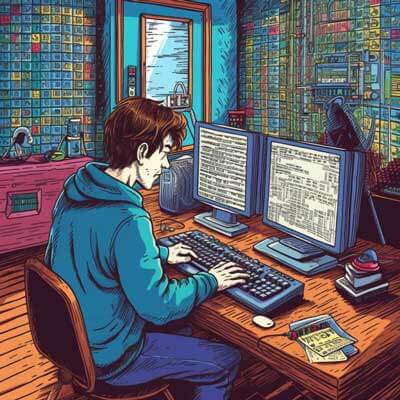
To check if a PHP string contains a specific word, you can use various string manipulation functions and techniques available in PHP. In this answer, we’ll explore two common approaches to accomplish this task.
1. Using the strpos() Function
The strpos() function is a built-in PHP function that can be used to find the position of the first occurrence of a substring within a string. By using this function, we can check if a word exists within a string and perform further actions based on the result. Here’s an example:
$string = "This is a sample string"; $word = "sample"; if (strpos($string, $word) !== false) { echo "The string contains the word '{$word}'"; } else { echo "The string does not contain the word '{$word}'"; }
In this example, we use the strpos() function to check if the word “sample” exists within the string “This is a sample string”. The strpos() function returns the position of the first occurrence of the word within the string, or false if the word is not found. We use the !== operator to perform a strict comparison, ensuring that the position returned by strpos() is not false and not equal to the boolean value false.
If the word is found within the string, the message “The string contains the word ‘sample'” will be displayed. Otherwise, the message “The string does not contain the word ‘sample'” will be displayed.
Related Article: How to Write to the Console in PHP
2. Using the preg_match() Function
Another approach to check if a PHP string contains a specific word is by using regular expressions with the preg_match() function. This function allows you to perform pattern matching within a string. Here’s an example:
$string = "This is a sample string"; $word = "sample"; if (preg_match("/\b{$word}\b/", $string)) { echo "The string contains the word '{$word}'"; } else { echo "The string does not contain the word '{$word}'"; }
In this example, we use the preg_match() function with a regular expression pattern that searches for the exact word match using word boundaries (\b). The pattern “/\b{$word}\b/” searches for the word “sample” within the string, surrounded by word boundaries.
If the word is found within the string, the message “The string contains the word ‘sample'” will be displayed. Otherwise, the message “The string does not contain the word ‘sample'” will be displayed.
Why is this question asked?
The question of how to check if a PHP string contains a specific word is commonly asked because it is a fundamental operation in many PHP applications. Knowing how to determine if a string contains a particular word can be useful in various scenarios, such as:
– Validating user input: You may want to check if a certain word or keyword is present in user-provided data, such as form input or search queries.
– Manipulating text: You may need to perform different actions or transformations on a string based on the presence or absence of a specific word.
– Filtering or searching: You may want to filter or search through a large dataset to find strings that contain a particular word.
Alternative Ideas and Suggestions
While the approaches mentioned above are commonly used and effective, there are alternative methods to check if a PHP string contains a specific word. Here are a few suggestions:
– Using the stristr() function: The stristr() function is similar to strpos() but performs a case-insensitive search. This can be useful if you want to check for a word regardless of its case in the string.
– Converting the string to an array and using in_array(): You can split the string into an array of words using the explode() function and then use the in_array() function to check if the desired word exists within the array.
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
Best Practices
When checking if a PHP string contains a specific word, keep the following best practices in mind:
– Consider case sensitivity: Depending on your requirements, you may want to perform a case-sensitive or case-insensitive search. Choose the appropriate function accordingly (e.g., strpos() for case-sensitive, stristr() for case-insensitive).
– Use word boundaries for exact matches: If you need to match the word exactly and not as part of a larger word, use word boundaries in your regular expressions (e.g., “/\b{$word}\b/”).
– Handle multibyte strings: If you’re working with multibyte strings, consider using the mb_strpos() or mb_stripos() functions to handle character encodings properly.
Overall, the choice of approach depends on your specific use case and requirements. Choose the method that best suits your needs in terms of performance, case sensitivity, and regular expression capabilities.
Feel free to explore the PHP documentation for more information on the available string manipulation functions and regular expression patterns:
– PHP strpos()
– PHP preg_match()