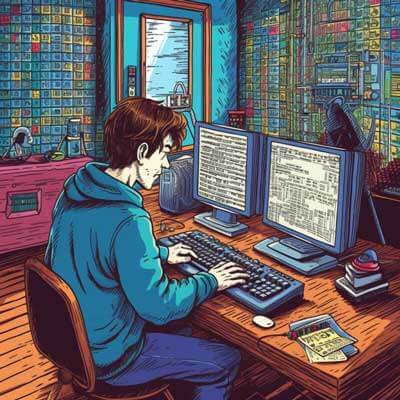
Table of Contents
Getting Started with Python Async Programming
Python Async Programming is a powerful paradigm that allows you to write concurrent and efficient code, making it ideal for handling I/O-bound tasks such as network requests or file operations. In this chapter, we will explore the basics of Python Async Programming and how you can leverage it to write more responsive and efficient applications.
Related Article: How To Iterate Over Rows In Pandas Dataframe
Understanding Asynchronous Programming
Before diving into Python Async Programming, let's first understand what asynchronous programming is. In traditional synchronous programming, a program executes each line of code sequentially, waiting for each operation to complete before moving on to the next one. Asynchronous programming, on the other hand, allows multiple operations to be executed concurrently, without waiting for each operation to finish before starting the next one.
This concurrent execution is achieved by utilizing non-blocking I/O operations and event-driven programming. Instead of waiting for an I/O operation to complete, the program can continue executing other tasks in the meantime, making efficient use of system resources.
Introducing Python's asyncio Module
Python provides the asyncio module as part of the standard library to support asynchronous programming. asyncio is built on top of coroutines, which are special functions that can be paused and resumed to allow concurrent execution. These coroutines are managed by an event loop, which schedules and executes the tasks.
To get started with asyncio, you need to create an event loop and run your tasks within it. Here's a basic example:
import asyncio async def hello(): print("Hello") await asyncio.sleep(1) print("World") loop = asyncio.get_event_loop() loop.run_until_complete(hello())
In this example, we define a coroutine hello()
that prints "Hello", waits for 1 second using asyncio.sleep()
, and then prints "World". We use the run_until_complete()
method of the event loop to run the coroutine until it's complete.
Awaitables and Futures
In Python Async Programming, you often need to wait for the result of an asynchronous operation to proceed with your code. You can use await
to pause the execution of a coroutine until the result is available. Awaitables are objects that can be awaited, such as coroutines, Tasks, and Futures.
Futures are special objects that represent the result of an asynchronous operation that hasn't completed yet. You can think of them as placeholders for the result. Here's an example of using a Future:
import asyncio async def get_data(): await asyncio.sleep(1) return "Data" async def main(): future = asyncio.Future() asyncio.ensure_future(get_data()) await future print(future.result()) loop = asyncio.get_event_loop() loop.run_until_complete(main())
In this example, we define a coroutine get_data()
that returns "Data" after waiting for 1 second. We create a Future object future
, schedule the execution of get_data()
using asyncio.ensure_future()
, and then await the completion of the Future. Finally, we print the result using future.result()
.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Building Asynchronous Applications
Python Async Programming allows you to build efficient and scalable applications by combining multiple coroutines and tasks. You can use features like asyncio.gather()
to execute multiple coroutines concurrently and wait for all of them to complete.
Here's an example of using asyncio.gather()
:
import asyncio async def task1(): await asyncio.sleep(1) print("Task 1 completed") async def task2(): await asyncio.sleep(2) print("Task 2 completed") async def main(): await asyncio.gather(task1(), task2()) loop = asyncio.get_event_loop() loop.run_until_complete(main())
In this example, we define two coroutines task1()
and task2()
, each waiting for a different amount of time. In the main()
coroutine, we use asyncio.gather()
to execute both tasks concurrently. As a result, the program prints the completion messages of both tasks.
Using Async and Await
Python's async
and await
keywords allow you to write asynchronous code that looks and behaves like synchronous code. This makes it much easier to write and understand asynchronous programs, especially for beginners. In this chapter, we will explore how to use async
and await
to write asynchronous code in Python.
Async Functions
To define an asynchronous function, you simply need to add the async
keyword before the function definition. An asynchronous function can contain one or more await
expressions, which allow the function to pause and resume execution while waiting for a result from another coroutine or a future.
Here's an example of an async function that simulates a network request using the asyncio.sleep
function:
import asyncio async def fetch_data(url): print("Fetching data from:", url) await asyncio.sleep(1) # Simulate network request print("Data fetched from:", url) return "Data from " + url
In this example, the fetch_data
function is defined as an async function. It uses the await
keyword to pause execution while waiting for the asyncio.sleep
function to complete. Once the sleep is finished, the function resumes execution and returns a result.
Await Expressions
An await
expression can only be used inside an async function. It allows you to pause the execution of the function until a coroutine or a future is complete. When an await
expression is encountered, the function is suspended, and control is returned to the event loop until the awaited object is ready.
Here's an example of using the await
keyword to wait for the result of an async function:
import asyncio async def main(): result = await fetch_data("https://example.com") print("Received data:", result) asyncio.run(main())
In this example, the main
function is defined as an async function. It uses the await
keyword to wait for the result of the fetch_data
function. Once the data is fetched, the function resumes execution and prints the received data.
Related Article: How to do Incrementing in Python
Running Async Code
To run async code, you need an event loop. The event loop is responsible for executing coroutines and managing their execution order. In Python, the asyncio
module provides an event loop and other tools for working with asynchronous code.
Here's an example of running an async function using the asyncio.run
function:
import asyncio async def main(): result = await fetch_data("https://example.com") print("Received data:", result) asyncio.run(main())
In this example, the asyncio.run
function is used to run the main
async function. It creates a new event loop, runs the function, and then closes the event loop.
Benefits of Async and Await
Using async
and await
in your code offers several benefits:
- Simplified syntax: Async and await allow you to write asynchronous code that looks and behaves like synchronous code, making it easier to understand and maintain.
- Improved performance: By allowing functions to pause and resume execution, async and await enable efficient utilization of system resources, leading to improved performance.
- Concurrency and parallelism: Async and await make it easier to write concurrent and parallel code, allowing you to perform multiple tasks simultaneously without blocking the execution of other tasks.
- Compatibility with other libraries: Many popular Python libraries support async and await, allowing you to integrate asynchronous code seamlessly into your projects.
In this chapter, we explored how to use async
and await
to write asynchronous code in Python. We learned about async functions, await expressions, and how to run async code using the event loop. Using async and await, you can write efficient and concurrent code that takes advantage of Python's async capabilities.
Handling Multiple Tasks Concurrently
One of the main advantages of async programming in Python is the ability to handle multiple tasks concurrently. This means that you can execute multiple tasks simultaneously without blocking the main thread of execution. This is extremely useful when dealing with I/O-bound tasks, such as making multiple API requests or fetching data from multiple sources.
In Python, there are several ways to handle multiple tasks concurrently. One common approach is to use the asyncio.gather()
function, which allows you to run multiple coroutines concurrently and wait for all of them to complete. Here's an example:
import asyncio async def task1(): print("Task 1 started") await asyncio.sleep(2) print("Task 1 completed") async def task2(): print("Task 2 started") await asyncio.sleep(1) print("Task 2 completed") async def main(): await asyncio.gather(task1(), task2()) asyncio.run(main())
In this example, we define two coroutines task1()
and task2()
, each representing a separate task. We then define a main()
coroutine, which uses asyncio.gather()
to run both tasks concurrently. When we call asyncio.run(main())
, the tasks will start running concurrently. The output will be:
Task 1 started Task 2 started Task 2 completed Task 1 completed
As you can see, both tasks start running immediately, and the main thread doesn't block while waiting for them to complete.
Another way to handle multiple tasks concurrently is by using asyncio.create_task()
. This function allows you to create a task for each coroutine and run them concurrently. Here's an example:
import asyncio async def task1(): print("Task 1 started") await asyncio.sleep(2) print("Task 1 completed") async def task2(): print("Task 2 started") await asyncio.sleep(1) print("Task 2 completed") async def main(): task1_obj = asyncio.create_task(task1()) task2_obj = asyncio.create_task(task2()) await task1_obj await task2_obj asyncio.run(main())
In this example, we create two tasks using asyncio.create_task()
and then await
each task separately in the main()
coroutine. The output will be the same as the previous example:
Task 1 started Task 2 started Task 2 completed Task 1 completed
Both examples demonstrate how to handle multiple tasks concurrently using async programming in Python. By running tasks concurrently, you can greatly improve the efficiency and performance of your code, especially when dealing with I/O-bound tasks.
It's important to note that handling multiple tasks concurrently doesn't necessarily mean they will complete in the exact order they were started. The order of completion depends on various factors, such as the complexity of the tasks and the system's resources. However, by using async programming, you can ensure that the tasks are executed concurrently and the main thread doesn't block while waiting for them to complete.
Creating and Using Coroutines
Coroutines are a key concept in asynchronous programming with Python. They allow us to write code that can be suspended and resumed at specific points, enabling efficient execution of multiple tasks concurrently.
To create a coroutine in Python, we use the async
keyword before the def
statement. This tells Python that the function is a coroutine and can be scheduled for execution by an event loop. Here's an example:
import asyncio async def greet(): print("Hello") await asyncio.sleep(1) print("World") asyncio.run(greet())
In this example, the greet()
function is a coroutine that prints "Hello", suspends its execution for 1 second using await asyncio.sleep(1)
, and then prints "World". To run the coroutine, we use the asyncio.run()
function.
To use a coroutine, we need to await it. The await
keyword is used to pause the execution of the current coroutine until the awaited coroutine completes. In the example above, we await the asyncio.sleep(1)
coroutine to pause the execution of the greet()
coroutine for 1 second.
Coroutines can also be used to chain multiple tasks together. We can await another coroutine inside a coroutine, allowing us to create a sequence of steps that execute one after another. Here's an example:
import asyncio async def greet(): print("Hello") await asyncio.sleep(1) await say_name("John") print("World") async def say_name(name): print(f"My name is {name}") asyncio.run(greet())
In this example, the greet()
coroutine awaits the say_name()
coroutine after waiting for 1 second. The say_name()
coroutine prints a given name.
Coroutines can also be used in combination with asyncio tasks to run multiple coroutines concurrently. We can use the asyncio.create_task()
function to create tasks for our coroutines and then await those tasks using await
. Here's an example:
import asyncio async def greet(): print("Hello") await asyncio.sleep(1) await say_name("John") print("World") async def say_name(name): print(f"My name is {name}") async def main(): task1 = asyncio.create_task(greet()) task2 = asyncio.create_task(greet()) await task1 await task2 asyncio.run(main())
In this example, we create two tasks using asyncio.create_task()
for the greet()
coroutine, and then await those tasks using await
. This allows the two coroutines to run concurrently.
Coroutines are a powerful feature in Python that enable us to write efficient and concise asynchronous code. By using the async
and await
keywords, we can create coroutines, await other coroutines, and run multiple coroutines concurrently using asyncio tasks.
Related Article: How To Check If a File Exists In Python
Working with Asyncio Event Loops
In asynchronous programming with Python, the asyncio
library provides a powerful way to manage concurrent tasks. At the heart of asyncio
is the concept of an event loop, which is responsible for scheduling and executing coroutines.
An event loop is an object that runs an asynchronous program by continuously checking for and executing pending tasks. It keeps track of all the coroutines and manages their execution, making sure that they run in an efficient and non-blocking manner.
To use an event loop in your Python program, you first need to create an instance of the asyncio
event loop class. Here's an example:
import asyncio # Create an event loop loop = asyncio.get_event_loop() # Run the event loop loop.run_forever()
In this example, we import the asyncio
module and then create an event loop using the get_event_loop()
function. We store the event loop in a variable called loop
. Finally, we call the run_forever()
method on the event loop to start the execution of the program.
Once you have an event loop, you can schedule coroutines to be executed by the event loop. The asyncio
library provides various functions for this purpose, such as loop.create_task()
and loop.run_until_complete()
.
Here's an example that demonstrates how to schedule a coroutine to be executed by the event loop:
import asyncio # Create an event loop loop = asyncio.get_event_loop() # Define a coroutine async def my_coroutine(): print("Hello, async world!") # Schedule the coroutine to be executed loop.create_task(my_coroutine()) # Run the event loop until all tasks are complete loop.run_until_complete()
In this example, we define a coroutine called my_coroutine()
that simply prints a message. We then use the loop.create_task()
function to schedule the coroutine to be executed by the event loop.
After scheduling the coroutine, we call the loop.run_until_complete()
method to run the event loop until all tasks are complete. This ensures that the program doesn't exit before the coroutine has finished executing.
The event loop also provides methods for managing and controlling the execution of coroutines. For example, you can use the loop.call_later()
method to schedule a callback function to be executed after a certain delay, or the loop.call_soon()
method to schedule a callback function to be executed in the next iteration of the event loop.
Here's an example that demonstrates how to use these methods:
import asyncio # Create an event loop loop = asyncio.get_event_loop() # Define a callback function def callback(): print("Callback function called") # Schedule the callback function to be executed after 2 seconds loop.call_later(2, callback) # Schedule the callback function to be executed in the next iteration of the event loop loop.call_soon(callback) # Run the event loop loop.run_forever()
In this example, we define a callback function called callback()
that simply prints a message. We then use the loop.call_later()
method to schedule the callback function to be executed after a delay of 2 seconds.
We also use the loop.call_soon()
method to schedule the callback function to be executed in the next iteration of the event loop, without any delay.
By using event loops and coroutines, you can write efficient and scalable asynchronous programs in Python. The asyncio
library provides a powerful and flexible framework for working with event loops, making it easier to write concurrent code that takes full advantage of modern hardware and network resources.
Synchronizing Tasks with Semaphores
In asynchronous programming, it's common to have multiple tasks running concurrently. However, there may be situations where you need to limit the number of tasks that can run simultaneously. This is where semaphores come in handy.
A semaphore is a synchronization primitive that allows a fixed number of threads or coroutines to access a shared resource simultaneously. It maintains a count of available resources and blocks or releases tasks based on that count.
Python provides the asyncio.Semaphore
class as part of the asyncio
module, which can be used to synchronize tasks in an asynchronous program.
To use a semaphore, you first create an instance of the Semaphore
class, specifying the maximum number of tasks that can acquire the semaphore at the same time. Then, you can use the acquire()
method to acquire the semaphore and the release()
method to release it.
Here's an example that demonstrates how to use a semaphore to limit the number of concurrent tasks:
import asyncio async def worker(semaphore): async with semaphore: print('Task started') await asyncio.sleep(1) print('Task finished') async def main(): semaphore = asyncio.Semaphore(2) # Allow only 2 tasks to run concurrently tasks = [] for _ in range(5): task = asyncio.create_task(worker(semaphore)) tasks.append(task) await asyncio.gather(*tasks) asyncio.run(main())
In this example, we define a worker
coroutine that simulates some work by sleeping for 1 second. We create a semaphore with a maximum count of 2, meaning only two tasks can run concurrently. We then create 5 tasks and add them to a list.
By using the async with semaphore
syntax, the worker
coroutine acquires the semaphore before starting its work and releases it when it finishes. The acquire()
and release()
methods are called implicitly when entering and exiting the async with
block.
When we run the main
coroutine using asyncio.run()
, only two tasks will be allowed to run simultaneously due to the semaphore. The output will show that tasks are started and finished in batches of two.
Using semaphores can be useful in scenarios where you want to limit the number of concurrent network requests, database connections, or any other resource-intensive tasks.
Keep in mind that semaphores should be used with caution as they can introduce potential bottlenecks and impact performance if not used appropriately. It's important to carefully determine the optimal number of concurrent tasks based on the available system resources.
Next, we'll explore another approach to synchronize tasks using event objects.
Dealing with Timeouts and Delays
In asynchronous programming, it's common to encounter situations where you need to handle timeouts and delays. Whether you are waiting for a response from a remote server or want to introduce a delay in your code, Python provides several ways to handle these scenarios effectively.
Timeouts
When making requests to external services or performing long-running operations, it's crucial to handle timeouts to avoid blocking the execution of your program indefinitely. Python's async ecosystem offers a few options to handle timeouts gracefully.
One approach is to use the asyncio.wait_for
function, which allows you to set a maximum time to wait for a coroutine to complete. If the coroutine takes longer than the specified timeout, a asyncio.TimeoutError
is raised, and you can handle it accordingly. Here's an example:
import asyncio async def fetch_data(): await asyncio.sleep(5) # Simulating a long-running task try: await asyncio.wait_for(fetch_data(), timeout=3) except asyncio.TimeoutError: print("The operation timed out")
In this example, fetch_data
simulates a long-running task using asyncio.sleep
. We set a timeout of 3 seconds for the wait_for
function. If the coroutine takes longer than 3 seconds to complete, a TimeoutError
is raised, and we handle it by printing a message.
Another option is to use the asyncio.shield
function in combination with a timeout
context manager from the async_timeout
package. This approach allows you to protect specific sections of your code from being interrupted by timeouts. Here's an example:
import asyncio import async_timeout async def fetch_data(): await asyncio.sleep(5) # Simulating a long-running task async def perform_operation(): async with async_timeout.timeout(3): await asyncio.shield(fetch_data()) try: await perform_operation() except asyncio.TimeoutError: print("The operation timed out")
In this example, the perform_operation
function protects the fetch_data
coroutine from being interrupted by a timeout. If the fetch_data
coroutine takes longer than 3 seconds to complete, a TimeoutError
is raised, and we handle it by printing a message.
Related Article: How to Normalize a Numpy Array to a Unit Vector in Python
Delays
Sometimes you may need to introduce delays between operations in your asynchronous code. This can be useful when you want to throttle requests to an external service or simulate real-world scenarios. Python provides the asyncio.sleep
function to introduce delays in your code. Here's an example:
import asyncio async def delay_example(): print("Before delay") await asyncio.sleep(2) print("After delay") await delay_example()
In this example, the delay_example
coroutine prints a message, waits for 2 seconds using asyncio.sleep
, and then prints another message. Running this code will demonstrate a delay of 2 seconds between the two print statements.
Remember, delays in asynchronous code are non-blocking, meaning that other coroutines can continue execution while a delay is in progress.
Implementing Parallel Processing with Asyncio
One of the key advantages of using asyncio in Python is the ability to implement parallel processing. By leveraging the asynchronous nature of asyncio, we can perform multiple tasks concurrently and take advantage of the full processing power of our machine.
To implement parallel processing with asyncio, we need to understand a few key concepts: coroutines, event loops, and tasks.
Coroutines are essentially functions that can be paused and resumed. They are defined using the async def
syntax and can be awaited inside other coroutines. In asyncio, coroutines are the building blocks for implementing concurrent operations.
The event loop is the core component of asyncio. It is responsible for managing and executing coroutines. The event loop schedules coroutines for execution and ensures that they are executed in an efficient manner. We can think of the event loop as a coordinator that keeps track of all the tasks and manages their execution.
Tasks are units of work that are managed by the event loop. They encapsulate coroutines and represent the progress of their execution. We can create tasks using the asyncio.create_task()
function and add them to the event loop for execution.
Now that we have a basic understanding of these concepts, let's see how we can implement parallel processing using asyncio.
First, we need to create an event loop using the asyncio.get_event_loop()
function:
import asyncio loop = asyncio.get_event_loop()
Next, we define our coroutines. Each coroutine represents a task that we want to execute concurrently. For example, let's say we have two coroutines that perform some CPU-intensive calculations:
import time async def calculate_sum(): # Simulate a CPU-intensive calculation print("Calculating sum...") total = 0 for i in range(10000000): total += i print("Sum calculated!") async def calculate_product(): # Simulate another CPU-intensive calculation print("Calculating product...") product = 1 for i in range(1, 100000): product *= i print("Product calculated!")
Now, we can create tasks for our coroutines and add them to the event loop:
task1 = loop.create_task(calculate_sum()) task2 = loop.create_task(calculate_product()) loop.run_until_complete(asyncio.gather(task1, task2))
In the above code, we use the create_task()
function to create tasks for our coroutines. We then use the gather()
function to schedule the tasks for execution in the event loop. The run_until_complete()
function is used to run the event loop until all tasks are completed.
When we run the above code, we will see the output of both coroutines interleaved, indicating that they are executed concurrently:
Calculating sum... Calculating product... Sum calculated! Product calculated!
By implementing parallel processing with asyncio, we can significantly improve the performance of our applications that involve CPU-intensive tasks. However, it's important to note that asyncio is not suitable for all types of parallel processing. If our tasks involve I/O operations, such as network requests or file operations, we should consider using asynchronous I/O libraries like aiohttp or aiofiles.
In this chapter, we learned how to implement parallel processing with asyncio using coroutines, event loops, and tasks. By leveraging asyncio's asynchronous nature, we can take full advantage of our machine's processing power and improve the performance of our applications.
Building a Web Scraper using Asyncio
Web scraping is a common task in which data is extracted from websites. Python provides several libraries for web scraping, but using Asyncio can greatly improve the performance of your scraper by allowing multiple requests to be made simultaneously.
What is Asyncio?
Asyncio is a Python library that provides a way to write asynchronous code using coroutines, multiplexing I/O access over sockets and other resources, running network clients and servers, and other related primitives. It allows you to write concurrent code more easily by using the async
and await
keywords.
Related Article: How to Pretty Print Nested Dictionaries in Python
Installing Dependencies
Before we start building our web scraper, we need to install the necessary dependencies. We will be using the aiohttp
library for making HTTP requests and beautifulsoup4
for parsing the HTML response.
To install the dependencies, you can use pip:
pip install aiohttp beautifulsoup4
Writing the Web Scraper
Let's start by importing the necessary modules:
import asyncio import aiohttp from bs4 import BeautifulSoup
Next, we'll define a function that will be responsible for making the HTTP request and parsing the HTML response:
async def scrape_website(url): async with aiohttp.ClientSession() as session: async with session.get(url) as response: html = await response.text() soup = BeautifulSoup(html, 'html.parser') # Parse the HTML and extract the data you need # ...
In this example, we're using the aiohttp.ClientSession
to make an asynchronous HTTP request to the specified URL. We then use the response.text()
method to retrieve the HTML content of the page. Finally, we use BeautifulSoup
to parse the HTML and extract the data we need.
Now, let's create a list of URLs that we want to scrape:
urls = [ 'https://example.com/', 'https://example.com/page1', 'https://example.com/page2', # Add more URLs here ]
To scrape these URLs concurrently, we can use the asyncio.gather
function:
async def scrape_websites(urls): tasks = [] for url in urls: tasks.append(asyncio.create_task(scrape_website(url))) await asyncio.gather(*tasks)
In this example, we're creating a list of tasks, where each task represents a call to the scrape_website
function with a different URL. We then use asyncio.gather
to run all the tasks concurrently.
Finally, we can run our web scraper by calling the scrape_websites
function:
if __name__ == '__main__': asyncio.run(scrape_websites(urls))
Running the Web Scraper
To run the web scraper, simply execute the Python script:
python scraper.py
The scraper will make concurrent requests to the specified URLs and extract the data you need from each page.
Integrating Asyncio in Real-World Applications
Now that you have learned the basics of asyncio and how to write asynchronous code, it's time to explore how to integrate asyncio into real-world applications. In this chapter, we will discuss some common use cases and demonstrate how asyncio can be used to improve the performance and scalability of your applications.
Related Article: How to Use Named Tuples in Python
Real-Time Applications
Asyncio is also well-suited for building real-time applications, such as chat servers or streaming services. These applications often require handling a large number of concurrent connections, which asyncio excels at.
import asyncio import websockets async def handle_connection(websocket, path): while True: message = await websocket.recv() # Process the message await websocket.send('Processed: ' + message) start_server = websockets.serve(handle_connection, 'localhost', 8765) asyncio.get_event_loop().run_until_complete(start_server) asyncio.get_event_loop().run_forever()
In this example, we use the websockets
library to create a WebSocket server. The handle_connection
function is called whenever a new connection is established. Inside the function, we continuously receive messages from the client using await websocket.recv()
, process the message, and send a response back using await websocket.send()
.
Database Operations
When working with databases, asyncio can be used to perform concurrent database operations, improving the overall performance of your application. Many database libraries provide asynchronous support, allowing you to interact with the database without blocking the event loop.
import asyncio import aiomysql async def main(): conn = await aiomysql.connect(host='localhost', port=3306, user='root', password='password', db='mydatabase') cursor = await conn.cursor() await cursor.execute('SELECT * FROM users') rows = await cursor.fetchall() for row in rows: print(row) await cursor.close() conn.close() loop = asyncio.get_event_loop() loop.run_until_complete(main())
In this example, we use the aiomysql
library to connect to a MySQL database. We define an async
function main
that establishes a connection, creates a cursor, and executes a SQL query to fetch all the rows from the users
table. We then iterate over the rows and print them. Finally, we close the cursor and the connection.
Optimizing Performance in Async Programming
In async programming, optimizing performance is crucial to ensure efficient execution of tasks and minimize resource usage. Here are some techniques to consider when aiming to improve the performance of your async Python code:
1. Use Async Libraries and Frameworks:
Utilize async libraries and frameworks, such as asyncio in Python, which provide built-in support for async programming. These libraries offer various tools and utilities to simplify async programming and enhance performance.
2. Use Asynchronous I/O:
Leverage asynchronous I/O operations to improve performance. Instead of waiting for a resource to become available, async I/O allows your program to continue executing other tasks. This can be achieved by using async functions and the "await" keyword to wait for I/O operations to complete without blocking the execution of other tasks.
Here's an example of using async I/O with the aiohttp library to make asynchronous HTTP requests:
import aiohttp import asyncio async def fetch(session, url): async with session.get(url) as response: return await response.text() async def main(): async with aiohttp.ClientSession() as session: tasks = [fetch(session, 'https://example.com') for _ in range(10)] responses = await asyncio.gather(*tasks) print(responses) asyncio.run(main())
In this example, multiple HTTP requests are made asynchronously using the aiohttp library. The responses are then collected using the asyncio.gather()
function, which waits for all the tasks to complete.
3. Consider Parallelism:
In some cases, you may have independent tasks that can be executed in parallel to further improve performance. Python provides the asyncio.gather()
method to concurrently execute multiple coroutines. By utilizing parallelism, you can leverage the full potential of multiple CPU cores and reduce the overall execution time.
4. Optimize Resource Usage:
Make sure to optimize the usage of system resources, such as memory and CPU, to improve performance. Avoid unnecessary blocking operations, minimize context switching, and release resources promptly when they are no longer needed.
5. Profile and Benchmark:
Profile and benchmark your code to identify performance bottlenecks and areas that can be optimized. Tools like cProfile
and timeit
can help you measure the performance of your async code and provide insights into areas that need improvement.
6. Leverage C Extensions:
Consider using C extensions or libraries for computationally intensive tasks. Python provides interfaces, such as the ctypes
module, to interact with C/C++ libraries, allowing you to leverage their performance benefits in your async code.
By applying these optimization techniques, you can significantly enhance the performance of your async Python code and achieve faster execution times with reduced resource usage. Remember to measure and profile your code to ensure that the optimizations you implement are effective.
Debugging and Error Handling in Async Programming
Debugging and error handling are crucial aspects of any programming language, and async programming in Python is no exception. Asynchronous programming introduces some unique challenges when it comes to debugging and handling errors, but with the right tools and techniques, you can effectively troubleshoot and fix issues in your async code.
Related Article: How to Convert String to Bytes in Python 3
Debugging Async Code
Debugging async code can be more challenging than debugging synchronous code because of the non-linear execution flow. However, Python provides several tools that can help you in this process.
One useful technique is to use print
statements strategically to trace the execution flow and identify any issues. You can print the values of variables or log messages at various points in your async code to understand what's happening.
Another powerful tool for debugging async code is the asyncio.get_event_loop().set_debug(True)
function. Enabling debug mode in the event loop can provide you with detailed information about the execution of your async code, including any warnings or errors.
Additionally, Python provides a debugger called pdb that you can use to step through your async code and inspect variables at each step. You can set breakpoints in your code using the import pdb; pdb.set_trace()
statement. This will start the debugger and allow you to interactively debug your code.
Error Handling in Async Code
Handling errors in async code is essential to ensure that your program behaves correctly and gracefully recovers from any unexpected situations. Python provides a variety of mechanisms for error handling in async programming.
One common approach is to use try-except
blocks to catch and handle exceptions. You can wrap your async code within a try
block and use except
blocks to catch specific exceptions and perform appropriate error handling.
Here's an example of how to use try-except
blocks in async code:
import asyncio async def divide(a, b): try: result = a / b print(f"The result is: {result}") except ZeroDivisionError: print("Error: Cannot divide by zero") async def main(): await divide(10, 5) await divide(10, 0) asyncio.run(main())
In the above example, the divide
function attempts to divide two numbers. If a ZeroDivisionError
occurs, it is caught in the except
block, and an appropriate error message is displayed.
Another technique for error handling in async code is to use the asyncio.create_task()
function to wrap your coroutines in tasks. This allows you to handle exceptions thrown by the coroutines independently and implement specific error handling logic for each task.
import asyncio async def task1(): raise ValueError("Something went wrong in task1") async def task2(): raise RuntimeError("An error occurred in task2") async def main(): try: task1 = asyncio.create_task(task1()) task2 = asyncio.create_task(task2()) await asyncio.gather(task1, task2) except ValueError as e: print(f"Error in task1: {str(e)}") except RuntimeError as e: print(f"Error in task2: {str(e)}") asyncio.run(main())
In this example, both task1
and task2
are wrapped in tasks using the asyncio.create_task()
function. If an exception is raised in either task, it can be caught and handled separately in the except
blocks.
By effectively debugging and handling errors in your async code, you can improve the reliability and stability of your programs. Remember to use tools like print
statements, the debug mode in the event loop, and the pdb
debugger, along with techniques like try-except
blocks and asyncio.create_task()
for error handling.
Exploring Advanced Techniques in Async Programming
In the previous chapter, we covered the basics of async programming in Python and how to use the asyncio
module to write asynchronous code. Now, let's dive deeper into some advanced techniques that will help you write more efficient and powerful async programs.
1. Using Asyncio Event Loops
The event loop is the core component of the asyncio
module. It manages the execution of coroutines and tasks in an async program. By default, asyncio
provides a default event loop that you can use. However, in some cases, you may need to create and manage your own event loop.
To create a custom event loop, you can use the asyncio.get_event_loop()
function, which returns the current event loop. You can then use the loop to execute coroutines and tasks using the run_until_complete()
or run_forever()
methods.
Here's an example of creating and using a custom event loop:
import asyncio loop = asyncio.get_event_loop() async def my_coroutine(): # Your coroutine code here task = loop.create_task(my_coroutine()) loop.run_until_complete(task)
Related Article: Python Numpy.where() Tutorial
2. Concurrent Futures and Executors
Python's concurrent.futures
module provides a high-level interface for asynchronously executing tasks in parallel. It includes the ThreadPoolExecutor
and ProcessPoolExecutor
classes, which allow you to execute functions in separate threads or processes, respectively.
These classes are useful when you have CPU-bound tasks that can benefit from parallel execution. To use them with asyncio
, you can wrap the executor in an asyncio
event loop using the run_in_executor()
method.
Here's an example of using a ThreadPoolExecutor
to execute a function asynchronously:
import asyncio from concurrent.futures import ThreadPoolExecutor async def my_coroutine(): # Your coroutine code here def blocking_function(): # Your blocking code here loop = asyncio.get_event_loop() executor = ThreadPoolExecutor() task = loop.run_in_executor(executor, blocking_function)
3. Coroutine Chaining and Composition
In async programming, it's common to chain coroutines together or compose them to build more complex workflows. This can be done using the asyncio.ensure_future()
function to schedule coroutines as tasks and the await
keyword to wait for their completion.
Here's an example of chaining coroutines:
import asyncio async def coroutine_1(): # Your code here async def coroutine_2(): # Your code here async def main(): await coroutine_1() await coroutine_2() loop = asyncio.get_event_loop() loop.run_until_complete(main())
4. Asynchronous Context Managers
Python 3.7 introduced support for asynchronous context managers, which allow you to use the async with
statement to manage resources asynchronously. This is especially useful when working with I/O operations that require resource cleanup.
To define an asynchronous context manager, you can use the asyncio.AbstractAsyncContextManager
base class and implement the __aenter__()
and __aexit__()
methods.
Here's an example of using an asynchronous context manager:
import asyncio class AsyncContextManager: async def __aenter__(self): # Acquire resources asynchronously return resource async def __aexit__(self, exc_type, exc_val, exc_tb): # Cleanup resources asynchronously async def main(): async with AsyncContextManager() as resource: # Use the resource asynchronously loop = asyncio.get_event_loop() loop.run_until_complete(main())
These advanced techniques will help you write more efficient and flexible async programs in Python. Experiment with them and explore the asyncio
module further to discover more powerful features that can enhance your async programming skills.