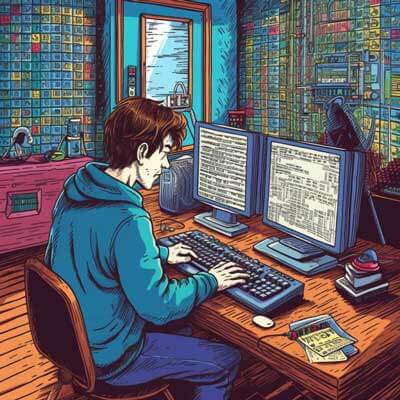
Django Chat Applications
Django is a useful web framework that allows developers to build robust and scalable web applications. One popular feature that can enhance a Django app is the integration of chat functionality. Chat applications can provide real-time communication between users, allowing for collaboration, support, and social interaction within the app.
To implement chat functionality in a Django app, there are several approaches that can be taken. One common method is to use WebSocket technology, which enables bidirectional communication between the client and the server. Django Channels is a great library that brings WebSocket support to Django, making it easy to build chat applications.
Below is an example of how to implement a simple chat application using Django Channels.
First, install Django Channels:
pip install channels
Next, create a new Django app for the chat functionality:
python manage.py startapp chat
In the settings.py
file, add the channels
app to the INSTALLED_APPS
list:
INSTALLED_APPS = [ ... 'channels', ... ]
Create a routing.py
file in the chat app directory and define the WebSocket routing:
from django.urls import re_path from . import consumers websocket_urlpatterns = [ re_path(r'ws/chat/(?P\w+)/$', consumers.ChatConsumer.as_asgi()), ]
Create a consumers.py
file in the chat app directory and define the chat consumer:
from channels.generic.websocket import AsyncWebsocketConsumer class ChatConsumer(AsyncWebsocketConsumer): async def connect(self): self.room_name = self.scope['url_route']['kwargs']['room_name'] self.room_group_name = 'chat_%s' % self.room_name await self.channel_layer.group_add( self.room_group_name, self.channel_name ) await self.accept() async def disconnect(self, close_code): await self.channel_layer.group_discard( self.room_group_name, self.channel_name ) async def receive(self, text_data): await self.channel_layer.group_send( self.room_group_name, { 'type': 'chat_message', 'message': text_data } ) async def chat_message(self, event): message = event['message'] await self.send(text_data=message)
Finally, update the project’s asgi.py
file to include the Django Channels routing:
from channels.routing import ProtocolTypeRouter, URLRouter from django.core.asgi import get_asgi_application import chat.routing application = ProtocolTypeRouter({ "http": get_asgi_application(), "websocket": URLRouter( chat.routing.websocket_urlpatterns ), })
With these steps completed, you can now use JavaScript on the client-side to establish a WebSocket connection and send/receive chat messages.
This is just a basic example, and there are many additional features and considerations that can be implemented in a Django chat application, such as user authentication, message persistence, and more. However, this example demonstrates the fundamental setup needed to get started with chat functionality in Django using Django Channels.
Related Article: 16 Amazing Python Libraries You Can Use Now
WebRTC Integration
WebRTC (Web Real-Time Communication) is a useful technology that enables real-time voice and video communication directly in web browsers. Integrating WebRTC into a Django app can open up a wide range of possibilities for building applications that require real-time communication features.
To integrate WebRTC into a Django app, there are a few steps that need to be followed. First, you need to set up a signaling server to facilitate the communication between peers. This server can be built using Django Channels or a separate server built with Node.js and a library like Socket.IO.
Below is an example of how to set up a basic WebRTC integration using Django Channels.
First, install the necessary dependencies:
pip install channels django-redis channels-redis
Next, create a new Django app for the WebRTC functionality:
python manage.py startapp webrtc
In the settings.py
file, add the channels
and webrtc
apps to the INSTALLED_APPS
list:
INSTALLED_APPS = [ ... 'channels', 'webrtc', ... ]
Create a routing.py
file in the webrtc app directory and define the WebSocket routing:
from django.urls import re_path from . import consumers websocket_urlpatterns = [ re_path(r'ws/webrtc/(?P\w+)/$', consumers.WebRTCConsumer.as_asgi()), ]
Create a consumers.py
file in the webrtc app directory and define the WebRTC consumer:
from channels.generic.websocket import AsyncWebsocketConsumer class WebRTCConsumer(AsyncWebsocketConsumer): async def connect(self): self.room_name = self.scope['url_route']['kwargs']['room_name'] self.room_group_name = 'webrtc_%s' % self.room_name await self.channel_layer.group_add( self.room_group_name, self.channel_name ) await self.accept() async def disconnect(self, close_code): await self.channel_layer.group_discard( self.room_group_name, self.channel_name ) async def receive(self, text_data): await self.channel_layer.group_send( self.room_group_name, { 'type': 'webrtc_message', 'message': text_data } ) async def webrtc_message(self, event): message = event['message'] await self.send(text_data=message)
Finally, update the project’s asgi.py
file to include the Django Channels routing:
from channels.routing import ProtocolTypeRouter, URLRouter from django.core.asgi import get_asgi_application import webrtc.routing application = ProtocolTypeRouter({ "http": get_asgi_application(), "websocket": URLRouter( webrtc.routing.websocket_urlpatterns ), })
With these steps completed, you can now use JavaScript on the client-side to establish a WebSocket connection, set up the WebRTC connection, and exchange voice/video streams between peers.
However, this is just a basic example, and there are many additional features and considerations that can be implemented when integrating WebRTC into a Django app, such as user authentication, signaling server security, and more. Nonetheless, this example provides the foundation needed to get started with WebRTC integration in Django using Django Channels.
SMS Gateways in Django
Integrating SMS gateways into a Django app can enable the sending of text notifications and OTPs (One-Time Passwords) to users’ mobile phones. This can be useful for various scenarios, such as account verification, password reset, and transactional notifications.
One popular SMS gateway provider is Twilio. Twilio provides a simple and reliable API for sending SMS messages, and it can be easily integrated into a Django app.
To integrate Twilio into a Django app, follow these steps:
1. Sign up for a Twilio account and obtain your API credentials, including the Account SID and Auth Token.
2. Install the Twilio Python library:
pip install twilio
3. In your Django project’s settings.py file, add the following configuration variables:
TWILIO_ACCOUNT_SID = 'your_account_sid' TWILIO_AUTH_TOKEN = 'your_auth_token' TWILIO_PHONE_NUMBER = '+1234567890' # Your Twilio phone number
4. Create a helper function in your Django app to send SMS messages using Twilio:
from twilio.rest import Client from django.conf import settings def send_sms(phone_number, message): client = Client(settings.TWILIO_ACCOUNT_SID, settings.TWILIO_AUTH_TOKEN) client.messages.create( body=message, from_=settings.TWILIO_PHONE_NUMBER, to=phone_number )
5. Now you can use the send_sms
function to send SMS messages from your Django app. For example, you can send a verification code to a user’s phone number:
from django.shortcuts import render from .utils import send_sms def send_verification_code(request): # Get user's phone number from the request phone_number = request.POST.get('phone_number') # Generate a random verification code verification_code = generate_verification_code() # Save the verification code in the database or session for later verification # Send the verification code via SMS message = f"Your verification code is: {verification_code}" send_sms(phone_number, message) return render(request, 'verification_code_sent.html')
Additional Resources
– Using Django Channels for WebSocket communication in Django
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps