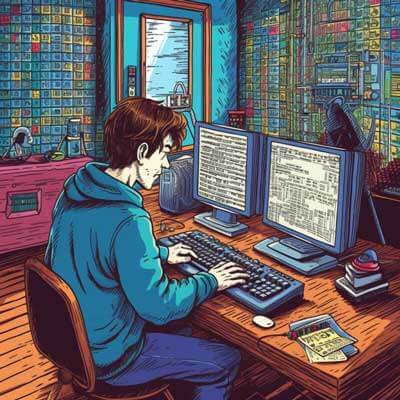
Table of Contents
There are several ways to print an exception in Python. In this guide, we will explore different approaches and best practices for printing exceptions in Python.
1. Using the traceback module
The traceback module provides functions for extracting, formatting, and printing stack traces. To print an exception using the traceback module, you can use the traceback.print_exc() function. Here's an example:
import traceback try: # code that may raise an exception ... except Exception as e: traceback.print_exc()
This will print the exception and the associated traceback to the standard error output.
Related Article: How to Manipulate Strings in Python and Check for Substrings
2. Using the sys module
The sys module provides access to some variables used or maintained by the interpreter and to functions that interact strongly with the interpreter. To print an exception using the sys module, you can use the sys.exc_info() function to get the exception information. Here's an example:
import sys try: # code that may raise an exception ... except Exception as e: exc_type, exc_value, exc_traceback = sys.exc_info() print(exc_type, exc_value)
This will print the exception type and the exception value.
Best practices for printing exceptions
When printing exceptions, it's important to provide enough information to understand and debug the issue. Here are some best practices to consider:
1. Include the exception type and the exception message: This helps to identify the type of exception that occurred and provides a brief description of the error.
2. Include the traceback: The traceback provides a detailed report of the execution path, including the line numbers where the exception occurred. This can be very helpful for identifying the root cause of the exception.
3. Handle exceptions gracefully: Instead of just printing the exception and terminating the program, consider handling the exception in a more graceful manner. This could involve logging the exception, displaying a user-friendly error message, or attempting to recover from the error.
4. Use logging: Instead of directly printing exceptions to the console, consider using a logging framework like the built-in logging module or third-party libraries like loguru or structlog. Logging provides more flexibility and control over how exceptions are handled and can be configured to write logs to different outputs.
5. Include contextual information: If possible, include additional contextual information in the exception message or the log message. This could include the values of relevant variables, the state of the program, or any other information that might be helpful for debugging.
Example: Printing an exception with traceback and logging
import logging import traceback def some_function(): try: # code that may raise an exception ... except Exception as e: logging.error("An exception occurred: %s", str(e)) logging.error(traceback.format_exc()) some_function()
In this example, the exception is logged using the logging module, which provides more control over the output and allows for easy configuration of log levels and log destinations.
Related Article: How To Get Substrings In Python: Python Substring Tutorial
Alternative approach: Raising the exception
In some cases, it may be necessary to re-raise the exception after printing it. This can be done by using the raise
statement without any arguments:
try: # code that may raise an exception ... except Exception as e: traceback.print_exc() raise
This allows the exception to be caught and handled at a higher level while still preserving the original exception and its traceback.