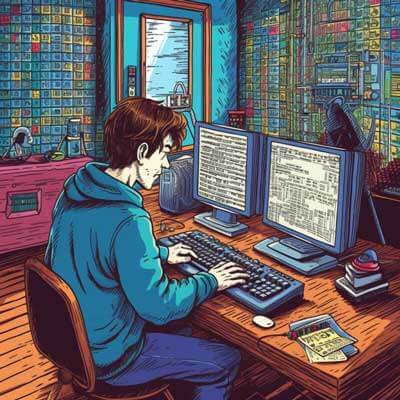
Table of Contents
The now
function in PHP is a built-in function that returns the current date and time. It is commonly used in web applications for various purposes such as displaying the current date and time, generating timestamps, or comparing dates. In this guide, we will explore how to use the now
function effectively in PHP.
Getting the Current Date and Time
To retrieve the current date and time in PHP, you can use the date
function with the appropriate format specifier. The format specifier for the current date and time is 'Y-m-d H:i:s'
. Here's an example that demonstrates how to use the date
function to get the current date and time:
$currentDateTime = date('Y-m-d H:i:s'); echo $currentDateTime;
This will output the current date and time in the format 'Y-m-d H:i:s'
, which represents the year, month, day, hour, minute, and second. For example, the output might look like this: 2022-01-01 12:34:56
.
Related Article: How to Echo or Print an Array in PHP
Generating Timestamps
Timestamps are commonly used in PHP to represent dates and times in a numeric format. The now
function can be used to generate timestamps by utilizing the strtotime
function. The strtotime
function is used to convert a human-readable date and time string into a Unix timestamp.
Here's an example that demonstrates how to use the now
function to generate a timestamp:
$timestamp = strtotime('now'); echo $timestamp;
This will output the current timestamp, which represents the number of seconds that have elapsed since January 1, 1970 (Unix epoch) until the current date and time.
Comparing Dates and Times
The now
function can also be used to compare dates and times in PHP. By comparing timestamps, you can easily check if a certain date and time is before or after the current date and time.
Here's an example that demonstrates how to compare dates and times using the now
function:
$currentDateTime = strtotime('now'); $targetDateTime = strtotime('2022-01-01 00:00:00'); if ($targetDateTime $currentDateTime) { echo 'The target date and time is in the future.'; } else { echo 'The target date and time is the same as the current date and time.'; }
This example compares the target date and time (2022-01-01 00:00:00
) with the current date and time. Depending on the result of the comparison, it will output the corresponding message.
Best Practices and Suggestions
When using the now
function in PHP, consider the following best practices and suggestions:
1. Use the appropriate format specifier when displaying the current date and time to ensure it matches your desired output format.
2. When comparing dates and times, always convert them to timestamps to ensure accurate and reliable comparisons.
3. Be mindful of the timezone settings in your PHP configuration. The now
function returns the current date and time based on the server's timezone.
4. Consider using the DateTime
class in PHP for more advanced date and time manipulation and calculations. It provides a more object-oriented and intuitive API for working with dates and times.
For more information, you can refer to the official PHP documentation on the date
function: PHP date function