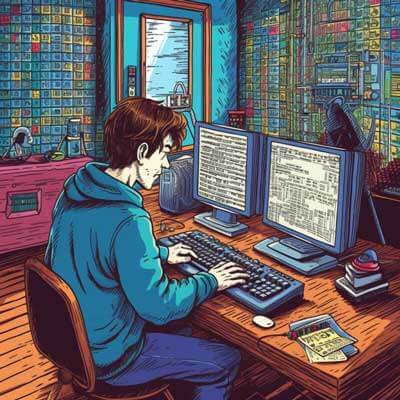
Table of Contents
Introduction to Overriding and Overloading
Overriding and overloading are two important concepts in Java that allow developers to create more flexible and reusable code. Understanding the difference between these two concepts is crucial for writing efficient and maintainable programs.
Related Article: How to Pass Arguments by Value in Java
Defining Overloading
Overloading refers to the ability to define multiple methods in a class with the same name but different parameters. This allows developers to create methods that perform similar operations but on different types of data or with different input parameters.
public class Calculator { public int add(int a, int b) { return a + b; } public double add(double a, double b) { return a + b; }}
In the above example, the Calculator
class defines two add
methods - one that takes two integers as parameters and another that takes two doubles. This allows the developer to add integers or doubles using the same method name.
Defining Overriding
Overriding, on the other hand, involves creating a method in a subclass that has the same name, return type, and parameters as a method in its superclass. By doing so, the subclass provides a different implementation of the method, effectively replacing the implementation inherited from the superclass.
public class Animal { public void makeSound() { System.out.println("The animal makes a sound"); }}public class Cat extends Animal { @Override public void makeSound() { System.out.println("The cat meows"); }}
In the above example, the Cat
class extends the Animal
class and overrides the makeSound
method. The Cat
class provides its own implementation of the method, which outputs "The cat meows" instead of "The animal makes a sound" like the superclass.
Contrasting Overloading and Overriding
While both overloading and overriding involve creating methods with the same name, they serve different purposes. Overloading allows for multiple methods with the same name but different parameters, while overriding involves creating a method in a subclass that replaces the implementation of a method inherited from the superclass.
It is important to note that overloading is determined at compile-time based on the method's parameters, while overriding is determined at runtime based on the actual type of the object.
Related Article: Java Exception Handling Tutorial
Best Practices for Overloading
When it comes to overloading methods, there are some best practices to keep in mind to ensure clean and readable code.
Use Case: Overloading for Different Input Parameters
One common use case for overloading is when you want to perform the same operation but on different types of input parameters. Let's consider an example where we want to calculate the area of different shapes:
public class AreaCalculator { public double calculateArea(double radius) { return Math.PI * radius * radius; } public double calculateArea(double length, double width) { return length * width; }}
In this example, the AreaCalculator
class defines two methods for calculating the area - one for a circle using the radius and another for a rectangle using the length and width. By overloading the calculateArea
method, we can provide a clear and concise way to calculate the area for different shapes.
Real World Example: Overloading Constructors
Another common use case for overloading is when creating constructors for a class. Overloading constructors allows for different ways to initialize objects of the class.
public class Person { private String name; private int age; public Person() { this.name = "Unknown"; this.age = 0; } public Person(String name) { this.name = name; this.age = 0; } public Person(String name, int age) { this.name = name; this.age = age; }}
In this example, the Person
class defines multiple constructors with different parameters. This allows developers to create Person
objects with just a name, with a name and age, or with no parameters at all.
These best practices for overloading methods can help improve code readability and provide flexibility in designing classes.
Best Practices for Overriding
Overriding methods in Java requires careful consideration to ensure correct behavior and maintainability of the code.
Related Article: How to Sort a List of ArrayList in Java
Use Case: Overriding to Change Method Behavior
One common use case for overriding is when you want to change the behavior of a method inherited from a superclass. Let's consider an example where we have a class hierarchy for different types of vehicles:
public class Vehicle { public void start() { System.out.println("The vehicle starts"); }}public class Car extends Vehicle { @Override public void start() { System.out.println("The car engine starts"); }}public class Motorcycle extends Vehicle { @Override public void start() { System.out.println("The motorcycle engine starts"); }}
In this example, the Car
and Motorcycle
classes override the start
method inherited from the Vehicle
class. Each subclass provides its own implementation of the method, reflecting the specific behavior of starting a car or a motorcycle.
Real World Example: Overriding toString Method
Another common use case for overriding is when you want to provide a custom string representation of an object using the toString
method. This can be useful for debugging purposes or displaying meaningful information about an object.
public class Person { private String name; private int age; // constructors and other methods @Override public String toString() { return "Person{name='" + name + "', age=" + age + "}"; }}
In this example, the Person
class overrides the toString
method inherited from the Object
class. The overridden method returns a string representation of the Person
object, including the name and age.
Performance Considerations: Overloading
When it comes to performance considerations, overloading methods in Java has minimal impact. The decision to overload methods should primarily be based on code readability and maintainability rather than performance optimization.
Performance Considerations: Overriding
Overriding methods in Java can have an impact on performance due to the process of dynamic method dispatch. When a method is overridden, the JVM must determine at runtime which version of the method to invoke based on the actual type of the object.
While the performance impact of overriding is generally negligible in most scenarios, it can become noticeable when dealing with a large number of method invocations or in performance-critical applications. In such cases, it is important to consider the potential impact and evaluate alternative approaches if necessary.
Related Article: How to Easily Print a Java Array
Advanced Techniques: Utilizing Polymorphism in Overriding
One of the key advantages of overriding methods in Java is the ability to leverage polymorphism. Polymorphism allows objects of different classes to be treated as objects of a common superclass, enabling code to be written in a more generic and reusable manner.
public class Animal { public void makeSound() { System.out.println("The animal makes a sound"); }}public class Cat extends Animal { @Override public void makeSound() { System.out.println("The cat meows"); }}public class Dog extends Animal { @Override public void makeSound() { System.out.println("The dog barks"); }}public class Main { public static void main(String[] args) { Animal animal1 = new Cat(); Animal animal2 = new Dog(); animal1.makeSound(); // Output: The cat meows animal2.makeSound(); // Output: The dog barks }}
In this example, the Animal
class is a superclass with a makeSound
method, which is overridden in the Cat
and Dog
subclasses. By utilizing polymorphism, we can treat objects of different subclasses as objects of the Animal
superclass and invoke the overridden method accordingly.
Advanced Techniques: Deciding When to Use Overloading
Determining when to use overloading can sometimes be a subjective decision. However, there are some guidelines to consider:
- Use overloading when you want to provide multiple ways to perform a similar operation with different input parameters.
- Avoid overloading methods that differ only in return type, as it can lead to confusion and potential compilation errors.
- Keep the number of overloaded methods manageable to maintain code readability.
Code Snippet: Overloading Methods
public class Calculator { public int add(int a, int b) { return a + b; } public double add(double a, double b) { return a + b; }}
This code snippet demonstrates the concept of overloading methods in Java by implementing an add
method with different parameter types.
Code Snippet: Overriding Methods
public class Animal { public void makeSound() { System.out.println("The animal makes a sound"); }}public class Cat extends Animal { @Override public void makeSound() { System.out.println("The cat meows"); }}
This code snippet illustrates the process of overriding methods in Java by creating a superclass Animal
with a makeSound
method, which is overridden in the Cat
subclass to provide a different implementation.
Related Article: Java Adapter Design Pattern Tutorial
Code Snippet: Overloading Constructors
public class Person { private String name; private int age; public Person() { this.name = "Unknown"; this.age = 0; } public Person(String name) { this.name = name; this.age = 0; } public Person(String name, int age) { this.name = name; this.age = age; }}
This code snippet showcases the usage of overloading constructors in Java to provide different ways to initialize objects of the Person
class.
Code Snippet: Overriding toString Method
public class Person { private String name; private int age; // constructors and other methods @Override public String toString() { return "Person{name='" + name + "', age=" + age + "}"; }}
This code snippet demonstrates how to override the toString
method in Java to provide a custom string representation of an object, in this case, the Person
class.
Code Snippet: Handling Errors in Overloading
public class MathUtils { public int divide(int dividend, int divisor) { if (divisor == 0) { throw new IllegalArgumentException("Divisor cannot be zero"); } return dividend / divisor; } public double divide(double dividend, double divisor) { if (divisor == 0) { throw new IllegalArgumentException("Divisor cannot be zero"); } return dividend / divisor; }}
This code snippet demonstrates how to handle errors in overloading methods by throwing an exception when the divisor is zero.
Code Snippet: Handling Errors in Overriding
public class Animal { public void makeSound() throws InterruptedException { System.out.println("The animal makes a sound"); Thread.sleep(1000); }}public class Cat extends Animal { @Override public void makeSound() throws InterruptedException { System.out.println("The cat meows"); Thread.sleep(500); }}
This code snippet showcases error handling in overriding methods by throwing a checked exception and handling it appropriately.
Related Article: Java OOP Tutorial
Error Handling: Anticipating Problems with Overloading
When overloading methods in Java, it is important to anticipate potential problems and design the overloaded methods carefully. Some common issues to consider include:
- Ambiguity: Avoid creating overloaded methods that have similar parameter types, as it can lead to ambiguity and potential compilation errors.
- Method resolution: Understand how the Java compiler determines the most specific method to invoke when multiple overloaded methods are available with different parameter types.
By anticipating these problems and adhering to best practices, you can avoid potential issues when using method overloading in your code.
Error Handling: Anticipating Problems with Overriding
When overriding methods in Java, it is crucial to anticipate potential problems and ensure correct behavior. Some common issues to consider include:
- Signature mismatch: Ensure that the overridden method has the same signature as the method in the superclass, including the return type, name, and parameters.
- Missing @Override
annotation: Although not required, it is good practice to use the @Override
annotation when overriding methods to catch potential mistakes at compile-time.
- Method visibility: Overriding methods should not reduce the visibility of the inherited method, i.e., the overridden method cannot have lower access modifiers than the method in the superclass.
By anticipating these problems and following best practices, you can avoid potential errors and ensure proper method overriding in your Java code.