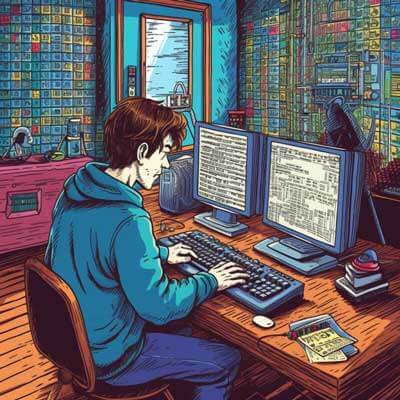
Table of Contents
Integrating Payment Gateways with Django Web Apps
Integrating payment gateways with Django web apps is essential for enabling secure and seamless financial transactions. Payment gateways, such as Stripe, PayPal, and Square, provide APIs that allow developers to connect their web apps to various payment processors. In this section, we will explore how to integrate payment gateways into Django web apps using code snippets and examples.
To get started, let's consider the example of integrating Stripe as a payment gateway in a Django web app. Stripe is a popular payment gateway that offers a comprehensive set of APIs for processing payments securely. To integrate Stripe with Django, you will need to follow these steps:
1. Install the Stripe Python library using pip:
$ pip install stripe
2. Create a Stripe account and obtain your API keys. You will need the secret key and publishable key for authentication and making API calls.
3. Add the Stripe API keys to your Django settings file:
# settings.py STRIPE_SECRET_KEY = 'your_stripe_secret_key' STRIPE_PUBLISHABLE_KEY = 'your_stripe_publishable_key'
4. Create a payment form in your Django app's template:
<!-- payment.html --> {% csrf_token %}
5. Define a view function in your Django app's views.py file to handle the payment processing:
# views.py import stripe def process_payment(request): if request.method == 'POST': card_number = request.POST['card_number'] expiry_date = request.POST['expiry_date'] cvc = request.POST['cvc'] stripe.api_key = settings.STRIPE_SECRET_KEY try: charge = stripe.Charge.create( amount=1000, # amount in cents currency='usd', source={ 'number': card_number, 'exp_month': expiry_date.split('/')[0], 'exp_year': expiry_date.split('/')[1], 'cvc': cvc }, description='Payment for Django Web App' ) # Process successful payment return render(request, 'success.html') except stripe.error.CardError as e: # Handle card error return render(request, 'error.html', {'error': e}) except Exception as e: # Handle other exceptions return render(request, 'error.html', {'error': e})
6. Configure the URL pattern in your Django app's urls.py file:
# urls.py from django.urls import path from .views import process_payment urlpatterns = [ path('payment/', process_payment, name='process_payment'), # other URL patterns ]
7. Finally, create the success.html and error.html templates to display the appropriate messages to users based on the payment status.
This example demonstrates how to integrate Stripe as a payment gateway in a Django web app. Similar steps can be followed to integrate other payment gateways, such as PayPal and Square, by using their respective APIs and SDKs.
Related Article: How to Work with Lists and Arrays in Python
Securing Transactions in Django Web Apps
Securing transactions in Django web apps is crucial to protect sensitive financial information and maintain trust with users. In this section, we will explore best practices and techniques for securing transactions in Django web apps.
1. Use HTTPS: Ensure that your Django web app is served over HTTPS to encrypt the communication between the client and the server. This prevents eavesdropping and protects sensitive data, such as credit card details, during transit.
2. Implement input validation and sanitization: Validate and sanitize all user inputs to prevent common security vulnerabilities, such as SQL injection and cross-site scripting (XSS) attacks. Django provides built-in form validation and sanitization features that can be used to ensure the integrity of user-submitted data.
3. Implement access controls and authentication: Implement proper access controls and authentication mechanisms to ensure that only authorized users can perform transaction-related operations. Django provides a robust authentication system that can be customized to meet the specific requirements of your web app.
4. Store sensitive data securely: Store sensitive data, such as credit card information, securely by following industry-standard encryption and storage practices. Django provides built-in encryption tools, such as the django-encrypted-fields
package, which can be used to encrypt sensitive data at rest.
5. Implement tokenization: Tokenization is the process of replacing sensitive data, such as credit card numbers, with unique tokens that can be used to reference the original data. Implementing tokenization can reduce the risk of data breaches and simplify compliance with Payment Card Industry Data Security Standard (PCI DSS) requirements. Payment gateways like Stripe provide tokenization services that can be integrated into Django web apps.
6. Regularly update dependencies: Keep all dependencies, including Django and payment gateway libraries, up to date to ensure that your web app is protected against known security vulnerabilities. Regularly check for security updates and apply them promptly.
7. Implement logging and monitoring: Implement logging and monitoring mechanisms to track and detect suspicious activities and potential security breaches. Django provides a logging framework that can be configured to log relevant transaction-related events and errors.
Best Practices for PCI Compliance in Django Web Apps
PCI compliance is essential for web apps that handle payment card data. The Payment Card Industry Data Security Standard (PCI DSS) is a set of security standards that must be followed by organizations that process, transmit, or store payment card data. In this section, we will explore best practices for achieving PCI compliance in Django web apps.
1. Understand PCI DSS requirements: Familiarize yourself with the specific PCI DSS requirements that are relevant to your Django web app. The PCI Security Standards Council provides detailed documentation and guidelines that outline the requirements for achieving compliance.
2. Use a PCI-compliant hosting provider: Choose a hosting provider that is PCI compliant and provides a secure infrastructure for hosting your Django web app. The hosting provider should offer features such as firewalls, intrusion detection systems, and regular security audits.
3. Limit cardholder data storage: Minimize the storage of cardholder data in your Django web app by implementing tokenization or outsourcing the storage to a PCI-compliant payment gateway. By reducing the amount of sensitive data stored in your app, you can simplify your compliance efforts and reduce the risk of data breaches.
4. Regularly update and patch your web app: Keep your Django web app and all its dependencies up to date by applying security patches and updates promptly. This includes Django itself, payment gateway libraries, and any other third-party packages used in your app.
5. Implement secure coding practices: Follow secure coding practices when developing your Django web app to minimize the risk of vulnerabilities. This includes input validation, output encoding, and proper error handling. Django provides built-in features and libraries, such as the ORM and form validation, that can help enforce secure coding practices.
6. Implement logging and monitoring: Implement logging and monitoring mechanisms to detect and respond to security incidents promptly. Log relevant transaction-related events and errors using Django's logging framework, and regularly monitor logs for unusual activities.
7. Regularly conduct security assessments and penetration testing: Conduct regular security assessments and penetration testing to identify vulnerabilities and weaknesses in your Django web app. This can help you uncover potential security flaws and address them before they are exploited.
8. Implement strong access controls: Implement strong access controls to limit access to cardholder data. Use role-based access control (RBAC) to ensure that only authorized individuals have access to sensitive data.
Handling Subscription-Based Payments in Django Web Apps
Subscription-based payments are a common business model for web apps that offer services or content on a recurring basis. Django provides robust features and libraries that can be used to handle subscription-based payments efficiently. In this section, we will explore how to handle subscription-based payments in Django web apps using code snippets and examples.
To handle subscription-based payments in Django, you will typically follow these steps:
1. Model your subscription plans: Define models in Django to represent your subscription plans. For example, you could create a Plan
model with fields such as name, price, duration, and features.
from django.db import models class Plan(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=6, decimal_places=2) duration = models.IntegerField(help_text='Duration in months') features = models.TextField(blank=True)
2. Create a model to track user subscriptions: Create a model to track user subscriptions and their associated plans. This model can have fields such as the user, the plan, the start date, and the end date.
from django.contrib.auth.models import User class Subscription(models.Model): user = models.ForeignKey(User, on_delete=models.CASCADE) plan = models.ForeignKey(Plan, on_delete=models.CASCADE) start_date = models.DateField(auto_now_add=True) end_date = models.DateField()
3. Implement a subscription form: Create a form in Django to allow users to select and subscribe to a plan. The form can be rendered in a template and submitted to a view for processing.
from django import forms class SubscriptionForm(forms.Form): plan = forms.ModelChoiceField(queryset=Plan.objects.all())
4. Process the subscription request: In your Django view, handle the subscription request by validating the form, creating a new subscription object, and associating it with the current user.
from django.shortcuts import render, redirect from django.contrib.auth.decorators import login_required @login_required def subscribe(request): if request.method == 'POST': form = SubscriptionForm(request.POST) if form.is_valid(): plan = form.cleaned_data['plan'] subscription = Subscription(user=request.user, plan=plan) subscription.end_date = subscription.start_date + relativedelta(months=plan.duration) subscription.save() return redirect('dashboard') else: form = SubscriptionForm() return render(request, 'subscribe.html', {'form': form})
5. Display subscription information in the user dashboard: In your Django template, display the user's subscription information, such as the plan name, start date, and end date.
<!-- dashboard.html --> <h2>My Subscription</h2> {% if subscription %} <p>Plan: {{ subscription.plan.name }}</p> <p>Start Date: {{ subscription.start_date }}</p> <p>End Date: {{ subscription.end_date }}</p> {% else %} <p>You are not subscribed to any plan.</p> {% endif %}
Related Article: How to Add a Matplotlib Legend in Python
Implementing Recurring Billing in Django Web Apps
Recurring billing is an essential feature for subscription-based web apps that charge users periodically for their subscriptions. In this section, we will explore how to implement recurring billing in Django web apps using code snippets and examples.
To implement recurring billing in Django, you can use a payment gateway like Stripe, which provides API functionality for managing recurring payments. Here's an example of how to implement recurring billing with Stripe in a Django web app:
1. Install the Stripe Python library using pip:
$ pip install stripe
2. Create a subscription plan in your Stripe account. You can do this through the Stripe dashboard or programmatically using the Stripe API.
3. Add the Stripe API keys to your Django settings file:
# settings.py STRIPE_SECRET_KEY = 'your_stripe_secret_key' STRIPE_PUBLISHABLE_KEY = 'your_stripe_publishable_key'
4. Create a view function in your Django app's views.py file to handle the subscription creation and management:
# views.py import stripe def subscribe(request): if request.method == 'POST': stripe.api_key = settings.STRIPE_SECRET_KEY plan_id = request.POST['plan_id'] try: customer = stripe.Customer.create( email=request.user.email, source=request.POST['stripeToken'] ) subscription = stripe.Subscription.create( customer=customer.id, items=[ { 'plan': plan_id } ] ) # Save subscription details in your Django models Subscription.objects.create( user=request.user, stripe_customer_id=customer.id, stripe_subscription_id=subscription.id, plan_id=plan_id ) return render(request, 'success.html') except stripe.error.CardError as e: # Handle card error return render(request, 'error.html', {'error': e}) except Exception as e: # Handle other exceptions return render(request, 'error.html', {'error': e}) plan_id = 'your_stripe_plan_id' return render(request, 'subscribe.html', {'plan_id': plan_id})
5. Create a template for the subscription form where users can select a plan and enter their payment details:
<!-- subscribe.html --> {% csrf_token %}
6. Configure the URL pattern in your Django app's urls.py file:
# urls.py from django.urls import path from .views import subscribe urlpatterns = [ path('subscribe/', subscribe, name='subscribe'), # other URL patterns ]
In this example, when a user submits the subscription form, Stripe's Checkout.js library is used to collect the payment details securely. Stripe's API is then used to create a customer and a subscription, and the subscription details are saved in your Django models.
Processing Refunds in Django Web Apps
Processing refunds is an important aspect of payment processing in web apps. In some cases, users may request refunds for various reasons, such as dissatisfaction with a product or accidental overpayment. In this section, we will explore how to process refunds in Django web apps using code snippets and examples.
To process refunds in Django, you will typically follow these steps:
1. Retrieve the payment details: Retrieve the payment details associated with the refund request. This could be the transaction ID or any other unique identifier that allows you to identify the payment.
2. Validate the refund request: Validate the refund request to ensure that it meets your refund policy criteria. For example, you may require the refund request to be made within a specific timeframe or for a valid reason.
3. Calculate the refund amount: Calculate the refund amount based on your refund policy. This could involve prorating the subscription amount if the refund is for a subscription-based payment.
4. Initiate the refund with the payment gateway: Use the payment gateway's API to initiate the refund and process the transaction. Most payment gateways provide APIs for refunding transactions.
Here's an example of how to process refunds in a Django web app using the Stripe payment gateway:
1. Add a refund request form in your Django template:
<!-- refund.html --> {% csrf_token %}
2. Define a view function in your Django app's views.py file to handle the refund request:
# views.py import stripe def refund(request): if request.method == 'POST': stripe.api_key = settings.STRIPE_SECRET_KEY payment_id = request.POST['payment_id'] try: refund = stripe.Refund.create( charge=payment_id ) # Process successful refund return render(request, 'refund_success.html') except stripe.error.StripeError as e: # Handle refund error return render(request, 'refund_error.html', {'error': e}) except Exception as e: # Handle other exceptions return render(request, 'refund_error.html', {'error': e}) return render(request, 'refund.html')
In this example, the refund request form collects the payment ID from the user. The payment ID is then used to initiate the refund using the Stripe API. If the refund is successful, the user is shown a success message. Otherwise, an error message is displayed.
Handling Disputes in Django Web Apps
Handling disputes is a critical aspect of payment processing in web apps. Disputes may arise when a customer disputes a charge, initiates a chargeback, or raises a complaint regarding a payment. In this section, we will explore how to handle disputes in Django web apps using code snippets and examples.
To handle disputes in Django, you will typically follow these steps:
1. Receive the dispute notification: Payment gateways, such as Stripe, provide webhooks or event notifications for dispute-related events. Configure your Django app to listen for these notifications and receive the dispute details.
2. Retrieve the payment details: Retrieve the payment details associated with the dispute. This could be the transaction ID or any other unique identifier that allows you to identify the payment.
3. Investigate the dispute: Review the dispute details and investigate the issue raised by the customer. This may involve communicating with the customer, gathering evidence, and verifying the validity of the dispute.
4. Respond to the dispute: Prepare a response to the dispute based on your investigation. This response should include supporting evidence, such as transaction records, communication history, and any other relevant information.
5. Submit the dispute response: Use the payment gateway's API to submit your response to the dispute. Most payment gateways provide APIs for responding to disputes.
Here's an example of how to handle disputes in a Django web app using the Stripe payment gateway:
1. Configure your Django app to receive dispute notifications using webhooks. You can do this by adding a webhook endpoint in your Django app's views.py file:
# views.py import stripe @csrf_exempt def handle_webhook(request): payload = request.body sig_header = request.META['HTTP_STRIPE_SIGNATURE'] endpoint_secret = 'your_webhook_endpoint_secret' try: event = stripe.Webhook.construct_event( payload, sig_header, endpoint_secret ) except ValueError as e: # Invalid payload return HttpResponse(status=400) except stripe.error.SignatureVerificationError as e: # Invalid signature return HttpResponse(status=400) # Handle dispute event if event['type'] == 'charge.dispute.created': dispute = event['data']['object'] # Retrieve payment details based on dispute ID payment_id = dispute['charge'] payment = Payment.objects.get(id=payment_id) # Investigate the dispute and prepare a response response = 'Your response to the dispute' # Submit the response to the payment gateway stripe.Dispute.submit_evidence( dispute['id'], response=response ) return HttpResponse(status=200)
2. Configure the webhook endpoint in your Django app's urls.py file:
# urls.py from django.urls import path from .views import handle_webhook urlpatterns = [ path('webhook/', handle_webhook, name='webhook'), # other URL patterns ]
In this example, the handle_webhook
view function receives the dispute notification from Stripe and extracts the dispute details. It then retrieves the payment details associated with the dispute, investigates the dispute, prepares a response, and submits the response to the payment gateway using the Stripe API.
Fraud Prevention Strategies for Django Web Apps
Fraud prevention is crucial for ensuring the security and integrity of payment processing in web apps. Fraudulent activities, such as unauthorized transactions and stolen credit card information, can have a significant impact on businesses. In this section, we will explore fraud prevention strategies for Django web apps using code snippets and examples.
To prevent fraud in Django, you can implement various strategies and techniques. Here are some best practices to consider:
1. Implement strong authentication and access controls: Use strong authentication mechanisms, such as multi-factor authentication (MFA), to ensure that only authorized individuals have access to sensitive data and payment processing features. Django provides a robust authentication system that can be customized to enforce strong access controls.
2. Monitor user behavior and transactions: Implement behavior-based monitoring to detect suspicious activities and patterns. Monitor user behavior, such as login attempts, transaction history, and IP addresses, for anomalies and potential signs of fraud. Django's logging and monitoring features can be utilized to track and analyze user behavior.
3. Use fraud detection tools and services: Integrate fraud detection tools and services, such as MaxMind and Sift Science, into your Django web app. These tools use machine learning algorithms and behavioral analysis to identify potentially fraudulent transactions and activities.
4. Implement address verification system (AVS): Use AVS to verify the billing address provided by the customer during the payment process. AVS can help detect fraudulent transactions by comparing the address provided with the address on file with the card-issuing bank.
5. Implement card verification value (CVV) checks: Require customers to provide the CVV code on their credit cards during the payment process. CVV checks can help verify that the customer possesses the physical card and reduce the risk of fraudulent transactions.
6. Implement velocity checks: Monitor the frequency and volume of transactions to detect unusual patterns. Implement velocity checks to identify and flag suspicious activities, such as a high number of transactions within a short period or multiple transactions from the same IP address.
7. Stay updated with fraud trends and industry news: Stay informed about the latest fraud trends, techniques, and industry news. Regularly review resources provided by organizations like the Payment Card Industry Security Standards Council (PCI SSC) and stay updated on best practices for fraud prevention.
Related Article: How To Move A File In Python
Implementing Mobile Payments and Digital Wallets in Django Web Apps
Implementing mobile payments and digital wallets in Django web apps allows users to make payments using their mobile devices or digital wallet applications. Mobile payments and digital wallets provide convenience and security for users, making them popular choices for online transactions. In this section, we will explore how to implement mobile payments and digital wallets in Django web apps using code snippets and examples.
To implement mobile payments and digital wallets in Django, you can leverage the APIs and SDKs provided by mobile payment platforms and digital wallet providers. Here's an example of how to implement mobile payments and digital wallets using the Stripe API:
1. Install the Stripe Python library using pip:
$ pip install stripe
2. Create a payment form in your Django app's template:
<!-- payment.html --> {% csrf_token %}
3. Define a view function in your Django app's views.py file to handle the payment processing:
# views.py import stripe def process_payment(request): if request.method == 'POST': amount = request.POST['amount'] source = request.POST['source'] stripe.api_key = settings.STRIPE_SECRET_KEY try: charge = stripe.Charge.create( amount=amount, # amount in cents currency='usd', source=source, description='Payment for Django Web App' ) # Process successful payment return render(request, 'success.html') except stripe.error.CardError as e: # Handle card error return render(request, 'error.html', {'error': e}) except Exception as e: # Handle other exceptions return render(request, 'error.html', {'error': e})
4. Configure the URL pattern in your Django app's urls.py file:
# urls.py from django.urls import path from .views import process_payment urlpatterns = [ path('payment/', process_payment, name='process_payment'), # other URL patterns ]
In this example, the payment form collects the amount and payment source from the user. The payment source can be a mobile payment method or a digital wallet token. The view function uses the Stripe API to create a charge with the provided amount and payment source.
Additional Resources
- Securing Transactions in Django