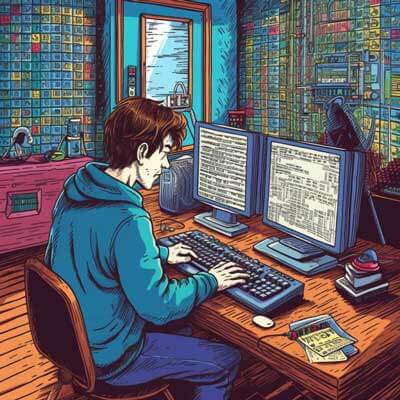
- Code Snippet 1 – How to Validate Email Address
- Code Snippet 2 – How to Generate Random Passwords
- Code Snippet 3 – How to Resize and Optimize Images
- Code Snippet 4 – How to Encrypt and Decrypt Data
- Code Snippet 5 – How to Generate Unique IDs
- Code Snippet 6 – How to Execute Shell Commands
- Code Snippet 7 – How to Manipulate Strings
- Code Snippet 8 – How to Validate Input Fields
- Code Snippet 9 – How to Handle Date and Time
- Code Snippet 10 – How to Send Email with SMTP
- Popular PHP Frameworks for Web Development
- Laravel
- Symfony
- CodeIgniter
- Yii
- Zend Framework
- Best Practices for Writing PHP Code
- Essential PHP Functions for Everyday Problem-Solving
- Common Syntax Errors in PHP and How to Fix Them
- Optimizing PHP Scripts for Better Performance
- Security Considerations when Writing PHP Code
- Debugging and Troubleshooting PHP Code
- External Sources
Code Snippet 1 – How to Validate Email Address
To validate an email address in PHP, you can use the filter_var function along with the FILTER_VALIDATE_EMAIL filter. Here’s an example code snippet:
$email = "example@example.com"; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "Valid email address"; } else { echo "Invalid email address"; }
This code snippet checks if the email address stored in the $email
variable is valid or not using the FILTER_VALIDATE_EMAIL
filter. If the email address is valid, it will output “Valid email address”, otherwise, it will output “Invalid email address”.
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
Code Snippet 2 – How to Generate Random Passwords
To generate a random password in PHP, you can make use of the random_bytes
or openssl_random_pseudo_bytes
functions to generate a random string of bytes and then convert it to a readable format. Here’s an example code snippet:
$length = 8; $password = bin2hex(random_bytes($length)); echo "Random password: " . $password;
In this code snippet, the $length
variable represents the length of the password you want to generate. The random_bytes
function generates a string of random bytes, and the bin2hex
function converts the binary data to a hexadecimal representation, which can be used as a password.
Code Snippet 3 – How to Resize and Optimize Images
To resize and optimize images in PHP, you can make use of the GD library, which provides functions for image manipulation. Here’s an example code snippet that resizes an image to a specific width and height:
$sourceImagePath = "path/to/source/image.jpg"; $destinationImagePath = "path/to/destination/image.jpg"; $newWidth = 500; $newHeight = 300; $sourceImage = imagecreatefromjpeg($sourceImagePath); $destinationImage = imagecreatetruecolor($newWidth, $newHeight); imagecopyresized($destinationImage, $sourceImage, 0, 0, 0, 0, $newWidth, $newHeight, imagesx($sourceImage), imagesy($sourceImage)); imagejpeg($destinationImage, $destinationImagePath, 80); imagedestroy($sourceImage); imagedestroy($destinationImage);
In this code snippet, you need to provide the paths to the source image and the destination image. The $newWidth
and $newHeight
variables represent the desired width and height of the resized image. The imagecreatefromjpeg
function creates a new image from the source image file, and imagecreatetruecolor
creates a new true color image with the specified width and height. The imagecopyresized
function resizes the source image to the specified dimensions and copies it to the destination image. Finally, imagejpeg
saves the destination image as a JPEG file with a compression quality of 80.
Code Snippet 4 – How to Encrypt and Decrypt Data
To encrypt and decrypt data in PHP, you can make use of the OpenSSL extension, which provides functions for encryption and decryption using various algorithms. Here’s an example code snippet that encrypts and decrypts a string using the AES-256-CBC algorithm:
$data = "Hello, world!"; $key = "secretkey"; $iv = random_bytes(16); $encryptedData = openssl_encrypt($data, "AES-256-CBC", $key, OPENSSL_RAW_DATA, $iv); $decryptedData = openssl_decrypt($encryptedData, "AES-256-CBC", $key, OPENSSL_RAW_DATA, $iv); echo "Encrypted data: " . base64_encode($encryptedData) . "<br>"; echo "Decrypted data: " . $decryptedData;
In this code snippet, the $data
variable represents the string you want to encrypt and decrypt. The $key
variable represents the encryption key, and the $iv
variable represents the initialization vector, which is used to ensure different ciphertexts are generated even if the same plaintext is encrypted multiple times. The openssl_encrypt
function encrypts the data using the AES-256-CBC algorithm, and the openssl_decrypt
function decrypts the encrypted data. The base64_encode
function is used to encode the encrypted data as a base64 string for display purposes.
Related Article: Preventing Redundant MySQL Queries in PHP Loops
Code Snippet 5 – How to Generate Unique IDs
To generate unique IDs in PHP, you can make use of the uniqid
function. Here’s an example code snippet:
$uniqueId = uniqid(); echo "Unique ID: " . $uniqueId;
The uniqid
function generates a unique identifier based on the current time in microseconds. It is useful for generating unique IDs for various purposes, such as database records or session identifiers.
Code Snippet 6 – How to Execute Shell Commands
To execute shell commands in PHP, you can make use of the exec
or shell_exec
functions. Here’s an example code snippet:
$command = "ls -l"; $output = shell_exec($command); echo "Command output: " . $output;
In this code snippet, the $command
variable represents the shell command you want to execute. The shell_exec
function executes the command and returns the output as a string. You can then use this output for further processing or display.
Code Snippet 7 – How to Manipulate Strings
To manipulate strings in PHP, you can make use of various built-in string functions. Here are a couple of examples code snippets:
Example 1: Converting a string to uppercase:
$string = "hello, world!"; $uppercaseString = strtoupper($string); echo "Uppercase string: " . $uppercaseString;
In this code snippet, the strtoupper
function converts all characters in the string to uppercase.
Example 2: Checking if a string contains another string:
$string = "Lorem ipsum dolor sit amet"; $substring = "ipsum"; if (strpos($string, $substring) !== false) { echo "Substring found"; } else { echo "Substring not found"; }
In this code snippet, the strpos
function is used to find the position of a substring within a string. If the substring is found, strpos
returns the position, otherwise it returns false
.
Related Article: Integrating Payment & Communication Features in Laravel
Code Snippet 8 – How to Validate Input Fields
To validate input fields in PHP, you can make use of various validation techniques, such as regular expressions or built-in functions. Here’s an example code snippet that validates a phone number using a regular expression:
$phoneNumber = "123-456-7890"; $pattern = "/^\d{3}-\d{3}-\d{4}$/"; if (preg_match($pattern, $phoneNumber)) { echo "Valid phone number"; } else { echo "Invalid phone number"; }
In this code snippet, the $phoneNumber
variable represents the phone number you want to validate, and the $pattern
variable represents the regular expression pattern for a phone number in the format “123-456-7890”. The preg_match
function checks if the phone number matches the pattern, and if it does, it outputs “Valid phone number”, otherwise it outputs “Invalid phone number”.
Code Snippet 9 – How to Handle Date and Time
To handle date and time in PHP, you can make use of the date
function along with various format specifiers. Here’s an example code snippet that displays the current date and time:
$currentDateTime = date("Y-m-d H:i:s"); echo "Current date and time: " . $currentDateTime;
In this code snippet, the date
function is used to format the current date and time using the “Y-m-d H:i:s” format specifier, which represents the year, month, day, hour, minute, and second.
Code Snippet 10 – How to Send Email with SMTP
To send emails with SMTP in PHP, you can make use of the PHPMailer
library, which provides a convenient and easy-to-use interface for sending emails. Here’s an example code snippet that sends an email using SMTP:
require 'vendor/autoload.php'; use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\Exception; $mail = new PHPMailer(true); try { $mail->isSMTP(); $mail->Host = 'smtp.example.com'; $mail->SMTPAuth = true; $mail->Username = 'your-email@example.com'; $mail->Password = 'your-email-password'; $mail->SMTPSecure = 'tls'; $mail->Port = 587; $mail->setFrom('your-email@example.com', 'Your Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); $mail->Subject = 'Test Email'; $mail->Body = 'This is a test email'; $mail->send(); echo 'Email sent successfully'; } catch (Exception $e) { echo 'Email could not be sent. Error: ' . $mail->ErrorInfo; }
In this code snippet, you need to include the PHPMailer
library by requiring the autoload file. Then, you can create a new instance of the PHPMailer
class and configure it with your SMTP server details, email credentials, and the email content. Finally, you can call the send
method to send the email.
Related Article: Harnessing Laravel WebSockets for Real-time Features
Popular PHP Frameworks for Web Development
Laravel
Laravel is a widely-used PHP framework known for its elegant syntax and comprehensive features. It follows the Model-View-Controller (MVC) architectural pattern and provides a rich set of tools and libraries for building web applications. Laravel offers features like routing, database migration, authentication, caching, and more, making it a popular choice for both small and large-scale projects.
Symfony
Symfony is a robust PHP framework that aims to speed up the development process by providing reusable components and a flexible architecture. It follows the MVC pattern and offers features like routing, templating, form handling, and security. Symfony is highly extensible and can be used to build a wide range of applications, from simple websites to complex enterprise systems.
Related Article: Optimizing Laravel Apps: Deployment and Scaling Techniques
CodeIgniter
CodeIgniter is a lightweight PHP framework that focuses on simplicity and performance. It provides a small footprint and requires minimal configuration, making it easy to learn and use. CodeIgniter offers features like MVC architecture, database abstraction, form validation, and session management. It is suitable for small to medium-sized projects that require a simple and fast framework.
Yii
Yii is a high-performance PHP framework that emphasizes efficiency and extensibility. It follows the MVC pattern and provides features like database abstraction, caching, security, and RESTful API development. Yii is known for its code generation tools and useful caching capabilities, making it a good choice for building complex and high-traffic applications.
Zend Framework
Zend Framework is a useful PHP framework that focuses on building scalable and secure web applications. It offers a wide range of components and follows the MVC pattern. Zend Framework provides features like routing, database abstraction, caching, and authentication. It is suitable for building enterprise-level applications that require a high level of customization and flexibility.
Related Article: How to Implement Asynchronous Code in PHP
Best Practices for Writing PHP Code
When writing PHP code, it is important to follow best practices to ensure your code is maintainable, scalable, and secure. Here are some best practices for writing PHP code:
1. Use a coding style guide: Adopt a coding style guide, such as PSR-12, to ensure consistency in your codebase. This makes it easier for other developers to understand and maintain your code.
2. Sanitize and validate user input: Always sanitize and validate user input to prevent security vulnerabilities like SQL injection and cross-site scripting (XSS) attacks. Use functions like htmlspecialchars
and filter_input
to sanitize and validate input.
3. Use prepared statements for database queries: To prevent SQL injection attacks, use prepared statements or parameterized queries when interacting with databases. This ensures that user-supplied data is properly escaped.
4. Avoid the use of deprecated or insecure functions: Stay updated with the latest PHP versions and avoid using deprecated or insecure functions. Use modern alternatives that provide better security and performance.
5. Use encryption for sensitive data: When handling sensitive data like passwords or credit card information, always encrypt the data using secure algorithms like bcrypt or Argon2. Avoid storing plain text passwords or sensitive information in databases.
6. Implement error handling and logging: Proper error handling and logging are essential for debugging and maintaining your application. Use try-catch blocks to catch and handle exceptions, and log errors to a central location for easy troubleshooting.
7. Optimize code for performance: Write efficient and optimized code to improve the performance of your application. Use techniques like caching, lazy loading, and code profiling to identify and optimize performance bottlenecks.
8. Use version control: Use a version control system like Git to track changes in your codebase. This allows you to easily revert to previous versions, collaborate with others, and keep your codebase organized.
9. Comment and document your code: Add comments and documentation to your code to make it easier for other developers to understand your code. Use clear and descriptive variable and function names to improve code readability.
10. Test your code: Write unit tests and integration tests to ensure the correctness of your code. Automated testing helps catch bugs early and provides confidence when making changes or refactoring code.
Essential PHP Functions for Everyday Problem-Solving
PHP provides a vast array of built-in functions that can help solve everyday programming problems. Here are some essential PHP functions for everyday problem-solving:
1. strlen
: Returns the length of a string.
$str = "Hello, world!"; $length = strlen($str); echo "Length of string: " . $length;
2. array_push
: Pushes one or more elements onto the end of an array.
$fruits = array("apple", "banana"); array_push($fruits, "orange", "mango"); print_r($fruits);
3. file_get_contents
: Reads the contents of a file into a string.
$content = file_get_contents("path/to/file.txt"); echo "File content: " . $content;
4. json_encode
: Converts a PHP value to a JSON string.
$data = array("name" => "John", "age" => 30); $json = json_encode($data); echo "JSON string: " . $json;
5. date
: Formats a local date and time.
$timestamp = time(); $date = date("Y-m-d H:i:s", $timestamp); echo "Formatted date and time: " . $date;
6. strtolower
: Converts a string to lowercase.
$str = "Hello, WORLD!"; $lowercase = strtolower($str); echo "Lowercase string: " . $lowercase;
7. array_sum
: Calculates the sum of values in an array.
$numbers = array(1, 2, 3, 4, 5); $sum = array_sum($numbers); echo "Sum of numbers: " . $sum;
8. explode
: Splits a string by a specified delimiter and returns an array.
$str = "apple,banana,orange"; $fruits = explode(",", $str); print_r($fruits);
9. array_search
: Searches for a value in an array and returns its key.
$fruits = array("apple", "banana", "orange"); $key = array_search("banana", $fruits); echo "Key of banana: " . $key;
10. file_put_contents
: Writes a string to a file.
$content = "Hello, world!"; file_put_contents("path/to/file.txt", $content);
Common Syntax Errors in PHP and How to Fix Them
Syntax errors are common mistakes that occur when writing PHP code. Here are some common syntax errors in PHP and how to fix them:
1. Missing semicolon:
echo "Hello, world!"
Error: Parse error: syntax error, unexpected ‘echo’ (T_ECHO), expecting ‘,’ or ‘;’
Fix: Add a semicolon at the end of the statement.
echo "Hello, world!";
2. Missing closing parenthesis:
if (condition { // code }
Error: Parse error: syntax error, unexpected ‘{‘, expecting ‘)’
Fix: Add a closing parenthesis after the condition.
if (condition) { // code }
3. Missing closing brace:
if (condition) { // code
Error: Parse error: syntax error, unexpected end of file
Fix: Add a closing brace at the end of the block.
if (condition) { // code }
4. Mismatched quotes:
$name = 'John";
Error: Parse error: syntax error, unexpected ‘”‘, expecting ‘,’ or ‘;’
Fix: Use matching quotes for string literals.
$name = 'John';
5. Misspelled function or variable name:
echho "Hello, world!";
Error: Fatal error: Uncaught Error: Call to undefined function echho()
Fix: Correct the misspelled function name.
echo "Hello, world!";
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
Optimizing PHP Scripts for Better Performance
Optimizing PHP scripts can significantly improve the performance and efficiency of your web applications. Here are some tips for optimizing PHP scripts:
1. Use caching: Implement caching mechanisms to store frequently accessed data and avoid unnecessary database queries or computations. Use tools like Memcached or Redis for caching data.
2. Minimize database queries: Reduce the number of database queries by optimizing your SQL statements, using efficient indexing, and implementing query caching.
3. Use opcode caching: Enable opcode caching to cache the compiled bytecode of PHP scripts. This reduces the overhead of parsing and compiling PHP scripts on each request.
4. Optimize loops and conditionals: Avoid unnecessary iterations and conditionals by optimizing your code logic. Use efficient looping constructs like foreach and array_map instead of traditional for loops.
5. Use appropriate data structures: Choose the right data structures for your application. Use arrays for indexed data, associative arrays for key-value pairs, and hash tables for efficient lookups.
6. Minimize file system operations: Reduce the number of file operations by caching file contents in memory or using alternative storage mechanisms like databases or object storages.
7. Optimize image resizing: When resizing images, use image manipulation libraries like GD or ImageMagick, and save the resized images to disk instead of generating them on every request.
8. Use lazy loading: Load resources or dependencies only when they are needed rather than loading everything upfront. This reduces the memory footprint and improves response times.
9. Enable compression: Enable compression for HTTP responses by using gzip or deflate. This reduces the size of the transferred data and improves network performance.
10. Profile and benchmark: Use profiling tools like Xdebug or Blackfire to identify performance bottlenecks in your PHP code. Benchmark your code to measure the impact of optimizations and track improvements.
Security Considerations when Writing PHP Code
When writing PHP code, it is crucial to consider security to protect your application and its users from potential vulnerabilities. Here are some security considerations when writing PHP code:
1. Input validation and sanitization: Always validate and sanitize user input to prevent security vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF) attacks. Use functions like filter_input
and htmlspecialchars
to sanitize and validate input.
2. Parameterized queries: Use prepared statements or parameterized queries when interacting with databases to prevent SQL injection attacks. Avoid directly concatenating user input into SQL queries.
3. Password hashing: Never store plain text passwords in your database. Instead, use secure hashing algorithms like bcrypt or Argon2 to hash passwords. When verifying passwords, use functions like password_hash
and password_verify
.
4. Cross-Site Scripting (XSS) prevention: Use output escaping techniques like htmlspecialchars
or a templating engine to prevent XSS attacks. Always sanitize user-generated content before displaying it in HTML.
5. Cross-Site Request Forgery (CSRF) prevention: Implement CSRF tokens in your forms to protect against CSRF attacks. Verify the token on form submission to ensure the request is legitimate.
6. Secure session management: Use secure session management techniques, such as regenerating session IDs after successful logins, setting secure session cookie attributes, and limiting session lifetimes.
7. Secure file uploads: Validate file uploads to prevent arbitrary file execution vulnerabilities. Restrict file types, limit file sizes, and store uploaded files outside the web root directory.
8. Access control and authorization: Implement proper access control and authorization mechanisms to restrict user access to sensitive resources. Use role-based access control (RBAC) or access control lists (ACL) to manage user permissions.
9. Secure configuration: Store sensitive configuration details, such as database credentials or API keys, outside the web root directory. Avoid hardcoding sensitive information directly in your code.
10. Regularly update and patch dependencies: Keep your PHP version and dependencies up to date to benefit from the latest security patches and bug fixes. Regularly check for updates and apply them as necessary.
Debugging and Troubleshooting PHP Code
Debugging and troubleshooting are essential skills for PHP developers to identify and fix issues in their code. Here are some tips for debugging and troubleshooting PHP code:
1. Enable error reporting: Set the error_reporting
directive in your PHP configuration to display all errors and warnings. This helps identify syntax errors and other issues.
2. Use error logging: Configure error logging in PHP to log errors and warnings to a file. This allows you to review the logs and identify recurring issues.
3. Use debugging tools: Utilize debugging tools like Xdebug or Zend Debugger to step through your code, set breakpoints, and inspect variables. These tools provide a more interactive debugging experience.
4. Print debugging statements: Use var_dump
, print_r
, or echo
statements to output variable values and trace execution flow. This helps identify unexpected behavior and track down issues.
5. Check server logs: Review server logs, such as Apache or Nginx error logs, for any relevant error messages or warnings. Server logs can provide insights into issues that may not be captured by PHP error logging.
6. Test in different environments: Test your code in different environments, such as development, staging, and production, to identify environment-specific issues. Reproduce the issue in a controlled environment for easier troubleshooting.
7. Break down the problem: If you encounter a complex issue, break it down into smaller parts and isolate the problematic code. This can help pinpoint the exact cause of the issue.
8. Use version control: Utilize version control systems like Git to track changes in your codebase. This allows you to revert to previous versions if issues arise after making changes.
9. Seek help from the community: If you’re unable to solve a problem on your own, seek help from the PHP community. Post questions on forums like Stack Overflow or join PHP-related communities to get assistance from experienced developers.
Related Article: How to Fix Error 419 Page Expired in Laravel Post Request
External Sources
– PHP Documentation
– Codecademy – Learn PHP
– YouTube – The Net Ninja PHP Tutorials