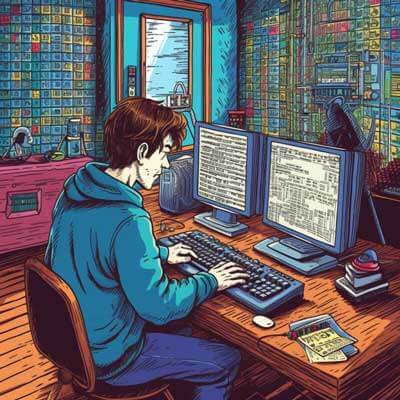
Table of Contents
Introduction to Singleton Design Pattern
The Singleton design pattern is a creational pattern that ensures a class has only one instance, and provides a global point of access to it. It is widely used in Java applications where only one instance of a class is needed to control actions that should not be handled by multiple instances. The Singleton pattern is particularly useful in scenarios where shared resources need to be managed, concurrent access must be controlled, or global state needs to be maintained.
Related Article: PHP vs Java: A Practical Comparison
Defining Singleton Class
To implement the Singleton pattern, a class must have a private constructor to prevent direct instantiation from external classes. It should provide a static method that returns the single instance of the class. The class should also contain a private static field to store the instance.
Code Snippet 1: Basic Singleton Class (Singleton.java)
public class Singleton { private static Singleton instance; private Singleton() { // private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
In the above example, the Singleton class has a private constructor and a static method getInstance()
that returns the single instance of the class. The instance is lazily initialized in the getInstance()
method, meaning it is created only when the method is called for the first time.
Basic Singleton Implementation
The basic implementation of the Singleton pattern allows lazy initialization of the instance, meaning the instance is created only when it is first requested. This approach is suitable for scenarios where the Singleton instance is not frequently used or when the initialization process is resource-intensive.
Code Snippet 2: Lazy Initialized Singleton (LazySingleton.java)
public class LazySingleton { private static LazySingleton instance; private LazySingleton() { // private constructor to prevent instantiation } public static synchronized LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } }
In the above example, the getInstance()
method is synchronized to ensure thread-safety in a multi-threaded environment. This prevents multiple threads from creating separate instances concurrently. However, this approach introduces performance overhead due to the synchronized keyword, even when the instance is already initialized.
Use Case 1: Managing Global State
One of the common use cases of the Singleton pattern is to manage global state within an application. This can include maintaining a cache, managing application configuration, or keeping track of user sessions.
Real World Example 1: Runtime Environment
In a Java application, the runtime environment is a global state that needs to be accessed from various parts of the codebase. By implementing the Singleton pattern, we can ensure that there is only one instance of the runtime environment and provide a centralized access point.
public class RuntimeEnvironment { private static RuntimeEnvironment instance; private RuntimeEnvironment() { // private constructor to prevent instantiation } public static synchronized RuntimeEnvironment getInstance() { if (instance == null) { instance = new RuntimeEnvironment(); } return instance; } public void initialize() { // perform initialization tasks } // other methods and properties }
In the above example, the RuntimeEnvironment
class follows the Singleton pattern and provides a method initialize()
to perform initialization tasks. Other parts of the application can access the runtime environment instance and utilize its functionality.
Real World Example 2: Logger Utility
A logger utility is another example where the Singleton pattern can be applied effectively. Logging is a common requirement in software applications for debugging, monitoring, and auditing purposes. By implementing the Singleton pattern, we can ensure that there is only one instance of the logger utility throughout the application.
public class Logger { private static Logger instance; private Logger() { // private constructor to prevent instantiation } public static synchronized Logger getInstance() { if (instance == null) { instance = new Logger(); } return instance; } public void log(String message) { // perform logging } // other logging methods and properties }
In the above example, the Logger
class follows the Singleton pattern and provides a method log()
to log messages. Other parts of the application can access the logger instance and use its logging capabilities.
Related Article: How To Convert Java Objects To JSON With Jackson
Use Case 2: Facilitating Resource Sharing
Another use case of the Singleton pattern is to facilitate resource sharing among multiple parts of an application. This can include database connections, file system access, or network connections.
Real World Example 3: Configuration Manager
In a Java application, a configuration manager is often used to manage application settings and properties. By implementing the Singleton pattern, we can ensure that there is only one instance of the configuration manager, enabling centralized access to configuration data.
public class ConfigurationManager { private static ConfigurationManager instance; private ConfigurationManager() { // private constructor to prevent instantiation } public static synchronized ConfigurationManager getInstance() { if (instance == null) { instance = new ConfigurationManager(); } return instance; } public String getProperty(String key) { // retrieve property value based on key } // other configuration management methods and properties }
In the above example, the ConfigurationManager
class follows the Singleton pattern and provides a method getProperty()
to retrieve configuration properties. Other parts of the application can access the configuration manager instance and obtain the required configuration data.
Use Case 3: Controlling Concurrent Access
Controlling concurrent access is another important aspect of the Singleton pattern. In multi-threaded environments, it is crucial to ensure that only one thread can access and modify the Singleton instance at a time.
Code Snippet 3: Thread-Safe Singleton (ThreadSafeSingleton.java)
public class ThreadSafeSingleton { private static ThreadSafeSingleton instance; private ThreadSafeSingleton() { // private constructor to prevent instantiation } public static ThreadSafeSingleton getInstance() { if (instance == null) { synchronized (ThreadSafeSingleton.class) { if (instance == null) { instance = new ThreadSafeSingleton(); } } } return instance; } }
In the above example, the getInstance()
method uses double-checked locking to ensure thread-safety. The synchronized block is applied only when the instance is not initialized, reducing the performance overhead compared to the synchronized keyword on the entire method.
Best Practice 1: Lazy Initialization
Lazy initialization is a common approach used in Singleton implementations to defer the creation of the instance until it is first requested. It allows for efficient resource utilization by creating the instance only when needed.
Code Snippet 2: Lazy Initialized Singleton (LazySingleton.java)
public class LazySingleton { private static LazySingleton instance; private LazySingleton() { // private constructor to prevent instantiation } public static synchronized LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } }
In the above code snippet, the getInstance()
method lazily initializes the instance by creating it only when instance
is null. However, this approach introduces performance overhead due to the synchronized keyword, even when the instance is already initialized.
Related Article: How To Convert String To Int In Java
Code Snippet 4: Enum Singleton (EnumSingleton.java)
public enum EnumSingleton { INSTANCE; // other methods and properties }
An alternative approach to lazy initialization is using an enumeration to implement the Singleton pattern. Enum singletons guarantee that only one instance is created and provide serialization safety out of the box. Enum singletons are also immune to reflection attacks and handle serialization and deserialization without any additional code.
Best Practice 2: Thread Safety
Thread safety is an essential consideration when implementing a Singleton pattern in a multi-threaded environment. Ensuring that only one instance is created and accessed concurrently requires synchronization mechanisms to prevent race conditions and inconsistent state.
Code Snippet 3: Thread-Safe Singleton (ThreadSafeSingleton.java)
public class ThreadSafeSingleton { private static ThreadSafeSingleton instance; private ThreadSafeSingleton() { // private constructor to prevent instantiation } public static ThreadSafeSingleton getInstance() { if (instance == null) { synchronized (ThreadSafeSingleton.class) { if (instance == null) { instance = new ThreadSafeSingleton(); } } } return instance; } }
In the above code snippet, the getInstance()
method uses double-checked locking to ensure thread-safety. The synchronized block is applied only when the instance is not initialized, reducing the performance overhead compared to the synchronized keyword on the entire method.
Best Practice 3: Serialization-safe Singleton
Serialization introduces additional challenges when implementing a Singleton pattern. Without proper measures, deserialization can create new instances and break the Singleton contract. To ensure serialization safety, the Singleton class needs to implement the Serializable
interface and provide custom serialization and deserialization methods.
Code Snippet 4: Enum Singleton (EnumSingleton.java)
public enum EnumSingleton implements Serializable { INSTANCE; // other methods and properties private Object readResolve() throws ObjectStreamException { return INSTANCE; } }
In the above code snippet, the EnumSingleton
class is made serializable by implementing the Serializable
interface. The readResolve()
method is used to ensure that deserialization returns the same instance instead of creating a new one. This guarantees the Singleton contract even after serialization and deserialization.
Related Article: How to Use Spring Configuration Annotation
Real World Example 1: Runtime Environment
In a Java application, the runtime environment is a global state that needs to be accessed from various parts of the codebase. By implementing the Singleton pattern, we can ensure that there is only one instance of the runtime environment and provide a centralized access point.
Code Snippet 2: Lazy Initialized Singleton (LazyInitializedRuntimeEnvironment.java)
public class LazyInitializedRuntimeEnvironment { private static LazyInitializedRuntimeEnvironment instance; private LazyInitializedRuntimeEnvironment() { // private constructor to prevent instantiation } public static synchronized LazyInitializedRuntimeEnvironment getInstance() { if (instance == null) { instance = new LazyInitializedRuntimeEnvironment(); } return instance; } public void initialize() { // perform initialization tasks } // other methods and properties }
In the above code snippet, the LazyInitializedRuntimeEnvironment
class follows the Singleton pattern and provides a method initialize()
to perform initialization tasks. Other parts of the application can access the runtime environment instance and utilize its functionality.
Real World Example 2: Logger Utility
A logger utility is another example where the Singleton pattern can be applied effectively. Logging is a common requirement in software applications for debugging, monitoring, and auditing purposes. By implementing the Singleton pattern, we can ensure that there is only one instance of the logger utility throughout the application.
Code Snippet 5: Double-Checked Locking Singleton (DoubleCheckedLockingLogger.java)
public class DoubleCheckedLockingLogger { private static volatile DoubleCheckedLockingLogger instance; private DoubleCheckedLockingLogger() { // private constructor to prevent instantiation } public static DoubleCheckedLockingLogger getInstance() { if (instance == null) { synchronized (DoubleCheckedLockingLogger.class) { if (instance == null) { instance = new DoubleCheckedLockingLogger(); } } } return instance; } public void log(String message) { // perform logging } // other logging methods and properties }
In the above code snippet, the DoubleCheckedLockingLogger
class follows the Singleton pattern and provides a method log()
to log messages. The double-checked locking technique is used to ensure thread-safety and efficient resource utilization.
Performance Consideration 1: Memory Overhead
One of the performance considerations when using the Singleton pattern is the memory overhead. Since the Singleton instance is stored in memory throughout the application's lifecycle, it can consume a significant amount of memory if it holds large data structures or caches.
Code Snippet 1: Basic Singleton Class (Singleton.java)
public class Singleton { private static Singleton instance; private Singleton() { // private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
In the above code snippet, the Singleton
class has a static field instance
that holds the single instance of the class. This instance remains in memory as long as the application is running, potentially consuming memory resources.
Related Article: Java Constructor Tutorial
Performance Consideration 2: Initialization Overhead
Another performance consideration is the initialization overhead of the Singleton instance. If the initialization process is resource-intensive or time-consuming, it can impact the application's startup time and overall performance.
Code Snippet 2: Lazy Initialized Singleton (LazySingleton.java)
public class LazySingleton { private static LazySingleton instance; private LazySingleton() { // private constructor to prevent instantiation } public static synchronized LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } }
In the above code snippet, the getInstance()
method of the LazySingleton
class lazily initializes the instance. If the initialization process involves heavy computations or resource allocations, it can introduce additional overhead during the first access to the Singleton instance.
Performance Consideration 3: Thread Contention
Thread contention can occur when multiple threads attempt to access the Singleton instance simultaneously. Synchronization mechanisms used to ensure thread-safety, such as the synchronized keyword or locks, can introduce performance overhead due to contention.
Code Snippet 3: Thread-Safe Singleton (ThreadSafeSingleton.java)
public class ThreadSafeSingleton { private static ThreadSafeSingleton instance; private ThreadSafeSingleton() { // private constructor to prevent instantiation } public static ThreadSafeSingleton getInstance() { if (instance == null) { synchronized (ThreadSafeSingleton.class) { if (instance == null) { instance = new ThreadSafeSingleton(); } } } return instance; } }
In the above code snippet, the getInstance()
method of the ThreadSafeSingleton
class uses double-checked locking to ensure thread-safety. However, the synchronized block can introduce contention if multiple threads try to access the instance simultaneously, leading to performance degradation.
Advanced Technique 1: Singleton with Enum
An advanced technique to implement the Singleton pattern in Java is by using an enumeration. Enum singletons guarantee that only one instance is created, provide serialization safety, and handle thread-safety without additional code.
Code Snippet 4: Enum Singleton (EnumSingleton.java)
public enum EnumSingleton { INSTANCE; // other methods and properties }
In the above code snippet, the EnumSingleton
enum class represents the Singleton instance. The INSTANCE
enum constant ensures that only one instance is created. Enum singletons are automatically thread-safe, serialization-safe, and immune to reflection attacks.
Related Article: How to Use the JsonProperty in Java
Advanced Technique 2: Double-Checked Locking
Double-checked locking is an advanced technique to achieve thread-safety and efficient resource utilization in a Singleton implementation. It optimizes the synchronization mechanism by applying it only when the instance is not initialized.
Code Snippet 5: Double-Checked Locking Singleton (DoubleCheckedLockingSingleton.java)
public class DoubleCheckedLockingSingleton { private static volatile DoubleCheckedLockingSingleton instance; private DoubleCheckedLockingSingleton() { // private constructor to prevent instantiation } public static DoubleCheckedLockingSingleton getInstance() { if (instance == null) { synchronized (DoubleCheckedLockingSingleton.class) { if (instance == null) { instance = new DoubleCheckedLockingSingleton(); } } } return instance; } }
In the above code snippet, the getInstance()
method of the DoubleCheckedLockingSingleton
class uses double-checked locking to ensure thread-safety. The synchronized block is applied only when the instance is not initialized, reducing the performance overhead compared to the synchronized keyword on the entire method.
Code Snippet 1: Basic Singleton Class (Singleton.java)
public class Singleton { private static Singleton instance; private Singleton() { // private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
The above code snippet shows a basic implementation of the Singleton pattern in Java. The Singleton class has a private constructor to prevent direct instantiation and a static method getInstance()
that returns the single instance of the class. The instance is lazily initialized in the getInstance()
method, meaning it is created only when the method is called for the first time.
Code Snippet 2: Lazy Initialized Singleton (LazySingleton.java)
public class LazySingleton { private static LazySingleton instance; private LazySingleton() { // private constructor to prevent instantiation } public static synchronized LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } }
The above code snippet demonstrates a lazy initialized Singleton implementation. The getInstance()
method is synchronized to ensure thread-safety in a multi-threaded environment. This prevents multiple threads from creating separate instances concurrently. However, this approach introduces performance overhead due to the synchronized keyword, even when the instance is already initialized.
Code Snippet 3: Thread-Safe Singleton (ThreadSafeSingleton.java)
public class ThreadSafeSingleton { private static ThreadSafeSingleton instance; private ThreadSafeSingleton() { // private constructor to prevent instantiation } public static ThreadSafeSingleton getInstance() { if (instance == null) { synchronized (ThreadSafeSingleton.class) { if (instance == null) { instance = new ThreadSafeSingleton(); } } } return instance; } }
The above code snippet illustrates a thread-safe implementation of the Singleton pattern using double-checked locking. The getInstance()
method uses synchronized blocks to ensure thread-safety. The outer if
statement checks if the instance is already initialized before acquiring the lock, reducing the performance overhead compared to using the synchronized keyword on the entire method.
Related Article: Tutorial: Sorted Data Structure Storage in Java
Handling Errors in Singleton Implementation
When implementing the Singleton pattern, it is important to handle potential errors or exceptions that may occur during the initialization or usage of the Singleton instance. Proper error handling ensures the robustness and reliability of the application.
Code Snippet 1: Basic Singleton Class (Singleton.java)
public class Singleton { private static Singleton instance; private Singleton() { // private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { try { instance = new Singleton(); } catch (Exception e) { // handle initialization error } } return instance; } }
In the above code snippet, the getInstance()
method of the Singleton
class includes error handling to catch any exceptions that may occur during the initialization of the instance. This allows for proper handling of initialization errors and prevents the application from crashing or entering an inconsistent state.
Testing Singleton Classes
Testing Singleton classes can be challenging due to their global state and the difficulty of creating multiple instances for different test scenarios. However, various techniques and approaches can be used to effectively test Singleton classes.
Code Snippet 1: Basic Singleton Class (Singleton.java)
public class Singleton { private static Singleton instance; private Singleton() { // private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
In the above code snippet, the Singleton
class has a static method getInstance()
that returns the single instance of the class. To test the Singleton class, we can write test cases that cover different scenarios, such as checking if the returned instance is the same for multiple calls to getInstance()
or verifying the behavior of the Singleton instance in different usage scenarios.
Overall, testing Singleton classes requires careful consideration of the global state and proper design of test cases to cover different aspects of the Singleton behavior.