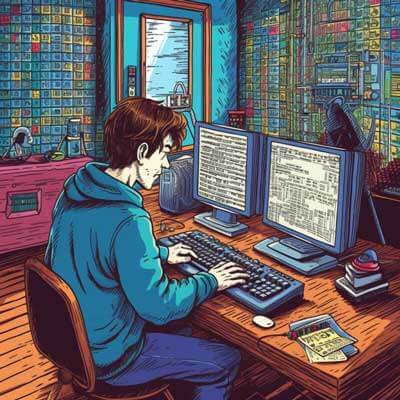
Table of Contents
Relative imports in Python 3 can sometimes be tricky to manage, especially when dealing with complex project structures or when attempting to run scripts from different directories. In this guide, we will explore different strategies to handle relative imports in Python 3.
Understanding Relative Imports
Relative imports in Python allow you to import modules or packages relative to the current module or package. They are especially useful when working within a package structure, as they provide a concise way to reference other modules within the same package.
Relative imports are specified using dot notation, where a single dot (.
) represents the current module or package, two dots (..
) represent the parent module or package, and so on. For example, if you have a package structure like this:
my_package/ __init__.py module1.py module2.py subpackage/ __init__.py module3.py
You can perform a relative import from module1.py
to module2.py
using the following syntax:
from . import module2
Or from module1.py
to module3.py
using:
from .subpackage import module3
Related Article: Tutorial: i18n in FastAPI with Pydantic & Handling Encoding
Handling "Attempted Relative Import with No Known Parent Package" Error
When attempting to use relative imports in Python, you may encounter the "Attempted relative import with no known parent package" error. This error typically occurs when you try to run a script directly using the Python interpreter instead of using the -m
flag.
To fix this error, you have a few options:
Option 1: Run the Script as a Module
Instead of running the script directly, run it as a module using the -m
flag. This tells Python to treat the script as a module and sets up the correct package context.
For example, if you have a script named my_script.py
located in the my_package
directory, you can run it using the following command:
python -m my_package.my_script
Option 2: Add the Project Root to sys.path
If you want to run the script directly without using the -m
flag, you can add the project root directory to the sys.path
list. This allows Python to locate the modules correctly.
To add the project root to sys.path
, you can include the following code at the beginning of your script:
import sys import os # Get the absolute path of the current script current_path = os.path.dirname(os.path.abspath(__file__)) # Get the absolute path of the project root project_root = os.path.dirname(current_path) # Add the project root to sys.path sys.path.append(project_root)
With the project root added to sys.path
, you should be able to use relative imports without encountering the "Attempted relative import with no known parent package" error.
Best Practices for Managing Relative Imports
To ensure smooth and consistent handling of relative imports in your Python projects, consider following these best practices:
1. Use Absolute Imports for Top-Level Packages
For top-level packages or modules, it is generally recommended to use absolute imports instead of relative imports. Absolute imports make your code more explicit and easier to understand, especially when working with larger projects.
For example, instead of using a relative import like this:
from .subpackage import module3
Use an absolute import like this:
from my_package.subpackage import module3
2. Avoid Circular Dependencies
Circular dependencies occur when two or more modules depend on each other, directly or indirectly. They can lead to import errors and make your codebase harder to maintain. To avoid circular dependencies, carefully design your package structure and dependencies, and consider refactoring your code if necessary.
3. Use Relative Imports Within a Package
Within a package, it is generally recommended to use relative imports to reference other modules within the same package. This helps maintain the integrity of the package structure and makes it easier to refactor or move modules without changing import statements.
4. Use Absolute Imports Across Different Packages
When importing modules from different packages, it is often better to use absolute imports to provide a clear and unambiguous reference. This helps prevent confusion and makes it easier to understand the origin of the imported module.
5. Follow a Consistent Import Style
Consistency is key when it comes to import styles. Choose a consistent import style for your project, whether it's relative imports or absolute imports, and stick to it throughout your codebase. This helps improve code readability and makes it easier for others to contribute to your project.
Alternative Approaches
In addition to the options described above, you may consider using alternative approaches to manage relative imports in Python. Some popular alternatives include:
1. Using a Virtual Environment
Using a virtual environment can help isolate your Python project and avoid conflicts with system-wide Python installations. Virtual environments provide a clean and separate Python environment where you can install packages and manage dependencies effectively.
2. Using a Package Manager
Package managers like pipenv and poetry offer additional features for managing packages and dependencies in Python projects. They can simplify the installation and management of packages, including handling relative imports within a project.
3. Refactoring Your Project Structure
If you find yourself struggling with complex relative imports or circular dependencies, it may be worth considering refactoring your project structure. By reorganizing your modules and packages, you can create a more logical and maintainable codebase.