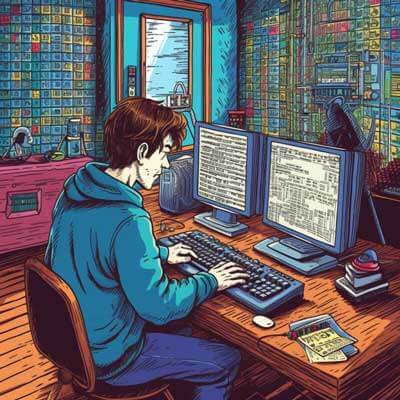
Table of Contents
Renaming column names in Pandas is a common task when working with dataframes. Whether it's to make column names more descriptive, standardize them, or simply to make them more readable, Pandas provides several methods to accomplish this. In this answer, we will explore different techniques to rename column names in Pandas and discuss their use cases.
Why is renaming column names necessary?
Before we dive into the various methods of renaming column names in Pandas, let's discuss why this task is necessary in the first place. There can be several reasons why you might want to rename column names in a dataframe:
1. **Improving readability**: Column names that are not self-explanatory or contain abbreviations can be difficult to understand. Renaming them to more descriptive names can make the dataframe easier to interpret.
2. **Standardization**: When working with multiple data sources, the column names may not be consistent. Renaming them to a common format can help standardize the data and make it easier to merge or analyze.
3. **Resolving conflicts**: If you are merging or concatenating dataframes, column name conflicts may arise. Renaming column names can help resolve these conflicts and prevent data loss.
4. **Conforming to naming conventions**: Sometimes, you may need to rename column names to adhere to specific naming conventions or coding standards.
Related Article: How to Define Stacks Data Structures in Python
Method 1: Using the rename()
method
Pandas provides a built-in rename()
method that allows you to rename column names in a dataframe. This method takes a dictionary-like object or a mapping function as input, where the keys represent the existing column names and the values represent the new column names.
Here's an example that demonstrates the usage of the rename()
method:
import pandas as pd # Create a sample dataframe data = {'Name': ['John', 'Emma', 'Mike'], 'Age': [25, 28, 32], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Rename the 'City' column to 'Location' df = df.rename(columns={'City': 'Location'}) print(df)
Output:
Name Age Location 0 John 25 New York 1 Emma 28 London 2 Mike 32 Paris
In the above example, the rename()
method is used to rename the 'City' column to 'Location'. The resulting dataframe has the updated column name.
It's worth noting that the rename()
method returns a new dataframe with the updated column names. If you want to modify the original dataframe in place, you can set the inplace
parameter to True
:
df.rename(columns={'City': 'Location'}, inplace=True)
Method 2: Using the set_axis()
method
Another way to rename column names in Pandas is by using the set_axis()
method. This method allows you to set new axis labels for either the columns or the index of the dataframe.
Here's an example that demonstrates the usage of the set_axis()
method to rename column names:
import pandas as pd # Create a sample dataframe data = {'Name': ['John', 'Emma', 'Mike'], 'Age': [25, 28, 32], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Rename the 'City' column to 'Location' using set_axis() df.set_axis(['Name', 'Age', 'Location'], axis=1, inplace=True) print(df)
Output:
Name Age Location 0 John 25 New York 1 Emma 28 London 2 Mike 32 Paris
In the above example, the set_axis()
method is used to rename the columns of the dataframe. The axis
parameter is set to 1
to indicate that we want to rename the column names. The new column names are passed as a list to the labels
parameter.
Method 3: Using the columns
attribute
Pandas dataframes have a built-in
columns
attribute that can be used to directly rename the column names. This approach allows you to modify the column names in place without creating a new dataframe.
Here's an example that demonstrates the usage of the columns
attribute to rename column names:
import pandas as pd # Create a sample dataframe data = {'Name': ['John', 'Emma', 'Mike'], 'Age': [25, 28, 32], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Rename the 'City' column to 'Location' using the columns attribute df.columns = ['Name', 'Age', 'Location'] print(df)
Output:
Name Age Location 0 John 25 New York 1 Emma 28 London 2 Mike 32 Paris
In the above example, the columns
attribute is directly assigned a new list of column names. This modifies the column names of the dataframe in place.
Related Article: How to Use the IsAlpha Function in Python
Method 4: Using the rename_axis()
method
If you want to rename the index name of the dataframe, you can use the rename_axis()
method. Although this method is primarily used to rename the index, it can also be used to rename the column names by specifying the columns
parameter.
Here's an example that demonstrates the usage of the rename_axis()
method to rename column names:
import pandas as pd # Create a sample dataframe data = {'Name': ['John', 'Emma', 'Mike'], 'Age': [25, 28, 32], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Rename the 'City' column to 'Location' using rename_axis() df = df.rename_axis(columns='Location') print(df)
Output:
Name Age Location 0 John 25 New York 1 Emma 28 London 2 Mike 32 Paris
In the above example, the rename_axis()
method is used to rename the column names. The columns
parameter is set to the desired new column name.
Best practices for renaming column names
When renaming column names in Pandas, it's important to follow some best practices to ensure code readability and maintainability:
1. **Use descriptive names**: Choose column names that accurately describe the data they represent. This helps other developers understand the dataframe structure and makes the code more readable.
2. **Be consistent**: Maintain consistent naming conventions across all column names. This makes it easier to work with the dataframe and avoids confusion.
3. **Avoid reserved keywords**: Avoid using reserved keywords or special characters in column names, as they can cause issues when accessing or manipulating the data.
4. **Use snake_case or camelCase**: It's common practice to use either snake_case or camelCase for column names. Choose one convention and stick to it throughout your codebase.
5. **Consider data type prefixes**: If you have columns with similar names but different data types (e.g., 'age' and 'age_group'), consider adding a prefix to indicate the data type. For example, 'int_age' and 'str_age_group'.
Alternative ideas
While the methods described above are the most common ways to rename column names in Pandas, there are a few alternative ideas worth considering:
1. **Using regular expressions**: If you have a large dataframe with many column names to rename, you can use regular expressions to match and replace specific patterns in the column names. This can be achieved using the re
module in Python.
2. **Using list comprehension**: If you need to apply a specific transformation or mapping function to rename column names, you can use list comprehension to iterate over the existing column names and generate a new list of renamed column names.
3. **Using third-party libraries**: There are several third-party libraries available that provide additional functionality for renaming column names in Pandas. Some popular libraries include pandas_flavor
and datarobot
.
Overall, the choice of method for renaming column names in Pandas depends on the specific requirements of your project and personal preference. It's important to choose a method that is both efficient and maintainable in the long run.