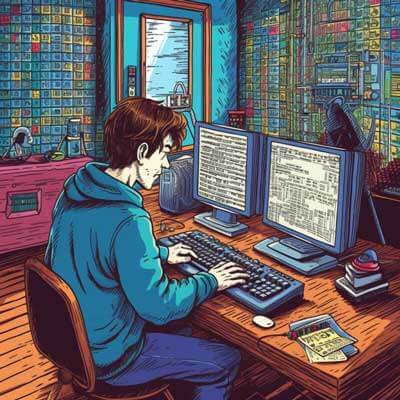
Table of Contents
Introduction to Comparable and Comparator
Related Article: How to Retrieve Current Date and Time in Java
Comparable
Comparable is an interface in Java that allows objects of a class to be compared to each other. It provides a natural ordering for the objects by implementing the compareTo()
method. This method compares the current object with the specified object and returns a negative integer, zero, or a positive integer depending on the comparison result.
Here's an example of implementing the Comparable interface for a class named Person
:
public class Person implements Comparable<Person> { private String name; private int age; // constructor and other methods @Override public int compareTo(Person otherPerson) { return this.age - otherPerson.age; } }
In this example, the compareTo()
method compares the ages of two Person
objects and returns the difference. This allows a list of Person
objects to be sorted based on their ages.
Comparator
Comparator is an interface in Java that provides an alternative way to compare objects. Unlike Comparable, which is implemented by the objects themselves, Comparator is implemented as a separate class. It allows for custom comparisons between objects of a class by implementing the compare()
method.
Here's an example of implementing a Comparator for the Person
class to compare based on the names:
public class NameComparator implements Comparator<Person> { @Override public int compare(Person person1, Person person2) { return person1.getName().compareTo(person2.getName()); } }
In this example, the compare()
method compares the names of two Person
objects using the compareTo()
method of the String class. This allows for sorting a list of Person
objects based on their names.
Use Case: Sorting a List with Comparable
Related Article: How to Change the Date Format in a Java String
Example 1: Sorting a List of Integers
Here's an example of sorting a list of integers using the Comparable interface:
List<Integer> numbers = new ArrayList<>(); numbers.add(5); numbers.add(2); numbers.add(8); numbers.add(1); Collections.sort(numbers); System.out.println(numbers); // Output: [1, 2, 5, 8]
In this example, the Collections.sort()
method is used to sort the list of integers in ascending order. The sort operation is possible because the Integer class implements the Comparable interface.
Example 2: Sorting a List of Strings
Here's an example of sorting a list of strings using the Comparable interface:
List<String> names = new ArrayList<>(); names.add("John"); names.add("Alice"); names.add("Bob"); names.add("Carol"); Collections.sort(names); System.out.println(names); // Output: [Alice, Bob, Carol, John]
In this example, the list of strings is sorted in lexicographic order (alphabetical order) using the Comparable interface implemented by the String class.
Use Case: Sorting a List with Comparator
Example 1: Sorting a List of Objects by a Field
Here's an example of sorting a list of objects based on a specific field using a Comparator:
List<Person> people = new ArrayList<>(); people.add(new Person("John", 25)); people.add(new Person("Alice", 30)); people.add(new Person("Bob", 20)); Comparator<Person> ageComparator = Comparator.comparingInt(Person::getAge); Collections.sort(people, ageComparator); System.out.println(people); // Output: [Bob (20), John (25), Alice (30)]
In this example, the list of Person
objects is sorted based on the age
field using a Comparator created with the comparingInt()
method. The comparingInt()
method takes a function that extracts the age from a Person
object.
Related Article: Java List Tutorial
Example 2: Sorting a List of Objects by Multiple Fields
Here's an example of sorting a list of objects by multiple fields using a Comparator:
List<Person> people = new ArrayList<>(); people.add(new Person("John", 25)); people.add(new Person("Alice", 30)); people.add(new Person("Bob", 20)); Comparator<Person> nameAgeComparator = Comparator.comparing(Person::getName) .thenComparingInt(Person::getAge); Collections.sort(people, nameAgeComparator); System.out.println(people); // Output: [Alice (30), Bob (20), John (25)]
In this example, the list of Person
objects is sorted first by the name
field and then by the age
field using the thenComparing()
method. This allows for sorting by multiple fields in the desired order.
Best Practice: Using Comparable for Natural Ordering
The Comparable interface is commonly used for achieving natural ordering of objects. Natural ordering refers to the default ordering that makes sense for the objects based on their intrinsic properties. For example, numbers can be naturally ordered in ascending or descending order, and strings can be naturally ordered in lexicographic order.
When implementing the Comparable interface, the compareTo() method should be implemented in a way that reflects the natural ordering of the objects. Here's an example of implementing Comparable for a class named Product
:
public class Product implements Comparable<Product> { private String name; private double price; // constructor and other methods @Override public int compareTo(Product otherProduct) { return Double.compare(this.price, otherProduct.price); } }
In this example, the compareTo()
method compares the prices of two Product
objects using the compare()
method of the Double class. This allows a list of Product
objects to be sorted based on their prices.
By implementing Comparable, objects of the class can be easily sorted using the Collections.sort()
method or any other sorting algorithm that relies on natural ordering.
Best Practice: Using Comparator for Custom Ordering
The Comparator interface is useful when the natural ordering of objects is not appropriate or when custom ordering is required. It allows for the comparison of objects based on specific criteria defined by the developer.
When using a Comparator, a separate class is implemented to encapsulate the custom comparison logic. Here's an example of implementing a Comparator for a class named Book
to compare based on the number of pages:
public class PageComparator implements Comparator<Book> { @Override public int compare(Book book1, Book book2) { return book1.getPages() - book2.getPages(); } }
In this example, the compare()
method compares the number of pages of two Book
objects and returns the difference. This allows for sorting a list of Book
objects based on the number of pages.
By using a Comparator, the sorting behavior can be customized without modifying the class itself. It provides flexibility in defining different sorting criteria for the same class.
Real World Example: Sorting Employee Objects
Sorting employee objects is a common use case where Comparable and Comparator can be applied to achieve sorting based on specific attributes such as name, age, or salary. Here's an example of sorting a list of employee objects based on their salaries:
public class Employee implements Comparable<Employee> { private String name; private int age; private double salary; // constructor and other methods @Override public int compareTo(Employee otherEmployee) { return Double.compare(this.salary, otherEmployee.salary); } } public class SalaryComparator implements Comparator<Employee> { @Override public int compare(Employee employee1, Employee employee2) { return Double.compare(employee1.getSalary(), employee2.getSalary()); } } // Sorting using Comparable List<Employee> employees = new ArrayList<>(); employees.add(new Employee("John", 25, 5000.0)); employees.add(new Employee("Alice", 30, 6000.0)); employees.add(new Employee("Bob", 20, 4000.0)); Collections.sort(employees); System.out.println(employees); // Output: [Bob (4000.0), John (5000.0), Alice (6000.0)] // Sorting using Comparator Comparator<Employee> salaryComparator = new SalaryComparator(); Collections.sort(employees, salaryComparator); System.out.println(employees); // Output: [Bob (4000.0), John (5000.0), Alice (6000.0)]
In this example, the Employee
class implements the Comparable interface to enable sorting based on the salary attribute. Additionally, a separate SalaryComparator class is implemented to allow sorting by salary using a Comparator.
Related Article: How To Fix Java Certification Path Error
Real World Example: Sorting Date Objects
Sorting date objects is another common use case where Comparable and Comparator can be utilized to achieve a desired ordering. Here's an example of sorting a list of date objects in ascending order:
public class DateComparator implements Comparator<Date> { @Override public int compare(Date date1, Date date2) { return date1.compareTo(date2); } } // Sorting using Comparable List<Date> dates = new ArrayList<>(); dates.add(new Date(2022, 1, 1)); dates.add(new Date(2021, 12, 31)); dates.add(new Date(2023, 1, 1)); Collections.sort(dates); System.out.println(dates); // Output: [2021-12-31, 2022-01-01, 2023-01-01] // Sorting using Comparator Comparator<Date> dateComparator = new DateComparator(); Collections.sort(dates, dateComparator); System.out.println(dates); // Output: [2021-12-31, 2022-01-01, 2023-01-01]
In this example, the DateComparator
class is implemented to compare two Date
objects using the compareTo()
method provided by the Date class. The list of date objects is then sorted using both the Comparable interface and the Comparator.
By utilizing Comparable and Comparator, date objects can be sorted in different orders or based on different criteria, allowing for flexibility in date sorting.
Performance Consideration: Comparable vs Comparator
When it comes to performance, there is no significant difference between using Comparable and Comparator. Both interfaces serve the purpose of comparing objects, and the choice between them depends on the specific requirements of the application.
Comparable is suitable for achieving natural ordering, where the comparison logic is intrinsic to the class and should be the default behavior. It allows for objects to be sorted without explicitly specifying a comparison strategy.
Comparator, on the other hand, provides flexibility in defining custom comparison logic. It is suitable when the default natural ordering is not appropriate or when multiple sorting criteria are required.
In terms of performance, the choice between Comparable and Comparator does not have a significant impact. The performance of the comparison logic itself is more important rather than the interface used. It is advised to focus on writing efficient comparison logic rather than worrying about the performance difference between Comparable and Comparator.
Performance Consideration: Sorting Efficiency
The efficiency of sorting algorithms is a crucial consideration when sorting large collections of objects. The choice of sorting algorithm can have a significant impact on the performance of sorting operations.
Java provides the Collections.sort()
method, which internally uses the TimSort algorithm. The TimSort algorithm is a hybrid sorting algorithm derived from merge sort and insertion sort. It offers good performance for most use cases and is generally efficient.
However, for certain scenarios where the number of elements is large or the sorting requirements are specific, it may be beneficial to consider alternative sorting algorithms. Some popular sorting algorithms include QuickSort, HeapSort, and RadixSort.
It is important to analyze the specific requirements and data characteristics of the application to choose the most suitable sorting algorithm. Additionally, optimizing the comparison logic and minimizing unnecessary comparisons can also contribute to improved sorting efficiency.
Advanced Technique: Using Comparator with Lambda Expressions
Lambda expressions introduced in Java 8 provide a concise way to create instances of functional interfaces, such as Comparator, without explicitly implementing the interface.
Here's an example of using a lambda expression to create a Comparator for sorting a list of strings based on their lengths:
List<String> names = new ArrayList<>(); names.add("John"); names.add("Alice"); names.add("Bob"); names.add("Carol"); Comparator<String> lengthComparator = (s1, s2) -> s1.length() - s2.length(); Collections.sort(names, lengthComparator); System.out.println(names); // Output: [Bob, John, Alice, Carol]
In this example, the lambda expression (s1, s2) -> s1.length() - s2.length()
represents a Comparator that compares two strings based on their lengths. The Comparator is then used to sort the list of names.
Lambda expressions provide a more concise and readable way to create Comparators, especially for simple comparison logic. They eliminate the need for creating separate Comparator classes and implementing the compare() method.
Related Article: Java Composition Tutorial
Advanced Technique: Using Comparator.thenComparing() Method
The thenComparing()
method provided by the Comparator interface allows for sorting by multiple criteria. It is useful when the primary comparison is not sufficient or when additional sorting levels are required.
Here's an example of using the thenComparing()
method to sort a list of Person objects by their names and ages:
List<Person> people = new ArrayList<>(); people.add(new Person("John", 25)); people.add(new Person("Alice", 30)); people.add(new Person("Bob", 20)); Comparator<Person> nameAgeComparator = Comparator.comparing(Person::getName) .thenComparingInt(Person::getAge); Collections.sort(people, nameAgeComparator); System.out.println(people); // Output: [Alice (30), Bob (20), John (25)]
In this example, the thenComparing()
method is used to specify a secondary level of comparison after comparing the names of the Person objects. The thenComparingInt()
method allows for comparing the ages as the secondary criterion.
By using the thenComparing()
method, sorting can be performed based on multiple criteria, providing more granular control over the sorting order.
Code Snippet: Implementing Comparable Interface
Here's a code snippet demonstrating the implementation of the Comparable interface for a class named Student
:
public class Student implements Comparable<Student> { private String name; private int age; // constructor and other methods @Override public int compareTo(Student otherStudent) { return this.name.compareTo(otherStudent.name); } }
In this code snippet, the compareTo()
method compares the names of two Student
objects using the compareTo()
method of the String class. This allows for sorting a list of Student
objects based on their names.
The Comparable interface is implemented by specifying the type parameter <Student>
after the class name.
Code Snippet: Implementing Comparator Interface
Here's a code snippet demonstrating the implementation of the Comparator interface for a class named Car
:
public class Car { private String brand; private int year; // constructor and other methods // Getter and Setter methods // Other methods // Comparator implementation public static Comparator<Car> brandComparator = Comparator.comparing(Car::getBrand); }
In this code snippet, a Comparator for the Car
class is implemented as a static field named brandComparator
. The brandComparator
uses the comparing()
method to compare the brand names of two Car
objects.
The comparing()
method takes a function that extracts the brand name from a Car
object using a method reference (Car::getBrand
). This allows for sorting a list of Car
objects based on their brand names.
Code Snippet: Using Comparator with Anonymous Class
Here's a code snippet demonstrating the usage of a Comparator with an anonymous class for sorting a list of Person
objects based on their ages:
List<Person> people = new ArrayList<>(); people.add(new Person("John", 25)); people.add(new Person("Alice", 30)); people.add(new Person("Bob", 20)); Comparator<Person> ageComparator = new Comparator<Person>() { @Override public int compare(Person person1, Person person2) { return person1.getAge() - person2.getAge(); } }; Collections.sort(people, ageComparator); System.out.println(people); // Output: [Bob (20), John (25), Alice (30)]
In this code snippet, an anonymous class is used to implement the Comparator interface. The anonymous class overrides the compare()
method to compare the ages of two Person
objects.
The Comparator is then used to sort the list of Person
objects using the Collections.sort()
method.
Related Article: How to Read a JSON File in Java Using the Simple JSON Lib
Code Snippet: Using Comparator with Lambda Expressions
Here's a code snippet demonstrating the usage of a Comparator with lambda expressions for sorting a list of Product
objects based on their prices:
List<Product> products = new ArrayList<>(); products.add(new Product("Laptop", 999.99)); products.add(new Product("Phone", 699.99)); products.add(new Product("Tablet", 499.99)); Comparator<Product> priceComparator = (product1, product2) -> Double.compare(product1.getPrice(), product2.getPrice()); Collections.sort(products, priceComparator); System.out.println(products); // Output: [Tablet ($499.99), Phone ($699.99), Laptop ($999.99)]
In this code snippet, a lambda expression is used to create a Comparator that compares the prices of two Product
objects. The lambda expression (product1, product2) -> Double.compare(product1.getPrice(), product2.getPrice())
represents the compare() method of the Comparator interface.
The Comparator is then used to sort the list of Product
objects using the Collections.sort()
method.
Error Handling: Common Issues with Comparable
When implementing the Comparable interface, there are a few common issues that developers may encounter:
1. Inconsistent comparison logic: The compareTo() method should follow the contract defined by the Comparable interface. It should return a negative integer, zero, or a positive integer depending on whether the current object is less than, equal to, or greater than the specified object, respectively. Inconsistent comparison logic can lead to unexpected sorting results.
2. Null handling: The compareTo() method should handle null values gracefully to avoid NullPointerExceptions. It is recommended to explicitly handle null values by checking for null references before performing comparisons.
3. Incompatible types: The compareTo() method should only be implemented for objects of the same type. Attempting to compare objects of different types can result in ClassCastException.
4. Inefficient comparison logic: The compareTo() method should be implemented efficiently to avoid unnecessary operations or unnecessary object creations. Inefficient comparison logic can impact the performance of sorting operations.
Error Handling: Common Issues with Comparator
When using the Comparator interface, developers may encounter the following common issues:
1. Inconsistent comparison logic: The compare() method of the Comparator interface should follow the contract defined by the interface. It should return a negative integer, zero, or a positive integer depending on whether the first argument is less than, equal to, or greater than the second argument, respectively. Inconsistent comparison logic can lead to unexpected sorting results.
2. Null handling: The compare() method should handle null values gracefully to avoid NullPointerExceptions. It is recommended to explicitly handle null values by checking for null references before performing comparisons.
3. Incorrect use of method references or lambda expressions: When using method references or lambda expressions to create Comparators, it is important to ensure that the expressions correctly capture the desired comparison logic. Incorrect use of method references or lambda expressions can lead to compilation errors or incorrect sorting results.
4. Inefficient comparison logic: The compare() method should be implemented efficiently to avoid unnecessary operations or unnecessary object creations. Inefficient comparison logic can impact the performance of sorting operations.