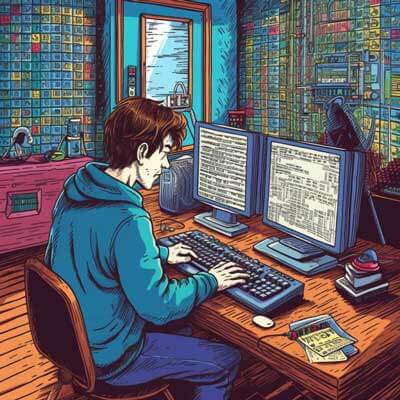
Writing JSON data to a file in Python is a common task in many applications. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy to read and write. It is widely used for storing and transmitting data between a server and a client, or between different parts of an application.
Method 1: Using the json module
The easiest and most straightforward way to write JSON data to a file in Python is by using the built-in json
module. This module provides functions for encoding Python objects as JSON strings and decoding JSON strings back into Python objects.
Here’s an example that demonstrates how to write JSON data to a file using the json
module:
import json data = { "name": "John", "age": 30, "city": "New York" } # Opening a file in write mode with open('data.json', 'w') as file: json.dump(data, file)
In this example, we define a Python dictionary data
that represents the JSON data we want to write to a file. We then open a file named data.json
in write mode using the open()
function. The json.dump()
function is used to write the JSON data to the file.
Related Article: How to Execute a Program or System Command in Python
Method 2: Using the io module
Another way to write JSON data to a file in Python is by using the io
module along with the json
module. This method allows more control over the file operations and can be useful in certain scenarios.
Here’s an example that demonstrates how to use the io
module to write JSON data to a file:
import io import json data = { "name": "John", "age": 30, "city": "New York" } # Opening a file in write mode with io.open('data.json', 'w', encoding='utf-8') as file: file.write(json.dumps(data, ensure_ascii=False))
In this example, we open a file named data.json
in write mode using the io.open()
function. The encoding='utf-8'
parameter ensures that the file is opened with UTF-8 encoding to support non-ASCII characters. We then use the json.dumps()
function to convert the Python dictionary to a JSON string, and the file.write()
method to write the JSON string to the file.
Best Practices
– It is recommended to use the json
module for writing JSON data to a file in Python, as it provides a high-level and easy-to-use interface.
– Always make sure to open the file in the appropriate mode ('w'
for writing) and close it properly after writing the JSON data.
– If you need to write multiple JSON objects to the same file, you can use the json.dump()
or json.dumps()
functions multiple times, each with a different object.
– If you want to pretty-print the JSON data with indentation and line breaks, you can pass the indent
parameter to the json.dump()
or json.dumps()
functions. For example, json.dump(data, file, indent=4)
.
Alternative Ideas
– If you need more advanced control over the JSON serialization process, you can define a custom encoder class by subclassing json.JSONEncoder
. This allows you to handle specific Python objects or customize the output format.
– If you are working with large JSON data sets that cannot fit into memory, you can consider using a streaming approach with libraries like ijson
or jsonlines
.
Related Article: How to Use Python with Multiple Languages (Locale Guide)