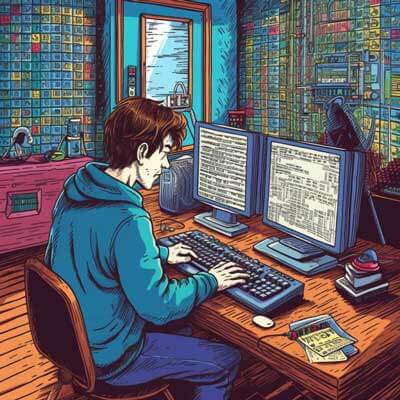
- Introduction
- Ecosystems Comparison
- PHP Ecosystem
- Java Ecosystem
- Web Development: A Comparative Analysis
- PHP Web Development
- Java Web Development
- Exploring Active Libraries
- PHP Libraries
- Java Libraries
- Productivity Metrics: A Comparative Study
- PHP Productivity Metrics
- Java Productivity Metrics
- Performance Metrics: A Comparative Analysis
- PHP Performance Metrics
- Java Performance Metrics
- Third-Party Tools: A Comparative Study
- PHP Third-Party Tools
- Java Third-Party Tools
- Security Considerations in Both Languages
- PHP Security Considerations
- Java Security Considerations
- Scalability of Web Applications: A Comparative Study
- PHP Web Application Scalability
- Java Web Application Scalability
- Use Cases: Language Selection Based on Requirements
- PHP Use Cases
- Java Use Cases
- Best Practices in Both Languages
- PHP Best Practices
- Java Best Practices
- Real-World Examples: Success Stories
- PHP Success Story: WordPress
- Java Success Story: Twitter
- Advanced Techniques in Web Development: A Comparative Study
- PHP Advanced Techniques
- Java Advanced Techniques
- Code Snippet: Database Connection
- PHP Code Snippet
- Java Code Snippet
- Code Snippet: User Authentication
- PHP Code Snippet
- Java Code Snippet
- Code Snippet: Data Validation
- PHP Code Snippet
- Java Code Snippet
- Code Snippet: Session Management
- PHP Code Snippet
- Java Code Snippet
- Error Handling: Best Practices
- PHP Error Handling Best Practices
- Java Error Handling Best Practices
Introduction
PHP and Java are two popular programming languages that are widely used for web development. While both languages have their own strengths and weaknesses, it is essential to understand their differences to make an informed decision when choosing the right language for your project. This article aims to provide a practical comparison of PHP and Java, exploring various aspects such as ecosystems, web development, libraries, productivity metrics, performance metrics, third-party tools, security considerations, scalability of web applications, use cases, best practices, real-world examples, advanced techniques, and error handling.
Related Article: Spring Boot Integration with Payment, Communication Tools
Ecosystems Comparison
PHP and Java have vibrant ecosystems with extensive libraries, frameworks, and tools that facilitate development. Let’s compare the ecosystems of both languages:
PHP Ecosystem
The PHP ecosystem revolves around the PHP Hypertext Preprocessor, a server-side scripting language. It offers a wide range of frameworks, such as Laravel, Symfony, and CodeIgniter, that simplify web application development. These frameworks provide robust features for routing, database interaction, and template rendering. Additionally, PHP has a vast collection of open-source libraries available via Composer, a dependency management tool.
Java Ecosystem
The Java ecosystem is centered around the Java Development Kit (JDK), which provides a powerful set of tools for Java development. Java has several popular frameworks, such as Spring, Hibernate, and Struts, which offer comprehensive solutions for building enterprise-level applications. The ecosystem also includes a vast array of libraries that can be easily integrated into Java projects using build tools like Maven or Gradle.
Related Article: Java Spring Security Customizations & RESTful API Protection
Web Development: A Comparative Analysis
Web development in PHP and Java involves the creation of dynamic and interactive websites. Let’s compare the web development process in both languages:
PHP Web Development
PHP excels in web development, thanks to its simplicity and ease of use. It enables developers to embed PHP code directly into HTML, allowing for seamless integration. Below is an example of a PHP code snippet for connecting to a MySQL database:
connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully"; ?>
Java Web Development
Java web development involves the use of frameworks like Spring or JavaServer Faces (JSF) to build robust and scalable applications. Below is an example of a Java code snippet for connecting to a MySQL database using JDBC:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DatabaseConnection { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { Connection conn = DriverManager.getConnection(url, username, password); System.out.println("Connected successfully"); } catch (SQLException e) { System.out.println("Connection failed: " + e.getMessage()); } } }
Related Article: Tutorial on Integrating Redis with Spring Boot
Exploring Active Libraries
Both PHP and Java have a vast array of active libraries that enhance development efficiency. Let’s explore some popular libraries in each language:
PHP Libraries
– Laravel: A powerful and elegant PHP framework that provides features like routing, ORM, and caching.
– Symfony: A robust framework that follows the MVC pattern and offers a set of reusable components for rapid development.
– CodeIgniter: A lightweight framework known for its simplicity and speed, suitable for building small to medium-sized applications.
Java Libraries
– Spring: A comprehensive framework that provides solutions for dependency injection, MVC, security, and many other aspects of Java development.
– Hibernate: An object-relational mapping (ORM) library that simplifies database interaction and allows developers to work with Java objects instead of SQL queries.
– Struts: A popular framework for building enterprise-level applications, known for its robustness and scalability.
Related Article: Identifying the Version of Your MySQL-Connector-Java
Productivity Metrics: A Comparative Study
Productivity is a crucial factor when considering a programming language for development. Let’s compare the productivity metrics of PHP and Java:
PHP Productivity Metrics
PHP is known for its rapid development capabilities, primarily due to its simplicity and ease of use. The language allows developers to quickly prototype and build web applications, making it an excellent choice for small to medium-sized projects.
Java Productivity Metrics
Java, despite being a statically-typed language, offers a high level of productivity, especially for large-scale projects. It provides robust tools, frameworks, and libraries that streamline the development process. However, Java’s learning curve and verbosity may require more effort initially.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Performance Metrics: A Comparative Analysis
Performance is a critical aspect of any application. Let’s compare the performance metrics of PHP and Java:
PHP Performance Metrics
PHP’s performance heavily depends on the web server and the PHP interpreter used. With proper optimization and caching techniques, PHP can handle moderate traffic loads. However, it may struggle with highly concurrent scenarios due to its single-threaded nature.
Java Performance Metrics
Java is renowned for its performance and scalability. It runs on the Java Virtual Machine (JVM), which optimizes code execution and provides efficient memory management. Java’s multi-threading capabilities make it suitable for high-concurrency scenarios, ensuring excellent performance even under heavy loads.
Related Article: How to Connect Java with MySQL
Third-Party Tools: A Comparative Study
Third-party tools are essential for development efficiency. Let’s compare the availability and usefulness of third-party tools in PHP and Java:
PHP Third-Party Tools
– Composer: A dependency management tool for PHP that simplifies the installation and updating of libraries and packages.
– PHPUnit: A unit testing framework for PHP that enables developers to write and execute tests for their code.
– Xdebug: A debugging and profiling tool for PHP that helps identify and fix issues in code.
Java Third-Party Tools
– Maven: A build automation tool that simplifies project setup, dependency management, and deployment.
– JUnit: A widely-used testing framework for Java that allows developers to write and run tests to ensure code correctness.
– IntelliJ IDEA: A popular integrated development environment (IDE) that offers advanced features for Java development, including debugging, refactoring, and code completion.
Related Article: How to Read a JSON File in Java Using the Simple JSON Lib
Security Considerations in Both Languages
Ensuring the security of web applications is crucial. Let’s compare the security considerations in PHP and Java:
PHP Security Considerations
– Use parameterized queries or prepared statements to prevent SQL injection attacks.
– Sanitize and validate user input to mitigate cross-site scripting (XSS) vulnerabilities.
– Implement proper authentication and authorization mechanisms to protect against unauthorized access.
– Regularly update PHP and its dependencies to patch security vulnerabilities.
Java Security Considerations
– Use frameworks like Spring Security to handle authentication and authorization effectively.
– Apply input validation and output encoding to prevent XSS and other injection attacks.
– Employ secure coding practices, such as avoiding hardcoded passwords and using cryptographic algorithms correctly.
– Keep the Java Runtime Environment (JRE) up to date to benefit from the latest security patches.
Related Article: How to Use Spring Configuration Annotation
Scalability of Web Applications: A Comparative Study
Scalability is crucial for web applications, especially when anticipating high traffic or future growth. Let’s compare the scalability of web applications in PHP and Java:
PHP Web Application Scalability
PHP web applications can be scaled horizontally by adding more servers and utilizing load balancers. However, PHP’s single-threaded nature may limit performance in highly concurrent scenarios.
Java Web Application Scalability
Java’s multi-threaded nature and advanced concurrency mechanisms make it highly scalable. Java web applications can be scaled horizontally by adding more servers and vertically by increasing system resources.
Related Article: How To Set Xms And Xmx Parameters For Jvm
Use Cases: Language Selection Based on Requirements
Choosing the right language depends on the project’s requirements. Let’s explore the use cases for PHP and Java:
PHP Use Cases
– Rapid prototyping and development of small to medium-sized web applications.
– Content management systems (CMS) and blogging platforms.
– E-commerce websites and online marketplaces.
– RESTful APIs and microservices.
Java Use Cases
– Enterprise-level applications with complex business logic.
– High-performance systems that require efficient memory management and concurrency.
– Large-scale web applications with high traffic loads.
– Android mobile app development.
Related Article: How to Use Spring Restcontroller in Java
Best Practices in Both Languages
Following best practices ensures code quality and maintainability. Let’s explore some best practices for PHP and Java:
PHP Best Practices
– Adhere to the principles of object-oriented programming (OOP) for code organization and reusability.
– Use a coding style guide, such as PSR-12, to maintain consistent code formatting.
– Apply proper error handling and logging techniques.
– Utilize version control systems, like Git, for collaborative development.
Java Best Practices
– Follow the Java Naming Conventions for class, method, and variable names.
– Utilize object-oriented design patterns to solve common problems.
– Write unit tests to ensure code correctness and facilitate future refactoring.
– Document your code using JavaDoc comments to enhance code understandability.
Related Article: Java Hibernate Interview Questions and Answers
Real-World Examples: Success Stories
Real-world examples of successful projects can provide valuable insights. Let’s explore some success stories in PHP and Java:
PHP Success Story: WordPress
WordPress, one of the most popular CMS platforms, is built using PHP. It powers millions of websites worldwide and offers a user-friendly interface, extensive plugin ecosystem, and customizable themes.
Java Success Story: Twitter
Twitter, a widely-used social media platform, relies on Java for its backend infrastructure. Java’s scalability, performance, and robustness make it an ideal choice for handling millions of tweets and user interactions daily.
Related Article: How to Use Hibernate in Java
Advanced Techniques in Web Development: A Comparative Study
Advanced web development techniques can enhance the functionality and performance of applications. Let’s compare some advanced techniques in PHP and Java:
PHP Advanced Techniques
– Caching: Implementing caching mechanisms, such as Redis or Memcached, to improve application performance.
– Asynchronous Programming: Utilizing asynchronous libraries like ReactPHP to handle concurrent operations efficiently.
– WebSockets: Implementing real-time communication using WebSockets for interactive features like chat systems or live updates.
Java Advanced Techniques
– Java Persistence API (JPA): Using JPA, a standard specification for ORM in Java, to simplify database operations.
– Message Queues: Implementing message queue systems like RabbitMQ or Apache Kafka for asynchronous processing and decoupling of components.
– Reactive Programming: Leveraging reactive frameworks like Spring WebFlux to build highly scalable and responsive applications.
Code Snippet: Database Connection
Here’s an example of connecting to a MySQL database in both PHP and Java:
PHP Code Snippet
connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully"; ?>
Java Code Snippet
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DatabaseConnection { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { Connection conn = DriverManager.getConnection(url, username, password); System.out.println("Connected successfully"); } catch (SQLException e) { System.out.println("Connection failed: " + e.getMessage()); } } }
Code Snippet: User Authentication
Implementing user authentication is a common requirement in web applications. Here’s an example of user authentication in both PHP and Java:
PHP Code Snippet
Java Code Snippet
import java.util.Scanner; public class UserAuthentication { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter username: "); String username = scanner.nextLine(); System.out.print("Enter password: "); String password = scanner.nextLine(); // Validate credentials and perform authentication logic if (username.equals("admin") && password.equals("password")) { System.out.println("Authentication successful"); } else { System.out.println("Invalid credentials"); } scanner.close(); } }
Code Snippet: Data Validation
Validating user input is crucial to prevent security vulnerabilities and ensure data integrity. Here’s an example of data validation in both PHP and Java:
PHP Code Snippet
Java Code Snippet
import java.util.Scanner; public class DataValidation { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter name: "); String name = scanner.nextLine(); System.out.print("Enter email: "); String email = scanner.nextLine(); // Validate name if (name.isEmpty()) { System.out.println("Name is required"); } else { System.out.println("Name is valid"); } // Validate email if (email.isEmpty()) { System.out.println("Email is required"); } else if (!email.matches("[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}")) { System.out.println("Invalid email address"); } else { System.out.println("Email is valid"); } scanner.close(); } }
Code Snippet: Session Management
Managing user sessions is essential for maintaining state and providing a personalized experience. Here’s an example of session management in both PHP and Java:
PHP Code Snippet
Java Code Snippet
import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; import java.io.IOException; public class SessionManagementServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { HttpSession session = request.getSession(false); // Check if the user is logged in if (session != null && session.getAttribute("username") != null) { String username = (String) session.getAttribute("username"); response.getWriter().write("Welcome, " + username); } else { response.getWriter().write("You are not logged in"); } } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { HttpSession session = request.getSession(false); // Perform logout if (session != null) { session.invalidate(); } response.sendRedirect("login.jsp"); } }
Error Handling: Best Practices
Proper error handling is crucial for identifying and resolving issues in software. Let’s explore some best practices for error handling in PHP and Java:
PHP Error Handling Best Practices
– Enable error reporting in development environments to catch and fix issues early.
– Use try-catch blocks to handle exceptions gracefully and provide meaningful error messages.
– Log errors to a centralized location for easier troubleshooting.
Java Error Handling Best Practices
– Use a logging framework like Log4j or SLF4J to log errors and exceptions.
– Implement custom exception classes to provide detailed information about specific errors.
– Leverage try-catch-finally blocks to ensure resources are properly released.
And that concludes our practical comparison of PHP and Java. Both languages have their strengths and are suitable for various types of projects. Consider the requirements, development team expertise, and project constraints when choosing the right language for your next endeavor.