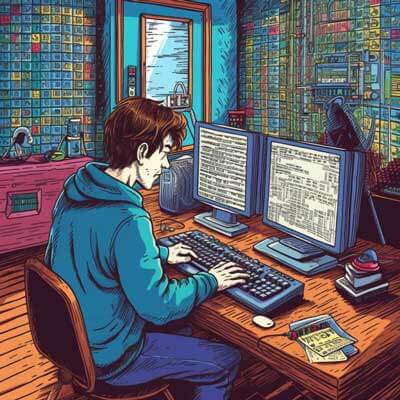
To convert a JSON string to a Java object, you can follow the steps outlined below:
Step 1: Create a Java Class
First, you need to create a Java class that represents the structure of the JSON object. The class should have fields that correspond to the keys in the JSON object. You can use libraries like Jackson, Gson, or JSON-B to automatically map the JSON object to Java objects.
Here’s an example of a Java class representing a JSON object:
public class Person { private String name; private int age; private String email; // getters and setters }
Related Article: How To Parse JSON In Java
Step 2: Choose a JSON Parsing Library
Next, you need to choose a JSON parsing library to parse the JSON string and convert it into a Java object. Some popular JSON parsing libraries in Java include Jackson, Gson, and JSON-B.
Step 3: Parse the JSON String
Once you’ve chosen a JSON parsing library, you can use its API to parse the JSON string and convert it into a Java object. The exact steps may vary depending on the library you choose.
Here’s an example using the Jackson library:
import com.fasterxml.jackson.databind.ObjectMapper; String jsonString = "{\"name\":\"John\", \"age\":30, \"email\":\"john@example.com\"}"; ObjectMapper objectMapper = new ObjectMapper(); Person person = objectMapper.readValue(jsonString, Person.class);
In this example, we use the ObjectMapper
class from the Jackson library to parse the JSON string and convert it into a Person
object.
Step 4: Handle Exceptions
When converting a JSON string to a Java object, it’s important to handle exceptions that may occur during the parsing process. JSON parsing libraries typically throw exceptions if the JSON string is malformed or if there is a problem with the mapping between the JSON object and the Java class.
Here’s an example of how to handle exceptions when using the Jackson library:
import com.fasterxml.jackson.databind.ObjectMapper; String jsonString = "{\"name\":\"John\", \"age\":30, \"email\":\"john@example.com\"}"; ObjectMapper objectMapper = new ObjectMapper(); try { Person person = objectMapper.readValue(jsonString, Person.class); // Do something with the person object } catch (Exception e) { // Handle the exception e.printStackTrace(); }
Related Article: How To Convert Array To List In Java
Step 5: Best Practices and Alternative Ideas
When converting JSON strings to Java objects, it’s important to follow some best practices to ensure the process is efficient and reliable:
– Validate the JSON string before parsing: Before attempting to parse the JSON string, you can use a JSON validator or schema to validate the string’s structure. This can help prevent errors during the parsing process.
– Use a library that supports annotations: Some JSON parsing libraries, like Jackson and Gson, support annotations that allow you to customize the mapping between the JSON object and the Java class. Annotations can simplify the mapping process and make it more flexible.
– Consider performance implications: JSON parsing can be resource-intensive, especially for large JSON strings. If performance is a concern, you can use streaming APIs provided by some JSON parsing libraries to process the JSON string incrementally without loading the entire string into memory.
– Use immutable objects: To ensure the integrity of the converted Java objects, it’s a good practice to make them immutable. Immutable objects are thread-safe and cannot be modified once created, which can help prevent unexpected changes to the object’s state.
– Consider using JSON-B: JSON-B is a standard JSON binding library introduced in Java EE 8. It provides a standardized way to convert JSON strings to Java objects and vice versa. If you’re working on a Java EE project, using JSON-B can provide a consistent and standardized approach to JSON parsing.