then printed out.
Related Article: How To Split A String In Java
Real World Use Case: Text Parsing
Regular expressions can be used for text parsing tasks such as extracting specific information from unstructured text. For example, suppose we have a string that contains multiple email addresses, and we want to extract all the email addresses from the string:
import java.util.regex.*; String input = "Contact us at info@example.com or support@example.com"; String regex = "\\b[A-Za-z0-9+_.-]+@(?:[A-Za-z0-9.-]+\\.[A-Za-z]{2,})\\b"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(input); while (matcher.find()) { System.out.println("Email address: " + matcher.group()); }
In this example, the regex pattern \\b[A-Za-z0-9+_.-]+@(?:[A-Za-z0-9.-]+\\.[A-Za-z]{2,})\\b
matches valid email addresses. The find()
method is used in a loop to find all occurrences of email addresses in the input string. The group()
method returns the matched email addresses, which are then printed out.
Best Practice: Using Precompiled Patterns
For improved performance, it is recommended to precompile regular expression patterns when they will be used multiple times. Here’s an example:
import java.util.regex.*; String input = "The quick brown fox jumps over the lazy dog."; Pattern pattern = Pattern.compile("quick"); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found!"); } else { System.out.println("No match found."); }
In this example, the regex pattern “quick” is compiled into a Pattern
object before it is used in the Matcher
. Precompiling the pattern allows for efficient reuse across multiple input strings.
Best Practice: Avoiding Catastrophic Backtracking
Catastrophic backtracking can occur when a regular expression matches the same part of a string multiple times in different ways. This can lead to significant performance issues. To avoid catastrophic backtracking, you can optimize your regular expressions by making them more specific and avoiding excessive use of quantifiers. Here’s an example:
import java.util.regex.*; String input = "aaaaaaaaaaaaaaaaaaaaaa"; Pattern pattern = Pattern.compile("a+b+"); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found!"); } else { System.out.println("No match found."); }
In this example, the regex pattern “a+b+” matches one or more “a” characters followed by one or more “b” characters. The input string consists of multiple “a” characters, which can lead to catastrophic backtracking. To avoid this, it is recommended to use more specific patterns that do not rely heavily on quantifiers.
Related Article: How To Convert Java Objects To JSON With Jackson
Best Practice: Using Non-Capturing Groups
Non-capturing groups can be used to group parts of a regular expression without capturing the matched text. This can be useful when you want to apply a quantifier to a group, but you don’t need to capture the matched text. Here’s an example:
import java.util.regex.*; String input = "Hello, World!"; Pattern pattern = Pattern.compile("(?:Hello, )+World!"); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found!"); } else { System.out.println("No match found."); }
In this example, the regex pattern “(?:Hello, )+World!” matches one or more occurrences of “Hello, ” followed by “World!”. The non-capturing group “(?:Hello, )” allows us to apply the “+” quantifier to the group without capturing the matched text.
Performance Consideration: Time Complexity
The time complexity of a regular expression can vary depending on the pattern and the input. Regular expressions that involve a lot of backtracking or nested quantifiers can have exponential time complexity, leading to performance issues. It is important to design efficient regular expressions to avoid unnecessary overhead. Additionally, you can optimize performance by using non-greedy quantifiers when appropriate.
Performance Consideration: Space Complexity
The space complexity of a regular expression refers to the amount of memory required to store the compiled pattern and perform the matching operation. While the space complexity of most regular expressions is relatively low, complex patterns with nested quantifiers or lookaheads/lookbehinds can result in increased memory usage. It is important to be mindful of the memory requirements of your regular expressions, especially when dealing with large input strings or processing a large number of patterns.
Related Article: Storing Contact Information in Java Data Structures
Advanced Technique: Lookahead Assertions
Lookahead assertions allow you to match a pattern only if it is followed by another pattern. This is useful when you want to match something based on its context without including the context in the match result. Here’s an example:
import java.util.regex.*; String input = "apple banana cherry"; Pattern pattern = Pattern.compile("\\w+(?=\\sbanana)"); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found: " + matcher.group()); } else { System.out.println("No match found."); }
In this example, the regex pattern “\\w+(?=\\sbanana)” matches one or more word characters that are followed by a space and the word “banana”. The lookahead assertion “(?=\\sbanana)” ensures that the matched word is followed by “banana” without including “banana” in the match result.
Advanced Technique: Lookbehind Assertions
Lookbehind assertions allow you to match a pattern only if it is preceded by another pattern. This is useful when you want to match something based on its context without including the context in the match result. Here’s an example:
import java.util.regex.*; String input = "apple banana cherry"; Pattern pattern = Pattern.compile("(?<=banana\\s)\\w+"); Matcher matcher = pattern.matcher(input); if (matcher.find()) { System.out.println("Match found: " + matcher.group()); } else { System.out.println("No match found."); }
In this example, the regex pattern “(?Advanced Technique: POSIX Character Classes
POSIX character classes provide a way to match characters based on their general category, such as letters, digits, or punctuation. In Java regex, you can use the \p{...}
syntax to match POSIX character classes. Here’s an example:
import java.util.regex.*; String input = "abc 123 !@#"; Pattern pattern = Pattern.compile("\\p{Alpha}+"); Matcher matcher = pattern.matcher(input); while (matcher.find()) { System.out.println("Match found: " + matcher.group()); }
In this example, the regex pattern “\\p{Alpha}+” matches one or more alphabetic characters. The \p{Alpha}
syntax is used to match any Unicode alphabetic character. The find()
method is used in a loop to find all occurrences of alphabetic characters in the input string. The group()
method returns the matched alphabetic characters, which are then printed out.
Error Handling: Common Regular Expression Errors
When working with regular expressions, it’s important to be aware of common errors that can occur. Here are some common errors and how to handle them:
– Invalid syntax: Regular expressions must follow specific syntax rules. If you encounter syntax errors, check your regex pattern for any typos or missing escape characters.
– Catastrophic backtracking: This occurs when a regex pattern matches the same part of a string multiple times in different ways, leading to performance issues. To avoid catastrophic backtracking, optimize your regex patterns by making them more specific and avoiding excessive use of quantifiers.
– Incorrect matching: Regular expressions can sometimes produce unexpected matches. Carefully review your regex pattern and ensure that it accurately represents the desired matching behavior.
– Incomplete matching: If your regex pattern is not capturing the desired parts of the input string, check for missing capturing groups or incorrect use of metacharacters.
Related Article: How to Convert JSON String to Java Object
Error Handling: Debugging Regular Expressions
Debugging regular expressions can be challenging due to their complex nature. Here are some techniques to help you debug regex patterns:
– Print intermediate results: Output intermediate results of your regex pattern matching to understand how it is being applied to the input string. This can help identify issues with the pattern.
– Use online regex testers: Online regex testers allow you to input your regex pattern and test it against sample input strings. They often provide explanations and highlights of the matches, helping you identify any issues.
– Break down complex patterns: If you have a complex regex pattern, break it down into smaller parts and test each part individually. This can help pinpoint specific parts of the pattern that may be causing issues.
– Consult documentation and resources: Regular expressions have a vast array of features and syntax. Consult the official documentation or reliable online resources to understand the nuances of specific regex constructs.