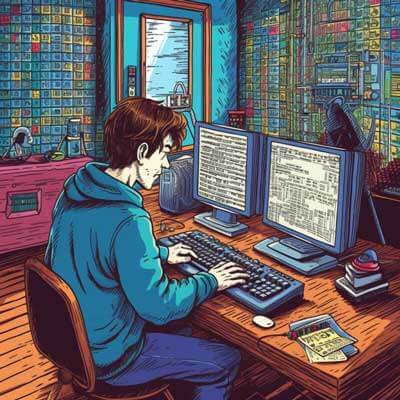
Table of Contents
Introduction to Data Types
Data types are a fundamental concept in programming languages, including Python. In Python, data types define the nature of variables and the operations that can be performed on them. Understanding data types is crucial for effectively using and manipulating data in Python.
Related Article: How to Use Python's Not Equal Operator
Fundamentals of Data Types
In Python, there are several built-in data types that are commonly used. These include:
- Numeric data types: integers, floats, and complex numbers
- Textual data types: strings
- Sequence data types: lists, tuples, and ranges
- Mapping data types: dictionaries
- Set data types: set and frozenset
- Boolean data type: True and False
- Binary data types: bytes and bytearray
Let's explore each of these data types in more detail.
Numeric Data Types and Their Manipulation
Numeric data types in Python are used to represent numbers. There are three main numeric data types: integers, floats, and complex numbers.
Integers are whole numbers without a decimal point. They can be positive or negative. Here's an example of defining an integer variable:
x = 5
Floats are numbers with a decimal point. They can also be positive or negative. Here's an example of defining a float variable:
y = 3.14
Complex numbers are numbers with a real part and an imaginary part. They are defined using the j
or J
suffix. Here's an example of defining a complex number variable:
z = 2 + 3j
Python provides various operations for manipulating numeric data types, such as addition, subtraction, multiplication, and division. Here are a few examples:
# Addition result = x + y print(result) # Output: 8.14 # Subtraction result = x - y print(result) # Output: 1.86 # Multiplication result = x * y print(result) # Output: 15.7 # Division result = x / y print(result) # Output: 1.5923566878980892
Textual Data Types and Their Manipulation
Textual data types in Python are used to represent strings of characters. A string is a sequence of characters enclosed in single quotes ('
) or double quotes ("
). Here's an example of defining a string variable:
message = "Hello, World!"
Python provides several operations and methods for manipulating strings. Here are a few examples:
# Concatenation greeting = "Hello" name = "Alice" message = greeting + " " + name print(message) # Output: Hello Alice # Length length = len(message) print(length) # Output: 11 # Accessing characters first_char = message[0] print(first_char) # Output: H last_char = message[-1] print(last_char) # Output: e substring = message[7:12] print(substring) # Output: World # String methods uppercase_message = message.upper() print(uppercase_message) # Output: HELLO, WORLD! lowercase_message = message.lower() print(lowercase_message) # Output: hello, world! # String formatting formatted_message = "Hello, {}. Today is {}.".format(name, "Monday") print(formatted_message) # Output: Hello, Alice. Today is Monday.
Related Article: Optimizing FastAPI Applications: Modular Design, Logging, and Testing
Sequence Data Types: Lists, Tuples, and Range
Sequence data types in Python are used to represent ordered collections of items. There are three main sequence data types: lists, tuples, and ranges.
A list is a mutable sequence of items enclosed in square brackets ([]
). Here's an example of defining a list variable:
fruits = ["apple", "banana", "orange"]
A tuple is an immutable sequence of items enclosed in parentheses (()
). Here's an example of defining a tuple variable:
colors = ("red", "green", "blue")
A range is a sequence of numbers. It is commonly used in for loops. Here's an example of defining a range variable:
numbers = range(1, 5)
Python provides various operations and methods for manipulating sequence data types. Here are a few examples:
# Accessing items first_fruit = fruits[0] print(first_fruit) # Output: apple last_color = colors[-1] print(last_color) # Output: blue # Slicing sliced_fruits = fruits[1:3] print(sliced_fruits) # Output: ['banana', 'orange'] # Length num_colors = len(colors) print(num_colors) # Output: 3 # Concatenation combined = fruits + colors print(combined) # Output: ['apple', 'banana', 'orange', 'red', 'green', 'blue'] # Range iteration for number in numbers: print(number) # Output: 1 2 3 4
Mapping Data Types: Dictionaries
Mapping data types in Python are used to represent key-value pairs. The main mapping data type in Python is the dictionary.
A dictionary is an unordered collection of key-value pairs enclosed in curly braces ({}
). Here's an example of defining a dictionary variable:
person = { "name": "Alice", "age": 30, "city": "New York" }
Python provides various operations and methods for manipulating dictionaries. Here are a few examples:
# Accessing values name = person["name"] print(name) # Output: Alice # Updating values person["age"] = 31 # Adding new key-value pairs person["country"] = "USA" # Length num_items = len(person) print(num_items) # Output: 4 # Iterating over keys and values for key, value in person.items(): print(key, value) # Output: name Alice age 31 city New York country USA
Set Data Types: Set and Frozenset
Set data types in Python are used to represent unordered collections of unique elements. There are two main set data types: set and frozenset.
A set is a mutable collection of unique elements enclosed in curly braces ({}
). Here's an example of defining a set variable:
fruits = {"apple", "banana", "orange"}
A frozenset is an immutable collection of unique elements enclosed in parentheses (()
). Here's an example of defining a frozenset variable:
colors = frozenset(["red", "green", "blue"])
Python provides various operations and methods for manipulating set data types. Here are a few examples:
# Adding elements fruits.add("pear") # Removing elements fruits.remove("banana") # Set operations all_fruits = fruits.union(colors) print(all_fruits) # Output: {'apple', 'orange', 'green', 'blue', 'red'} common_fruits = fruits.intersection(colors) print(common_fruits) # Output: set() # Length num_colors = len(colors) print(num_colors) # Output: 3 # Iterating over elements for fruit in fruits: print(fruit) # Output: apple orange pear
Boolean Data Type and Its Manipulation
Boolean data type in Python is used to represent truth values. There are two possible boolean values: True and False.
Boolean values are commonly used in conditional statements and logical operations. Here are a few examples:
# Conditional statements x = 5 if x > 0: print("Positive") # Logical operations is_raining = True is_sunny = False # Logical AND if is_raining and not is_sunny: print("Take an umbrella") # Logical OR if is_raining or is_sunny: print("Weather is unpredictable") # Logical NOT if not is_sunny: print("It's not sunny")
Related Article: How To Use Python'S Equivalent For A Case Switch Statement
Binary Data Types
Binary data types in Python are used to represent sequences of bytes. There are two main binary data types: bytes and bytearray.
A bytes object is an immutable sequence of bytes. It is defined using the b
prefix before a string literal. Here's an example of defining a bytes variable:
data = b"Hello"
A bytearray object is a mutable sequence of bytes. It is defined using the bytearray()
constructor. Here's an example of defining a bytearray variable:
data = bytearray(b"Hello")
Binary data types are commonly used when working with binary files or when encoding and decoding data. Here are a few examples:
# Accessing elements first_byte = data[0] print(first_byte) # Output: 72 # Updating elements data[1] = 101 # Length num_bytes = len(data) print(num_bytes) # Output: 5 # Binary operations binary_or = data[0] | data[1] print(binary_or) # Output: 101 binary_xor = data[0] ^ data[1] print(binary_xor) # Output: 37
Type Conversion in Real-World Scenarios
In real-world scenarios, you often need to convert data between different data types. Python provides built-in functions for type conversion, allowing you to convert data from one type to another.
Here are a few examples of type conversion:
# Converting numeric data types x = 5 y = float(x) print(y) # Output: 5.0 z = complex(x) print(z) # Output: (5+0j) # Converting textual data types message = "123" number = int(message) print(number) # Output: 123 # Converting sequence data types fruits = ["apple", "banana", "orange"] fruits_tuple = tuple(fruits) print(fruits_tuple) # Output: ('apple', 'banana', 'orange') # Converting mapping data types person = { "name": "Alice", "age": 30 } person_list = list(person.items()) print(person_list) # Output: [('name', 'Alice'), ('age', 30)] # Converting set data types colors_set = set(colors) print(colors_set) # Output: {'blue', 'green', 'red'} # Converting boolean data type is_positive = bool(x > 0) print(is_positive) # Output: True
Data Types: Use Cases
Different data types in Python have specific use cases based on their characteristics and properties. Here are a few use cases for different data types:
- Numeric data types: Used for arithmetic calculations, scientific computations, and representing numerical data.
- Textual data types: Used for manipulating and processing textual data, such as strings, file contents, and user input.
- Sequence data types: Used for representing ordered collections of items, such as lists of names, tuples of coordinates, and ranges of numbers.
- Mapping data types: Used for representing key-value pairs, such as dictionaries of configuration settings, database records, and JSON data.
- Set data types: Used for handling collections of unique elements and performing set operations, such as finding common elements and removing duplicates.
- Boolean data type: Used for representing truth values and controlling program flow based on conditions.
- Binary data types: Used for working with binary files, encoding and decoding data, and performing bitwise operations.
Understanding the appropriate use cases for different data types is essential for writing efficient and effective Python code.
Best Practices for Using Data Types
When working with data types in Python, it is important to follow best practices to ensure code readability, maintainability, and performance. Here are a few best practices for using data types:
- Use meaningful variable names: Choose variable names that accurately describe the data they represent. This improves code readability and makes it easier to understand the purpose of variables.
- Use type annotations: Type annotations provide hints about the expected types of variables and function return values. They help improve code clarity and enable static type checking tools to catch type-related errors.
- Avoid unnecessary type conversions: Convert data types only when necessary. Unnecessary type conversions can introduce overhead and potentially lead to errors or loss of information.
- Be aware of data type limitations: Understand the limitations and characteristics of different data types. For example, floating-point numbers may have precision issues, and strings are immutable.
- Handle data type errors gracefully: When working with user input or external data, validate and sanitize the input to prevent unexpected data type errors. Use exception handling to gracefully handle errors and provide meaningful error messages.
Related Article: How to Find a Value in a Python List
Performance Considerations: Numeric Data Types
When working with numeric data types in Python, it is important to consider performance implications, especially when dealing with large datasets or computationally intensive operations.
Here are a few performance considerations for numeric data types:
- Use appropriate data types: Choose the most appropriate numeric data type for your specific use case. For example, if you don't need decimal precision, use integers instead of floats.
- Be aware of integer overflow: Python integers have unlimited precision, but this comes at the cost of performance. If you know the range of values you're working with, consider using fixed-size integer types like numpy.int32
for better performance.
- Use built-in functions and libraries: Python provides built-in functions and libraries for optimized numerical computations, such as math
and numpy
. Utilize these functions and libraries when performing complex mathematical operations.
- Minimize unnecessary operations: Avoid unnecessary mathematical operations or redundant computations. These can have a significant impact on performance, especially when performed inside loops or on large datasets.
- Use vectorized operations: Whenever possible, utilize vectorized operations provided by libraries like numpy
. These operations are optimized for performance and can significantly speed up numerical computations.
By considering these performance considerations, you can optimize your numeric computations in Python.
Performance Considerations: Textual Data Types
When working with textual data types in Python, such as strings, it is important to consider performance implications, especially when dealing with large strings or repetitive string operations.
Here are a few performance considerations for textual data types:
- Use string interpolation instead of concatenation: String interpolation, using formatted string literals or the format()
method, is generally faster and more readable than concatenating multiple strings.
- Utilize string methods efficiently: Python provides various string methods for manipulating and processing strings. Be aware of the performance characteristics of these methods and choose the most efficient ones for your specific use case.
- Use list comprehension for string manipulation: List comprehension can often be faster than traditional string concatenation methods, especially when performing complex string operations on large datasets.
- Avoid unnecessary string conversions: Convert between string representations only when necessary. Unnecessary conversions can introduce overhead and degrade performance.
- Consider using join()
for concatenating multiple strings: When concatenating many strings, using the join()
method can be more efficient than repeatedly concatenating strings with the +
operator.
- Be mindful of string immutability: Remember that strings in Python are immutable, meaning they cannot be modified in-place. Each string manipulation operation usually creates a new string object, which can impact performance when performed repeatedly in a loop or on large strings.
By considering these performance considerations, you can optimize your string operations in Python.
Performance Considerations: Sequence Data Types
When working with sequence data types in Python, such as lists, tuples, and ranges, it is important to consider performance implications, especially when dealing with large collections or performing frequent sequence operations.
Here are a few performance considerations for sequence data types:
- Prefer lists for mutable sequences: If you need to modify the elements of a sequence, use a list instead of a tuple. Lists are mutable and allow in-place modifications, whereas tuples are immutable and require creating a new tuple object for each modification.
- Utilize list comprehensions and generator expressions: List comprehensions and generator expressions are concise and efficient ways to create new lists or perform filtering and mapping operations on existing lists.
- Consider the appropriate data structure for your use case: Depending on your specific requirements, you may need to choose a different sequence data type. For example, if you frequently need to check for membership, a set may be more appropriate than a list.
- Be aware of the time complexity of sequence operations: Different sequence operations have different time complexities. For example, appending an element to a list is an O(1) operation, whereas inserting an element in the middle of a list is an O(n) operation.
- Use slicing and indexing efficiently: Slicing and indexing operations can be used to extract specific elements or ranges from a sequence. Be mindful of the time complexity of these operations and use them efficiently, especially when working with large sequences.
- Consider using built-in functions and libraries: Python provides built-in functions and libraries for efficient sequence operations, such as map()
, filter()
, and itertools
. Utilize these functions and libraries when performing complex sequence manipulations.
By considering these performance considerations, you can optimize your sequence operations in Python.
Performance Considerations: Mapping Data Types
When working with mapping data types in Python, such as dictionaries, it is important to consider performance implications, especially when dealing with large dictionaries or performing frequent dictionary operations.
Here are a few performance considerations for mapping data types:
- Prefer dictionaries for key-value mappings: If you need to store key-value pairs, use a dictionary instead of a list or tuple. Dictionaries provide fast and efficient lookup based on keys.
- Utilize dictionary comprehensions: Dictionary comprehensions are concise and efficient ways to create new dictionaries or perform filtering and mapping operations on existing dictionaries.
- Be aware of the time complexity of dictionary operations: Dictionary operations such as insertion, deletion, and lookup have an average time complexity of O(1). However, in the worst case, these operations can have a time complexity of O(n), where n is the number of elements in the dictionary.
- Use built-in functions and methods efficiently: Python provides various built-in functions and methods for mapping operations, such as keys()
, values()
, and items()
. Be aware of the performance characteristics of these functions and methods and choose the most efficient ones for your specific use case.
- Minimize unnecessary dictionary operations: Avoid unnecessary dictionary operations or redundant computations. These can have a significant impact on performance, especially when performed inside loops or on large dictionaries.
By considering these performance considerations, you can optimize your dictionary operations in Python.
Related Article: How To Create Pandas Dataframe From Variables - Valueerror
Performance Considerations: Set Data Types
When working with set data types in Python, such as sets and frozensets, it is important to consider performance implications, especially when dealing with large sets or performing frequent set operations.
Here are a few performance considerations for set data types:
- Prefer sets for unique elements: If you need to store unique elements, use a set instead of a list or tuple. Sets provide fast and efficient membership testing and eliminate duplicates automatically.
- Utilize set comprehensions: Set comprehensions are concise and efficient ways to create new sets or perform filtering and mapping operations on existing sets.
- Be aware of the time complexity of set operations: Set operations such as membership testing, insertion, deletion, and intersection have an average time complexity of O(1). However, in the worst case, these operations can have a time complexity of O(n), where n is the number of elements in the set.
- Use built-in functions and methods efficiently: Python provides various built-in functions and methods for set operations, such as union()
, intersection()
, and difference()
. Be aware of the performance characteristics of these functions and methods and choose the most efficient ones for your specific use case.
- Minimize unnecessary set operations: Avoid unnecessary set operations or redundant computations. These can have a significant impact on performance, especially when performed inside loops or on large sets.
By considering these performance considerations, you can optimize your set operations in Python.
Performance Considerations: Boolean and Binary Data Types
When working with boolean and binary data types in Python, such as booleans, bytes, and bytearrays, it is important to consider performance implications, especially when dealing with large datasets or performing frequent boolean or binary operations.
Here are a few performance considerations for boolean and binary data types:
- Use boolean operators efficiently: Python provides boolean operators (and
, or
, not
) for combining boolean values. Be mindful of short-circuiting behavior and use them efficiently to avoid unnecessary evaluations.
- Utilize bitwise operations: Bitwise operations (&
, |
, ^
, ~
) can be used to manipulate individual bits in binary data. These operations are generally faster than boolean operations when working with binary data.
- Be aware of the time complexity of binary operations: Some binary operations, such as bitwise shifting or operations on large bytearrays, can have a time complexity of O(n), where n is the number of bits or bytes being manipulated.
- Use built-in functions and methods efficiently: Python provides various built-in functions and methods for boolean and binary operations, such as bin()
, hex()
, and bit_length()
. Be aware of the performance characteristics of these functions and methods and choose the most efficient ones for your specific use case.
- Minimize unnecessary boolean and binary operations: Avoid unnecessary boolean and binary operations or redundant computations. These can have a significant impact on performance, especially when performed inside loops or on large datasets.
By considering these performance considerations, you can optimize your boolean and binary operations in Python.
Advanced Techniques: Immutable vs Mutable Data Types
In Python, data types can be classified as either immutable or mutable. Immutable data types cannot be modified once they are created, whereas mutable data types can be modified.
Immutable data types include numeric types (int, float, complex), string, tuple, frozenset, and bytes. Mutable data types include list, dictionary, set, bytearray, and user-defined classes.
Understanding the difference between immutable and mutable data types is important for writing efficient and bug-free code. Here are a few key points to consider:
- Immutable data types are hashable and can be used as keys in dictionaries or elements in sets.
- Immutable data types are safer to use in multi-threaded or concurrent environments because they cannot be modified by multiple threads simultaneously.
- Mutable data types allow in-place modifications, which can be more efficient than creating a new object for each modification.
- Mutable data types can have aliasing issues. If two variables refer to the same mutable object, modifying the object through one variable will also affect the other variable.
When choosing between mutable and immutable data types, consider the specific requirements of your use case. If you need to modify the object frequently or store it in a data structure that requires hashability, mutable data types may be more appropriate. Otherwise, immutable data types are generally preferred for their safety and ease of use.
Advanced Techniques: Dynamic Typing
Python is a dynamically typed language, which means that variables can hold values of different types during the execution of a program. This flexibility allows for more concise and expressive code, but it also introduces some considerations.
Here are a few key points to understand about dynamic typing in Python:
- Variables in Python don't have explicit type declarations. The type of a variable is determined dynamically based on the value assigned to it.
- Dynamic typing allows for more flexibility and makes it easier to write code quickly. However, it also requires careful attention to avoid type-related errors.
- The type()
function can be used to determine the type of a variable at runtime. For example, type(x)
returns the type of variable x
.
- Python uses a combination of reference counting and garbage collection to manage memory. This allows variables to be dynamically allocated and deallocated as needed.
- Dynamic typing can lead to runtime errors if the wrong type of value is assigned to a variable or if incompatible operations are performed on variables of different types. Proper testing and error handling can help mitigate these issues.
Dynamic typing is one of Python's powerful features, but it also requires careful consideration and testing to ensure code correctness and avoid type-related errors.
Related Article: Python Async Programming: A Beginner's Guide
Advanced Techniques: Type Annotations
Type annotations in Python provide a way to specify the expected types of variables, function parameters, and return values. While Python is dynamically typed, type annotations allow for static type checking and improved code clarity.
Here's an example of using type annotations:
def add(a: int, b: int) -> int: return a + b
In this example, the add()
function takes two integer parameters a
and b
and returns an integer. The type annotations provide hints to both humans and static type checkers about the expected types.
Type annotations are not enforced at runtime by the Python interpreter. However, they can be checked using static type checkers like Mypy. Static type checking helps catch type-related errors early in the development process and improves code maintainability.
Type annotations can be added to variables, function parameters, return values, and class attributes. They support standard Python types as well as user-defined types and external libraries.
While type annotations are optional, using them can significantly improve code clarity, especially in larger projects or when working in a team. It is recommended to adopt type annotations gradually and use a static type checker to catch potential type errors.
Code Snippet: Numeric Data Types
Here's an example code snippet demonstrating the use of numeric data types in Python:
# Integer x = 5 # Float y = 3.14 # Complex z = 2 + 3j # Addition result = x + y print(result) # Output: 8.14 # Subtraction result = x - y print(result) # Output: 1.86 # Multiplication result = x * y print(result) # Output: 15.7 # Division result = x / y print(result) # Output: 1.5923566878980892
This code snippet demonstrates the creation and manipulation of integer, float, and complex number variables. It also shows common arithmetic operations performed on numeric data types.
Code Snippet: Textual Data Types
Here's an example code snippet demonstrating the use of textual data types in Python:
# String concatenation greeting = "Hello" name = "Alice" message = greeting + " " + name print(message) # Output: Hello Alice # String length length = len(message) print(length) # Output: 11 # Accessing characters first_char = message[0] print(first_char) # Output: H last_char = message[-1] print(last_char) # Output: e substring = message[7:12] print(substring) # Output: World # String methods uppercase_message = message.upper() print(uppercase_message) # Output: HELLO, WORLD! lowercase_message = message.lower() print(lowercase_message) # Output: hello, world! # String formatting formatted_message = "Hello, {}. Today is {}.".format(name, "Monday") print(formatted_message) # Output: Hello, Alice. Today is Monday.
This code snippet demonstrates string manipulation operations, such as concatenation, length calculation, character access, slicing, and common string methods.
Code Snippet: Sequence Data Types
Here's an example code snippet demonstrating the use of sequence data types in Python:
# List fruits = ["apple", "banana", "orange"] # Tuple colors = ("red", "green", "blue") # Range numbers = range(1, 5) # Accessing items first_fruit = fruits[0] print(first_fruit) # Output: apple last_color = colors[-1] print(last_color) # Output: blue # Slicing sliced_fruits = fruits[1:3] print(sliced_fruits) # Output: ['banana', 'orange'] # Length num_colors = len(colors) print(num_colors) # Output: 3 # Concatenation combined = fruits + colors print(combined) # Output: ['apple', 'banana', 'orange', 'red', 'green', 'blue'] # Range iteration for number in numbers: print(number) # Output: 1 2 3 4
This code snippet demonstrates the creation and manipulation of list, tuple, and range variables. It also shows common operations performed on sequence data types, such as item access, slicing, length calculation, and concatenation.
Related Article: Python's Dict Tutorial: Is a Dictionary a Data Structure?
Code Snippet: Mapping Data Types
Here's an example code snippet demonstrating the use of mapping data types in Python:
# Dictionary person = { "name": "Alice", "age": 30, "city": "New York" } # Accessing values name = person["name"] print(name) # Output: Alice # Updating values person["age"] = 31 # Adding new key-value pairs person["country"] = "USA" # Length num_items = len(person) print(num_items) # Output: 4 # Iterating over keys and values for key, value in person.items(): print(key, value) # Output: name Alice age 31 city New York country USA
This code snippet demonstrates the creation and manipulation of dictionary variables. It shows how to access values, update values, add new key-value pairs, calculate the length, and iterate over keys and values.
Code Snippet: Set Data Types
Here's an example code snippet demonstrating the use of set data types in Python:
# Set fruits = {"apple", "banana", "orange"} # Frozenset colors = frozenset(["red", "green", "blue"]) # Adding elements fruits.add("pear") # Removing elements fruits.remove("banana") # Set operations all_fruits = fruits.union(colors) print(all_fruits) # Output: {'apple', 'orange', 'green', 'blue', 'red'} common_fruits = fruits.intersection(colors) print(common_fruits) # Output: set() # Length num_colors = len(colors) print(num_colors) # Output: 3 # Iterating over elements for fruit in fruits: print(fruit) # Output: apple orange pear
This code snippet demonstrates the creation and manipulation of set and frozenset variables. It shows how to add and remove elements, perform set operations (union and intersection), calculate the length, and iterate over elements.
Code Snippet: Boolean and Binary Data Types
Here's an example code snippet demonstrating the use of boolean and binary data types in Python:
# Conditional statements x = 5 if x > 0: print("Positive") # Logical operations is_raining = True is_sunny = False # Logical AND if is_raining and not is_sunny: print("Take an umbrella") # Logical OR if is_raining or is_sunny: print("Weather is unpredictable") # Logical NOT if not is_sunny: print("It's not sunny") # Binary operations data = bytearray(b"Hello") # Accessing elements first_byte = data[0] print(first_byte) # Output: 72 # Updating elements data[1] = 101 # Length num_bytes = len(data) print(num_bytes) # Output: 5 # Binary operations binary_or = data[0] | data[1] print(binary_or) # Output: 101 binary_xor = data[0] ^ data[1] print(binary_xor) # Output: 37
This code snippet demonstrates the use of boolean values in conditional statements and logical operations. It also shows the creation and manipulation of binary data using the bytearray data type.
Error Handling and Data Types
When working with data types in Python, it is important to handle potential errors that may occur during operations or conversions. Error handling allows you to gracefully handle exceptions and prevent program crashes.
Here's an example of error handling when working with data types:
# Error handling for type conversion try: number = int("123") print(number) # Output: 123 except ValueError: print("Invalid number") # Error handling for out-of-bounds index fruits = ["apple", "banana", "orange"] try: fruit = fruits[3] print(fruit) # This line won't execute except IndexError: print("Invalid index") # Error handling for key not found person = { "name": "Alice", "age": 30 } try: city = person["city"] print(city) # This line won't execute except KeyError: print("Key not found")
This code snippet demonstrates error handling using the try-except
statement. It shows how to catch and handle specific types of errors that may occur when working with data types, such as ValueError
, IndexError
, and KeyError
. By handling these errors, you can provide meaningful error messages and prevent program crashes.