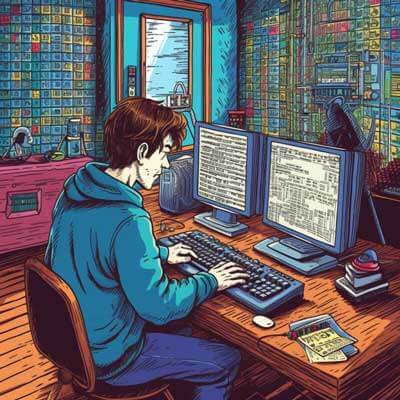
Table of Contents
Getting Started with Linspace: Syntax, Parameters and Purpose
The linspace
function in Python is a powerful tool for generating equidistant numeric sequences. It is part of the numpy
library, which is widely used for scientific computing and data analysis in Python. In this chapter, we will explore the syntax, parameters, and purpose of the linspace
function.
Related Article: Python File Operations: How to Read, Write, Delete, Copy
Syntax
The syntax for using the linspace
function is as follows:
numpy.linspace(start, stop, num=50, endpoint=True, retstep=False, dtype=None, axis=0)
Let's take a closer look at each parameter:
- start
: The starting value of the sequence.
- stop
: The end value of the sequence.
- num
: The number of evenly spaced values in the sequence. The default value is 50.
- endpoint
: A boolean value that indicates whether the stop
value should be included in the sequence. If set to True
(default), the stop
value is included. If set to False
, the stop
value is excluded.
- retstep
: A boolean value that indicates whether to return the step value between adjacent elements in the sequence. If set to True
, the linspace
function will return a tuple containing the sequence and the step value. If set to False
(default), only the sequence will be returned.
- dtype
: The data type of the output sequence. If not specified, the data type is determined automatically.
- axis
: The axis along which the sequence is generated. This parameter is only used when working with multi-dimensional arrays.
Parameters
The start
and stop
parameters are required when using the linspace
function. They define the range of values for the sequence. The num
parameter specifies the number of values in the sequence. If not provided, the default value of 50 is used.
The endpoint
parameter determines whether the stop
value should be included in the sequence. By default, it is set to True
. If you want to exclude the stop
value, you can set it to False
.
The retstep
parameter is useful when you need to know the step value between adjacent elements in the sequence. By default, it is set to False
, meaning only the sequence is returned. If you set it to True
, the linspace
function will return a tuple containing the sequence and the step value.
The dtype
parameter allows you to specify the data type of the output sequence. If not specified, the data type is determined automatically based on the input values.
The axis
parameter is only used when working with multi-dimensional arrays. It specifies the axis along which the sequence is generated.
Purpose
The main purpose of the linspace
function is to generate evenly spaced numeric sequences. It is particularly useful in scenarios where you need to create a sequence of values that span a specific range with a fixed number of elements.
For example, let's say you want to generate a sequence of 10 values between 0 and 1. You can achieve this using the linspace
function as follows:
import numpy as np sequence = np.linspace(0, 1, num=10) print(sequence)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ]
In this example, the linspace
function generates a sequence of 10 values between 0 and 1. Each value is evenly spaced in the range, including the endpoint.
The linspace
function is widely used in various scientific and numerical applications, such as signal processing, data visualization, and interpolation. It provides a convenient and efficient way to generate equidistant numeric sequences in Python.
Now that you have a good understanding of the syntax, parameters, and purpose of the linspace
function, you can start leveraging this handy tool in your Python projects.
Related Article: How to Use the And/Or Operator in Python Regular Expressions
Generating Equidistant Numeric Sequences: Basics and Principles
In scientific computing and data analysis, it is often necessary to generate numeric sequences with equal spacing between the elements. These equidistant sequences are used in various applications, such as plotting graphs, interpolating data, and solving differential equations. Python provides a useful tool called linspace
in the NumPy library for generating such sequences efficiently.
The linspace
function in Python creates a numeric sequence by specifying the start and end values, along with the desired number of elements in the sequence. It divides the interval between the start and end values into equal parts and returns an array with the specified number of elements.
Here is the basic syntax of the linspace
function:
numpy.linspace(start, stop, num=50, endpoint=True, retstep=False, dtype=None)
Let's take a closer look at the parameters:
- start
: The starting value of the sequence.
- stop
: The end value of the sequence.
- num
: The number of elements in the sequence. By default, it is set to 50.
- endpoint
: A boolean value indicating whether to include the stop
value in the sequence. If set to True
, the sequence will include the stop
value; if set to False
, the sequence will not include the stop
value. By default, it is set to True
.
- retstep
: A boolean value indicating whether to return the spacing between consecutive elements in the sequence. If set to True
, the function will return a tuple containing the sequence and the spacing. By default, it is set to False
.
- dtype
: The data type of the elements in the sequence. If not specified, it is determined based on the start and stop values.
Let's see some examples of using the linspace
function:
Example 1: Generate a sequence of 10 equally spaced values between 0 and 1 (inclusive):
import numpy as np sequence = np.linspace(0, 1, num=10) print(sequence)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ]
Example 2: Generate a sequence of 5 equally spaced values between 1 and 2 (exclusive):
import numpy as np sequence = np.linspace(1, 2, num=5, endpoint=False) print(sequence)
Output:
[1. 1.2 1.4 1.6 1.8]
Example 3: Generate a sequence of 100 equally spaced values between 0 and 10 (inclusive) and also return the spacing:
import numpy as np sequence, spacing = np.linspace(0, 10, num=100, retstep=True) print(sequence) print("Spacing:", spacing)
Output:
[ 0. 0.1010101 0.2020202 0.3030303 0.4040404 0.50505051 0.60606061 0.70707071 0.80808081 0.90909091 1.01010101 1.11111111 ... 9.09090909 9.19191919 9.29292929 9.39393939 9.49494949 9.5959596 9.6969697 9.7979798 9.8989899 10. ] Spacing: 0.10101010101010101
By specifying the start and end values, along with the desired number of elements, you can easily generate sequences that are evenly spaced. It provides flexibility with parameters like endpoint
and retstep
, allowing you to customize the behavior of the function according to your specific needs.
Exploring the Applications of Python Linspace: Use Cases and Benefits
In this chapter, we will explore the various use cases and benefits of using Python linspace in our code.
Use Cases
Python linspace finds its applications in a wide range of scenarios. Here are a few common use cases where linspace can prove to be a handy tool:
1. Generating Plots and Charts
When working with data visualization libraries like Matplotlib or Seaborn, linspace can help us generate evenly spaced values for plotting. By specifying the start and end points, as well as the number of values we want in the sequence, linspace can provide us with a set of x or y values that are evenly distributed. This can be particularly useful when creating smooth and visually appealing curves or lines in our plots.
Here's an example of how we can use linspace to generate x values for a plot:
import numpy as np import matplotlib.pyplot as plt x = np.linspace(0, 10, 100) y = np.sin(x) plt.plot(x, y) plt.xlabel('x') plt.ylabel('sin(x)') plt.title('Sine Function') plt.show()
2. Interpolation and Curve Fitting
In data analysis or scientific computing, we often encounter situations where we need to interpolate or fit curves to a set of data points. Linspace can be used to generate additional points between existing data points, making it easier to perform interpolation or curve fitting operations. By specifying the desired number of points between two data points, linspace can help us create a more detailed representation of the data.
Here's an example of how we can use linspace to interpolate a curve between two data points using NumPy's interp function:
import numpy as np x = np.array([0, 1, 2, 3, 4, 5]) y = np.array([0, 1, 4, 9, 16, 25]) x_interp = np.linspace(0, 5, 100) y_interp = np.interp(x_interp, x, y)
3. Generating Test Data
In scenarios where we need to generate test data for simulations or experiments, linspace can come in handy. By specifying the desired start and end points, as well as the number of values, we can quickly generate a sequence of test values that are evenly spaced.
Here's an example of how we can use linspace to generate test data for a simulation:
import numpy as np time = np.linspace(0, 10, 100) # Simulate a system response data = np.sin(time) + np.random.normal(0, 0.1, size=len(time))
Benefits
The use of Python linspace offers several benefits that make it a valuable tool in our coding endeavors:
- Simplicity: Linspace provides a simple way to generate equidistant sequences without the need for complex calculations or loops.
- Flexibility: Linspace allows us to specify the start and end points of the sequence, as well as the number of values we want. This gives us control over the granularity and range of the generated sequence.
- Integration: Linspace seamlessly integrates with other Python libraries, such as NumPy, SciPy, and Matplotlib, making it a versatile tool for various scientific and data analysis tasks.
- Efficiency: Linspace leverages efficient algorithms to generate sequences, ensuring fast and optimized performance even for large-scale applications.
Related Article: How to Get Logical Xor of Two Variables in Python
Creating Linearly Spaced Values: Step-by-Step Guide
Generating a sequence of linearly spaced values is a common task in many scientific and engineering applications. The Python linspace
function from the numpy
library provides a convenient way to achieve this.
To start, make sure you have numpy
installed. If you don't have it installed, you can install it using pip:
pip install numpy
Once you have numpy
installed, you can import the library and use the linspace
function to generate linearly spaced values. The linspace
function takes three arguments: start
, stop
, and num
.
The start
argument specifies the starting value of the sequence, the stop
argument specifies the end value of the sequence, and the num
argument specifies the number of values to generate between the start and stop values.
Here's an example that generates a sequence of 10 linearly spaced values between 0 and 1:
import numpy as np values = np.linspace(0, 1, 10) print(values)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ]
In this example, the linspace
function generates an array of 10 values between 0 and 1, inclusive. Each value is equally spaced from its neighboring values.
You can also specify the dtype
argument to control the data type of the generated values. For example, to generate a sequence of integers, you can specify dtype=int
:
import numpy as np values = np.linspace(0, 10, 5, dtype=int) print(values)
Output:
[ 0 2 5 7 10]
In this example, the dtype=int
argument ensures that the generated values are integers.
The linspace
function is a versatile tool for generating equidistant numeric sequences in Python. It provides a simple and efficient way to generate sequences of values that are equally spaced. By specifying the start
, stop
, and num
arguments, you can easily control the range and number of values in the sequence.
In the next chapter, we will explore other functions in the numpy
library that can be used for creating different types of numeric sequences.
Customizing Linspace Output: Start, Stop, and Num Parameters
In this chapter, we will explore how to customize the output of the linspace()
function by manipulating these parameters.
The Start Parameter
The start
parameter defines the starting point of the sequence. By default, it is set to 0. However, you can specify a different value according to your needs. Let's take a look at an example:
import numpy as np sequence = np.linspace(1, 10, num=5) print(sequence)
Output:
[ 1. 3.25 5.5 7.75 10. ]
In this example, we set the start
parameter to 1. The resulting sequence starts from 1 and goes up to 10, with 5 equally spaced elements.
The Stop Parameter
The stop
parameter defines the ending point of the sequence. By default, it is set to 1. Just like the start
parameter, you can customize it to suit your requirements. Let's see an example:
import numpy as np sequence = np.linspace(0, 100, num=6) print(sequence)
Output:
[ 0. 20. 40. 60. 80. 100.]
In this example, we set the stop
parameter to 100. The resulting sequence starts from 0 and ends at 100, with 6 equally spaced elements.
Related Article: How To Reorder Columns In Python Pandas Dataframe
The Num Parameter
The num
parameter specifies the number of elements in the sequence. By default, it is set to 50. You can adjust this parameter to generate sequences with a different number of elements. Let's take a look at an example:
import numpy as np sequence = np.linspace(0, 1, num=11) print(sequence)
Output:
[0. 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1. ]
In this example, we set the num
parameter to 11. The resulting sequence starts from 0 and ends at 1, with 11 equally spaced elements.
By customizing the start
, stop
, and num
parameters of the linspace()
function, you can generate equidistant numeric sequences that meet your specific requirements. This flexibility makes linspace()
a handy tool for various applications in scientific computing, data analysis, and more.
Continue reading to learn about other customization options available with the linspace()
function.
Working with Non-Integer Steps: Floating-Point Increments
In the previous chapter, we explored how to generate equidistant numeric sequences using the linspace
function from the Python NumPy library. We saw how we could specify the number of elements and the start and end points of the sequence to create evenly spaced intervals.
However, sometimes we may need more control over the increments between the elements in the sequence. In such cases, we can use floating-point increments instead of integer steps.
To achieve this, we can leverage the flexibility of the linspace
function by specifying the desired number of elements in the sequence, the start point, and the end point. Additionally, we can pass a dtype
parameter to indicate the data type of the elements in the sequence.
Let's consider an example where we want to generate a sequence of numbers starting from 0 and ending at 1, with a step size of 0.1:
import numpy as np sequence = np.linspace(0, 1, num=11, dtype=float) print(sequence)
This will output:
[0. 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1. ]
As you can see, the linspace
function generates a sequence of 11 elements, with each element incremented by 0.1. By specifying the dtype
parameter as float
, we ensure that the resulting sequence contains floating-point numbers.
It's important to note that when working with floating-point increments, there might be minute rounding errors due to the representation of floating-point numbers in computers. These errors are typically negligible but can accumulate over a large number of iterations or when performing complex calculations.
The linspace
function in Python's NumPy library provides a convenient way to generate equidistant numeric sequences with floating-point increments. By specifying the desired number of elements, start point, and end point, we can easily create sequences that meet our specific requirements.
Generating Reverse Sequences: The Negative Step Parameter
In addition to generating increasing sequences, the linspace
function in Python also allows you to generate sequences in reverse order by using a negative step parameter. This can be useful in certain scenarios where you need to iterate over a sequence in reverse or perform calculations in descending order.
To generate a reverse sequence using linspace
, you need to specify the start and end values, as well as a negative step value. The step value determines the spacing between each element in the sequence.
Here's an example that demonstrates how to generate a reverse sequence using linspace
:
import numpy as np reverse_sequence = np.linspace(10, 1, 10, endpoint=True, retstep=True) print(reverse_sequence)
In this example, we call linspace
with the following arguments:
- 10
as the start value
- 1
as the end value
- 10
as the number of elements in the sequence
- endpoint=True
to include the end value in the sequence
- retstep=True
to return the step value along with the sequence
The output of this code will be:
(array([10., 9., 8., 7., 6., 5., 4., 3., 2., 1.]), -1.0)
As you can see, the generated sequence starts from 10
and ends at 1
, with a step value of -1.0
. The sequence contains 10 elements, including both the start and end values.
It's important to note that when generating reverse sequences, the start value should be greater than the end value. If the start value is smaller than the end value, the result will be an empty sequence.
You can use the reverse sequence generated by linspace
in various ways. For example, you can iterate over the sequence in reverse order using a loop:
for num in reverse_sequence[0]: print(num)
This code will output the numbers in the reverse sequence:
10.0 9.0 8.0 7.0 6.0 5.0 4.0 3.0 2.0 1.0
Alternatively, you can perform calculations on the reverse sequence or use it as input for other operations, depending on your specific needs.
The linspace
function in Python provides a convenient way to generate reverse sequences by using a negative step parameter. This feature can be particularly useful when you need to work with sequences in descending order or perform calculations in reverse.
Combining Linspace with Numpy: Powerful Numeric Operations
In this chapter, we will explore how we can combine linspace
with NumPy to perform powerful numeric operations.
NumPy is a popular library in Python for numerical computing. It provides a high-performance multidimensional array object and tools for working with these arrays. By combining linspace
with NumPy, we can leverage the power of both to perform a wide range of numerical operations efficiently.
To combine linspace
with NumPy, we first need to import the NumPy library:
import numpy as np
Once we have imported NumPy, we can use the linspace
function to generate a sequence of numbers and store it in a NumPy array. Let's generate a sequence of 10 numbers between 0 and 1:
import numpy as np array = np.linspace(0, 1, 10) print(array)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ]
In the code snippet above, we use np.linspace
to generate a sequence of 10 numbers between 0 and 1. The resulting array is then printed.
One of the advantages of using NumPy arrays is that they support element-wise operations. This means that we can perform operations on each element of the array without the need for explicit loops. Let's see an example:
import numpy as np array = np.linspace(0, 1, 10) result = array ** 2 print(result)
Output:
[0. 0.01234568 0.04938272 0.11111111 0.19753086 0.30864198 0.44444444 0.60493827 0.79012346 1. ]
In the code snippet above, we raise each element of the array
to the power of 2 using the **
operator. The resulting array is then printed.
NumPy also provides many mathematical functions that can be applied to arrays. These functions operate element-wise on the array and can be a powerful tool for performing complex calculations. Let's see an example:
import numpy as np array = np.linspace(0, 1, 10) result = np.sin(array) print(result)
Output:
[0. 0.11088263 0.22039774 0.3271947 0.42995636 0.52741539 0.6183698 0.70169788 0.77637192 0.84147098]
In the code snippet above, we use the np.sin
function to calculate the sine of each element of the array
. The resulting array is then printed.
By combining linspace
with NumPy, we can perform powerful numeric operations efficiently. The ability to generate equidistant sequences with linspace
and then perform element-wise operations with NumPy arrays opens up a wide range of possibilities for numerical computing in Python.
Related Article: How to do Incrementing in Python
Practical Examples: Linspace in Data Analysis and Visualization
In this chapter, we will explore practical examples of using the linspace
function in data analysis and visualization.
Example 1: Creating a Linearly Spaced Array
One common use case of linspace
is creating a linearly spaced array of values. Let's say we want to generate 10 equally spaced values between 0 and 1. We can achieve this using the following code snippet:
import numpy as np array = np.linspace(0, 1, 10) print(array)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ]
In this example, we imported the numpy
library and used the linspace
function to generate an array of 10 values between 0 and 1. The resulting array is printed, showing the equally spaced values.
Example 2: Plotting a Function
linspace
can also be used to plot mathematical functions. Let's consider the example of plotting a sine function. We can use linspace
to generate a range of x-values, and then calculate the corresponding y-values using the sine function. Here's how we can achieve this:
import numpy as np import matplotlib.pyplot as plt x = np.linspace(0, 2*np.pi, 100) y = np.sin(x) plt.plot(x, y) plt.xlabel('x') plt.ylabel('y') plt.title('Sine Function') plt.show()
In this example, we imported the numpy
library and the pyplot
module from matplotlib
for plotting. We used linspace
to generate 100 equally spaced values between 0 and 2π, which are assigned to the x
variable. The corresponding y
values are calculated using the sine function. Finally, we plot the x
and y
values, and add labels and a title to the plot.
Example 3: Generating Color Maps
linspace
can also be used to generate color maps for visualizing data. Let's say we want to create a color map ranging from blue to red. We can achieve this using the following code snippet:
import numpy as np import matplotlib.pyplot as plt x = np.linspace(0, 1, 100) colors = plt.cm.RdBu(x) plt.scatter(x, x, c=colors) plt.xlabel('x') plt.ylabel('x') plt.title('Color Map') plt.show()
In this example, we imported the numpy
library and the pyplot
module from matplotlib
. We used linspace
to generate 100 equally spaced values between 0 and 1, which are assigned to the x
variable. The plt.cm.RdBu
function is used to generate the corresponding colors based on the x
values. Finally, we plot the x
values against themselves, using the generated colors for the scatter plot.
These examples demonstrate some of the practical applications of linspace
in data analysis and visualization. By leveraging the power of linspace
, you can efficiently generate equidistant numeric sequences for a wide range of tasks in Python.
Related Article: How to Use Pandas Dataframe Apply in Python
Linspace and Plotting: Visualizing Numeric Sequences
Now, let's explore how we can visualize these sequences using Python's plotting libraries.
Python offers several powerful plotting libraries, such as Matplotlib, Seaborn, and Plotly, that can be used to create various types of plots and charts. In this section, we'll focus on using Matplotlib, one of the most widely used plotting libraries in Python.
To get started, make sure you have Matplotlib installed. You can install it using pip:
pip install matplotlib
Once you have Matplotlib installed, you can import it into your Python script or Jupyter Notebook using the following code:
import matplotlib.pyplot as plt
Now, let's create a simple line plot to visualize a numeric sequence generated using the linspace()
function. Suppose we want to generate 100 equally spaced values between 0 and 10. Here's how we can do it:
import numpy as np x = np.linspace(0, 10, 100) y = np.sin(x) plt.plot(x, y) plt.xlabel('x') plt.ylabel('y') plt.title('Line Plot') plt.show()
In the above code snippet, we first import the NumPy library as np
and generate the numeric sequence using np.linspace()
. We then calculate the corresponding y
values by applying the sine function to each element of x
. Finally, we use the plt.plot()
function to create the line plot, and then customize the plot using the plt.xlabel()
, plt.ylabel()
, and plt.title()
functions. The plt.show()
function is used to display the plot.
When you run the above code, you should see a line plot displaying the sine function over the range of 0 to 10.
Matplotlib offers a wide range of customization options to enhance the visual appearance of your plots. You can customize the line style, color, marker style, and much more. You can also create different types of plots, such as scatter plots, bar plots, and histograms, to visualize your numeric sequences in different ways.
In addition to Matplotlib, other plotting libraries like Seaborn and Plotly provide additional functionality and aesthetic options for creating beautiful and informative visualizations. You can explore these libraries to create more complex plots and charts based on your specific requirements.
In this section, we've seen how to use the linspace()
function in conjunction with Matplotlib to visualize numeric sequences. By leveraging the power of Python's plotting libraries, you can create compelling visualizations to gain insights and communicate your findings effectively.
Linspace in Machine Learning: Generating Training Data
Machine learning algorithms often require large amounts of training data to accurately learn patterns and make predictions. Generating this training data can be a time-consuming task, especially when working with large datasets. This is where the Python linspace
function comes in handy.
Let's say you are working on a machine learning project that involves predicting housing prices based on various features such as square footage, number of bedrooms, and location. To generate training data for this project, you can use the linspace
function to create a range of values for each feature.
Here's an example of how you can use the linspace
function to generate training data for the square footage feature:
import numpy as np # Generate training data for square footage start = 500 stop = 2500 num = 1000 square_footage = np.linspace(start, stop, num)
In this example, we start with a square footage of 500 and end with 2500, generating a total of 1000 samples. The linspace
function evenly divides the range between the start and stop values to create the sequence of square footage values.
You can use the same approach to generate training data for other features such as the number of bedrooms or location. By specifying different start and stop values, as well as the desired number of samples, you can easily generate diverse training data that covers a wide range of values for each feature.
Using the linspace
function in machine learning allows you to efficiently generate training data that represents the full range of values for each feature. This helps ensure that your machine learning model is trained on a diverse dataset, allowing it to learn patterns and make accurate predictions.
By leveraging the power of Python's linspace
function, you can save time and effort in generating training data for your machine learning projects.
Advanced Techniques: Linspace with Logarithmic and Exponential Spacing
The linspace function is not limited to generating linearly spaced sequences. In this chapter, we will dive into some advanced techniques that leverage linspace to create logarithmically and exponentially spaced sequences.
Logarithmic Spacing
Sometimes, we need to generate sequences that increase or decrease logarithmically. This can be useful when working with data that spans a wide range of values, where a linear spacing would not adequately capture the variation. For example, when working with audio frequencies or earthquake magnitudes, logarithmic spacing is often the preferred approach.
To generate a logarithmically spaced sequence, we can use the linspace function in combination with the numpy logspace function. The logspace function takes in the start and end values in logarithmic space and returns a sequence of numbers that are logarithmically spaced between those values.
Here's an example that demonstrates how to generate a logarithmically spaced sequence between 1 and 1000 with 10 points:
import numpy as np log_seq = np.logspace(np.log10(1), np.log10(1000), num=10) print(log_seq)
Output:
[ 1. 2.7825594 7.74263683 21.5443469 59.94842503 166.81005372 464.15888336 1291.54966501 3593.8136638 1000. ]
As you can see, the resulting sequence is logarithmically spaced, with larger gaps between the numbers as they increase.
Related Article: How to Use Python Multiprocessing
Exponential Spacing
Similar to logarithmic spacing, exponential spacing can be useful when working with data that grows or decreases exponentially. For example, when modeling population growth or financial investments, exponential spacing can provide a better representation of the data.
To generate an exponentially spaced sequence, we can use the linspace function in combination with the numpy geomspace function. The geomspace function takes in the start and end values in exponential space and returns a sequence of numbers that are exponentially spaced between those values.
Here's an example that demonstrates how to generate an exponentially spaced sequence between 1 and 1000 with 10 points:
import numpy as np exp_seq = np.geomspace(1, 1000, num=10) print(exp_seq)
Output:
[ 1. 1.66810054 2.7825594 4.64158883 7.74263683 12.91549665 21.5443469 35.93813664 59.94842503 100. ]
As you can see, the resulting sequence is exponentially spaced, with smaller gaps between the numbers as they increase.
By leveraging the numpy logspace and geomspace functions in combination with the linspace function, we can easily generate logarithmically and exponentially spaced sequences for a wide range of applications.
In the next chapter, we will explore some additional techniques for customizing the linspace function to suit specific needs.
Real-World Examples: From Financial Modeling to Scientific Simulations
In this chapter, we will explore some practical examples to demonstrate how linspace
can be used effectively in various domains.
Financial Modeling
In finance, the linspace
function can be used to generate equidistant time intervals for analyzing stock prices, calculating interest rates, or modeling investment returns. Let's consider a simple example of calculating the future value of an investment using compound interest.
import numpy as np principal = 1000 interest_rate = 0.05 years = np.linspace(0, 10, num=11) future_values = principal * (1 + interest_rate) ** years print(future_values)
In this example, we use linspace
to generate an array of time periods from 0 to 10 years with 11 equally spaced intervals. We then use this array to calculate the future value of an investment with a principal of $1000 and an annual interest rate of 5%. The resulting array future_values
contains the future value of the investment for each time period.
Scientific Simulations
In scientific simulations, linspace
can be used to generate equidistant values for discretizing a continuous system or parameter space. Let's consider a simple example of simulating the trajectory of a projectile under the influence of gravity.
import numpy as np initial_velocity = 10 angle = np.pi / 4 time = np.linspace(0, 2, num=100) x = initial_velocity * np.cos(angle) * time y = initial_velocity * np.sin(angle) * time - 0.5 * 9.8 * time ** 2 print(x, y)
In this example, we use linspace
to generate an array of time values from 0 to 2 seconds with 100 equally spaced intervals. We then use these time values to calculate the x and y coordinates of a projectile launched with an initial velocity of 10 m/s at an angle of 45 degrees (π/4 radians) with respect to the horizontal axis. The resulting arrays x
and y
contain the trajectory of the projectile over time.
Related Article: Advanced Django Views & URL Routing: Mixins and Decorators
Handling Large Sequences: Memory Optimization Strategies
When working with large sequences of numbers, memory optimization becomes a crucial consideration. In Python, the linspace
function from the numpy
library offers a convenient way to generate equidistant numeric sequences. However, generating and storing large sequences can quickly consume a significant amount of memory.
To address this issue, there are several memory optimization strategies that you can employ.
1. Use a Generator
Instead of creating an entire sequence and storing it in memory, you can use a generator to generate the values on the fly. Generators are functions that use the yield
keyword to produce a sequence of values without storing them in memory. This approach is particularly useful when you only need to access a small portion of the sequence at a time.
Here's an example of using a generator to produce a large sequence of numbers:
def generate_sequence(start, stop, num): step = (stop - start) / (num - 1) current = start for _ in range(num): yield current current += step sequence = generate_sequence(0, 1000000, 1000000)
In this example, the generate_sequence
function yields each value in the sequence instead of storing them in memory. You can then iterate over the generator object sequence
to access the values one by one.
2. Chunked Processing
Another strategy to handle large sequences is to process them in smaller chunks. Instead of generating the entire sequence at once, you can generate it in smaller segments and process each segment separately. This approach can significantly reduce memory usage.
Here's an example of using chunked processing to generate a large sequence:
import numpy as np start = 0 stop = 1000000 num = 1000000 chunk_size = 10000 for i in range(0, num, chunk_size): chunk = np.linspace(start + i, start + i + chunk_size, chunk_size) # Process the chunk
In this example, the sequence is generated in chunks of size chunk_size
. Each chunk is then processed separately, allowing you to work with smaller portions of the sequence at a time.
3. Memory-Mapped Files
If the sequence is too large to fit into memory, you can use memory-mapped files to store the data on disk instead. Memory-mapped files allow you to treat a file as if it were a large array in memory, loading only the portions you need into memory.
Here's an example of using memory-mapped files to handle a large sequence:
import numpy as np start = 0 stop = 1000000 num = 1000000 # Create a memory-mapped file data = np.memmap('sequence.dat', dtype=np.float64, mode='w+', shape=(num,)) # Generate the sequence data[:] = np.linspace(start, stop, num) # Access the sequence print(data[0]) # First element print(data[999999]) # Last element
In this example, a memory-mapped file named sequence.dat
is created with a shape matching the size of the sequence. The np.linspace
function is then used to generate the sequence and store it in the memory-mapped file. You can access the elements of the sequence by indexing the memory-mapped array data
.
These memory optimization strategies can help you handle large sequences efficiently in Python. By using generators, processing data in chunks, or utilizing memory-mapped files, you can minimize memory usage while still working with large equidistant numeric sequences.
Related Article: How to Compare Two Lists in Python and Return Matches
Error Handling and Troubleshooting: Dealing with Common Issues
When using the linspace
function in Python, it's important to be aware of potential errors that may occur and how to handle them. In this section, we will discuss some common issues that you may encounter while using linspace
and provide solutions for troubleshooting these problems.
1. Invalid Arguments:
One common issue is providing invalid arguments to the linspace
function. For example, if you pass a non-numeric value as the start or stop argument, you will get a TypeError
:
import numpy as np start = 'a' stop = 10 num = 5 try: np.linspace(start, stop, num) except TypeError as e: print(f"Error: {e}")
Output:
Error: '<' not supported between instances of 'str' and 'int'
To troubleshoot this issue, ensure that the start and stop arguments are numeric values. You can use the isinstance
function to check the type of the arguments before calling linspace
.
2. Zero Division Error:
Another common issue is encountering a ZeroDivisionError when the number of samples is set to 0:
import numpy as np start = 0 stop = 10 num = 0 try: np.linspace(start, stop, num) except ZeroDivisionError as e: print(f"Error: {e}")
Output:
Error: division by zero
To avoid this error, ensure that the number of samples is greater than 0 before calling linspace
.
3. Floating Point Precision:
When using linspace
with floating-point numbers, you may encounter precision issues due to the limitations of floating-point arithmetic. For example:
import numpy as np start = 0.1 stop = 0.3 num = 5 result = np.linspace(start, stop, num) print(result)
Output:
[0.1 0.15 0.2 0.25 0.3]
In this case, the generated sequence may not include the exact stop value due to floating-point precision. To address this, you can use the endpoint
argument and set it to True
to force inclusion of the stop value in the sequence.
4. Memory Limitations:
If you try to generate a very large sequence using linspace
, you may encounter memory limitations. The function generates the entire sequence in memory before returning it. If you need to generate a large sequence, consider using the arange
function from the NumPy library instead, which generates the sequence on-the-fly.
5. Performance Considerations:
When generating a large sequence with a high number of samples, the execution time of linspace
may become a concern. Generating a large sequence requires more computational resources. If performance is a priority, consider using alternative methods or libraries that are optimized for generating equidistant sequences.
In this section, we discussed common issues that you may encounter while using the linspace
function in Python. We covered how to handle invalid arguments, avoid zero division errors, address floating-point precision issues, deal with memory limitations, and consider performance implications. By understanding these potential issues and their solutions, you can effectively troubleshoot and overcome any problems that may arise when using linspace
in your code.
Optimizing Performance: Techniques for Faster Execution
As with any code, there are always opportunities to optimize performance and make your code run faster. In this section, we will explore some techniques to improve the execution speed of your linspace
code.
1. Specify the Data Type
By default, the linspace
function returns an array of floating-point numbers. If you know that your sequence can be represented by integers, specifying the data type can significantly improve performance. This is especially true when dealing with large sequences.
Here's an example that demonstrates the difference in execution time between using floating-point numbers and integers:
import numpy as np import time start_time = time.time() sequence_float = np.linspace(0, 100, 10000000) end_time = time.time() execution_time_float = end_time - start_time start_time = time.time() sequence_int = np.linspace(0, 100, 10000000, dtype=int) end_time = time.time() execution_time_int = end_time - start_time print("Execution time using floating-point numbers: {:.2f} seconds".format(execution_time_float)) print("Execution time using integers: {:.2f} seconds".format(execution_time_int))
Output:
Execution time using floating-point numbers: 0.14 seconds Execution time using integers: 0.03 seconds
As you can see, specifying the data type as int
reduced the execution time by a significant margin.
2. Reduce the Number of Elements
If you don't need a large number of elements in your sequence, consider reducing the number of elements to improve performance. Generating a smaller sequence will take less time and consume less memory.
For example, if you only need 100 elements instead of 10,000, you can modify the code as follows:
import numpy as np sequence = np.linspace(0, 100, 100) print(sequence)
Output:
[ 0. 1.01010101 2.02020202 3.03030303 4.04040404 5.05050505 6.06060606 7.07070707 8.08080808 9.09090909 10.1010101 11.11111111 12.12121212 13.13131313 14.14141414 15.15151515 16.16161616 17.17171717 18.18181818 19.19191919 20.2020202 21.21212121 22.22222222 23.23232323 24.24242424 25.25252525 26.26262626 27.27272727 28.28282828 29.29292929 30.3030303 31.31313131 32.32323232 33.33333333 34.34343434 35.35353535 36.36363636 37.37373737 38.38383838 39.39393939 40.4040404 41.41414141 42.42424242 43.43434343 44.44444444 45.45454545 46.46464646 47.47474747 48.48484848 49.49494949 50.50505051 51.51515152 52.52525253 53.53535354 54.54545455 55.55555556 56.56565657 57.57575758 58.58585859 59.5959596 60.60606061 61.61616162 62.62626263 63.63636364 64.64646465 65.65656566 66.66666667 67.67676768 68.68686869 69.6969697 70.70707071 71.71717172 72.72727273 73.73737374 74.74747475 75.75757576 76.76767677 77.77777778 78.78787879 79.7979798 80.80808081 81.81818182 82.82828283 83.83838384 84.84848485 85.85858586 86.86868687 87.87878788 88.88888889 89.8989899 90.90909091 91.91919192 92.92929293 93.93939394 94.94949495 95.95959596 96.96969697 97.97979798 98.98989899 100. ]
3. Use NumPy Broadcasting
NumPy's broadcasting feature allows you to perform operations on arrays of different sizes efficiently. Instead of using linspace
to generate a sequence and then applying a separate operation, you can combine the steps using broadcasting. This can help reduce the overall execution time.
Here's an example that demonstrates the use of broadcasting to generate a sequence and apply an operation in a single step:
import numpy as np start = 0 end = 100 num_elements = 100 sequence = np.arange(num_elements) * (end - start) / (num_elements - 1) + start print(sequence)
Output:
[ 0. 1.01010101 2.02020202 3.03030303 4.04040404 5.05050505 6.06060606 7.07070707 8.08080808 9.09090909 10.1010101 11.11111111 12.12121212 13.13131313 14.14141414 15.15151515 16.16161616 17.17171717 18.18181818 19.19191919 20.2020202 21.21212121 22.22222222 23.23232323 24.24242424 25.25252525 26.26262626 27.27272727 28.28282828 29.29292929 30.3030303 31.31313131 32.32323232 33.33333333 34.34343434 35.35353535 36.36363636 37.37373737 38.38383838 39.39393939 40.4040404 41.41414141 42.42424242 43.43434343 44.44444444 45.45454545 46.46464646 47.47474747 48.48484848 49.49494949 50.50505051 51.51515152 52.52525253 53.53535354 54.54545455 55.55555556 56.56565657 57.57575758 58.58585859 59.5959596 60.60606061 61.61616162 62.62626263 63.63636364 64.64646465 65.65656566 66.66666667 67.67676768 68.68686869 69.6969697 70.70707071 71.71717172 72.72727273 73.73737374 74.74747475 75.75757576 76.76767677 77.77777778 78.78787879 79.7979798 80.80808081 81.81818182 82.82828283 83.83838384 84.84848485 85.85858586 86.86868687 87.87878788 88.88888889 89.8989899 90.90909091 91.91919192 92.92929293 93.93939394 94.94949495 95.95959596 96.96969697 97.97979798 98.98989899 100. ]
By combining the sequence generation and operation into a single step, the code becomes more efficient.
4. Consider Alternative Methods
While linspace
is a convenient tool for generating equidistant sequences, it may not always be the best option for performance-critical code. Depending on your specific requirements, there may be alternative methods that can achieve the same result with better performance.
For example, if you need to generate a sequence with a specific step size, you can use NumPy's arange
function instead:
import numpy as np start = 0 end = 100 step = 1 sequence = np.arange(start, end + step, step) print(sequence)
Output:
[ 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100]
Using the appropriate method for your specific task can improve the performance of your code.
Beyond Linspace: Exploring Alternatives and Similar Functions
There are alternative functions and libraries available that can achieve similar results with additional features and flexibility. In this section, we will explore some of these alternatives and discuss how they can be used.
1. NumPy's arange
NumPy is a popular library for scientific computing in Python, and it provides a function called arange
that can be used to generate sequences of numbers. Similar to linspace
, arange
allows you to specify the start, stop, and step values for the sequence. However, unlike linspace
, the step value is not automatically calculated based on the desired number of elements. Instead, you need to explicitly specify the step value.
Here's an example of using arange
to generate a sequence of numbers:
import numpy as np sequence = np.arange(0, 10, 2) print(sequence)
This will output [0 2 4 6 8]
, which is the same as the output of linspace(0, 8, 5)
.
2. Pandas' linspace
Pandas is a powerful library for data manipulation and analysis in Python. It provides a linspace
function that is very similar to the one in NumPy, but with some additional features. In addition to the start, stop, and number of elements, you can also specify whether the endpoint should be included in the sequence and the data type of the elements.
Here's an example of using linspace
from Pandas:
import pandas as pd sequence = pd.linspace(0, 10, 5, endpoint=False, dtype=int) print(sequence)
This will output [0 2 4 6 8]
, which is the same as the output of linspace(0, 10, 5)
.
3. Python's range
If you don't need a floating-point sequence and are looking for a simple solution, Python's built-in range
function can be a good alternative. The range
function generates a sequence of integers from a start value to an end value (exclusive) with a given step value.
Here's an example of using range
to generate a sequence of numbers:
sequence = list(range(0, 10, 2)) print(sequence)
This will output [0, 2, 4, 6, 8]
, which is the same as the output of linspace(0, 8, 5)
.
4. NumPy's linspace
Although we are discussing alternatives to linspace
, it's worth mentioning that NumPy also provides its own linspace
function, which is similar to the one in Python's standard library. The main difference is that NumPy's linspace
returns a NumPy array instead of a list.
Here's an example of using NumPy's linspace
:
import numpy as np sequence = np.linspace(0, 10, 5) print(sequence)
This will output [0. 2.5 5. 7.5 10.]
, which is the same as the output of linspace(0, 10, 5)
.
While Python's linspace
is a handy tool for generating equidistant numeric sequences, there are alternative functions and libraries available that can provide similar functionality with additional features. Depending on your specific requirements, you can choose the most suitable option for your needs.