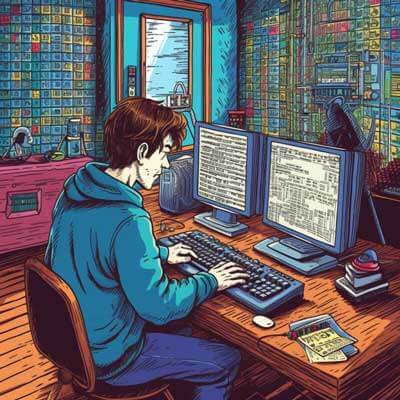
Table of Contents
To effectively debug and troubleshoot PHP applications, it is essential to display PHP errors. By enabling error reporting, you can easily identify and fix potential issues in your code. This step-by-step guide will walk you through the process of displaying PHP errors.
Step 1: Understanding PHP Error Reporting Levels
PHP provides different error reporting levels to control the verbosity of error messages. These levels help you determine the severity of errors that should be displayed. Here are the commonly used error reporting levels:
- Error: Display all types of errors, including fatal errors, warnings, and notices.
- Warning: Display warnings and notices, but ignore fatal errors.
- Notice: Display only notices, ignoring warnings and fatal errors.
- None: Do not display any errors.
Related Article: How To Check If A PHP String Contains A Specific Word
Step 2: Setting the Error Reporting Level in PHP Configuration
One way to display PHP errors is by modifying the PHP configuration file (php.ini). This method applies the error reporting setting globally, affecting all PHP scripts on the server. Follow these steps to set the error reporting level:
1. Locate the php.ini file on your server. The location may vary depending on your operating system and PHP installation.
2. Open the php.ini file using a text editor.
3. Search for the error_reporting
directive. By default, it is usually commented out with a semicolon (;).
4. Uncomment the error_reporting
directive by removing the semicolon (;) at the beginning of the line.
5. Set the desired error reporting level by assigning the appropriate constant. For example, to display all errors, use the following value:
error_reporting = E_ALL
6. Save the changes to the php.ini file.
7. Restart your web server to apply the new configuration.
Step 3: Changing the Error Reporting Level in PHP Scripts
If you want to change the error reporting level for a specific PHP script instead of globally, you can modify the error reporting setting within the script itself. Here's how:
1. Open the PHP script that you want to modify using a text editor.
2. Add the following line at the beginning of the script to set the error reporting level:
error_reporting(E_ALL);
3. Save the changes to the PHP script.
4. Execute the modified PHP script, and the error reporting level will be applied only to that script.
Step 4: Displaying Errors on Development Environments
During the development phase, it is crucial to display PHP errors to catch and fix issues as early as possible. To ensure error reporting is enabled on your development environment, follow these steps:
1. Set the error reporting level to E_ALL
in your PHP configuration file or directly in your development environment's PHP script.
2. Additionally, enable the display_errors
directive by setting it to On
in your PHP configuration file:
display_errors = On
3. Save the changes and restart your web server.
Enabling display_errors
ensures that PHP errors are shown directly on the webpage, allowing you to identify and address them promptly.
Related Article: PHP vs Python: How to Choose the Right Language
Step 5: Logging Errors to a File
In addition to displaying errors on the webpage, you can also log PHP errors to a file for further analysis. This approach is useful for production environments where displaying errors directly to users may not be desirable. Here's how you can log PHP errors to a file:
1. Open your PHP configuration file (php.ini).
2. Locate the error_log
directive. By default, it is usually commented out with a semicolon (;).
3. Uncomment the error_log
directive by removing the semicolon (;) at the beginning of the line.
4. Set the file path where you want to store the error log. For example:
error_log = /var/log/php_errors.log
5. Save the changes to the php.ini file.
6. Restart your web server.
Step 6: Displaying Specific Types of Errors
In some cases, you may only want to display specific types of errors while ignoring others. PHP provides various error reporting constants that allow you to fine-tune the error reporting level. Here are a few commonly used constants:
- E_ALL
: Display all types of errors.
- E_ERROR
: Display fatal errors only.
- E_WARNING
: Display warnings only.
- E_NOTICE
: Display notices only.
- E_DEPRECATED
: Display deprecated function usage notices.
- E_STRICT
: Display strict standards notices.
To display specific types of errors, follow these steps:
1. Open your PHP configuration file (php.ini).
2. Locate the error_reporting
directive.
3. Set the desired error reporting level by assigning the appropriate constant. For example, to display only warnings and notices, use the following value:
error_reporting = E_WARNING | E_NOTICE
4. Save the changes to the php.ini file.
5. Restart your web server.
Step 7: Using Error Reporting Functions
PHP provides several built-in functions that allow you to customize error reporting at runtime. These functions offer flexibility for handling errors dynamically within your PHP code. Here are a few commonly used error reporting functions:
- error_reporting()
: Sets the error reporting level at runtime.
- ini_set()
: Modifies the value of a configuration option at runtime.
- ini_get()
: Retrieves the value of a configuration option.
- error_log()
: Logs an error message to a file or the system log.
- trigger_error()
: Generates a user-level error message.
Step 8: Best Practices for Error Reporting
To effectively use error reporting in PHP, consider the following best practices:
1. During development, set the error reporting level to E_ALL
and enable display_errors
to catch and fix errors early in the development process.
2. Avoid displaying PHP errors on production environments, as they may contain sensitive information that could be exploited by attackers. Instead, log errors to a file and monitor the log regularly.
3. Regularly review the error log to identify recurring errors and proactively address them to improve the stability and performance of your PHP applications.
4. Use error reporting functions to handle errors dynamically within your PHP code. This allows you to customize error handling based on specific scenarios or requirements.
5. Ensure that your PHP configuration file (php.ini) is properly secured to prevent unauthorized access and modifications to error reporting settings.