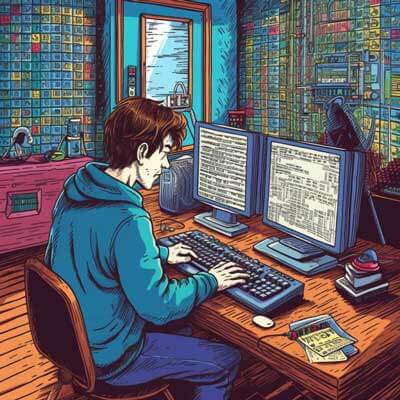
- Model Inheritance in Django ORM
- Abstract Base Classes
- Multi-table Inheritance
- Proxy Models
- Database Transactions in Django ORM
- Query Optimization in Django ORM
- Select_related
- Only
- Prefetch_related
- Complex Queries in Django ORM
- Filtering
- Sorting
- Aggregating
- Query Expressions in Django ORM
- Database Functions
- Arithmetic Operations
- Logical Operations
- Django Q Objects for Advanced Querying
- Efficient Querying Techniques in Django ORM
- Use select_related and prefetch_related
- Use only to retrieve specific fields
- Use values or values_list for lightweight queries
- Working with Django Model Managers
- Defining Custom Managers
- Chaining Custom Manager Methods
- Default Manager
- Additional Resources
Model Inheritance in Django ORM
Model inheritance is a useful feature in Django ORM that allows you to create a hierarchy of models, where child models inherit fields and behaviors from their parent models. This can be useful when you have multiple models that share common fields and functionality.
In Django ORM, there are three types of model inheritance: abstract base classes, multi-table inheritance, and proxy models.
Related Article: Python Programming for Kids
Abstract Base Classes
Abstract base classes are used when you want to define a common set of fields and methods that will be inherited by multiple child models. However, abstract base classes cannot be instantiated on their own.
Let’s look at an example:
from django.db import models class BaseModel(models.Model): created_at = models.DateTimeField(auto_now_add=True) updated_at = models.DateTimeField(auto_now=True) class Meta: abstract = True class Product(BaseModel): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) class Order(BaseModel): products = models.ManyToManyField(Product) total_amount = models.DecimalField(max_digits=10, decimal_places=2)
In this example, the BaseModel
class is an abstract base class that defines the created_at
and updated_at
fields. The Product
and Order
models then inherit these fields along with their own specific fields.
Multi-table Inheritance
Multi-table inheritance allows you to create a separate table for each model in the inheritance hierarchy. Each table contains its own fields and a foreign key to the parent model.
Let’s consider the following example:
from django.db import models class BaseProduct(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) class DiscountedProduct(BaseProduct): discount_percentage = models.DecimalField(max_digits=5, decimal_places=2)
In this example, the BaseProduct
model defines the common fields name
and price
. The DiscountedProduct
model then inherits these fields along with its own specific field discount_percentage
. Django ORM automatically creates a foreign key from the DiscountedProduct
table to the BaseProduct
table.
Proxy Models
Proxy models allow you to create a new model that has the same fields and methods as an existing model, but with a different manager or additional methods.
Let’s see an example:
from django.db import models class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) class DiscountedProduct(Product): class Meta: proxy = True def get_discounted_price(self): return self.price * 0.9
In this example, the DiscountedProduct
model is a proxy model that inherits from the Product
model. It has the same fields and methods as the Product
model, but also defines an additional method get_discounted_price()
.
Model inheritance in Django ORM provides a flexible way to reuse code and create hierarchies of models. Whether you need to define a common set of fields and methods, create separate tables for each model, or add additional methods to an existing model, Django ORM has you covered.
Related Article: Working with Numpy Concatenate
Database Transactions in Django ORM
Database transactions in Django ORM provide a way to group multiple database operations into a single unit of work. Transactions ensure that all operations within the transaction are applied atomically, meaning that either all operations succeed or none of them do.
In Django ORM, you can use the transaction.atomic()
decorator or context manager to define a block of code that should be executed within a transaction.
Let’s look at an example using the transaction.atomic()
decorator:
from django.db import transaction @transaction.atomic def create_order(customer_id, product_ids): try: # Create a new order order = Order.objects.create(customer_id=customer_id) # Add products to the order for product_id in product_ids: product = Product.objects.get(id=product_id) order.products.add(product) # Calculate the total amount total_amount = sum(product.price for product in order.products.all()) order.total_amount = total_amount order.save() # Perform some other operations ... # Commit the transaction transaction.commit() except Exception as e: # Rollback the transaction in case of an exception transaction.rollback() raise e
In this example, the create_order()
function is wrapped with the transaction.atomic()
decorator. This ensures that all database operations within the function will be applied atomically. If an exception occurs, the transaction will be rolled back.
Alternatively, you can use the transaction.atomic()
context manager to define a block of code that should be executed within a transaction:
from django.db import transaction def create_order(customer_id, product_ids): try: with transaction.atomic(): # Create a new order order = Order.objects.create(customer_id=customer_id) # Add products to the order for product_id in product_ids: product = Product.objects.get(id=product_id) order.products.add(product) # Calculate the total amount total_amount = sum(product.price for product in order.products.all()) order.total_amount = total_amount order.save() # Perform some other operations ... except Exception as e: # Rollback the transaction in case of an exception transaction.set_rollback(True) raise e
In this example, the with transaction.atomic():
statement defines the block of code that should be executed within a transaction. If an exception occurs, the transaction will be rolled back.
Database transactions in Django ORM are essential for maintaining data integrity and ensuring that complex operations are applied atomically. By using the transaction.atomic()
decorator or context manager, you can easily manage transactions in your Django applications.
Query Optimization in Django ORM
Query optimization is an important aspect of database performance tuning. In Django ORM, there are several techniques you can use to optimize your queries and improve the overall performance of your application.
Select_related
The select_related
method is a useful optimization technique in Django ORM that allows you to retrieve related objects in a single database query, instead of making multiple queries. This can significantly reduce the number of database round-trips and improve the performance of your application.
Let’s consider an example:
from django.db import models class Author(models.Model): name = models.CharField(max_length=100) class Book(models.Model): title = models.CharField(max_length=100) author = models.ForeignKey(Author, on_delete=models.CASCADE)
In this example, the Book
model has a foreign key to the Author
model. If you want to retrieve all books along with their authors, you can use the select_related
method as follows:
books = Book.objects.select_related('author')
This will fetch all books and their authors in a single query, instead of making a separate query for each book’s author.
Related Article: Diphthong Detection Methods in Python
Only
The only
method is another optimization technique in Django ORM that allows you to specify the fields you want to retrieve from the database. By only retrieving the necessary fields, you can reduce the amount of data transferred between the database and your application, resulting in improved query performance.
Let’s continue with the previous example:
books = Book.objects.only('title')
In this example, only the title
field of the Book
model will be retrieved from the database.
Prefetch_related
The prefetch_related
method is similar to the select_related
method, but it is used for ManyToMany and reverse ForeignKey relationships. It allows you to prefetch related objects in a single database query, instead of making multiple queries.
Let’s consider an example:
from django.db import models class Tag(models.Model): name = models.CharField(max_length=100) class Article(models.Model): title = models.CharField(max_length=100) tags = models.ManyToManyField(Tag)
In this example, the Article
model has a ManyToMany relationship with the Tag
model. If you want to retrieve all articles along with their tags, you can use the prefetch_related
method as follows:
articles = Article.objects.prefetch_related('tags')
This will fetch all articles and their tags in a single query, instead of making a separate query for each article’s tags.
Query optimization techniques like select_related
, only
, and prefetch_related
can greatly improve the performance of your Django ORM queries by reducing the number of database round-trips and optimizing the amount of data transferred between the database and your application. It’s important to analyze your application’s query patterns and apply the appropriate optimization techniques to ensure optimal performance.
Complex Queries in Django ORM
Django ORM provides a rich set of query capabilities that allow you to perform complex queries on your database. Whether you need to filter, sort, or aggregate data, Django ORM has you covered.
Related Article: How to Create a Null Matrix in Python
Filtering
Filtering is one of the most common operations when working with databases. Django ORM provides a useful filtering mechanism that allows you to retrieve records based on specific criteria.
Let’s consider an example:
from django.db import models class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) category = models.CharField(max_length=100) # Retrieve all products with a price greater than 100 products = Product.objects.filter(price__gt=100) # Retrieve all products in the 'Electronics' category products = Product.objects.filter(category='Electronics') # Retrieve all products with a price between 50 and 100 products = Product.objects.filter(price__range=(50, 100))
In this example, the filter()
method is used to retrieve products that match specific criteria. The price__gt
lookup filters products with a price greater than 100, the category
lookup filters products in the ‘Electronics’ category, and the price__range
lookup filters products with a price between 50 and 100.
Sorting
Sorting allows you to order query results based on specific fields. Django ORM provides the order_by()
method to specify the order in which records should be retrieved.
Continuing with the previous example:
# Retrieve all products and order them by price in ascending order products = Product.objects.order_by('price') # Retrieve all products and order them by price in descending order products = Product.objects.order_by('-price')
In this example, the order_by()
method is used to order products by price. By default, products are ordered in ascending order. To order products in descending order, you can prefix the field name with a hyphen.
Aggregating
Aggregating allows you to perform calculations on query results, such as calculating the sum, average, count, or maximum value of a field. Django ORM provides the aggregate()
method to perform aggregations.
Continuing with the previous example:
from django.db.models import Sum, Avg, Count, Max # Calculate the total price of all products total_price = Product.objects.aggregate(Sum('price')).get('price__sum') # Calculate the average price of all products average_price = Product.objects.aggregate(Avg('price')).get('price__avg') # Count the number of products product_count = Product.objects.aggregate(Count('id')).get('id__count') # Retrieve the most expensive product most_expensive_product = Product.objects.aggregate(Max('price')).get('price__max')
In this example, the aggregate()
method is used to calculate the total price, average price, count, and the maximum price of products.
Django ORM provides a wide range of query capabilities that allow you to perform complex operations on your database. Whether you need to filter, sort, or aggregate data, Django ORM has you covered with its intuitive and useful query API.
Related Article: How to Integrate Python with MySQL for Database Queries
Query Expressions in Django ORM
Query expressions in Django ORM allow you to perform complex database operations using Python expressions. They provide a way to include database functions, arithmetic operations, and logical operations directly in your queries.
Let’s look at some examples of query expressions in Django ORM:
Database Functions
Django ORM provides a set of built-in database functions that can be used in queries. These functions allow you to perform various calculations and transformations on fields.
from django.db.models import F, Sum # Increase the price of all products by 10% Product.objects.update(price=F('price') * 1.1) # Calculate the total price of all products using the Sum function total_price = Product.objects.aggregate(total_price=Sum('price')).get('total_price')
In this example, the F()
expression is used to reference the value of a field in a query. The update()
method increases the price of all products by 10%, and the aggregate()
method calculates the total price of all products using the Sum()
function.
Arithmetic Operations
Django ORM allows you to perform arithmetic operations directly in your queries.
from django.db.models import F # Retrieve all products with a price greater than twice the average price average_price = Product.objects.aggregate(average_price=Avg('price')).get('average_price') products = Product.objects.filter(price__gt=F('price') * 2)
In this example, the F()
expression is used in the filter condition to compare the price of each product with twice the average price.
Related Article: Python Data Types & Data Modeling
Logical Operations
Django ORM allows you to perform logical operations in your queries using Q objects.
from django.db.models import Q # Retrieve all products with a price greater than 100 or a category of 'Electronics' products = Product.objects.filter(Q(price__gt=100) | Q(category='Electronics'))
In this example, the Q()
object is used to build a logical OR condition in the filter. This retrieves all products with a price greater than 100 or a category of ‘Electronics’.
Query expressions in Django ORM provide a useful way to perform complex database operations using Python expressions. Whether you need to include database functions, perform arithmetic operations, or use logical operators in your queries, Django ORM has you covered.
Django Q Objects for Advanced Querying
Django Q objects provide a way to build complex queries with logical operators such as AND, OR, and NOT. They allow you to combine multiple conditions in a single query, making it easier to express complex query requirements.
Let’s consider an example:
from django.db.models import Q # Retrieve all products with a price greater than 100 and a category of 'Electronics' products = Product.objects.filter(Q(price__gt=100) & Q(category='Electronics')) # Retrieve all products with a price greater than 100 or a category of 'Electronics' products = Product.objects.filter(Q(price__gt=100) | Q(category='Electronics')) # Retrieve all products with a price greater than 100 and NOT in the category 'Books' products = Product.objects.filter(Q(price__gt=100) & ~Q(category='Books'))
In this example, the Q()
object is used to build complex queries with logical operators. The &
operator corresponds to the logical AND operation, the |
operator corresponds to the logical OR operation, and the ~
operator corresponds to the logical NOT operation.
Django Q objects can be used to express complex query requirements in a concise and readable way. Whether you need to combine multiple conditions with logical operators or negate a condition, Django Q objects provide a useful tool for advanced querying in Django ORM.
Efficient Querying Techniques in Django ORM
Efficient querying techniques can greatly improve the performance of your Django ORM queries. By optimizing your queries, you can reduce the number of database round-trips, optimize the amount of data transferred between the database and your application, and improve the overall responsiveness of your application.
Related Article: Structuring Data for Time Series Analysis with Python
Use select_related and prefetch_related
The select_related
and prefetch_related
methods are useful optimization techniques in Django ORM that allow you to retrieve related objects in a single database query, instead of making multiple queries. By using these methods, you can reduce the number of database round-trips and improve query performance.
Let’s consider an example:
from django.db import models class Author(models.Model): name = models.CharField(max_length=100) class Book(models.Model): title = models.CharField(max_length=100) author = models.ForeignKey(Author, on_delete=models.CASCADE)
In this example, the Book
model has a foreign key to the Author
model. If you want to retrieve all books along with their authors, you can use the select_related
method as follows:
books = Book.objects.select_related('author')
This will fetch all books and their authors in a single query, instead of making a separate query for each book’s author.
Similarly, if you have a ManyToMany or reverse ForeignKey relationship, you can use the prefetch_related
method to prefetch related objects in a single query:
from django.db import models class Tag(models.Model): name = models.CharField(max_length=100) class Article(models.Model): title = models.CharField(max_length=100) tags = models.ManyToManyField(Tag)
In this example, the Article
model has a ManyToMany relationship with the Tag
model. If you want to retrieve all articles along with their tags, you can use the prefetch_related
method as follows:
articles = Article.objects.prefetch_related('tags')
This will fetch all articles and their tags in a single query, instead of making a separate query for each article’s tags.
Use only to retrieve specific fields
The only
method allows you to specify the fields you want to retrieve from the database. By only retrieving the necessary fields, you can reduce the amount of data transferred between the database and your application, resulting in improved query performance.
Continuing from the previous example:
books = Book.objects.only('title')
In this example, only the title
field of the Book
model will be retrieved from the database.
Using the only
method, you can fine-tune your queries and optimize the amount of data transferred between the database and your application, leading to improved query performance.
Use values or values_list for lightweight queries
The values
and values_list
methods allow you to retrieve a subset of fields from the database and return them as dictionaries or tuples, respectively. These methods can be used to perform lightweight queries that only retrieve the necessary data, resulting in improved query performance.
Let’s consider an example:
books = Book.objects.values('title', 'author__name')
In this example, the values
method is used to retrieve the title
and author__name
fields of the Book
model as dictionaries.
Similarly, you can use the values_list
method to retrieve the fields as tuples:
books = Book.objects.values_list('title', 'author__name')
Efficient querying techniques such as using select_related
, prefetch_related
, only
, and values
or values_list
can greatly improve the performance of your Django ORM queries. By reducing the number of database round-trips, optimizing the amount of data transferred between the database and your application, and retrieving only the necessary fields, you can ensure optimal query performance in your Django applications.
Related Article: Implementation of Data Structures in Python
Working with Django Model Managers
Django model managers provide a way to encapsulate common query operations and custom query logic in a reusable and centralized place. They allow you to define custom methods that can be used to perform complex queries or retrieve specific subsets of data from your models.
Defining Custom Managers
To define a custom manager for a model, you need to subclass the models.Manager
class and add the desired methods to perform custom queries.
Let’s consider an example:
from django.db import models class ProductManager(models.Manager): def get_expensive_products(self): return self.filter(price__gt=100) class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) objects = ProductManager()
In this example, a custom manager ProductManager
is defined with a method get_expensive_products()
that retrieves products with a price greater than 100. The Product
model then specifies the custom manager by assigning an instance of ProductManager
to the objects
attribute.
With this setup, you can now use the custom manager methods to perform complex queries:
expensive_products = Product.objects.get_expensive_products()
Chaining Custom Manager Methods
One of the benefits of using custom managers is the ability to chain multiple methods to perform complex queries.
Continuing from the previous example:
from django.db import models class ProductManager(models.Manager): def get_expensive_products(self): return self.filter(price__gt=100) def get_filtered_products(self, category): return self.filter(category=category) class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) category = models.CharField(max_length=100) objects = ProductManager()
In this updated example, a new method get_filtered_products()
is added to the ProductManager
class. This method retrieves products with a specific category. By chaining these two methods, you can retrieve expensive products with a specific category:
expensive_electronics = Product.objects.get_expensive_products().get_filtered_products('Electronics')
This chaining of custom manager methods allows you to build complex queries in a readable and expressive way.
Related Article: How to Access Python Data Structures with Square Brackets
Default Manager
In Django, every model has a default manager that is used for queries on the model. By default, the objects
attribute is the default manager. However, you can specify a different manager to be the default manager by setting the default_manager_name
attribute in the model’s Meta
class.
Let’s consider an example:
from django.db import models class ProductManager(models.Manager): def get_expensive_products(self): return self.filter(price__gt=100) class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) objects = ProductManager() class Meta: default_manager_name = 'objects'
In this example, the Product
model specifies the custom manager ProductManager
as the default manager by setting default_manager_name
to 'objects'
.
Additional Resources
– Django ORM: A Primer
– Django ORM: Aggregation
– Django ORM: Annotating QuerySets