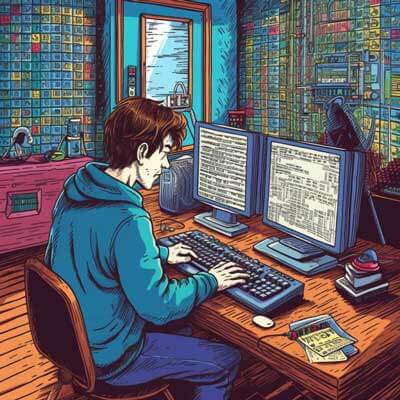
- Class-based views in Django
- Mixin classes and their usage in class-based views
- Understanding URL routing in Django
- Dynamic URLs using reverse URL patterns
- Modifying class-based views with decorators
- View functions and their differences from class-based views
- Extracting URL parameters in Django
- Defining URL patterns in Django
- Utilizing regular expressions in URL routing
- Organizing URLs with URL namespaces
- Additional Resources
Class-based views in Django
Class-based views in Django are a useful way to structure and organize your code when building web applications. They provide a reusable and modular approach to handling HTTP requests and generating responses. Unlike function-based views, which are defined as individual functions, class-based views are defined as classes.
To create a class-based view in Django, you need to define a class that inherits from one of the provided view classes provided by Django. These view classes are designed to handle different types of HTTP requests, such as GET, POST, PUT, DELETE, etc.
Here’s an example of a simple class-based view in Django:
from django.views import View from django.http import HttpResponse class MyView(View): def get(self, request): return HttpResponse("Hello, World!")
In this example, MyView
is a class-based view that handles GET requests. When a GET request is made to the corresponding URL, the get
method is called, and it returns an HttpResponse
object with the message “Hello, World!”.
Class-based views offer several advantages over function-based views. They promote code reuse and allow you to define common behavior in a base class and inherit from it. They also provide a more structured and object-oriented approach to handling requests, making it easier to organize and maintain your code as your application grows.
Related Article: Python Programming for Kids
Mixin classes and their usage in class-based views
Mixin classes in Django are a way to add additional functionality to class-based views without having to modify the base view class. They allow you to define reusable pieces of code that can be mixed into multiple views, promoting code reuse and keeping your views clean and modular.
Mixin classes are typically used to add specific behavior to a view, such as authentication, caching, or pagination. They are designed to be combined with other classes through multiple inheritance.
Here’s an example of a mixin class that adds authentication to a class-based view:
from django.contrib.auth.mixins import LoginRequiredMixin from django.views import View from django.http import HttpResponse class AuthenticatedView(LoginRequiredMixin, View): def get(self, request): return HttpResponse("Authenticated view")
In this example, the AuthenticatedView
class inherits from LoginRequiredMixin
and View
. The LoginRequiredMixin
is a built-in mixin class provided by Django that requires the user to be authenticated before accessing the view. The View
class is the base class for all class-based views.
Mixin classes can be used in conjunction with multiple inheritance to create complex and reusable views that combine different behaviors. They provide a flexible way to extend the functionality of class-based views and keep your code modular and maintainable.
Understanding URL routing in Django
URL routing is a fundamental concept in Django that allows you to map URLs to views in your web application. It determines how incoming requests are matched to the appropriate view for processing.
In Django, URL routing is typically defined in the urls.py
file of your project or app. This file contains a list of URL patterns, each of which is associated with a specific view or view function.
URL patterns in Django can be defined using regular expressions or simple strings. Regular expressions provide a more flexible and useful way to match URLs, while simple strings are useful for exact matches.
Here’s an example of URL routing in Django using regular expressions:
from django.urls import path from .views import MyView urlpatterns = [ path('hello/', MyView.as_view(), name='hello'), ]
In this example, the path
function is used to define a URL pattern. The first argument is the URL pattern itself, which is the string “hello/”. The second argument is the view that will be called when this URL pattern is matched, which is MyView.as_view()
. The third argument is an optional name for the URL pattern, which can be used to reverse the URL in your templates or views.
When a request is made to the URL “/hello/”, Django will match it to this URL pattern and call the get
method of the MyView
class.
URL routing in Django provides a flexible and useful way to map URLs to views in your web application. It allows you to define complex URL patterns and handle different types of requests using class-based views or view functions.
Dynamic URLs using reverse URL patterns
In Django, reverse URL patterns allow you to dynamically generate URLs based on the name of a URL pattern and its parameters. This is useful when you want to link to a specific URL in your templates or views without hardcoding the URL.
To use reverse URL patterns in Django, you need to provide a name for each URL pattern in your urls.py
file. This name can then be used in your templates or views to generate the corresponding URL.
Here’s an example of using reverse URL patterns in Django:
from django.urls import reverse from django.http import HttpResponse def my_view(request): url = reverse('hello') return HttpResponse(f"The URL is: {url}")
In this example, the reverse
function is used to generate the URL for the “hello” URL pattern. The generated URL is then returned as a response.
Reverse URL patterns can also accept additional arguments to generate dynamic URLs. For example:
from django.urls import reverse from django.http import HttpResponse def my_view(request, id): url = reverse('detail', args=[id]) return HttpResponse(f"The URL is: {url}")
In this example, the reverse
function is used to generate the URL for the “detail” URL pattern, which expects an id
parameter. The args
argument is used to pass the value of the id
parameter to the reverse function.
Reverse URL patterns provide a convenient way to generate dynamic URLs in your templates and views. They allow you to decouple your code from specific URLs, making it easier to maintain and update your application.
Related Article: Working with Numpy Concatenate
Modifying class-based views with decorators
Decorators in Python are a way to modify the behavior of functions or classes by wrapping them with additional functionality. They allow you to add or modify the behavior of a view without modifying its code directly.
In Django, decorators can be used to modify class-based views by applying them to the class definition. This is done using the @
syntax.
Here’s an example of using a decorator to modify a class-based view in Django:
from django.views.decorators.cache import cache_page from django.views import View from django.http import HttpResponse @cache_page(60 * 15) # Cache the view for 15 minutes class MyView(View): def get(self, request): return HttpResponse("Hello, World!")
In this example, the @cache_page
decorator is applied to the MyView
class. This decorator caches the output of the view for a specified amount of time (in this case, 15 minutes).
Decorators can also be applied to individual methods within a class-based view. For example:
from django.views.decorators.csrf import csrf_exempt from django.views import View from django.http import HttpResponse class MyView(View): @csrf_exempt # Disable CSRF protection for this view def post(self, request): return HttpResponse("Post request received")
In this example, the @csrf_exempt
decorator is applied to the post
method of the MyView
class. This decorator disables the Cross-Site Request Forgery (CSRF) protection for this particular method.
Decorators provide a flexible way to modify the behavior of class-based views in Django. They allow you to add or modify functionality without directly modifying the view code, making it easier to reuse and maintain your views.
View functions and their differences from class-based views
In addition to class-based views, Django also supports view functions, which are defined as individual functions rather than classes. View functions are a simpler and more lightweight alternative to class-based views.
Here’s an example of a view function in Django:
from django.http import HttpResponse def my_view(request): return HttpResponse("Hello, World!")
In this example, my_view
is a view function that takes a request
object as a parameter and returns an HttpResponse
object with the message “Hello, World!”.
View functions have a few key differences from class-based views:
1. Syntax: View functions are defined as individual functions, while class-based views are defined as classes.
2. Request handling: In view functions, the request object is passed as a parameter to the function. In class-based views, the request object is passed as an attribute of the view instance.
3. HTTP methods: View functions typically handle a specific HTTP method, such as GET or POST, by checking the request.method
attribute. Class-based views handle different HTTP methods using separate methods, such as get()
or post()
.
4. Decorators: View functions can be decorated with additional functionality using the @
syntax, similar to class-based views.
View functions are often used for simpler views that don’t require the additional structure and functionality provided by class-based views. They are lightweight and easy to understand, making them a good choice for simple use cases.
Extracting URL parameters in Django
In Django, URL parameters are values that are extracted from the URL and passed to the view or view function as arguments. They allow you to capture dynamic parts of the URL and use them in your views or view functions.
URL parameters in Django are typically defined using angle brackets () in the URL pattern. The captured values are then passed as arguments to the view or view function.
Here’s an example of extracting URL parameters in Django:
from django.urls import path from .views import MyView urlpatterns = [ path('hello//', MyView.as_view(), name='hello'), ]
In this example, the URL pattern “hello//” captures a string parameter named “name”. The captured value will be passed as an argument to the get
method of the MyView
class.
To access the URL parameter in the view or view function, you simply define a parameter with the same name as the captured value. For example:
from django.http import HttpResponse def my_view(request, name): return HttpResponse(f"Hello, {name}!")
In this example, the my_view
function takes a name
parameter, which corresponds to the captured value from the URL pattern. It returns an HttpResponse
object with a personalized greeting using the captured name.
URL parameters provide a flexible way to capture dynamic parts of the URL and use them in your views or view functions. They allow you to create dynamic and interactive web applications in Django.
Related Article: Diphthong Detection Methods in Python
Defining URL patterns in Django
URL patterns in Django are defined using the urlpatterns
list in the urls.py
file of your project or app. Each URL pattern is associated with a specific view or view function and determines how incoming requests are matched to the appropriate code for processing.
URL patterns in Django can be defined using simple strings or regular expressions. Simple strings are useful for exact matches, while regular expressions provide a more flexible and useful way to match URLs.
Here’s an example of defining URL patterns in Django using simple strings:
from django.urls import path from .views import MyView urlpatterns = [ path('hello/', MyView.as_view(), name='hello'), path('goodbye/', MyView.as_view(), name='goodbye'), ]
In this example, the URL patterns “hello/” and “goodbye/” are associated with the MyView
class. When a request is made to either of these URLs, Django will call the corresponding view’s get
method.
URL patterns can also include parameters to capture dynamic parts of the URL. For example:
from django.urls import path from .views import MyView urlpatterns = [ path('hello//', MyView.as_view(), name='hello'), ]
In this example, the URL pattern “hello//” captures a string parameter named “name”. The captured value will be passed as an argument to the get
method of the MyView
class.
URL patterns provide a flexible and useful way to define the structure of your web application’s URLs. They allow you to map incoming requests to the appropriate views or view functions, enabling you to build complex and interactive web applications in Django.
Utilizing regular expressions in URL routing
Regular expressions are a useful tool for pattern matching and manipulation. In Django, regular expressions can be used to define URL patterns, allowing for more flexible and dynamic URL routing.
To use regular expressions in URL routing in Django, you need to import the re_path
function from the django.urls
module. This function allows you to define URL patterns using regular expressions.
Here’s an example of utilizing regular expressions in URL routing in Django:
from django.urls import re_path from .views import MyView urlpatterns = [ re_path(r'^hello/(?P[a-zA-Z]+)/$', MyView.as_view(), name='hello'), ]
In this example, the re_path
function is used to define a URL pattern using a regular expression. The regular expression ^hello/(?P[a-zA-Z]+)/$
matches URLs that start with “hello/”, followed by a string of one or more alphabetic characters.
The captured value from the regular expression, named “name”, is passed as an argument to the get
method of the MyView
class.
Regular expressions provide a useful way to define complex URL patterns in Django. They allow you to match URLs based on specific patterns and capture dynamic parts of the URL for further processing in your views or view functions.
Organizing URLs with URL namespaces
URL namespaces in Django allow you to organize and group related URL patterns together, providing a more structured and modular way to define your application’s URLs.
To define a URL namespace in Django, you need to use the include
function and specify a unique name for the namespace.
Here’s an example of organizing URLs with URL namespaces in Django:
from django.urls import include, path from .views import MyView app_name = 'myapp' urlpatterns = [ path('hello/', MyView.as_view(), name='hello'), ] # Include the URLs of another app with a namespace urlpatterns += [ path('other/', include('otherapp.urls', namespace='other')), ]
In this example, the app_name
variable is set to the name of the app, which is “myapp”. This sets the default namespace for the URLs defined in this file.
The URL pattern “hello/” is associated with the MyView
class and has the name “hello” within the “myapp” namespace.
The include
function is used to include the URLs of another app, which is “otherapp”, and specify a namespace for these URLs. The namespace is set to “other”.
With URL namespaces, you can organize your URLs into logical groups based on functionality or app, making it easier to manage and maintain your application’s URLs. They also provide a way to avoid naming conflicts between different apps in your Django project.
Related Article: How to Use Inline If Statements for Print in Python