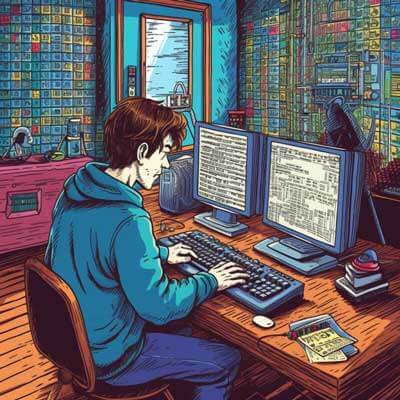
Table of Contents
What are some arithmetic operations I can perform in bash?
Bash provides various arithmetic operations that you can use in your scripts. Some of the commonly used arithmetic operations include addition, subtraction, multiplication, division, modulus, and exponentiation.
Here is a table that shows the syntax for these arithmetic operations:
| Operation | Syntax |
|--------------|-------------------------------|
| Addition | result=$((num1 + num2))
|
| Subtraction | result=$((num1 - num2))
|
| Multiplication | result=$((num1 * num2))
|
| Division | result=$((num1 / num2))
|
| Modulus | result=$((num1 % num2))
|
| Exponentiation | result=$((num1 ** num2))
|
Here is an example of a bash script that performs arithmetic operations:
#!/bin/bash num1=10 num2=5 addition=$((num1 + num2)) subtraction=$((num1 - num2)) multiplication=$((num1 * num2)) division=$((num1 / num2)) modulus=$((num1 % num2)) exponentiation=$((num1 ** num2)) echo "Addition: $addition" echo "Subtraction: $subtraction" echo "Multiplication: $multiplication" echo "Division: $division" echo "Modulus: $modulus" echo "Exponentiation: $exponentiation"
When you run this script, it will output:
Addition: 15 Subtraction: 5 Multiplication: 50 Division: 2 Modulus: 0 Exponentiation: 100000
Related Article: How to Sync Local and Remote Directories with Rsync
How can I add numbers in a bash script?
In bash, you can add numbers using the arithmetic expansion feature. This feature allows you to perform arithmetic operations within double parentheses (( ))
or by using the $(( ))
syntax.
Here is an example of a bash script that adds two numbers:
#!/bin/bash num1=10 num2=5 sum=$((num1 + num2)) echo "Sum: $sum"
When you run this script, it will output:
Sum: 15
How can I pass inputs to a bash script?
In a bash script, you can pass inputs to the script by using command-line arguments. Command-line arguments are values or parameters passed to a script when it is executed. The arguments can be accessed within the script using special variables. The first argument is accessed using $1
, the second argument using $2
, and so on. If you have more than 9 arguments, you can access them using curly braces: ${10}
, ${11}
, and so on.
Here is an example of a bash script that takes two arguments and displays them:
#!/bin/bash echo "First argument: $1" echo "Second argument: $2"
When you run this script with two arguments, like this: ./script.sh hello world
, the output will be:
First argument: hello Second argument: world
How do I prompt the user for input in a bash script?
In bash, you can prompt the user for input using the read
command. The read
command reads a line from the input and assigns it to a variable.
Here is an example of a bash script that prompts the user for their name and displays a greeting:
#!/bin/bash echo "Enter your name:" read name echo "Hello, $name!"
When you run this script, it will prompt you to enter your name. After you enter your name, it will display a greeting like this:
Enter your name: John Hello, John!
Related Article: How to Continue a Bash Script Post cURL Command
What is command substitution in bash?
Command substitution is a feature in bash that allows you to execute a command and replace it with its output. The output of the command is captured and can be assigned to a variable or used in an expression.
There are two syntaxes for command substitution in bash:
- $(command)
- This syntax is recommended and more readable.
-
command
- This syntax is deprecated and less readable.
Here is an example of a bash script that uses command substitution to assign the current date to a variable:
#!/bin/bash current_date=$(date +%Y-%m-%d) echo "Current date: $current_date"
When you run this script, it will output the current date in the format YYYY-MM-DD.
Can I perform calculations with command substitution in bash?
Yes, you can perform calculations with command substitution in bash. You can use the arithmetic expansion feature within the command substitution syntax to perform calculations.
Here is an example of a bash script that calculates the square of a number using command substitution:
#!/bin/bash number=5 square=$(echo "$number * $number" | bc) echo "Square of $number: $square"
In this script, the echo "$number * $number" | bc
command calculates the square of the number using the bc
command-line calculator. The result is then assigned to the square
variable using command substitution.
When you run this script, it will output:
Square of 5: 25
How do I handle multiple inputs in a bash script?
To handle multiple inputs in a bash script, you can use a combination of command-line arguments and the read
command. You can pass some inputs as command-line arguments and prompt the user for the remaining inputs using the read
command.
Here is an example of a bash script that handles multiple inputs:
#!/bin/bash echo "First name: $1" echo "Last name: $2" echo "Enter your age:" read age echo "Name: $1 $2" echo "Age: $age"
When you run this script with two command-line arguments and enter the age when prompted, it will display the name and age:
$ ./script.sh John Doe Enter your age: 25 Name: John Doe Age: 25
Are there any built-in functions for summing inputs in bash?
Bash does not have built-in functions specifically for summing inputs. However, you can write a bash script that uses a loop to iterate over the inputs and calculate the sum.
Here is an example of a bash script that sums a list of numbers passed as command-line arguments:
#!/bin/bash sum=0 for number in "$@" do sum=$((sum + number)) done echo "Sum: $sum"
When you run this script with a list of numbers as command-line arguments, it will calculate the sum and display it.
For example, if you run the script like this: ./script.sh 1 2 3 4 5
, the output will be:
Sum: 15
In this script, the for
loop iterates over the command-line arguments and adds each number to the sum
variable using arithmetic expansion. Finally, the script displays the sum.
Related Article: Adding Color to Bash Scripts in Linux
Additional Resources
- How to pass arguments to a bash script?