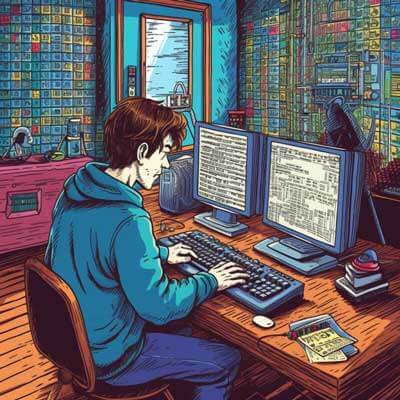
Table of Contents
Setting Up Python
Before diving into data engineering with Python, we need to set up our development environment. Python can be installed on various operating systems, including Windows, macOS, and Linux. To install Python, follow the instructions provided on the official Python website (https://www.python.org/).
Once Python is installed, we can check the version by running the following command in a terminal:
python --version
Related Article: How To Handle Ambiguous Truth Value In Python Series
Python Libraries for Data Engineering
Python offers a wide range of libraries that are specifically designed for data engineering tasks. Some of the most commonly used libraries include:
pandas
pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames, which allow us to easily handle and process large datasets. pandas also offers a variety of functions for data cleaning, merging, reshaping, and aggregating.
import pandas as pd # Load a CSV file into a pandas DataFrame df = pd.read_csv('data.csv') # Perform data cleaning and manipulation df_cleaned = df.dropna() df_transformed = df_cleaned.groupby('category').sum() # Save the transformed data to a new CSV file df_transformed.to_csv('transformed_data.csv', index=False)
numpy
numpy is a fundamental library for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently.
import numpy as np # Create a numpy array from a list arr = np.array([1, 2, 3, 4, 5]) # Perform mathematical operations on the array arr_squared = np.square(arr) arr_sum = np.sum(arr_squared) # Compute the mean and standard deviation of the array arr_mean = np.mean(arr) arr_std = np.std(arr)
Related Article: How to Use Python with Multiple Languages (Locale Guide)
Apache Spark
Apache Spark is a distributed data processing framework that provides high-level APIs for data engineering tasks. It is written in Scala but also provides a Python API called PySpark. PySpark allows us to leverage the power of Spark's distributed computing capabilities using Python.
from pyspark import SparkContext # Initialize a SparkContext sc = SparkContext(appName='DataEngineering') # Load data from a file into an RDD rdd = sc.textFile('data.txt') # Perform data transformations and actions on the RDD rdd_cleaned = rdd.filter(lambda line: 'error' not in line) rdd_transformed = rdd_cleaned.map(lambda line: line.split(',')) rdd_result = rdd_transformed.reduceByKey(lambda a, b: a + b) # Save the final result to a file rdd_result.saveAsTextFile('result.txt') # Terminate the SparkContext sc.stop()
Understanding Data Science and its Applications
Data Science is a multidisciplinary field that combines various techniques, tools, and methodologies to extract insights and knowledge from data. It involves a combination of programming, statistics, and domain knowledge to solve complex problems and make data-driven decisions.
In recent years, data science has gained significant popularity due to the exponential growth of data and the increasing need for businesses to leverage this data to gain a competitive advantage. Data scientists play a crucial role in helping organizations make sense of their data by using advanced analytical techniques and machine learning algorithms.
Data Science Applications:
Data science has a wide range of applications across different industries. Here are some common areas where data science is applied:
1. Business Analytics: Data science helps businesses analyze their data to gain insights and make informed decisions. It involves techniques such as data visualization, exploratory data analysis, and predictive modeling to understand customer behavior, optimize marketing campaigns, and improve operational efficiency.
2. Healthcare: Data science is revolutionizing the healthcare industry by enabling personalized medicine, disease prediction, and early detection. It helps healthcare providers analyze large volumes of patient data to improve diagnostics, treatment plans, and patient outcomes.
3. Finance: Data science is extensively used in the finance industry for fraud detection, risk assessment, and algorithmic trading. It helps financial institutions analyze vast amounts of financial data to identify patterns, detect anomalies, and make data-driven investment decisions.
4. Social Media and Advertising: Data science plays a crucial role in social media platforms and digital advertising. It helps companies analyze user behavior, sentiment analysis, and perform targeted advertising campaigns to maximize user engagement and conversion rates.
Python for Data Science:
Python has become one of the most popular programming languages for data science due to its simplicity, extensive libraries, and strong community support. Python provides powerful libraries such as NumPy, Pandas, and Matplotlib, which make data manipulation, analysis, and visualization tasks much easier.
Here is an example of how Python can be used for data analysis:
import pandas as pd # Load data from a CSV file data = pd.read_csv('data.csv') # Perform basic data exploration print(data.head()) # Perform data analysis and visualization data['age'].hist()
In the above example, we use the Pandas library to read a CSV file and load it into a DataFrame. We then perform basic data exploration by printing the first few rows of the data. Finally, we use the Matplotlib library to plot a histogram of the 'age' column.
In addition to data analysis, Python also provides powerful libraries such as TensorFlow and Scikit-learn for machine learning and deep learning tasks. These libraries enable data scientists to build and deploy complex machine learning models for tasks such as image recognition, natural language processing, and recommendation systems.
In conclusion, data science is a rapidly growing field with numerous applications across various industries. Python, with its vast array of libraries and tools, has become the go-to language for data scientists. By leveraging Python's capabilities, data engineers and data scientists can effectively analyze, manipulate, and visualize data to extract valuable insights and drive informed decision-making.
Python Basics for Data Engineering and Data Science
Python is a versatile programming language that is widely used in the fields of data engineering and data science. Its simplicity and readability make it an ideal language for these domains. In this chapter, we will cover some Python basics that are essential for data engineering and data science tasks.
Variables and Data Types
In Python, variables are used to store values. Unlike other programming languages, Python does not require explicit declaration of variables. You can simply assign a value to a variable and Python will infer its data type.
# Example of assigning a value to a variable x = 10 y = "Hello, World!"
Python supports several built-in data types, such as integers, floating-point numbers, strings, lists, tuples, dictionaries, and sets. It also allows you to create your own data types using classes.
Related Article: Python Ceiling Function Explained
Control Flow Statements
Control flow statements are used to control the execution of code based on certain conditions. Python provides several control flow statements, including if-else statements, for loops, while loops, and try-except statements.
# Example of an if-else statement x = 10 if x > 5: print("x is greater than 5") else: print("x is less than or equal to 5") # Example of a for loop for i in range(5): print(i) # Example of a while loop x = 0 while x < 5: print(x) x += 1 # Example of a try-except statement try: x = 10 / 0 except ZeroDivisionError: print("Error: Division by zero")
Functions
Functions are reusable blocks of code that perform specific tasks. They help in organizing code and making it more modular. In Python, you can define functions using the def
keyword.
# Example of defining a function def greet(name): print("Hello, " + name + "!") # Example of calling a function greet("Alice")
Python also allows you to define functions with default arguments and variable-length arguments, providing flexibility and reusability.
Modules and Packages
Python provides a rich ecosystem of modules and packages that extend its functionality. Modules are simply Python files that contain code, while packages are directories that contain multiple modules. You can import modules and packages using the import
keyword.
# Example of importing a module import math # Example of using a module print(math.pi) # Example of importing a module with an alias import numpy as np # Example of using a module with an alias arr = np.array([1, 2, 3])
Python's extensive library of modules and packages is one of its biggest strengths for data engineering and data science tasks.
File Handling
Python provides built-in functions for reading from and writing to files. You can open a file using the open()
function, specify the mode (read, write, append, etc.), and perform operations on the file using methods like read()
, write()
, and close()
.
# Example of reading from a file with open("data.txt", "r") as file: data = file.read() print(data) # Example of writing to a file with open("output.txt", "w") as file: file.write("Hello, World!")
Proper handling of files is crucial in data engineering and data science tasks, as data is often read from and written to external sources.
Python provides many more features and functionalities that are useful for data engineering and data science tasks. In this chapter, we have covered some of the basics, including variables and data types, control flow statements, functions, modules and packages, and file handling. Familiarizing yourself with these concepts will lay a solid foundation for your journey into the world of data engineering and data science with Python.
Related Article: How to Remove a Key from a Python Dictionary
Loading and Reading Data
Python offers several libraries to load and read data from different sources. One of the most widely used libraries is pandas, which provides data structures and functions for efficient data manipulation. Let's take a look at how to load a CSV file using pandas:
import pandas as pd # Load CSV file data = pd.read_csv('data.csv')
The read_csv()
function reads the contents of a CSV file and stores it in a pandas DataFrame object. You can then perform various operations on the data, such as filtering, sorting, and aggregating.
Writing Data
Once you have processed and transformed your data, you may need to save it to a file or a database. Python offers different methods for writing data, depending on the desired output format.
If you want to write data to a CSV file, you can use the to_csv()
function in pandas:
# Write DataFrame to a CSV file data.to_csv('output.csv', index=False)
The to_csv()
function writes the contents of a DataFrame to a CSV file. The index=False
argument ensures that the row index is not included in the output.
Cleaning and Transforming Data
Data cleaning and transformation are essential steps in the data engineering and data science process. Python provides various libraries and tools to clean and transform data efficiently.
For example, the pandas library offers functions to handle missing values, remove duplicates, and perform data transformations. Let's see an example of how to remove duplicates from a DataFrame:
# Remove duplicates from DataFrame data = data.drop_duplicates()
The drop_duplicates()
function removes duplicate rows from a DataFrame, keeping only the first occurrence of each unique row.
Aggregating Data
Aggregating data involves combining multiple rows into a single row, typically by performing calculations such as sum, count, average, or max/min.
Pandas provides the groupby()
function to group data based on one or more columns and apply aggregation functions. Here's an example of how to calculate the average value of a column based on another column's values:
# Group data by 'category' and calculate average 'price' average_price = data.groupby('category')['price'].mean()
The groupby()
function groups the data by the 'category' column and then calculates the mean of the 'price' column for each group. The result is a new DataFrame with the average price for each category.
Related Article: How To Find Index Of Item In Python List
Working with Dates and Time
Data often contains date and time information, and Python provides powerful libraries to handle such data.
The datetime module in Python's standard library offers classes and functions to manipulate dates and times. For example, you can convert a string representation of a date to a datetime object using the strptime()
function:
from datetime import datetime # Convert string to datetime object date_string = '2022-01-01' date_object = datetime.strptime(date_string, '%Y-%m-%d')
The strptime()
function parses the date string using the specified format ('%Y-%m-%d') and returns a datetime object.
Loading and Inspecting Data
Before diving into EDA, we first need to load the data into our Python environment. The most common data formats include CSV, Excel, and databases. Let's take a look at how to load a CSV file using the pandas
library.
import pandas as pd # Load data from a CSV file data = pd.read_csv('data.csv') # Display the first few rows data.head()
Once the data is loaded, it's essential to inspect its structure and get a sense of its contents. We can use various pandas functions to understand the data better, such as info()
, describe()
, and shape
.
# Get general information about the data data.info() # Summary statistics of the numerical columns data.describe() # Dimensions of the data (rows, columns) data.shape
Handling Missing Values
Missing values are a common occurrence in real-world datasets and can affect the accuracy of our analysis. Python provides several approaches to handle missing values, such as dropping rows or columns, imputing values, or using advanced techniques like interpolation.
# Identify columns with missing values data.isnull().sum() # Drop rows with missing values data.dropna(inplace=True) # Impute missing values with mean data.fillna(data.mean(), inplace=True)
Visualizing Data
Visualizations are powerful tools for understanding the data and gaining insights. Python offers several libraries for creating interactive and static visualizations, such as matplotlib
, seaborn
, and plotly
. Let's look at a simple example using matplotlib
.
import matplotlib.pyplot as plt # Plot a histogram of a numerical column plt.hist(data['age']) plt.xlabel('Age') plt.ylabel('Frequency') plt.title('Distribution of Age') plt.show()
We can create various types of visualizations, including scatter plots, bar charts, box plots, and heatmaps, to explore relationships, distributions, and outliers in the data.
Related Article: How to Remove an Element from a List by Index in Python
Feature Engineering
Feature engineering involves creating new features from existing ones to enhance the predictive power of our models. Python provides a range of techniques for feature engineering, such as one-hot encoding, scaling, and creating interaction variables.
# Create dummy variables for categorical columns data_encoded = pd.get_dummies(data, columns=['gender']) # Scale numerical columns using min-max scaling from sklearn.preprocessing import MinMaxScaler scaler = MinMaxScaler() data_scaled = scaler.fit_transform(data[['age', 'income']])
Feature engineering plays a crucial role in improving the performance of machine learning models by providing them with more meaningful and informative input features.
Statistical Analysis
Python offers a wide range of statistical libraries that enable us to conduct various statistical analyses on our data. We can perform hypothesis testing, calculate correlation coefficients, and fit statistical models.
from scipy.stats import ttest_ind from statsmodels.formula.api import ols # Perform a t-test between two groups group1 = data[data['group'] == 'A']['value'] group2 = data[data['group'] == 'B']['value'] t_stat, p_value = ttest_ind(group1, group2) # Fit a linear regression model model = ols('y ~ x', data).fit() model.summary()
Statistical analysis helps us understand the relationships between variables, make predictions, and draw inferences from the data.
Data Manipulation Techniques in Python
Data manipulation is a crucial step in data engineering and data science tasks. Python provides a rich set of libraries and tools that make it easy to manipulate and transform data efficiently. In this chapter, we will explore some commonly used data manipulation techniques in Python.
Loading and Reading Data
Before we can manipulate data, we need to load it into our Python environment. Python offers several libraries for reading and loading different types of data, such as CSV, Excel, JSON, and databases. Let's take a look at how to read a CSV file using the pandas library:
import pandas as pd # Read a CSV file data = pd.read_csv('data.csv') # Display the first few rows of the data print(data.head())
In the above code snippet, we import the pandas library and use the read_csv
function to read a CSV file named 'data.csv'. We then display the first few rows of the data using the head
function.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Data Cleaning
Data often contains missing values, outliers, or inconsistencies that need to be addressed before further analysis. Python provides various techniques to clean and preprocess data. Let's consider an example where we want to remove missing values from a pandas DataFrame:
import pandas as pd # Read a CSV file data = pd.read_csv('data.csv') # Remove rows with missing values data = data.dropna() # Display the cleaned data print(data.head())
In the code snippet above, we load a CSV file into a DataFrame and then use the dropna
function to remove rows with missing values. The resulting DataFrame contains only rows without missing values.
Data Transformation
Data transformation involves converting data into a different format or structure. Python offers powerful tools for data transformation, such as applying functions to data, merging datasets, and reshaping data. Let's see an example of how to calculate the average of a column in a pandas DataFrame:
import pandas as pd # Read a CSV file data = pd.read_csv('data.csv') # Calculate the average of a column average = data['column_name'].mean() # Display the average print(average)
In the code snippet above, we load a CSV file into a DataFrame and then use the mean
function to calculate the average of a specific column named 'column_name'. The result is stored in the variable average
and then printed.
Data Aggregation
Data aggregation involves combining multiple data points into a single value, usually by applying a specific function or operation. Python provides various techniques for data aggregation, such as grouping data, applying functions to groups, and summarizing data. Let's consider an example where we want to calculate the total sales for each product in a pandas DataFrame:
import pandas as pd # Read a CSV file data = pd.read_csv('data.csv') # Group data by product and calculate total sales total_sales = data.groupby('product')['sales'].sum() # Display the total sales print(total_sales)
In the above code snippet, we load a CSV file into a DataFrame and then use the groupby
function to group the data by the 'product' column. We then apply the sum
function to the 'sales' column within each group to calculate the total sales for each product. The result is stored in the variable total_sales
and then printed.
Data Visualization
Data visualization is essential for exploring and understanding data. Python offers various libraries for creating visualizations, such as Matplotlib, Seaborn, and Plotly. Let's see an example of how to create a bar chart using the Matplotlib library:
import pandas as pd import matplotlib.pyplot as plt # Read a CSV file data = pd.read_csv('data.csv') # Create a bar chart plt.bar(data['category'], data['sales']) # Add labels and title plt.xlabel('Category') plt.ylabel('Sales') plt.title('Sales by Category') # Display the chart plt.show()
In the code snippet above, we load a CSV file into a DataFrame and then use the bar
function from Matplotlib to create a bar chart. We add labels and a title to the chart using the xlabel
, ylabel
, and title
functions. Finally, we display the chart using the show
function.
These are just a few examples of the data manipulation techniques available in Python. Python's versatility and extensive library ecosystem make it a powerful tool for data engineering and data science tasks. By leveraging these techniques, you can efficiently manipulate and transform data to gain valuable insights and make informed decisions.
Related Article: How to Sort a Pandas Dataframe by One Column in Python
Data Visualization with Python
Data visualization is a crucial aspect of data engineering and data science. It allows us to understand and communicate data insights effectively. Python provides several powerful libraries for creating visualizations, making it a popular choice among data professionals.
Matplotlib
One of the most widely used libraries for data visualization in Python is Matplotlib. It provides a flexible and comprehensive set of tools for creating various types of plots, such as line plots, scatter plots, bar plots, and histograms.
To use Matplotlib, we first need to import the library:
import matplotlib.pyplot as plt
Let's start with a simple example of creating a line plot:
# Create some data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Plot the data plt.plot(x, y) # Add labels and title plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Line Plot') # Display the plot plt.show()
This code will generate a line plot with the given data points. We can customize the plot by adding labels, titles, legends, and changing the style of the plot.
Seaborn
Seaborn is another popular data visualization library in Python. It is built on top of Matplotlib and provides a higher-level interface for creating attractive and informative statistical graphics.
To use Seaborn, we first need to import the library:
import seaborn as sns
Let's create a scatter plot using the built-in tips dataset from Seaborn:
# Load the tips dataset tips = sns.load_dataset('tips') # Create a scatter plot sns.scatterplot(x='total_bill', y='tip', data=tips) # Add labels and title plt.xlabel('Total Bill') plt.ylabel('Tip') plt.title('Scatter Plot') # Display the plot plt.show()
This code will generate a scatter plot using the 'total_bill' and 'tip' columns from the tips dataset. Seaborn provides various functions for different types of plots, making it easy to create visually appealing visualizations.
Plotly
Plotly is a library that focuses on interactive and web-based visualizations. It provides a wide range of visualization types and allows for easy sharing of visualizations online.
To use Plotly, we first need to install the library:
!pip install plotly
Let's create an interactive bar plot using Plotly:
import plotly.express as px # Load the iris dataset iris = px.data.iris() # Create a bar plot fig = px.bar(iris, x='species', y='sepal_width', color='species', barmode='group') # Display the plot fig.show()
This code will generate an interactive bar plot using the 'species' and 'sepal_width' columns from the iris dataset. Plotly allows us to zoom, pan, and hover over the data points to explore the visualization in detail.
Related Article: How to Use Redis with Django Applications
Introduction to Machine Learning with Python
Machine Learning is a branch of Artificial Intelligence that focuses on creating algorithms and models that enable computers to learn and make predictions or decisions without being explicitly programmed. Python is a popular programming language for Machine Learning due to its simplicity, flexibility, and extensive range of libraries and frameworks.
In this chapter, we will introduce you to the basics of Machine Learning with Python. We will cover the two main types of Machine Learning: Supervised Learning and Unsupervised Learning, as well as provide an overview of the steps involved in building a machine learning model.
Supervised Learning
Supervised Learning is a type of Machine Learning where the input data is labeled with the correct output. The goal is to learn a mapping function that can predict the output for new, unseen inputs. There are two main types of Supervised Learning: Classification and Regression.
In Classification, the output variable is a category or class label. The machine learning model is trained on labeled data, and the goal is to predict the class label for new, unseen instances. Popular algorithms for classification include Decision Trees, Random Forests, and Support Vector Machines (SVM).
Regression, on the other hand, deals with predicting a continuous output variable. The model learns the relationship between the input variables and the output variable and can be used to make predictions on new data. Linear Regression and Gradient Boosting are common algorithms used for regression tasks.
Let's take a look at an example of supervised learning in Python using the Scikit-learn library. Suppose we have a dataset of housing prices with features such as the number of rooms and the age of the house. We can train a model to predict the price of a house given its features.
import pandas as pd from sklearn.linear_model import LinearRegression # Load the dataset data = pd.read_csv('housing.csv') # Split the data into input features and target variable X = data[['rooms', 'age']] y = data['price'] # Create a linear regression model model = LinearRegression() # Train the model model.fit(X, y) # Make predictions new_data = pd.DataFrame({'rooms': [3], 'age': [10]}) predicted_price = model.predict(new_data) print(predicted_price)
Unsupervised Learning
Unsupervised Learning is a type of Machine Learning where the input data is not labeled or categorized. The goal is to find patterns or structure in the data without any specific guidance. Clustering and Dimensionality Reduction are common tasks in Unsupervised Learning.
Clustering algorithms group similar instances together based on their similarity or distance measures. K-means clustering and Hierarchical clustering are popular algorithms for this task. Dimensionality Reduction, on the other hand, aims to reduce the number of input features while preserving the important information. Principal Component Analysis (PCA) and t-SNE are commonly used for dimensionality reduction.
Let's explore an example of unsupervised learning using the K-means clustering algorithm in Python with the Scikit-learn library. Suppose we have a dataset of customer information, including their age and income. We can use K-means clustering to group similar customers together based on these features.
import pandas as pd from sklearn.cluster import KMeans # Load the dataset data = pd.read_csv('customer_info.csv') # Select the features for clustering X = data[['age', 'income']] # Create a K-means clustering model model = KMeans(n_clusters=3) # Fit the model to the data model.fit(X) # Assign cluster labels to each data point labels = model.labels_ # Print the cluster labels print(labels)
Machine Learning with Python offers a wide range of possibilities for solving complex problems and making data-driven decisions. It is a powerful tool for Data Engineers and Data Scientists to extract valuable insights from data. In the next chapters, we will delve deeper into different aspects of Machine Learning and explore more advanced topics and techniques.
Supervised Learning Algorithms in Python
Supervised learning is a popular approach in machine learning where the algorithm learns from labeled data to make predictions or decisions. In this chapter, we will explore some common supervised learning algorithms and how to implement them using Python.
Related Article: How to Use Class And Instance Variables in Python
1. Linear Regression
Linear regression is a simple yet powerful algorithm used for predicting a continuous target variable based on one or more input features. It assumes a linear relationship between the input variables and the target variable. The goal is to find the best-fit line that minimizes the sum of squared differences between the predicted and actual values.
Here's an example of implementing linear regression in Python using the scikit-learn library:
from sklearn.linear_model import LinearRegression # Create a linear regression model model = LinearRegression() # Fit the model to the training data model.fit(X_train, y_train) # Make predictions on the test data predictions = model.predict(X_test)
2. Logistic Regression
Logistic regression is a classification algorithm used to predict the probability of a binary or categorical target variable. It uses the logistic function to model the relationship between the input variables and the probability of a certain outcome.
Here's an example of implementing logistic regression in Python using scikit-learn:
from sklearn.linear_model import LogisticRegression # Create a logistic regression model model = LogisticRegression() # Fit the model to the training data model.fit(X_train, y_train) # Make predictions on the test data predictions = model.predict(X_test)
3. Decision Trees
Decision trees are versatile algorithms used for both classification and regression tasks. They create a flowchart-like model where each internal node represents a feature, each branch represents a decision rule, and each leaf node represents an outcome. Decision trees are easy to interpret and can handle both numerical and categorical data.
Here's an example of implementing a decision tree classifier in Python using scikit-learn:
from sklearn.tree import DecisionTreeClassifier # Create a decision tree classifier model = DecisionTreeClassifier() # Fit the model to the training data model.fit(X_train, y_train) # Make predictions on the test data predictions = model.predict(X_test)
4. Random Forests
Random forests are an ensemble learning method that combines multiple decision trees to make predictions. Each tree in the forest is trained on a random subset of the training data and a random subset of the input features. The final prediction is made by averaging or voting the predictions of each individual tree.
Here's an example of implementing a random forest classifier in Python using scikit-learn:
from sklearn.ensemble import RandomForestClassifier # Create a random forest classifier model = RandomForestClassifier() # Fit the model to the training data model.fit(X_train, y_train) # Make predictions on the test data predictions = model.predict(X_test)
Related Article: Intro to Django External Tools: Monitoring, ORMs & More
5. Support Vector Machines
Support Vector Machines (SVM) are a powerful algorithm used for both classification and regression tasks. SVM finds the best hyperplane that separates the data into different classes while maximizing the margin between the classes. It can handle linear and non-linear decision boundaries by using different kernel functions.
Here's an example of implementing a support vector machine classifier in Python using scikit-learn:
from sklearn.svm import SVC # Create a support vector machine classifier model = SVC() # Fit the model to the training data model.fit(X_train, y_train) # Make predictions on the test data predictions = model.predict(X_test)
These are just a few examples of supervised learning algorithms that you can implement using Python. Each algorithm has its strengths and weaknesses, and the choice of algorithm depends on the specific problem and data at hand. Experiment with different algorithms and parameters to find the best model for your data.
Unsupervised Learning Algorithms in Python
Unsupervised learning is a branch of machine learning where the goal is to find hidden patterns or structures in a dataset without any prior knowledge or labeled data. It is an essential tool in data engineering and data science, as it allows us to gain insights from unstructured or unlabeled data.
In this chapter, we will explore some popular unsupervised learning algorithms in Python and see how they can be applied to various real-world scenarios. We will cover the following algorithms:
1. K-Means Clustering: K-means is a widely used clustering algorithm that partitions data into K clusters based on similarity. It is particularly useful for customer segmentation, anomaly detection, and image compression. Here's an example of how to use K-means clustering in Python:
from sklearn.cluster import KMeans # Load data X = ... # Create K-means model kmeans = KMeans(n_clusters=3) # Fit the model to the data kmeans.fit(X) # Predict the cluster labels for new data points labels = kmeans.predict(X)
2. Hierarchical Clustering: Hierarchical clustering builds a hierarchy of clusters by either agglomerative (bottom-up) or divisive (top-down) approaches. It is suitable for analyzing gene expression data, social network analysis, and document clustering. Here's an example of how to use hierarchical clustering in Python:
from sklearn.cluster import AgglomerativeClustering # Load data X = ... # Create hierarchical clustering model hc = AgglomerativeClustering(n_clusters=3) # Fit the model to the data hc.fit(X) # Predict the cluster labels for new data points labels = hc.labels_
3. Principal Component Analysis (PCA): PCA is a dimensionality reduction technique that identifies the most important features or components in a dataset. It is commonly used for visualizations, feature extraction, and noise reduction. Here's an example of how to use PCA in Python:
from sklearn.decomposition import PCA # Load data X = ... # Create PCA model pca = PCA(n_components=2) # Fit the model to the data pca.fit(X) # Transform the data to the new coordinate system X_transformed = pca.transform(X)
4. Gaussian Mixture Models (GMM): GMM is a probabilistic model that represents the distribution of data as a mixture of Gaussian distributions. It is often used for density estimation, image segmentation, and anomaly detection. Here's an example of how to use GMM in Python:
from sklearn.mixture import GaussianMixture # Load data X = ... # Create GMM model gmm = GaussianMixture(n_components=3) # Fit the model to the data gmm.fit(X) # Predict the cluster labels for new data points labels = gmm.predict(X)
These are just a few examples of unsupervised learning algorithms in Python. Each algorithm has its own strengths and weaknesses, and the choice of algorithm depends on the specific problem and dataset at hand. By understanding and applying these algorithms, you will be better equipped to handle unsupervised learning tasks in your data engineering and data science projects.
Evaluation and Validation Techniques in Python
When working with data engineering and data science projects, it is crucial to evaluate and validate the models and algorithms being used. This helps ensure that the results obtained are accurate and reliable. In this chapter, we will explore various evaluation and validation techniques that can be implemented using Python.
Evaluation Metrics
Evaluation metrics are used to measure the performance of a model or algorithm. Different metrics are used depending on the nature of the problem being solved. Let's take a look at some commonly used evaluation metrics.
1. Accuracy: It measures the proportion of correct predictions among all the predictions made by a model.
from sklearn.metrics import accuracy_score y_true = [1, 0, 1, 0, 1] y_pred = [1, 1, 0, 0, 1] accuracy = accuracy_score(y_true, y_pred) print(f"Accuracy: {accuracy}")
2. Precision, Recall, and F1-Score: These metrics are used to evaluate the performance of binary classification models.
from sklearn.metrics import precision_score, recall_score, f1_score y_true = [1, 0, 1, 0, 1] y_pred = [1, 1, 0, 0, 1] precision = precision_score(y_true, y_pred) recall = recall_score(y_true, y_pred) f1 = f1_score(y_true, y_pred) print(f"Precision: {precision}") print(f"Recall: {recall}") print(f"F1-Score: {f1}")
3. Mean Squared Error (MSE): It measures the average squared difference between the predicted and actual values in regression problems.
from sklearn.metrics import mean_squared_error y_true = [1, 2, 3, 4, 5] y_pred = [1.5, 2.2, 2.8, 3.9, 4.5] mse = mean_squared_error(y_true, y_pred) print(f"Mean Squared Error: {mse}")
Related Article: How to Force Pip to Reinstall the Current Version in Python
Validation Techniques
Validation techniques are used to ensure that the model's performance is not biased or overfitted to the training data. Here are a few commonly used validation techniques.
1. Train-Test Split: In this technique, the dataset is divided into two parts: a training set and a testing set. The model is trained on the training set and evaluated on the testing set.
from sklearn.model_selection import train_test_split X = [[1, 2], [3, 4], [5, 6], [7, 8]] y = [1, 0, 1, 0] X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
2. Cross-Validation: Cross-validation is a technique where the dataset is divided into multiple subsets or folds. The model is trained and evaluated on different combinations of these folds.
from sklearn.model_selection import cross_val_score from sklearn.linear_model import LogisticRegression X = [[1, 2], [3, 4], [5, 6], [7, 8]] y = [1, 0, 1, 0] model = LogisticRegression() scores = cross_val_score(model, X, y, cv=5) print(f"Cross-Validation Scores: {scores}")
Feature Selection
Feature selection is the process of selecting a subset of the most relevant features from the dataset. It helps to improve model performance by reducing overfitting and reducing the complexity of the model.
There are several techniques for feature selection, including:
- Filter methods: These methods use statistical measures to rank features based on their relevance to the target variable. Examples include correlation coefficient and chi-square test.
- Wrapper methods: These methods select features by training and evaluating different subsets of features using a specific machine learning algorithm. Examples include recursive feature elimination and forward selection.
- Embedded methods: These methods incorporate feature selection as part of the model training process. Examples include L1 regularization (Lasso) and decision tree-based feature importance.
Let's take a look at an example of using the chi-square test for feature selection:
import pandas as pd from sklearn.feature_selection import SelectKBest from sklearn.feature_selection import chi2 # Load the dataset data = pd.read_csv('data.csv') # Separate the features and target variable X = data.drop('target', axis=1) y = data['target'] # Apply feature selection selector = SelectKBest(score_func=chi2, k=3) X_new = selector.fit_transform(X, y) # Get the selected features selected_features = X.columns[selector.get_support()]
Feature Extraction
Feature extraction involves creating new features from existing ones to capture more meaningful information. It can be done through techniques such as dimensionality reduction, creating interaction terms, and transforming variables.
One popular technique for feature extraction is Principal Component Analysis (PCA), which reduces the dimensionality of the dataset while preserving the most important information. Here's an example of using PCA for feature extraction:
from sklearn.decomposition import PCA # Load the dataset data = pd.read_csv('data.csv') # Separate the features and target variable X = data.drop('target', axis=1) y = data['target'] # Apply feature extraction pca = PCA(n_components=2) X_new = pca.fit_transform(X) # Plot the transformed data plt.scatter(X_new[:, 0], X_new[:, 1], c=y) plt.xlabel('Principal Component 1') plt.ylabel('Principal Component 2') plt.show()
Feature Transformation
Feature transformation involves changing the scale or distribution of features to improve model performance. It can be done through techniques such as normalization, standardization, and logarithmic transformation.
One common technique for feature transformation is Min-Max scaling, which scales the features to a specific range (e.g., 0 to 1). Here's an example of using Min-Max scaling for feature transformation:
from sklearn.preprocessing import MinMaxScaler # Load the dataset data = pd.read_csv('data.csv') # Separate the features and target variable X = data.drop('target', axis=1) y = data['target'] # Apply feature transformation scaler = MinMaxScaler() X_new = scaler.fit_transform(X)
Related Article: How to Use Assert in Python: Explained
Handling Categorical Variables
Categorical variables are non-numeric variables that represent categories or groups. They need to be properly encoded to be used in machine learning models. There are several techniques for encoding categorical variables, including one-hot encoding, label encoding, and target encoding.
One-hot encoding creates binary variables for each category of a categorical feature. Here's an example of using one-hot encoding for handling categorical variables:
from sklearn.preprocessing import OneHotEncoder # Load the dataset data = pd.read_csv('data.csv') # Separate the features and target variable X = data.drop('target', axis=1) y = data['target'] # Apply one-hot encoding encoder = OneHotEncoder() X_encoded = encoder.fit_transform(X)
The Pandas Library
Pandas is a popular library in the Python ecosystem for data manipulation and analysis. It provides a DataFrame object, which is a two-dimensional table-like data structure, similar to a spreadsheet or SQL table. Pandas allows us to load, transform, and analyze large datasets efficiently.
To demonstrate the power of Pandas for big data processing, let's consider a large CSV file containing millions of records. We can load this file into a Pandas DataFrame using the read_csv
function:
import pandas as pd df = pd.read_csv('large_dataset.csv')
Once the data is loaded into a DataFrame, we can perform various operations such as filtering, aggregating, and joining data. Pandas provides a wide range of functions and methods for these operations. Here's an example of filtering data based on a condition:
filtered_df = df[df['column_name'] > 100]
Pandas also supports parallel processing using the Dask
library, which allows us to scale our data processing capabilities even further.
The PySpark Library
PySpark is the Python API for Apache Spark, a popular big data processing framework. Spark provides a distributed computing environment for processing large datasets across a cluster of machines. PySpark allows us to leverage the power of Spark using Python.
To get started with PySpark, we first need to install it and set up a SparkContext:
from pyspark.sql import SparkSession spark = SparkSession.builder .appName("BigDataProcessing") .getOrCreate()
Once we have a SparkContext, we can load data into a Spark DataFrame and perform distributed data processing operations. Here's an example of loading a CSV file into a Spark DataFrame:
df = spark.read.csv('large_dataset.csv', header=True)
Spark provides a rich set of functions and operations for data processing, including filtering, aggregating, and joining data. These operations can be performed on large datasets in parallel across the cluster.
The Dask Library
Dask is a flexible library in the Python ecosystem for parallel computing. It provides a way to scale our computations across multiple CPUs or even multiple machines. Dask is particularly useful for processing big data in a distributed manner.
To get started with Dask, we first need to install it:
pip install dask
Once Dask is installed, we can create a Dask DataFrame, which is a parallel and distributed version of the Pandas DataFrame. Dask DataFrame provides a familiar interface for data manipulation and analysis, similar to Pandas.
import dask.dataframe as dd df = dd.read_csv('large_dataset.csv')
Dask operations are lazy, meaning they are not executed immediately. Instead, they build up a computational graph, which is then executed when needed. This allows Dask to optimize the execution and memory usage for big data processing.
Related Article: How To Check If Key Exists In Python Dictionary
Example 1: Sales Forecasting
One common use case for predictive analytics is sales forecasting. By analyzing historical sales data, we can build a predictive model to estimate future sales. Let's take a look at an example using Python.
import pandas as pd from sklearn.linear_model import LinearRegression # Load the sales data sales_data = pd.read_csv('sales.csv') # Split the data into input (X) and output (y) variables X = sales_data[['Year', 'Month']] y = sales_data['Sales'] # Train the model model = LinearRegression() model.fit(X, y) # Predict future sales future_sales = model.predict([[2022, 1], [2022, 2], [2022, 3]]) print(future_sales)
In this example, we load the sales data from a CSV file and split it into input (X) and output (y) variables. We then train a linear regression model using the LinearRegression
class from the sklearn.linear_model
module. Finally, we use the trained model to predict future sales for the first three months of 2022.
Example 2: Churn Prediction
Churn prediction is another important application of predictive analytics. It involves predicting the likelihood of a customer leaving a service or canceling a subscription. Let's see how we can use Python to build a churn prediction model.
import pandas as pd from sklearn.ensemble import RandomForestClassifier from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score # Load the churn data churn_data = pd.read_csv('churn.csv') # Split the data into input (X) and output (y) variables X = churn_data.drop('Churn', axis=1) y = churn_data['Churn'] # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train the model model = RandomForestClassifier() model.fit(X_train, y_train) # Predict churn for the test set y_pred = model.predict(X_test) # Evaluate the model accuracy = accuracy_score(y_test, y_pred) print('Accuracy:', accuracy)
In this example, we load the churn data from a CSV file and split it into input (X) and output (y) variables. We then split the data into training and testing sets using the train_test_split
function from the sklearn.model_selection
module. Next, we train a random forest classifier using the RandomForestClassifier
class from the sklearn.ensemble
module. Finally, we use the trained model to predict churn for the test set and evaluate its accuracy.
Example 3: Stock Price Prediction
Predicting stock prices is a challenging task but can be done using predictive analytics techniques. Let's see how Python can be used to build a stock price prediction model.
import pandas as pd from sklearn.svm import SVR from sklearn.preprocessing import MinMaxScaler import matplotlib.pyplot as plt # Load the stock price data stock_data = pd.read_csv('stock_prices.csv') # Normalize the data scaler = MinMaxScaler() stock_data['NormalizedPrice'] = scaler.fit_transform(stock_data['Price'].values.reshape(-1, 1)) # Split the data into input (X) and output (y) variables X = stock_data[['Day', 'Month', 'Year']] y = stock_data['NormalizedPrice'] # Train the model model = SVR(kernel='rbf', C=100, gamma=0.1, epsilon=0.1) model.fit(X, y) # Predict stock prices predicted_prices = model.predict([[30, 12, 2022], [31, 12, 2022], [1, 1, 2023]]) # Plot the predicted prices plt.plot(predicted_prices) plt.xlabel('Days') plt.ylabel('Normalized Price') plt.show()
In this example, we load the stock price data from a CSV file and normalize it using the MinMaxScaler
class from the sklearn.preprocessing
module. We then split the data into input (X) and output (y) variables. Next, we train a support vector regression model using the SVR
class from the sklearn.svm
module. Finally, we use the trained model to predict stock prices for the last two days of 2022 and the first day of 2023, and plot the predicted prices using matplotlib
.
Content-Based Recommendation
Content-based recommendation is a popular approach that recommends items to users based on their preferences and similarities to other items. It leverages the characteristics or features of items to make recommendations. Let's take a look at an example of building a content-based movie recommendation system.
import pandas as pd from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.metrics.pairwise import cosine_similarity # Load the movie dataset movies = pd.read_csv('movies.csv') # Preprocess the movie genres movies['genres'] = movies['genres'].str.replace('|', ' ') # Create a TF-IDF vectorizer vectorizer = TfidfVectorizer() # Compute the TF-IDF matrix tfidf_matrix = vectorizer.fit_transform(movies['genres']) # Compute the cosine similarity matrix cosine_sim = cosine_similarity(tfidf_matrix, tfidf_matrix) # Function to recommend movies based on similarity def recommend_movies(movie_title, cosine_sim=cosine_sim, movies=movies): # Get the index of the movie title idx = movies[movies['title'] == movie_title].index[0] # Get the pairwise similarity scores sim_scores = list(enumerate(cosine_sim[idx])) # Sort the movies based on similarity scores sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True) # Get the top 10 similar movies sim_scores = sim_scores[1:11] # Get the movie indices movie_indices = [i[0] for i in sim_scores] # Return the top 10 similar movies return movies['title'].iloc[movie_indices] # Example usage recommend_movies('Toy Story')
In this example, we load a movie dataset and preprocess the genres column. Next, we create a TF-IDF vectorizer to convert the text data into numerical features. We then compute the TF-IDF matrix and the cosine similarity matrix. Finally, we define a function to recommend movies based on similarity scores and use it to recommend movies similar to "Toy Story".
Related Article: Python Math Operations: Floor, Ceil, and More
Collaborative Filtering
Collaborative filtering is another popular approach used in recommender systems. It recommends items based on the preferences of similar users or the similarities between items. Let's explore an example of building a collaborative filtering recommendation system.
import pandas as pd from surprise import Dataset, Reader, KNNBasic # Load the movie ratings dataset ratings = pd.read_csv('ratings.csv') # Define the rating scale reader = Reader(rating_scale=(1, 5)) # Load the dataset for Surprise data = Dataset.load_from_df(ratings[['userId', 'movieId', 'rating']], reader) # Build the item-based collaborative filtering model model = KNNBasic(sim_options={'user_based': False}) # Train the model trainset = data.build_full_trainset() model.fit(trainset) # Function to recommend movies based on collaborative filtering def recommend_movies(userId, model=model, ratings=ratings): # Get the list of all movie ids movieIds = ratings['movieId'].unique() # Get the predicted ratings for the user predicted_ratings = [] for movieId in movieIds: predicted_rating = model.predict(userId, movieId).est predicted_ratings.append((movieId, predicted_rating)) # Sort the movies based on predicted ratings predicted_ratings = sorted(predicted_ratings, key=lambda x: x[1], reverse=True) # Get the top 10 recommended movies recommended_movies = predicted_ratings[:10] # Return the top 10 recommended movies return recommended_movies # Example usage recommend_movies(1)
In this example, we load a movie ratings dataset and define the rating scale. Next, we load the dataset using the Surprise library and build an item-based collaborative filtering model using the KNNBasic algorithm. We then train the model and define a function to recommend movies based on collaborative filtering. Finally, we use the function to recommend movies for a specific user (user ID 1).
Sentiment Analysis
Sentiment analysis is the process of determining the sentiment expressed in a piece of text, whether it is positive, negative, or neutral. Python offers several libraries for sentiment analysis, and one of the most popular ones is the Natural Language Toolkit (NLTK). NLTK provides various tools and resources for working with human language data.
Here's an example of using NLTK for sentiment analysis:
import nltk from nltk.sentiment import SentimentIntensityAnalyzer # Initialize the sentiment analyzer sia = SentimentIntensityAnalyzer() # Analyze the sentiment of a sentence sentence = "I love this movie!" sentiment = sia.polarity_scores(sentence) # Print the sentiment scores print(sentiment)
This code snippet uses the SentimentIntensityAnalyzer
class from the NLTK library to analyze the sentiment of a given sentence. The polarity_scores
method returns a dictionary with the sentiment scores, including positive, negative, neutral, and compound scores.
Named Entity Recognition
Named Entity Recognition (NER) is the process of identifying and classifying named entities in text into predefined categories such as person names, organizations, locations, medical codes, time expressions, quantities, monetary values, percentages, etc. Python offers several libraries for NER, including spaCy.
Here's an example of using spaCy for named entity recognition:
import spacy # Load the English language model nlp = spacy.load("en_core_web_sm") # Process a text text = "Apple Inc. is looking to buy a startup in the autonomous vehicle space." doc = nlp(text) # Print the named entities for entity in doc.ents: print(entity.text, entity.label_)
This code snippet uses the spaCy library to perform named entity recognition on a given text. The en_core_web_sm
model is used to process the text and identify the named entities. The named entities and their corresponding labels are then printed.
Text Classification
Text classification is the process of categorizing text into predefined categories or classes. It is a fundamental task in NLP and has various applications, such as spam detection, sentiment analysis, topic classification, and more. Python provides several libraries and tools for text classification, including scikit-learn.
Here's an example of using scikit-learn for text classification:
from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.svm import LinearSVC from sklearn.pipeline import Pipeline # Define the training data X_train = ["I love this product!", "This is terrible.", "Great experience!"] y_train = ["positive", "negative", "positive"] # Create a pipeline for text classification pipeline = Pipeline([ ("vectorizer", TfidfVectorizer()), ("classifier", LinearSVC()) ]) # Train the classifier pipeline.fit(X_train, y_train) # Predict the sentiment of a new text text = "This is amazing!" predicted_sentiment = pipeline.predict([text]) # Print the predicted sentiment print(predicted_sentiment)
This code snippet demonstrates how to use scikit-learn to perform text classification. The TfidfVectorizer
is used to convert text into numerical features, and the LinearSVC
classifier is trained on the labeled data. The trained classifier is then used to predict the sentiment of a new text.
Related Article: How to Pip Install From a Git Repo Branch
Data Engineering Techniques
The field of data engineering focuses on the process of collecting, storing, and processing large volumes of data. In this section, we will explore some advanced techniques that can be used in data engineering.
1. Data Pipelines
Data pipelines play a crucial role in data engineering as they enable the smooth and efficient flow of data between different stages of the data processing pipeline. Python offers several libraries and frameworks for building data pipelines, such as Apache Airflow and Luigi.
Let's take a look at an example of a simple data pipeline using Apache Airflow:
from airflow import DAG from airflow.operators.python_operator import PythonOperator from datetime import datetime def extract(): # Code for extracting data from a source def transform(): # Code for transforming the data def load(): # Code for loading the transformed data into a destination with DAG('data_pipeline', start_date=datetime(2022, 1, 1), schedule_interval='@daily') as dag: extract_task = PythonOperator(task_id='extract', python_callable=extract) transform_task = PythonOperator(task_id='transform', python_callable=transform) load_task = PythonOperator(task_id='load', python_callable=load) extract_task >> transform_task >> load_task
This example shows a simple data pipeline that consists of three tasks: extract, transform, and load. Each task is executed in a sequential manner, where the output of one task serves as the input for the next task.
2. Data Integration
Data integration involves combining data from multiple sources into a unified view. Python provides several libraries and tools for data integration, such as Pandas and PySpark.
Pandas is a powerful library for data manipulation and analysis. It provides a wide range of functions and methods for merging, joining, and concatenating data from different sources. Here's an example of using Pandas to merge two dataframes:
import pandas as pd df1 = pd.DataFrame({'id': [1, 2, 3], 'name': ['John', 'Jane', 'Alice']}) df2 = pd.DataFrame({'id': [2, 3, 4], 'age': [25, 30, 35]}) merged_df = pd.merge(df1, df2, on='id')
PySpark is another popular tool for data integration and processing. It provides a distributed computing framework for processing large-scale datasets. Here's an example of using PySpark to join two dataframes:
from pyspark.sql import SparkSession spark = SparkSession.builder.getOrCreate() df1 = spark.createDataFrame([(1, 'John'), (2, 'Jane'), (3, 'Alice')], ['id', 'name']) df2 = spark.createDataFrame([(2, 25), (3, 30), (4, 35)], ['id', 'age']) joined_df = df1.join(df2, on='id')
3. Data Quality
Ensuring data quality is a critical aspect of data engineering. Poor data quality can lead to inaccurate analysis and incorrect insights. Python provides several libraries and techniques for data quality assessment and improvement.
One such library is Great Expectations, which allows you to define and validate expectations about your data. It provides a set of functions and methods for checking data quality, such as checking for missing values, data types, and statistical properties.
Here's an example of using Great Expectations to check for missing values in a dataframe:
import great_expectations as ge df = pd.DataFrame({'id': [1, 2, None], 'name': ['John', None, 'Alice']}) suite = ge.dataset.PandasDataset(df).expect_column_values_to_not_be_null('id') result = suite.validate()
This example defines an expectation that the 'id' column should not have any missing values. The validate
method checks whether the data meets the defined expectations and returns a validation result.
Related Article: How to Use Python's Numpy.Linalg.Norm Function
Data Science Techniques
The field of data science focuses on extracting insights and knowledge from data using statistical and machine learning techniques. In this section, we will explore some advanced techniques that can be used in data science.
1. Dimensionality Reduction
Dimensionality reduction is a technique used to reduce the number of features or variables in a dataset. It is particularly useful when dealing with high-dimensional data. Python provides several libraries for dimensionality reduction, such as Scikit-learn and TensorFlow.
One popular method for dimensionality reduction is Principal Component Analysis (PCA). PCA transforms the original features into a new set of uncorrelated features called principal components. Here's an example of using PCA with Scikit-learn:
from sklearn.decomposition import PCA from sklearn.datasets import load_iris iris = load_iris() X = iris.data pca = PCA(n_components=2) X_reduced = pca.fit_transform(X)
This example applies PCA to the Iris dataset and reduces the dimensionality from four features to two principal components.
2. Model Evaluation
Model evaluation is an essential step in the data science workflow. It involves assessing the performance of machine learning models and selecting the best model for a given task. Python provides several libraries and techniques for model evaluation, such as Scikit-learn and Keras.
One common technique for model evaluation is cross-validation. Cross-validation involves splitting the data into multiple subsets, training the model on each subset, and evaluating its performance. Here's an example of using cross-validation with Scikit-learn:
from sklearn.datasets import load_iris from sklearn.model_selection import cross_val_score from sklearn.tree import DecisionTreeClassifier iris = load_iris() X = iris.data y = iris.target model = DecisionTreeClassifier() scores = cross_val_score(model, X, y, cv=5)
This example uses cross-validation to evaluate the performance of a decision tree classifier on the Iris dataset. The cross_val_score
function splits the data into five subsets and trains the model on each subset, returning the evaluation scores.
3. Model Deployment
Model deployment involves making trained machine learning models available for use in production environments. Python provides several libraries and frameworks for model deployment, such as Flask and TensorFlow Serving.
Flask is a lightweight web framework that can be used to create APIs for serving machine learning models. Here's an example of using Flask to deploy a model:
from flask import Flask, request, jsonify import joblib app = Flask(__name__) model = joblib.load('model.pkl') @app.route('/predict', methods=['POST']) def predict(): data = request.json prediction = model.predict(data) return jsonify(prediction.tolist()) if __name__ == '__main__': app.run()
This example defines a Flask application that serves a trained model. The /predict
endpoint accepts a JSON payload and returns the model's prediction.
These advanced techniques in data engineering and data science can help you tackle complex data problems and extract valuable insights from your data. By leveraging Python's powerful libraries and tools, you can build robust data pipelines, integrate and clean diverse datasets, and apply advanced statistical and machine learning techniques to drive data-driven decision making.
Related Article: Implementing Security Practices in Django
Optimizing Performance in Python
Python is a powerful and flexible language for data engineering and data science tasks, but it can sometimes suffer from performance limitations. In this chapter, we will explore various techniques and best practices for optimizing the performance of your Python code.
1. Use Efficient Data Structures
Choosing the right data structures can significantly impact the performance of your Python code. For example, using lists instead of sets for membership tests can result in slower lookup times. Similarly, using dictionaries instead of nested lists can improve the efficiency of searching and updating values.
Here's an example of using a dictionary to optimize a code snippet that counts the frequency of words in a text file:
# Count word frequency using a dictionary word_counts = {} with open('text_file.txt', 'r') as file: for line in file: words = line.strip().split() for word in words: if word not in word_counts: word_counts[word] = 1 else: word_counts[word] += 1
2. Utilize Vectorized Operations
Python provides powerful libraries such as NumPy and pandas that allow for efficient vectorized operations on arrays and dataframes. Vectorized operations are performed element-wise, which can dramatically improve performance compared to traditional looping constructs.
For example, consider the following code snippet that calculates the element-wise product of two arrays:
import numpy as np # Calculate element-wise product using vectorized operation array1 = np.array([1, 2, 3, 4, 5]) array2 = np.array([6, 7, 8, 9, 10]) result = array1 * array2
By utilizing vectorized operations, you can optimize the performance of your code and avoid unnecessary looping.
3. Leverage Parallel Computing
Python provides several libraries, such as multiprocessing and concurrent.futures, which allow for parallel computing. By dividing a task into smaller subtasks and executing them simultaneously on multiple processors or cores, you can significantly improve the performance of your code.
Here's an example of using the multiprocessing library to parallelize a function that performs a time-consuming task:
import multiprocessing def process_data(data): # Perform time-consuming task on data ... if __name__ == '__main__': data = [...] pool = multiprocessing.Pool() results = pool.map(process_data, data) pool.close() pool.join()
By leveraging parallel computing, you can effectively utilize the available computational resources and reduce the overall execution time of your code.
Related Article: Django 4 Best Practices: Leveraging Asynchronous Handlers for Class-Based Views
4. Implement Memory Optimization Techniques
Memory optimization is crucial when dealing with large datasets. Python provides several techniques for reducing memory usage, such as using generators instead of lists, using compression libraries like gzip or zlib, and utilizing sparse data structures.
For instance, consider the following code snippet that uses a generator to process a large file line by line, without loading it entirely into memory:
# Process large file line by line using a generator def process_large_file(file_path): with open(file_path, 'r') as file: for line in file: # Process line yield line.strip() for line in process_large_file('large_file.txt'): # Do something with each line ...
By implementing memory optimization techniques, you can efficiently handle large datasets in Python.
5. Profile and Optimize Bottlenecks
Profiling your code can help identify performance bottlenecks and areas that require optimization. Python provides built-in profiling tools, such as cProfile and line_profiler, which can be used to measure the execution time of different parts of your code.
Once you have identified the bottlenecks, you can optimize them by using techniques such as algorithmic improvements, code refactoring, or utilizing specialized libraries.
In this chapter, we have explored various techniques for optimizing the performance of your Python code. By using efficient data structures, leveraging vectorized operations, utilizing parallel computing, implementing memory optimization techniques, and profiling your code, you can significantly improve the performance of your data engineering and data science tasks.
Parallel Processing
Python provides several libraries for parallel processing, which can significantly speed up data processing tasks. One popular library is multiprocessing, which allows us to create multiple processes to execute tasks concurrently. Here's an example of using multiprocessing to process data in parallel:
import multiprocessing def process_data(data): # process data here if __name__ == '__main__': data = get_data() pool = multiprocessing.Pool() results = pool.map(process_data, data) pool.close() pool.join()
In the above code, we define a process_data
function that processes a single piece of data. We use multiprocessing.Pool
to create a pool of worker processes and the map
function to distribute the data across the workers. Finally, we close the pool and wait for all the processes to finish using pool.close()
and pool.join()
.
Distributed Computing
When dealing with very large datasets or computationally intensive tasks, parallel processing on a single machine may not be enough. In such cases, distributed computing frameworks like Apache Spark can be used to scale data pipelines across multiple machines. Python provides a powerful library called PySpark for working with Spark.
Here's an example of using PySpark to process data in a distributed manner:
from pyspark.sql import SparkSession spark = SparkSession.builder .appName("Data Processing") .getOrCreate() data = spark.read.csv("data.csv") # process data using Spark transformations and actions spark.stop()
In the above code, we create a SparkSession using SparkSession.builder
. We then read the data from a CSV file using spark.read.csv
. We can then apply various Spark transformations and actions to process the data in a distributed manner. Finally, we stop the SparkSession using spark.stop()
.
Related Article: How to Use Collections with Python
Data Streaming
In some cases, data pipelines need to process data in real-time as it arrives, rather than in batch mode. Python provides several libraries for building real-time data pipelines, such as kafka-python and pulsar-client.
Here's an example of using kafka-python to process streaming data:
from kafka import KafkaConsumer consumer = KafkaConsumer('topic', bootstrap_servers='localhost:9092') for message in consumer: # process message here
In the above code, we create a KafkaConsumer object that consumes messages from a Kafka topic. We then iterate over the messages, processing each message as it arrives.
Unit Testing
Unit testing is a technique that allows us to test individual units of code, such as functions or methods, to ensure they are working as expected. By isolating and testing these units in isolation, we can quickly identify any errors or bugs.
In Python, the unittest
module provides a framework for writing and running unit tests. Let's take a look at an example:
import unittest def add_numbers(a, b): return a + b class TestAddNumbers(unittest.TestCase): def test_add_numbers(self): result = add_numbers(2, 3) self.assertEqual(result, 5) if __name__ == '__main__': unittest.main()
In this example, we define a function add_numbers
that adds two numbers. We then create a test class TestAddNumbers
that inherits from unittest.TestCase
. Inside this class, we define a test method test_add_numbers
where we call the add_numbers
function and assert that the result is equal to the expected value.
To run the unit test, we use the unittest.main()
function. If all the tests pass, we will see an output indicating the success of the test. Otherwise, any failures or errors will be displayed, helping us identify the problematic areas in our code.
Integration Testing
Integration testing involves testing the interaction between different components or modules of our data engineering or data science project. This type of testing helps ensure that the integrated parts work together correctly and produce the desired outcomes.
In data engineering, integration testing can be performed on data pipelines or ETL (Extract, Transform, Load) processes. Data can be fed into the pipeline, and the output can be compared against the expected results.
Similarly, in data science, integration testing can involve testing the interaction between different models or algorithms. We can test if the outputs of one model are correctly used as inputs for another model.
Debugging
Debugging is the process of identifying and fixing errors or bugs in our code. It is an essential skill for data engineers and data scientists, as even a small error can lead to incorrect results or wasted resources.
Python provides several tools and techniques to help us debug our code. One commonly used tool is the print
statement. By strategically placing print
statements throughout our code, we can inspect the intermediate values of variables and identify any issues.
Another powerful debugging tool is the Python debugger (pdb
). The pdb
module allows us to set breakpoints in our code and step through it line by line, examining the values of variables at each step. This can be particularly useful when dealing with complex data transformations or model training.
Here's an example of using the Python debugger:
import pdb def add_numbers(a, b): result = a + b pdb.set_trace() # Set a breakpoint return result result = add_numbers(2, 3) print(result)
In this example, we set a breakpoint using pdb.set_trace()
inside the add_numbers
function. When the code reaches this point, it will pause execution and open the debugger prompt. We can then inspect the values of variables, execute statements, and step through the code using commands such as next
or continue
.
Related Article: How to Install Specific Package Versions With Pip in Python
Deploying Data Engineering and Data Science Solutions
Once you have built and tested your data engineering and data science solutions, the next step is to deploy them so that they can be used in production environments. Deploying these solutions involves setting up the necessary infrastructure, ensuring scalability and performance, and monitoring the deployed applications.
Infrastructure Setup
Before deploying your data engineering and data science solutions, you need to set up the necessary infrastructure. This includes provisioning servers, configuring networking, and installing the required software dependencies. There are several options available for infrastructure setup, depending on your specific needs and preferences.
One popular option is to use cloud providers such as Amazon Web Services (AWS), Google Cloud Platform (GCP), or Microsoft Azure. These cloud providers offer a wide range of services and tools that can be used to deploy and manage data engineering and data science solutions. For example, you can use AWS Elastic Beanstalk or GCP App Engine to deploy web applications, or use AWS EMR or GCP Dataproc to deploy big data processing clusters.
Another option is to use containerization technologies such as Docker and Kubernetes. With Docker, you can package your application and its dependencies into a container, which can then be deployed on any machine that has Docker installed. Kubernetes, on the other hand, provides a container orchestration platform that allows you to deploy and manage containers at scale.
Scalability and Performance
When deploying data engineering and data science solutions, it is important to ensure scalability and performance. This involves designing your applications in a way that allows them to handle increasing workloads and meet performance requirements.
One approach to achieving scalability is to use distributed computing frameworks such as Apache Spark or Apache Hadoop. These frameworks allow you to process large volumes of data in parallel across multiple machines, which can significantly improve performance and scalability.
Another approach is to use cloud services that offer auto-scaling capabilities. For example, AWS Auto Scaling allows you to automatically adjust the number of instances in a fleet based on demand, ensuring that your application can handle varying workloads.
To improve performance, you can optimize your code and algorithms, use caching mechanisms to reduce data retrieval times, and use efficient data storage solutions such as columnar databases or distributed file systems.
Monitoring
Monitoring is an essential part of deploying data engineering and data science solutions. It allows you to track the performance and health of your applications, detect and diagnose issues, and ensure that your solutions are running smoothly.
There are various tools and services available for monitoring your applications. For example, you can use open-source tools such as Prometheus or Grafana to collect and visualize metrics, or use cloud services such as AWS CloudWatch or GCP Stackdriver for monitoring and logging.
When monitoring your applications, it is important to define relevant metrics and alerts based on your specific requirements. For example, you might want to monitor resource utilization, response times, error rates, or data quality.
Related Article: How to Use 'In' in a Python If Statement
Example: Deploying a Machine Learning Model
To illustrate the deployment process, let's consider an example of deploying a machine learning model. Suppose you have built a model for sentiment analysis using Python and scikit-learn, and you want to deploy it as a web service.
First, you would need to set up a web server to host your application. You can use a cloud service such as AWS Elastic Beanstalk or GCP App Engine for this purpose. Once the web server is set up, you can deploy your application code and dependencies.
Next, you would need to expose an API endpoint that accepts input data and returns the predicted sentiment. You can use a web framework such as Flask or Django to implement this API endpoint.
Finally, you would need to monitor the performance and health of your deployed application. You can use tools such as Prometheus or AWS CloudWatch to collect and visualize metrics, and set up alerts based on predefined thresholds.
In this example, we have demonstrated the deployment process for a machine learning model. However, the deployment process may vary depending on the specific requirements and technologies used in your data engineering and data science solutions.
Deploying data engineering and data science solutions requires careful planning and consideration of factors such as infrastructure setup, scalability and performance, and monitoring. By following best practices and using appropriate tools and technologies, you can ensure that your solutions are deployed successfully and can deliver value in production environments.
Use Virtual Environments
Virtual environments are an essential tool for managing dependencies and isolating project environments. They allow you to create an isolated Python environment for each project, ensuring that the project's dependencies are kept separate from other projects. This helps to avoid conflicts between different versions of libraries and makes it easier to reproduce the project environment.
To create a virtual environment, you can use the built-in venv
module in Python. Here's an example of how to create and activate a virtual environment:
$ python3 -m venv myenv # Create a virtual environment $ source myenv/bin/activate # Activate the virtual environment
Use a Version Control System
Version control systems (VCS) such as Git are crucial for tracking changes to your codebase, collaborating with other team members, and easily reverting to previous versions if needed. It is recommended to use a VCS from the beginning of your project to ensure you have a complete history of your code.
GitHub, GitLab, and Bitbucket are popular platforms that provide hosting for Git repositories. You can create a new repository on one of these platforms and then clone it to your local machine using the following command:
$ git clone
Follow PEP 8 Style Guide
PEP 8 is the official style guide for Python code. Following consistent coding conventions improves code readability and maintainability, making it easier for others to understand and contribute to your projects. Some key recommendations from PEP 8 include:
- Use 4 spaces for indentation.
- Limit lines to a maximum of 79 characters.
- Use descriptive variable and function names.
- Use whitespace between operators and after commas.
You can use linters like Flake8 or Pylint to automatically check your code for PEP 8 compliance.
Related Article: How to Use Python's isnumeric() Method
Optimize Pandas Code
Pandas is a powerful library for data manipulation and analysis in Python. However, it can be slow when working with large datasets. To optimize your Pandas code, consider the following tips:
- Use vectorized operations instead of iterating over rows.
- Avoid unnecessary copying of data.
- Utilize the built-in functions and methods provided by Pandas.
- Use appropriate data types to reduce memory usage.
Here's an example of how to use vectorized operations in Pandas to calculate the square of each element in a column:
import pandas as pd # Create a DataFrame data = {'numbers': [1, 2, 3, 4, 5]} df = pd.DataFrame(data) # Use vectorized operation to calculate squares df['squared'] = df['numbers'] ** 2
Handle Missing Data
Missing data is a common issue in data engineering and data science projects. Pandas provides several methods to handle missing data, such as:
- Dropping rows or columns with missing values using dropna()
.
- Filling missing values with a specific value using fillna()
.
- Interpolating missing values based on existing data using interpolate()
.
Here's an example of how to drop rows with missing values in a Pandas DataFrame:
import pandas as pd # Create a DataFrame with missing values data = {'name': ['John', 'Jane', 'Alice', 'Bob'], 'age': [25, None, 30, 35]} df = pd.DataFrame(data) # Drop rows with missing values df = df.dropna()