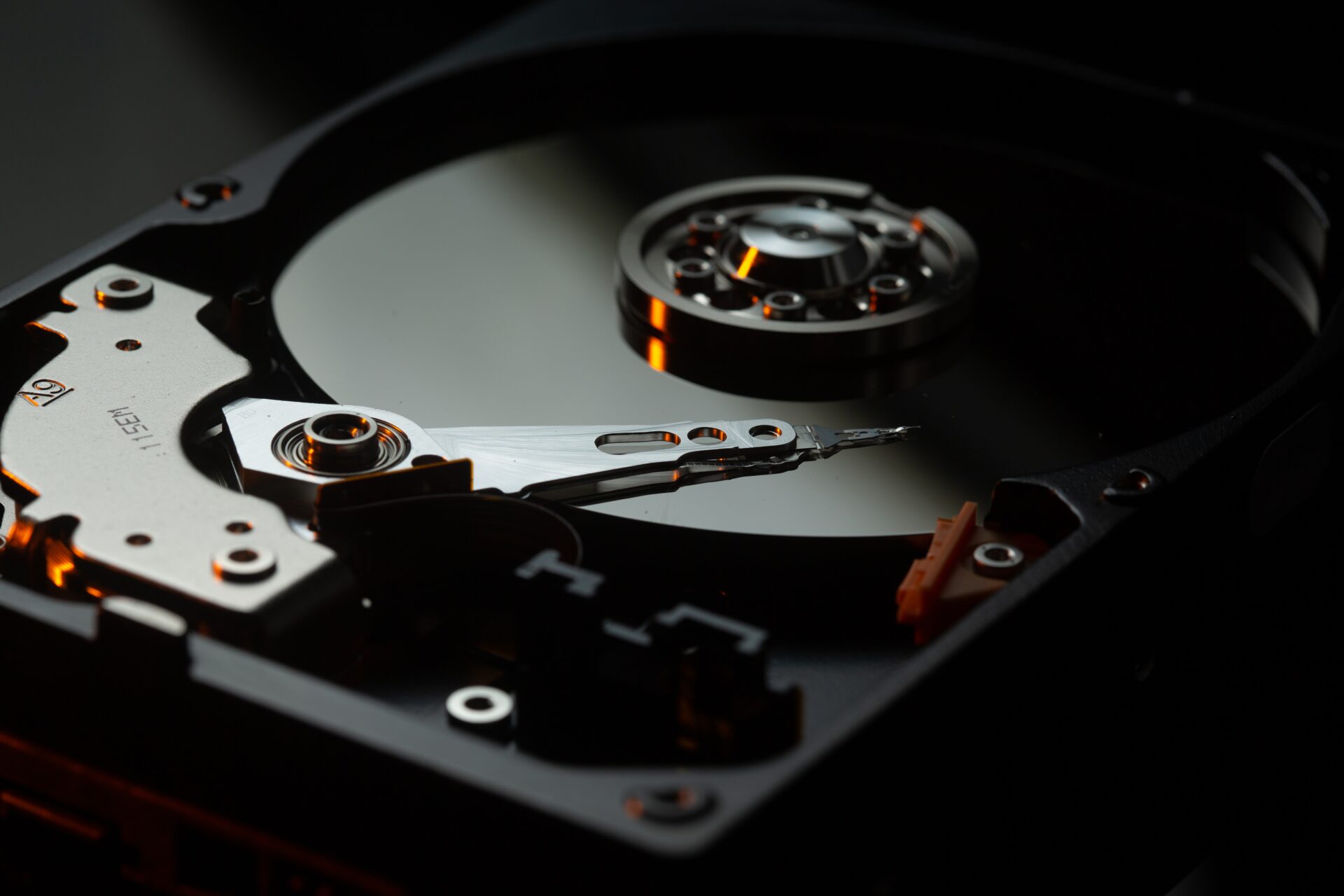
- Intro
- Understanding the Importance of Database Performance
- Best Practices and Tips
- Use select_related for ForeignKey and OneToOneField relationships
- Use prefetch_related for ManyToManyField relationships
- Use values() or values_list() to retrieve only the required fields from the database
- Use filter() instead of exclude() to narrow down the queryset
- Use annotate() to perform aggregations at the database level
- Use only() to fetch only the required fields from the database
- Use defer() to defer loading of less frequently used fields
- Transactions
- 1. Basic Transaction
- 2. Manual Commit
- 3. Using transaction.atomic() as a Decorator
- 4. Nested Transactions
- 5. Savepoint and Rollback
- Use Profiling
- Advanced Techniques
- Raw SQL Queries
- Database Indexing
- Database denormalization
- Database Query Optimization Tools
- Database Connection Pooling
- PostgreSQL
- Conclusion
Intro
As a Django developer, optimizing database queries is a crucial skill for improving the performance of your web applications and providing a better user experience when the requests hit the database. In the context of Django, Django rest framework (and its ecosystem), mastering database techniques can significantly enhance the speed and efficiency of your apps. In this article, we will explore various best practices and strategies for optimizing database queries to boost the performance of your web applications.
Related Article: How To Exit Python Virtualenv
Understanding the Importance of Database Performance
Efficient database optimization and performance is critical for the overall Django performance and responsiveness of web applications. Slow or inefficient database queries can lead to increased load times, decreased user experience, and decreased application performance. In Django, which follows the Object-Relational Mapping (ORM) pattern, database queries are performed using Python code, making it essential to optimize them to reduce the number of database queries, minimize data retrieval, and reduce the response time.
Best Practices and Tips
1. Use Database Indexes: Indexes are used to speed up database queries by allowing the database to quickly locate the rows that match the conditions in the query. By adding indexes to the appropriate fields in your models, you can significantly improve performance.
2. Optimize Database-related Queries: Django provides a powerful query API, known as the Django ORM, which allows you to interact with the database in an object-oriented manner. Use techniques such as selecting only the required fields, using queryset caching, and avoiding unnecessary database hits to reduce the number of database queries and improve performance.
3. Use select_related()
and prefetch_related()
methods: These methods allow you to improve database queries by reducing database hits when querying related objects. select_related()
fetches the related objects in a single statement using SQL joins, while prefetch_related fetches the related objects in a separate query, but still reduces the number of database hits compared to accessing related objects without optimization. Remember that querysets are lazy in Django,
4. Use QuerySet’s values()
and values_list()
methods: These methods allow you to fetch only the specific fields that you need from the database, rather than fetching all the fields of the model. This can significantly reduce the amount of data retrieved from the database, resulting in improved performance.
5. Use annotate() and aggregate() methods: These methods allow you to perform calculations and aggregations on the database side, rather than fetching all the data and performing calculations in Python code – also reducing the need for additional queries. This can help reduce the amount of data transferred from the database and improve performance.
6. Use Database Caching: Caching is a powerful technique for reducing database hits and improving performance. Django provides built-in caching support that allows you to cache the results of database queries, reducing the load on the database and improving response times.
7. Optimize the Database Schema: Normalize your database schema, use appropriate data types, and avoid redundant data to minimize storage space and reduce the complexity of database queries. A properly optimized database goes a long way in the journey of reducing response times.
8. Use Database Transactions: Database transactions allow you to group multiple database operations into a single transaction, which can improve performance by reducing the overhead of committing changes to the database. Django provides support for database transactions, allowing you to manage transactions manually or automatically.
9. Use Connection Pooling: Connection pooling allows you to reuse existing database connections instead of creating a new connection for every query, reducing the overhead of establishing a new database connection. There are third-party libraries available that provide connection pooling functionality, such as django-db-connection-pool
.
10. Monitor and Optimize Database Performance: Regularly monitor your database for performance problems using database monitoring tools, analyze query execution plans, and optimize slow or complex queries. Monitor your database server’s hardware resources, such as CPU, memory, and disk usage, and optimize them as needed to ensure optimal performance.
11. Use Asynchronous Database Operations: Django provides support for asynchronous database operations using asynchronous views, asynchronous task queues, and asynchronous database drivers. Asynchronous operations can help improve performance by allowing concurrent execution of multiple database operations and reducing the wait time for blocking database operations.
12. Database Triggers and Stored Procedures: Database triggers and stored procedures can offload certain database operations to the database server, reducing the need for repetitive database queries from the application code. This can help improve performance by reducing the amount of data transferred between the application and the database server. The downside of using triggers and procedures is related to potentially moving business logic out of the application, resulting in a code base that is hard to maintain.
13. Optimize Database Server Configuration: Properly configure your database server to optimize performance. This includes tuning database server parameters such as buffer sizes, cache sizes, and connection settings based on the specific requirements of your application.
14. Use Database Replication and Sharding: for large datasets and very high traffic and high load websites.
Use select_related for ForeignKey and OneToOneField relationships
# Without improvements books = Book.objects.all() for book in books: print(book.title) print(book.author.name) # With improvement books = Book.objects.select_related('author').all() for book in books: print(book.title) print(book.author.name)
Related Article: How to Integrate Python with MySQL for Database Queries
Use prefetch_related for ManyToManyField relationships
# Without improvement books = Book.objects.all() for book in books: print(book.title) print(book.genres.all()) # With improvement books = Book.objects.prefetch_related('genres').all() for book in books: print(book.title) print(book.genres.all())
Use values() or values_list() to retrieve only the required fields from the database
# Without improvement books = Book.objects.all() for book in books: print(book.title) # With improvement books = Book.objects.values('title').all() for book in books: print(book['title'])
Use filter() instead of exclude() to narrow down the queryset
# Without improvement books = Book.objects.all().exclude(status='published') # With improvement books = Book.objects.filter(status__ne='published')
Related Article: 16 Amazing Python Libraries You Can Use Now
Use annotate() to perform aggregations at the database level
# Without optimization books = Book.objects.all() total_pages = sum(book.page_count for book in books) # With optimization from django.db.models import Sum total_pages = Book.objects.aggregate(Sum('page_count'))['page_count__sum']
Use only() to fetch only the required fields from the database
# Without optimization books = Book.objects.all() for book in books: print(book.title) # With optimization books = Book.objects.only('title').all() for book in books: print(book.title)
Use defer() to defer loading of less frequently used fields
# Without optimization books = Book.objects.all() for book in books: print(book.title) # Accessing less frequently used field, e.g.description
print(book.description) # With optimization books = Book.objects.defer('description').all() for book in books: print(book.title) # Accessingdescription
will fetch from the database only when needed print(book.description)
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Transactions
Transactions are important to use in a web project in several scenarios:
1. Multiple Database Operations: When your app needs to perform multiple database operations that are related and need to be executed as a single unit of work, it’s important to use transactions. For example, when creating a new user account that involves inserting records into multiple tables, updating related data, and committing the changes as a whole, a transaction can ensure that all changes are committed or rolled back together.
2. Data Integrity: Transactions are crucial for maintaining data integrity in a web app. They help to ensure that the database is in a consistent state by allowing you to wrap multiple database operations in a transaction and either commit all changes or roll back if any operation fails. This prevents data from being left in an inconsistent state due to partial updates.
3. Concurrent Access: Transactions are essential in a web app that has concurrent access to the database, where multiple users or processes may be modifying the same data simultaneously. Transactions provide isolation and prevent issues such as race conditions or conflicts due to concurrent updates. They ensure that each transaction is executed in isolation and provides a consistent view of the data during the transaction’s lifespan.
4. Error Handling: Transactions can be used to handle errors. If an error occurs during a transaction, you can roll back the transaction to undo any changes made so far, and handle the error gracefully. This helps in maintaining data consistency and integrity in case of any unexpected errors during database operations.
5. Django Performance Optimization: In some cases, using transactions can also optimize performance by reducing database operations. By grouping multiple database operations within a single transaction, you can reduce the overhead of committing changes to the database multiple times and improve the overall performance of your app.
In summary, transactions are important to use in a Django app when you need to ensure data integrity, handle concurrent access, optimize performance, and execute multiple related database operations as a single unit of work. Carefully consider the requirements and characteristics of your application to determine when and how to use transactions effectively.
1. Basic Transaction:
from django.db import transaction # Wrap the database operation in a transaction with transaction.atomic(): # Code for performing database operations # ... # If an exception occurs within this block, the transaction will be rolled back
2. Manual Commit:
from django.db import transaction # Start a transaction manually transaction.set_autocommit(False) try: # Code for performing database operations # ... # Commit the transaction transaction.commit() except: # Rollback the transaction if an exception occurs transaction.rollback() finally: # Restore autocommit to default state transaction.set_autocommit(True)
Related Article: How To Convert A Tensor To Numpy Array In Tensorflow
3. Using transaction.atomic()
as a Decorator:
from django.db import transaction @transaction.atomic def my_view(request): # Code for performing database operations # ... # If an exception occurs within this function, the transaction will be rolled back
4. Nested Transactions:
from django.db import transaction # Outer transaction with transaction.atomic(): # Code for performing database operations # ... # Inner transaction with transaction.atomic(): # Code for performing more database operations # ... # If an exception occurs within this inner block, only the inner transaction will be rolled back, # while the outer transaction remains active
5. Savepoint and Rollback:
from django.db import transaction # Start a transaction manually transaction.set_autocommit(False) try: # Code for performing database operations # ... # Create a savepoint sid = transaction.savepoint() try: # Code for performing more database operations # ... # Rollback to the savepoint transaction.savepoint_rollback(sid) except: # Rollback the transaction if an exception occurs within the inner block transaction.rollback() except: # Rollback the transaction if an exception occurs within the outer block transaction.rollback() finally: # Restore autocommit to default state transaction.set_autocommit(True)
Note: It’s important to use transactions judiciously and consider the specific requirements and performance characteristics of your web app. Transactions should be used when necessary to ensure data consistency and integrity, but excessive use of transactions can impact performance. Always test and measure the performance of your application with transactions enabled to ensure optimal performance.
Related Article: How to Normalize a Numpy Array to a Unit Vector in Python
Use Profiling
Database profiling is an important technique for identifying performance bottlenecks and optimizing the number of queries. Here are some best practices for doing database profiling:
1. Django Debug Toolbar: Debug Toolbar is a third-party package that provides a set of panels displaying various debug information about database queries, cache usage, request/response headers, and more. It’s a powerful tool for profiling database queries in applications and identifying slow or inefficient queries.
Example usage:
# Install django-debug-toolbar using pip pip install django-debug-toolbar # Add 'debug_toolbar' to your Django app's settings.py file INSTALLED_APPS = [ # ... 'debug_toolbar', # ... ] # Include the debug_toolbar URLs in your Django app's urls.py file from django.conf.urls import include urlpatterns = [ # ... path('__debug__/', include('debug_toolbar.urls')), # ... ]
2. Django Silk: Silk is another popular third-party package for database profiling. It provides a set of middleware and a web interface for analyzing and profiling database queries, cache usage, and view functions. Silk also allows you to log query execution times and analyze them for performance optimization.
Example usage:
# Install django-silk using pip pip install django-silk # Add 'silk' to your Django app's settings.py file INSTALLED_APPS = [ # ... 'silk', # ... ] # Include the silk URLs in your app's urls.py file from silk import urls as silk_urls urlpatterns = [ # ... path('silk/', include(silk_urls)), # ... ]
3. Built-in logging: Django has built-in logging capabilities that can be used to log database query execution times and analyze them for performance profiling. You can configure logging settings in your Django app’s settings.py file to capture query execution times, analyze them, and optimize database queries accordingly.
Example usage:
# Add the following logging settings to your app's settings.py file LOGGING = { 'loggers': { 'django.db.backends': { 'level': 'DEBUG', 'handlers': ['console'], }, }, }
4. QuerySet API: Django’s QuerySet API provides built-in methods such as query.explain()
and query.count()
that can be used to profile and analyze database queries. These methods allow you to examine the query execution plan, count the number of database queries executed, and analyze them for optimization.
Example usage:
# Use explain() to print the query execution plan queryset = MyModel.objects.filter(...) # replace with your actual queryset queryset = queryset.explain() # Use count() to count the number of database queries executed queryset = MyModel.objects.filter(...) # replace with your actual queryset queryset.count()
In addition to the above techniques, you can also use APM services (tools like New Relic, Datadog) and database monitoring tools, such as slow query logs provided by your database server, to profile and optimize database queries in your application. By using a combination of these techniques, you can effectively profile, analyze, and optimize database queries to boost the performance of your application.
Advanced Techniques
Django provides several advanced database query techniques that can help improve the performance of your web applications. Here are some optimization tips worth experimenting with:
Raw SQL Queries
Django allows you to execute raw SQL queries using the django.db.connections
object, which gives you direct access to the database connection. This can be useful for complex queries or performance-critical operations where you need fine-grained control over the SQL query generation and execution.
Example:
from django.db import connections # Retrieve a raw SQL query with connections['my_db_alias'].cursor() as cursor: cursor.execute('SELECT * FROM myapp_mymodel WHERE my_field = %s', [my_value]) rows = cursor.fetchall()
Related Article: How to Adjust Font Size in a Matplotlib Plot
Database Indexing
Database indexing is a powerful technique for improving relational database queries. Django allows you to define database indexes (similar to a primary key) using the db_index
attribute on model fields or by using the db_index=True
argument when creating fields. This can significantly speed up queries that involve indexed fields.
Example:
class MyModel(models.Model): my_field = models.CharField(max_length=100, db_index=True) # ...
Database denormalization
Denormalization is the process of duplicating data across tables to eliminate the need for complex joins and reduce the number of database queries. In Django, you can denormalize your database by using fields like ForeignKey
, OneToOneField
, or ManyToManyField
to store related data in the same table, eliminating the need for additional database queries to fetch related data.
Example:
class Author(models.Model): name = models.CharField(max_length=100) # ... class Book(models.Model): title = models.CharField(max_length=100) author = models.ForeignKey(Author, on_delete=models.CASCADE) # ...
Database Query Optimization Tools
Django provides several third-party tools and libraries that can help you optimize database queries. For example, tools like django-silk
and django-debug-toolbar
provide detailed insights into database queries, allowing you to identify and optimize slow or redundant queries. These tools can be invaluable for diagnosing and fixing performance issues in your applications.
Related Article: How to Position the Legend Outside the Plot in Matplotlib
Database Connection Pooling
Database connection pooling is a technique that allows you to reuse database connections instead of creating a new connection for every query. Django provides third-party libraries like django-db-connection-pool
that implement database connection pooling, which can help reduce the overhead of creating and tearing down database connections, resulting in improved performance.
Here’s an example of how you can implement database connection pooling in Django using the django-db-connection-pool
library.
1. Install the django-db-connection-pool
library using pip:
pip install django-db-connection-pool
2. Update your Django settings to configure the database connection pooling:
# settings.py # Import the required library from django_db_connection_pool import * # Configure the database connection pooling DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'your_database_name', 'USER': 'your_database_user', 'PASSWORD': 'your_database_password', 'HOST': 'your_database_host', 'PORT': 'your_database_port', 'POOL': { 'MAX_OVERFLOW': 10, 'POOL_SIZE': 10, 'RECYCLE': 300, 'USE_THREADLOCAL': True, }, }, }
In the above example, we are using the django-db-connection-pool
library to configure the database connection pooling settings. We have set the maximum overflow to 10, the pool size to 10, and the recycle time to 300 seconds (5 minutes). We are also using the USE_THREADLOCAL
option to enable thread-local connections for thread-safety.
3. Use the database connection as usual in your views or models:
# views.py from django.db import connections def my_view(request): # Get a database connection from the pool with connections['default'].cursor() as cursor: # Execute a query cursor.execute('SELECT * FROM myapp_mymodel WHERE my_field = %s', [my_value]) rows = cursor.fetchall() # ...
In the above example, we are using the connections
object to get a database connection from the pool and execute a raw SQL query. The library will handle the connection pooling for us, reusing existing connections from the pool instead of creating a new connection for every query, thus improving performance.
Note: It’s important to thoroughly test and benchmark the performance of your application with and without connection pooling to determine its effectiveness in your specific use case. Also, remember to configure the connection pool settings appropriately based on your application’s needs and the characteristics of your database server.
These are just a few examples of advanced database query optimizations in Django. Depending on the specific requirements and characteristics of your web application, other techniques like database partitioning, query batching, and query caching can also be considered for further performance optimization. It’s important to thoroughly analyze and profile your database queries and choose the appropriate optimization techniques based on your application’s needs.
PostgreSQL
When using Postgres as the database backend for an application, there are several performance optimizations that can be applied to improve the overall performance of the application. Here are some performance optimizations:
1. Indexing: Indexes are used to speed up database queries by providing a fast lookup mechanism. You can use various types of indexes such as B-tree, Hash, GIN, GIST, and SP-GiST, depending on the type of data and query patterns in your app. By properly indexing the database tables used in your models, you can significantly improve query performance.
Example usage:
from django.db import models class MyModel(models.Model): field1 = models.CharField(max_length=100, db_index=True) # Use db_index=True to create an index field2 = models.IntegerField() # ...
2. Query Optimization: PostgreSQL provides various query optimization techniques such as query planning, query execution, and caching. By analyzing and optimizing the SQL queries generated by Django ORM, you can boost the performance of your Django project. You can use Django’s QuerySet API to optimize queries by chaining filters, using select_related()
to fetch related objects efficiently, and using only()
or defer()
to specify the fields to be fetched.
Example usage:
# Chaining filters to optimize queries queryset = MyModel.objects.filter(field1=value1).filter(field2=value2) # Using select_related() to fetch related objects efficiently queryset = MyModel.objects.filter(...).select_related('related_model') # Using only() or defer() to specify fields to be fetched queryset = MyModel.objects.only('field1', 'field2').filter(...) queryset = MyModel.objects.defer('field3').filter(...)
3. Connection Pooling: Connection pooling is a technique that helps to reuse existing database connections instead of creating a new connection for every database query. This can significantly reduce the overhead of establishing and tearing down database connections, resulting in improved performance. You can use third-party libraries such as psycopg2
or django-db-multitenant
to implement connection pooling in your Django app.
Example usage:
# Using psycopg2 connection pooling import psycopg2.pool # Create a connection pool pg_pool = psycopg2.pool.SimpleConnectionPool( minconn=5, # Minimum number of connections maxconn=20, # Maximum number of connections dbname='mydb', # Database name user='myuser', # Database user password='mypassword', # Database password host='localhost', # Database host port='5432', # Database port ) # Get a connection from the pool conn = pg_pool.getconn() # Use the connection for database queries cur = conn.cursor() cur.execute('SELECT * FROM myapp_mymodel') rows = cur.fetchall() # Release the connection back to the pool pg_pool.putconn(conn)
4. Configuration Tuning: PostgreSQL provides various configuration settings that can be tuned to optimize performance. Some common settings that can be adjusted for performance improvement include shared_buffers
, work_mem
, maintenance_work_mem
, and effective_cache_size
. These settings can be adjusted in the PostgreSQL configuration file or using the ALTER SYSTEM
command.
Example usage:
# Adjusting shared_buffers in postgresql.conf shared_buffers = 256MB # Adjusting work_mem in postgresql.conf work_mem = 8MB # Adjusting maintenance_work_mem in postgresql.conf maintenance_work_mem = 256MB # Adjusting effective_cache_size in postgresql.conf effective_cache_size
Conclusion
Optimizing database queries is a critical aspect of Python and Django web development for improving the performance of your web applications. By following best practices, utilizing queryset methods, caching query results, optimizing database relationships, and analyzing queries, you can greatly enhance the efficiency and speed of your Django applications. By mastering database query optimization techniques in Django, you can unlock the full potential of this powerful web framework and create high-performance web applications that provide a seamless user experience.