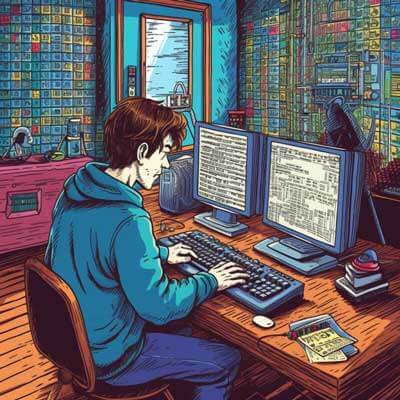
Table of Contents
How to Work with Arrays in PHP
Arrays are a fundamental data structure in PHP that allow you to store and manipulate collections of values. In this article, we will explore the basics of working with arrays in PHP, including a comprehensive list of array functions, advanced examples, real-world use cases, and best practices.
Related Article: The Path to Speed: How to Release Software to Production All Day, Every Day (Intro)
Basics of Arrays in PHP
In PHP, an array is an ordered map that associates values with keys. The keys can be either integers or strings, and the values can be of any data type, including other arrays. Here's an example of creating an array in PHP:
$fruits = array("apple", "banana", "orange");
You can access individual elements of an array using their keys. For example, to access the first element of the $fruits
array, you can use the following code:
echo $fruits[0]; // Output: apple
Array Functions in PHP
PHP provides a wide range of built-in functions to work with arrays. Here are some commonly used array functions:
count()
The count()
function returns the number of elements in an array. Here's an example:
$fruits = array("apple", "banana", "orange"); echo count($fruits); // Output: 3
array_push()
The array_push()
function adds one or more elements to the end of an array. Here's an example:
$fruits = array("apple", "banana"); array_push($fruits, "orange", "mango"); print_r($fruits); // Output: Array ( [0] => apple [1] => banana [2] => orange [3] => mango )
array_pop()
The array_pop()
function removes and returns the last element of an array. Here's an example:
$fruits = array("apple", "banana", "orange"); $lastFruit = array_pop($fruits); echo $lastFruit; // Output: orange
array_merge()
The array_merge()
function merges two or more arrays into a single array. Here's an example:
$fruits1 = array("apple", "banana"); $fruits2 = array("orange", "mango"); $mergedFruits = array_merge($fruits1, $fruits2); print_r($mergedFruits); // Output: Array ( [0] => apple [1] => banana [2] => orange [3] => mango )
array_search()
The array_search()
function searches an array for a given value and returns the corresponding key if found. Here's an example:
$fruits = array("apple", "banana", "orange"); $key = array_search("banana", $fruits); echo $key; // Output: 1
For a complete list of array functions in PHP, you can refer to the official PHP documentation: PHP Array Functions.
Advanced Examples
Multidimensional Arrays
A multidimensional array is an array that contains one or more arrays as its elements. This allows you to represent complex data structures. Here's an example of a multidimensional array in PHP:
$students = array( array("John", 20, "Male"), array("Jane", 22, "Female"), array("David", 19, "Male") );
You can access individual elements of a multidimensional array using multiple indices. For example, to access the age of the second student, you can use the following code:
echo $students[1][1]; // Output: 22
Array Map & Filters
The array_map()
function applies a callback function to each element of an array and returns a new array with the modified values. Here's an example that doubles each element of an array:
$numbers = array(1, 2, 3, 4, 5); $doubledNumbers = array_map(function($num) { return $num * 2; }, $numbers); print_r($doubledNumbers); // Output: Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
The array_filter()
function filters the elements of an array based on a callback function and returns a new array with the filtered values. Here's an example that filters out the odd numbers from an array:
$numbers = array(1, 2, 3, 4, 5); $evenNumbers = array_filter($numbers, function($num) { return $num % 2 == 0; }); print_r($evenNumbers); // Output: Array ( [1] => 2 [3] => 4 )
Array Reduce
The array_reduce()
function applies a callback function to each element of an array, reducing it to a single value. Here's an example that calculates the sum of all elements in an array:
$numbers = array(1, 2, 3, 4, 5); $sum = array_reduce($numbers, function($carry, $num) { return $carry + $num; }); echo $sum; // Output: 15
Array Diff
The array_diff()
function compares two or more arrays and returns the values from the first array that are not present in the other arrays. Here's an example:
$array1 = array("apple", "banana", "orange"); $array2 = array("banana", "kiwi", "orange"); $diff = array_diff($array1, $array2); print_r($diff); // Output: Array ( [0] => apple )
Array Column
The array_column()
function returns the values from a single column of a multidimensional array. Here's an example:
$students = array( array("name" => "John", "age" => 20), array("name" => "Jane", "age" => 22), array("name" => "David", "age" => 19) ); $names = array_column($students, "name"); print_r($names); // Output: Array ( [0] => John [1] => Jane [2] => David )
Real-World Examples
Arrays are widely used in real-world PHP applications. Here are a few examples of how arrays can be used:
1. Storing user information: You can use an array to store user details like name, email, and address.
$user = array( "name" => "John Doe", "email" => "john@example.com", "address" => "123 Main St" );
2. Managing product inventory: You can use an array to store information about products, such as name, price, and quantity.
$products = array( array("name" => "iPhone", "price" => 999, "quantity" => 10), array("name" => "Samsung Galaxy", "price" => 899, "quantity" => 5), array("name" => "Google Pixel", "price" => 799, "quantity" => 3) );
Related Article: Comparing GraphQL vs REST & How To Manage APIs
Best Practices
When working with arrays in PHP, it's important to follow some best practices to ensure clean and efficient code:
1. Use meaningful variable and array names to improve code readability.
2. Avoid using magic numbers as array indices. Instead, use constants or variables to represent indices.
3. Validate array keys and indices before accessing them to avoid errors.
4. Take advantage of built-in array functions to simplify your code and improve performance.
5. Document your code and provide clear comments to explain the purpose and usage of arrays.
By following these best practices, you can write maintainable and robust PHP code when working with arrays.